Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / WinForms / Managed / System / WinForms / ContextMenuStrip.cs / 1 / ContextMenuStrip.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.ComponentModel; using System.Drawing; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; ///this class is just a conceptual wrapper around ToolStripDropDownMenu. [ ComVisible(true), ClassInterface(ClassInterfaceType.AutoDispatch), DefaultEvent("Opening"), SRDescription(SR.DescriptionContextMenuStrip) ] public class ContextMenuStrip : ToolStripDropDownMenu { /// /// Summary of ContextMenuStrip. /// public ContextMenuStrip(IContainer container) : base() { // this constructor ensures ContextMenuStrip is disposed properly since its not parented to the form. if (container == null) { throw new ArgumentNullException("container"); } container.Add(this); } public ContextMenuStrip(){ } protected override void Dispose(bool disposing) { base.Dispose(disposing); } [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.ContextMenuStripSourceControlDescr) ] public Control SourceControl { [UIPermission(SecurityAction.Demand, Window=UIPermissionWindow.AllWindows)] get { return SourceControlInternal; } } // minimal Clone implementation for DGV support only. internal ContextMenuStrip Clone() { // VERY limited support for cloning. ContextMenuStrip contextMenuStrip = new ContextMenuStrip(); // copy over events contextMenuStrip.Events.AddHandlers(this.Events); contextMenuStrip.AutoClose = AutoClose; contextMenuStrip.AutoSize = AutoSize; contextMenuStrip.Bounds = Bounds; contextMenuStrip.ImageList = ImageList; contextMenuStrip.ShowCheckMargin = ShowCheckMargin; contextMenuStrip.ShowImageMargin = ShowImageMargin; // copy over relevant properties for (int i = 0; i < Items.Count; i++) { ToolStripItem item = Items[i]; if (item is ToolStripSeparator) { contextMenuStrip.Items.Add(new ToolStripSeparator()); } else if (item is ToolStripMenuItem) { ToolStripMenuItem menuItem = item as ToolStripMenuItem; contextMenuStrip.Items.Add(menuItem.Clone()); } } return contextMenuStrip; } // internal overload so we know whether or not to show mnemonics. internal void ShowInternal(Control source, Point location, bool isKeyboardActivated) { Show(source, location); // if we were activated by keyboard - show mnemonics. if (isKeyboardActivated) { ToolStripManager.ModalMenuFilter.Instance.ShowUnderlines = true; } } internal void ShowInTaskbar(int x, int y) { // we need to make ourselves a topmost window WorkingAreaConstrained = false; Rectangle bounds = CalculateDropDownLocation(new Point(x,y), ToolStripDropDownDirection.AboveLeft); Rectangle screenBounds = Screen.FromRectangle(bounds).Bounds; if (bounds.Y < screenBounds.Y) { bounds = CalculateDropDownLocation(new Point(x,y), ToolStripDropDownDirection.BelowLeft); } else if (bounds.X < screenBounds.X) { bounds = CalculateDropDownLocation(new Point(x,y), ToolStripDropDownDirection.AboveRight); } bounds = WindowsFormsUtils.ConstrainToBounds(screenBounds, bounds); Show(bounds.X, bounds.Y); } protected override void SetVisibleCore(bool visible) { if (!visible) { WorkingAreaConstrained = true; } base.SetVisibleCore(visible); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.ComponentModel; using System.Drawing; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; ///this class is just a conceptual wrapper around ToolStripDropDownMenu. [ ComVisible(true), ClassInterface(ClassInterfaceType.AutoDispatch), DefaultEvent("Opening"), SRDescription(SR.DescriptionContextMenuStrip) ] public class ContextMenuStrip : ToolStripDropDownMenu { /// /// Summary of ContextMenuStrip. /// public ContextMenuStrip(IContainer container) : base() { // this constructor ensures ContextMenuStrip is disposed properly since its not parented to the form. if (container == null) { throw new ArgumentNullException("container"); } container.Add(this); } public ContextMenuStrip(){ } protected override void Dispose(bool disposing) { base.Dispose(disposing); } [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.ContextMenuStripSourceControlDescr) ] public Control SourceControl { [UIPermission(SecurityAction.Demand, Window=UIPermissionWindow.AllWindows)] get { return SourceControlInternal; } } // minimal Clone implementation for DGV support only. internal ContextMenuStrip Clone() { // VERY limited