Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / Data / DefaultAsyncDataDispatcher.cs / 1305600 / DefaultAsyncDataDispatcher.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Default async scheduler for data operations. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Threading; using System.Windows; using System.Windows.Data; using MS.Internal; namespace MS.Internal.Data { internal class DefaultAsyncDataDispatcher : IAsyncDataDispatcher { //----------------------------------------------------- // // Interface: IAsyncDataDispatcher // //----------------------------------------------------- ///Add a request to the dispatcher's queue void IAsyncDataDispatcher.AddRequest(AsyncDataRequest request) { lock (_list.SyncRoot) { _list.Add(request); } ThreadPool.QueueUserWorkItem(new WaitCallback(ProcessRequest), request); } ///Cancel all requests in the dispatcher's queue void IAsyncDataDispatcher.CancelAllRequests() { lock (_list.SyncRoot) { for (int i=0; i<_list.Count; ++i) { AsyncDataRequest request = (AsyncDataRequest)_list[i]; request.Cancel(); } _list.Clear(); } } //------------------------------------------------------ // // Private methods // //----------------------------------------------------- // Run a single request. This method gets scheduled on a worker thread // from the process ThreadPool. void ProcessRequest(object o) { AsyncDataRequest request = (AsyncDataRequest)o; // PreSharp uses message numbers that the C# compiler doesn't know about. // Disable the C# complaints, per the PreSharp documentation. #pragma warning disable 1634, 1691 // PreSharp complains about catching NullReference (and other) exceptions. // In this case, these are precisely the ones we want to catch the most, // so that a failure on a worker thread doesn't affect the main thread. #pragma warning disable 56500 // run the request - this may take a while try { request.Complete(request.DoWork()); } // Catch all exceptions. There is no app code on the stack, // so the exception isn't actionable by the app. // Yet we don't want to crash the app. catch (Exception ex) { if (CriticalExceptions.IsCriticalApplicationException(ex)) throw; request.Fail(ex); } catch // non CLS compliant exception { request.Fail(new InvalidOperationException(SR.Get(SRID.NonCLSException, "processing an async data request"))); } #pragma warning restore 56500 #pragma warning restore 1634, 1691 // remove the request from the list lock (_list.SyncRoot) { _list.Remove(request); } } //------------------------------------------------------ // // Private data // //------------------------------------------------------ ArrayList _list = new ArrayList(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Default async scheduler for data operations. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Threading; using System.Windows; using System.Windows.Data; using MS.Internal; namespace MS.Internal.Data { internal class DefaultAsyncDataDispatcher : IAsyncDataDispatcher { //----------------------------------------------------- // // Interface: IAsyncDataDispatcher // //----------------------------------------------------- ///Add a request to the dispatcher's queue void IAsyncDataDispatcher.AddRequest(AsyncDataRequest request) { lock (_list.SyncRoot) { _list.Add(request); } ThreadPool.QueueUserWorkItem(new WaitCallback(ProcessRequest), request); } ///Cancel all requests in the dispatcher's queue void IAsyncDataDispatcher.CancelAllRequests() { lock (_list.SyncRoot) { for (int i=0; i<_list.Count; ++i) { AsyncDataRequest request = (AsyncDataRequest)_list[i]; request.Cancel(); } _list.Clear(); } } //------------------------------------------------------ // // Private methods // //----------------------------------------------------- // Run a single request. This method gets scheduled on a