Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / ProcessModelInfo.cs / 1305376 / ProcessModelInfo.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * ProcessInfo class */ namespace System.Web { using System.Runtime.Serialization.Formatters; using System.Threading; using System.Security.Permissions; public class ProcessModelInfo { [AspNetHostingPermission(SecurityAction.Demand, Level=AspNetHostingPermissionLevel.High)] static public ProcessInfo GetCurrentProcessInfo() { HttpContext context = HttpContext.Current; if (context == null || context.WorkerRequest == null || !(context.WorkerRequest is System.Web.Hosting.ISAPIWorkerRequestOutOfProc)) { throw new HttpException(SR.GetString(SR.Process_information_not_available)); } int dwReqExecuted = 0; int dwReqExecuting = 0; long tmCreateTime = 0; int pid = 0; int mem = 0; int iRet = UnsafeNativeMethods.PMGetCurrentProcessInfo ( ref dwReqExecuted, ref dwReqExecuting, ref mem, ref tmCreateTime, ref pid); if (iRet < 0) throw new HttpException(SR.GetString(SR.Process_information_not_available)); DateTime startTime = DateTime.FromFileTime(tmCreateTime); TimeSpan age = DateTime.Now.Subtract(startTime); return new ProcessInfo(startTime, age, pid, dwReqExecuted, ProcessStatus.Alive, ProcessShutdownReason.None, mem); } [AspNetHostingPermission(SecurityAction.Demand, Level=AspNetHostingPermissionLevel.High)] static public ProcessInfo[] GetHistory(int numRecords) { HttpContext context = HttpContext.Current; if (context == null || context.WorkerRequest == null || !(context.WorkerRequest is System.Web.Hosting.ISAPIWorkerRequestOutOfProc)) { throw new HttpException(SR.GetString(SR.Process_information_not_available)); } if (numRecords < 1) return null; int [] dwPID = new int [numRecords]; int [] dwExed = new int [numRecords]; int [] dwExei = new int [numRecords]; int [] dwPend = new int [numRecords]; int [] dwReas = new int [numRecords]; long [] tmCrea = new long [numRecords]; long [] tmDeat = new long [numRecords]; int [] mem = new int [numRecords]; int iRows = UnsafeNativeMethods.PMGetHistoryTable (numRecords, dwPID, dwExed, dwPend, dwExei, dwReas, mem, tmCrea, tmDeat); if (iRows < 0) throw new HttpException(SR.GetString(SR.Process_information_not_available)); ProcessInfo[] ret = new ProcessInfo[iRows]; for (int iter=0; iter0) age = DateTime.FromFileTime(tmDeat[iter]).Subtract(startTime); if ((dwReas[iter] & 0x0004) != 0) status = ProcessStatus.Terminated; else if ((dwReas[iter] & 0x0002) != 0) status = ProcessStatus.ShutDown; else status = ProcessStatus.ShuttingDown; if ((0x0040 & dwReas[iter]) != 0) rea = ProcessShutdownReason.IdleTimeout; else if ((0x0080 & dwReas[iter]) != 0) rea = ProcessShutdownReason.RequestsLimit; else if ((0x0100 & dwReas[iter]) != 0) rea = ProcessShutdownReason.RequestQueueLimit; else if ((0x0020 & dwReas[iter]) != 0) rea = ProcessShutdownReason.Timeout; else if ((0x0200 & dwReas[iter]) != 0) rea = ProcessShutdownReason.MemoryLimitExceeded; else