Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / System.Runtime.DurableInstancing / System / Runtime / AssertHelper.cs / 1305376 / AssertHelper.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- // This class needs to function even if it was built retail. That is, a debug caller calling against a retail // build of this assembly should still have asserts fire. To achieve that, we need to define DEBUG here. // We do not do the registry override in retail because that would require shipping a test hook. We // do not generally ship test hooks today. #if DEBUG #define DEBUG_FOR_REALS #else #define DEBUG #endif namespace System.Runtime { using System; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Runtime.CompilerServices; using System.Runtime.Interop; using System.Security; using System.Runtime.Versioning; static class AssertHelper { [SuppressMessage(FxCop.Category.ReliabilityBasic, FxCop.Rule.InvariantAssertRule, Justification = "Assert implementation")] [ResourceConsumption(ResourceScope.Process)] internal static void FireAssert(string message) { try { #if DEBUG_FOR_REALS InternalFireAssert(ref message); #endif } finally { Debug.Assert(false, message); } } #if DEBUG_FOR_REALS [SuppressMessage(FxCop.Category.Globalization, FxCop.Rule.DoNotPassLiteralsAsLocalizedParameters, Justification = "Debug Only")] [Fx.Tag.SecurityNote(Critical = "Calls into various critical methods", Safe = "Exists only on debug versions")] [SecuritySafeCritical] static void InternalFireAssert(ref string message) { try { string debugMessage = "Assert fired! --> " + message + "\r\n"; if (Debugger.IsAttached) { Debugger.Log(0, Debugger.DefaultCategory, debugMessage); Debugger.Break(); } if (UnsafeNativeMethods.IsDebuggerPresent()) { UnsafeNativeMethods.OutputDebugString(debugMessage); UnsafeNativeMethods.DebugBreak(); } if (Fx.AssertsFailFast) { try { Fx.Exception.TraceFailFast(message); } finally { Environment.FailFast(message); } } } catch (Exception exception) { if (Fx.IsFatal(exception)) { throw; } string newMessage = "Exception during FireAssert!"; try { newMessage = string.Concat(newMessage, " [", exception.GetType().Name, ": ", exception.Message, "] --> ", message); } finally { message = newMessage; } throw; } } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
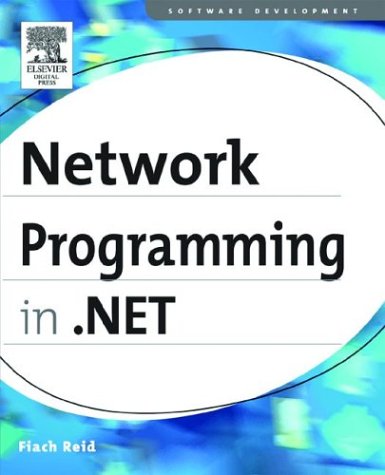
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SpStreamWrapper.cs
- DesignerLabelAdapter.cs
- WebEvents.cs
- ChannelToken.cs
- CompilerResults.cs
- PeerToPeerException.cs
- PageVisual.cs
- Attributes.cs
- XdrBuilder.cs
- MailDefinition.cs
- ModelPerspective.cs
- WebPartCloseVerb.cs
- SystemTcpConnection.cs
- UrlAuthFailedErrorFormatter.cs
- NameValueConfigurationCollection.cs
- PartialToken.cs
- DBSqlParserTable.cs
- DataRelationCollection.cs
- FixedPageAutomationPeer.cs
- BaseHashHelper.cs
- ProcessHostConfigUtils.cs
- NetCodeGroup.cs
- wgx_sdk_version.cs
- Button.cs
- ListViewItem.cs
- ConnectionConsumerAttribute.cs
- EntitySqlQueryBuilder.cs
- dbdatarecord.cs
- LineServices.cs
- input.cs
- HyperLinkStyle.cs
- BitmapEffectGeneralTransform.cs
- SpecialFolderEnumConverter.cs
- CoreSwitches.cs
- XmlSchemaSimpleContent.cs
- OdbcPermission.cs
- XmlSchemaNotation.cs
- WebRequest.cs
- EventListener.cs
- ControlAdapter.cs
- AssemblyResourceLoader.cs
- SHA256Managed.cs
- SafeNativeMethodsOther.cs
- HtmlElementErrorEventArgs.cs
- HostingEnvironmentSection.cs
- RemotingClientProxy.cs
- TextSimpleMarkerProperties.cs
- CaseInsensitiveComparer.cs
- RestHandler.cs
- SelectionBorderGlyph.cs
- Formatter.cs
- SoapTypeAttribute.cs
- Logging.cs
- ConfigWriter.cs
- EntityDataSourceMemberPath.cs
- WindowsTitleBar.cs
- MediaPlayer.cs
- DataObjectFieldAttribute.cs
- ResolveNameEventArgs.cs
- Renderer.cs
- RequestFactory.cs
- TdsParserSafeHandles.cs
- KnownIds.cs
- DateTimeFormatInfo.cs
- ParseHttpDate.cs
- RSAOAEPKeyExchangeFormatter.cs
- ServiceContractGenerationContext.cs
- StringValidatorAttribute.cs
- XPathSelectionIterator.cs
- TableSectionStyle.cs
- HyperLinkField.cs
- HeaderedItemsControl.cs
- CancelEventArgs.cs
- NativeMethods.cs
- templategroup.cs
- MessagePropertyVariants.cs
- SafeNativeMethods.cs
- _LocalDataStoreMgr.cs
- BaseCodeDomTreeGenerator.cs
- UIElementParaClient.cs
- ResourceAttributes.cs
- DesignerLoader.cs
- TextContainerChangedEventArgs.cs
- PackagingUtilities.cs
- ControlPropertyNameConverter.cs
- Compiler.cs
- basecomparevalidator.cs
- ControlSerializer.cs
- CheckBoxBaseAdapter.cs
- InProcStateClientManager.cs
- RuleAttributes.cs
- SecurityPolicySection.cs
- HttpRequestTraceRecord.cs
- CaseInsensitiveHashCodeProvider.cs
- EntityContainerEmitter.cs
- MetadataArtifactLoaderResource.cs
- EventRoute.cs
- ExpressionPrefixAttribute.cs
- SystemColors.cs
- BindingManagerDataErrorEventArgs.cs