worker thread // from the process ThreadPool. void ProcessRequest(object o) { AsyncDataRequest request = (AsyncDataRequest)o; // PreSharp uses message numbers that the C# compiler doesn't know about. // Disable the C# complaints, per the PreSharp documentation. #pragma warning disable 1634, 1691 // PreSharp complains about catching NullReference (and other) exceptions. // In this case, these are precisely the ones we want to catch the most, // so that a failure on a worker thread doesn't affect the main thread. #pragma warning disable 56500 // run the request - this may take a while try { request.Complete(request.DoWork()); } // Catch all exceptions. There is no app code on the stack, // so the exception isn't actionable by the app. // Yet we don't want to crash the app. catch (Exception ex) { if (CriticalExceptions.IsCriticalApplicationException(ex)) throw; request.Fail(ex); } catch // non CLS compliant exception { request.Fail(new InvalidOperationException(SR.Get(SRID.NonCLSException, "processing an async data request"))); } #pragma warning restore 56500 #pragma warning restore 1634, 1691 // remove the request from the list lock (_list.SyncRoot) { _list.Remove(request); } } //------------------------------------------------------ // // Private data // //------------------------------------------------------ ArrayList _list = new ArrayList(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
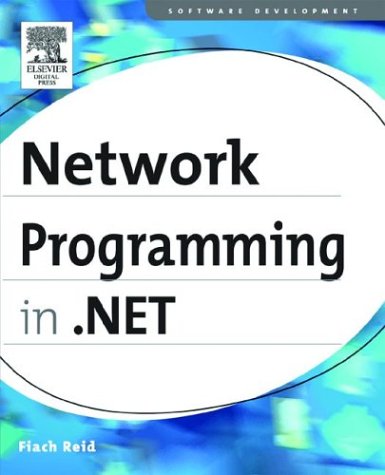
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AttributeCollection.cs
- GridErrorDlg.cs
- PublisherIdentityPermission.cs
- RNGCryptoServiceProvider.cs
- TextStore.cs
- VisualTransition.cs
- Enum.cs
- ConstructorExpr.cs
- EntityDataSourceState.cs
- ListBindableAttribute.cs
- SystemIPInterfaceProperties.cs
- ArgumentOutOfRangeException.cs
- ConfigXmlAttribute.cs
- SecurityRuntime.cs
- GrammarBuilderRuleRef.cs
- indexingfiltermarshaler.cs
- RelatedEnd.cs
- BookmarkEventArgs.cs
- XmlText.cs
- Transform.cs
- AssemblyBuilderData.cs
- WSTrust.cs
- ContentElementCollection.cs
- QueryOutputWriter.cs
- CodeTypeReferenceExpression.cs
- SqlUDTStorage.cs
- SingleKeyFrameCollection.cs
- IDReferencePropertyAttribute.cs
- SchemaImporter.cs
- LinkClickEvent.cs
- ParentQuery.cs
- FontStyleConverter.cs
- SecurityHelper.cs
- TakeQueryOptionExpression.cs
- Int64Storage.cs
- AggregateNode.cs
- ListenerElementsCollection.cs
- Screen.cs
- MessageDispatch.cs
- Site.cs
- SqlExpander.cs
- BooleanFacetDescriptionElement.cs
- BitmapCodecInfoInternal.cs
- EntityCommandDefinition.cs
- CheckBoxAutomationPeer.cs
- WorkflowLayouts.cs
- XmlSchemaObjectCollection.cs
- LayoutInformation.cs
- TTSEngineTypes.cs
- AppDomainCompilerProxy.cs
- CodeDOMProvider.cs
- MulticastOption.cs
- ExpressionBindings.cs
- ContainerParaClient.cs
- WebPartDisplayModeCancelEventArgs.cs
- ValueConversionAttribute.cs
- Matrix3D.cs
- DynamicMethod.cs
- ApplicationActivator.cs
- AutomationEvent.cs
- Or.cs
- TextInfo.cs
- ParserExtension.cs
- RegularExpressionValidator.cs
- HttpModuleCollection.cs
- SecurityPermission.cs
- TemplateApplicationHelper.cs
- ArrayTypeMismatchException.cs
- BindUriHelper.cs
- StrongNameIdentityPermission.cs
- SymbolPair.cs
- GridViewColumn.cs
- CheckBoxPopupAdapter.cs
- JapaneseCalendar.cs
- Image.cs
- HintTextConverter.cs
- ScrollBarAutomationPeer.cs
- TypeRefElement.cs
- EncoderParameter.cs
- MouseButton.cs
- CollectionsUtil.cs
- WindowsContainer.cs
- RangeBase.cs
- LogSwitch.cs
- UIElementParagraph.cs
- DecimalKeyFrameCollection.cs
- Roles.cs
- Stylesheet.cs
- CalculatedColumn.cs
- SqlDependency.cs
- DataSetSchema.cs
- ArglessEventHandlerProxy.cs
- SortExpressionBuilder.cs
- CodeNamespaceImport.cs
- ValidationEventArgs.cs
- TypeDescriptor.cs
- Operator.cs
- ValueTypeIndexerReference.cs
- EnumMember.cs
- DataGridViewCellEventArgs.cs