if ((0x0400 & dwReas[iter]) != 0) rea = ProcessShutdownReason.PingFailed; else if ((0x0800 & dwReas[iter]) != 0) rea = ProcessShutdownReason.DeadlockSuspected; else rea = ProcessShutdownReason.Unexpected; } ret[iter] = new ProcessInfo(startTime, age, dwPID[iter], dwExed[iter], status, rea, mem[iter]); } return ret; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * ProcessInfo class */ namespace System.Web { using System.Runtime.Serialization.Formatters; using System.Threading; using System.Security.Permissions; public class ProcessModelInfo { [AspNetHostingPermission(SecurityAction.Demand, Level=AspNetHostingPermissionLevel.High)] static public ProcessInfo GetCurrentProcessInfo() { HttpContext context = HttpContext.Current; if (context == null || context.WorkerRequest == null || !(context.WorkerRequest is System.Web.Hosting.ISAPIWorkerRequestOutOfProc)) { throw new HttpException(SR.GetString(SR.Process_information_not_available)); } int dwReqExecuted = 0; int dwReqExecuting = 0; long tmCreateTime = 0; int pid = 0; int mem = 0; int iRet = UnsafeNativeMethods.PMGetCurrentProcessInfo ( ref dwReqExecuted, ref dwReqExecuting, ref mem, ref tmCreateTime, ref pid); if (iRet < 0) throw new HttpException(SR.GetString(SR.Process_information_not_available)); DateTime startTime = DateTime.FromFileTime(tmCreateTime); TimeSpan age = DateTime.Now.Subtract(startTime); return new ProcessInfo(startTime, age, pid, dwReqExecuted, ProcessStatus.Alive, ProcessShutdownReason.None, mem); } [AspNetHostingPermission(SecurityAction.Demand, Level=AspNetHostingPermissionLevel.High)] static public ProcessInfo[] GetHistory(int numRecords) { HttpContext context = HttpContext.Current; if (context == null || context.WorkerRequest == null || !(context.WorkerRequest is System.Web.Hosting.ISAPIWorkerRequestOutOfProc)) { throw new HttpException(SR.GetString(SR.Process_information_not_available)); } if (numRecords < 1) return null; int [] dwPID = new int [numRecords]; int [] dwExed = new int [numRecords]; int [] dwExei = new int [numRecords]; int [] dwPend = new int [numRecords]; int [] dwReas = new int [numRecords]; long [] tmCrea = new long [numRecords]; long [] tmDeat = new long [numRecords]; int [] mem = new int [numRecords]; int iRows = UnsafeNativeMethods.PMGetHistoryTable (numRecords, dwPID, dwExed, dwPend, dwExei, dwReas, mem, tmCrea, tmDeat); if (iRows < 0) throw new HttpException(SR.GetString(SR.Process_information_not_available)); ProcessInfo[] ret = new ProcessInfo[iRows]; for (int iter=0; iter0) age = DateTime.FromFileTime(tmDeat[iter]).Subtract(startTime); if ((dwReas[iter] & 0x0004) != 0) status = ProcessStatus.Terminated; else if ((dwReas[iter] & 0x0002) != 0) status = ProcessStatus.ShutDown; else status = ProcessStatus.ShuttingDown; if ((0x0040 & dwReas[iter]) != 0) rea = ProcessShutdownReason.IdleTimeout; else if ((0x0080 & dwReas[iter]) != 0) rea = ProcessShutdownReason.RequestsLimit; else if ((0x0100 & dwReas[iter]) != 