support for cloning. ContextMenuStrip contextMenuStrip = new ContextMenuStrip(); // copy over events contextMenuStrip.Events.AddHandlers(this.Events); contextMenuStrip.AutoClose = AutoClose; contextMenuStrip.AutoSize = AutoSize; contextMenuStrip.Bounds = Bounds; contextMenuStrip.ImageList = ImageList; contextMenuStrip.ShowCheckMargin = ShowCheckMargin; contextMenuStrip.ShowImageMargin = ShowImageMargin; // copy over relevant properties for (int i = 0; i < Items.Count; i++) { ToolStripItem item = Items[i]; if (item is ToolStripSeparator) { contextMenuStrip.Items.Add(new ToolStripSeparator()); } else if (item is ToolStripMenuItem) { ToolStripMenuItem menuItem = item as ToolStripMenuItem; contextMenuStrip.Items.Add(menuItem.Clone()); } } return contextMenuStrip; } // internal overload so we know whether or not to show mnemonics. internal void ShowInternal(Control source, Point location, bool isKeyboardActivated) { Show(source, location); // if we were activated by keyboard - show mnemonics. if (isKeyboardActivated) { ToolStripManager.ModalMenuFilter.Instance.ShowUnderlines = true; } } internal void ShowInTaskbar(int x, int y) { // we need to make ourselves a topmost window WorkingAreaConstrained = false; Rectangle bounds = CalculateDropDownLocation(new Point(x,y), ToolStripDropDownDirection.AboveLeft); Rectangle screenBounds = Screen.FromRectangle(bounds).Bounds; if (bounds.Y < screenBounds.Y) { bounds = CalculateDropDownLocation(new Point(x,y), ToolStripDropDownDirection.BelowLeft); } else if (bounds.X < screenBounds.X) { bounds = CalculateDropDownLocation(new Point(x,y), ToolStripDropDownDirection.AboveRight); } bounds = WindowsFormsUtils.ConstrainToBounds(screenBounds, bounds); Show(bounds.X, bounds.Y); } protected override void SetVisibleCore(bool visible) { if (!visible) { WorkingAreaConstrained = true; } base.SetVisibleCore(visible); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
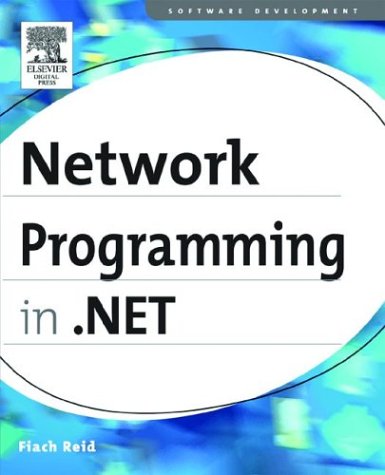
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SHA1Cng.cs
- MergeFilterQuery.cs
- SinglePageViewer.cs
- RestHandler.cs
- XNodeNavigator.cs
- MasterPageParser.cs
- TraceContextRecord.cs
- AxisAngleRotation3D.cs
- processwaithandle.cs
- MachineKeySection.cs
- EntityContainer.cs
- UrlPath.cs
- nulltextnavigator.cs
- XamlPathDataSerializer.cs
- Delegate.cs
- XmlNamespaceMapping.cs
- InOutArgumentConverter.cs
- ImageInfo.cs
- DbMetaDataFactory.cs
- ZipFileInfoCollection.cs
- StringExpressionSet.cs
- MultiBinding.cs
- TopClause.cs
- OdbcInfoMessageEvent.cs
- TrustManagerMoreInformation.cs
- EncodingFallbackAwareXmlTextWriter.cs
- CodeAttributeDeclarationCollection.cs
- CacheChildrenQuery.cs
- DataGridTable.cs
- ConsumerConnectionPoint.cs
- FileDialog_Vista_Interop.cs
- EntityDataSourceMemberPath.cs
- AutomationElementCollection.cs
- TextTreeTextElementNode.cs
- SourceLineInfo.cs
- CheckBoxList.cs
- FrameworkTemplate.cs
- RC2CryptoServiceProvider.cs
- SmiMetaDataProperty.cs
- TemplateComponentConnector.cs
- TypedReference.cs
- ButtonBase.cs
- SafeFindHandle.cs
- SqlDataSourceCache.cs
- XmlMapping.cs
- FileStream.cs
- columnmapfactory.cs
- ProjectedSlot.cs
- Exceptions.cs
- InvalidCastException.cs
- ArrayConverter.cs
- IBuiltInEvidence.cs
- SmtpFailedRecipientException.cs
- ExpressionEditorAttribute.cs
- Line.cs
- oledbmetadatacolumnnames.cs
- SamlSubject.cs
- _AcceptOverlappedAsyncResult.cs
- Verify.cs
- KeysConverter.cs
- PtsCache.cs
- AttributeQuery.cs
- TrackingDataItem.cs
- DataGridViewColumn.cs
- DispatcherExceptionFilterEventArgs.cs
- ByteAnimation.cs
- DataGridViewImageColumn.cs
- TextBoxBase.cs
- CorruptingExceptionCommon.cs
- MenuAutomationPeer.cs
- SqlServices.cs
- SettingsPropertyIsReadOnlyException.cs
- ClipboardData.cs
- ArcSegment.cs
- ConfigViewGenerator.cs
- BinaryObjectReader.cs
- AsnEncodedData.cs
- SByte.cs
- ObjectToIdCache.cs
- TreeNodeBindingCollection.cs
- ThrowHelper.cs
- PropertyMapper.cs
- DataServiceHost.cs
- MenuItem.cs
- BinHexEncoder.cs
- HttpModuleCollection.cs
- HandleExceptionArgs.cs
- ExpressionConverter.cs
- GridViewUpdatedEventArgs.cs
- XmlValidatingReader.cs
- VersionedStream.cs
- DesignerSerializationManager.cs
- LongSumAggregationOperator.cs
- XPathQilFactory.cs
- MenuItemAutomationPeer.cs
- PingReply.cs
- AdornedElementPlaceholder.cs
- NetworkInformationPermission.cs
- Inline.cs
- WindowsContainer.cs