0) rea = ProcessShutdownReason.RequestQueueLimit; else if ((0x0020 & dwReas[iter]) != 0) rea = ProcessShutdownReason.Timeout; else if ((0x0200 & dwReas[iter]) != 0) rea = ProcessShutdownReason.MemoryLimitExceeded; else if ((0x0400 & dwReas[iter]) != 0) rea = ProcessShutdownReason.PingFailed; else if ((0x0800 & dwReas[iter]) != 0) rea = ProcessShutdownReason.DeadlockSuspected; else rea = ProcessShutdownReason.Unexpected; } ret[iter] = new ProcessInfo(startTime, age, dwPID[iter], dwExed[iter], status, rea, mem[iter]); } return ret; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
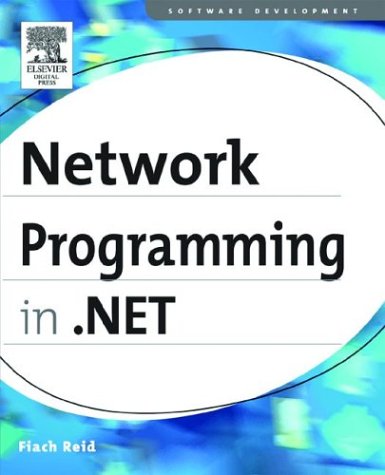
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextTabProperties.cs
- UriWriter.cs
- XXXOnTypeBuilderInstantiation.cs
- ChannelManagerBase.cs
- ToolStripPanelCell.cs
- EntityProviderFactory.cs
- Button.cs
- RecognizedAudio.cs
- MultiByteCodec.cs
- InputScopeNameConverter.cs
- TransactionFlowElement.cs
- WindowsRichEdit.cs
- EmptyReadOnlyDictionaryInternal.cs
- TypeConverterBase.cs
- Matrix3D.cs
- PointValueSerializer.cs
- Int64.cs
- X509ChainPolicy.cs
- StoreItemCollection.cs
- InputBindingCollection.cs
- ResourceContainer.cs
- MenuItem.cs
- ToolStripLocationCancelEventArgs.cs
- HostProtectionPermission.cs
- BindToObject.cs
- JsonFormatReaderGenerator.cs
- CaseInsensitiveHashCodeProvider.cs
- OSFeature.cs
- TemplateColumn.cs
- Item.cs
- OpCodes.cs
- PointKeyFrameCollection.cs
- FixedBufferAttribute.cs
- QueryCacheEntry.cs
- ConfigurationManagerInternal.cs
- PolicyReader.cs
- AVElementHelper.cs
- HtmlInputCheckBox.cs
- Operand.cs
- EventManager.cs
- QuaternionValueSerializer.cs
- HtmlInputImage.cs
- FileSecurity.cs
- ItemCollection.cs
- Assert.cs
- ConfigsHelper.cs
- HMACSHA384.cs
- AutomationElement.cs
- EntityDataReader.cs
- SortAction.cs
- Literal.cs
- ComponentGuaranteesAttribute.cs
- SplineKeyFrames.cs
- XmlChildEnumerator.cs
- EventMap.cs
- TiffBitmapEncoder.cs
- RegexTree.cs
- ProvidersHelper.cs
- XmlDataSourceDesigner.cs
- WebDisplayNameAttribute.cs
- ServiceObjectContainer.cs
- HttpCookieCollection.cs
- EntityConnectionStringBuilder.cs
- GrammarBuilderWildcard.cs
- ArgumentElement.cs
- OleCmdHelper.cs
- DataTablePropertyDescriptor.cs
- EDesignUtil.cs
- EntityAdapter.cs
- ImportCatalogPart.cs
- TraceData.cs
- NextPreviousPagerField.cs
- MetadataItem_Static.cs
- StaticDataManager.cs
- HijriCalendar.cs
- MenuItemBinding.cs
- PrimaryKeyTypeConverter.cs
- StylusLogic.cs
- BuildProviderAppliesToAttribute.cs
- CodeIdentifier.cs
- RightsManagementPermission.cs
- CurrencyManager.cs
- RangeValidator.cs
- DataContext.cs
- DoubleKeyFrameCollection.cs
- Validator.cs
- ViewBase.cs
- smtpconnection.cs
- XmlILOptimizerVisitor.cs
- IntellisenseTextBox.designer.cs
- ProfessionalColorTable.cs
- SocketException.cs
- AuthorizationRuleCollection.cs
- CodeIdentifiers.cs
- AssertSection.cs
- Floater.cs
- AccessText.cs
- complextypematerializer.cs
- AuthenticationModulesSection.cs
- DBCSCodePageEncoding.cs