Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / MIT / System / Web / UI / MobileControls / Design / TextViewDesigner.cs / 1305376 / TextViewDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design.MobileControls { using System.ComponentModel; using System.Diagnostics; using System.Web.UI.MobileControls; using System.Web.UI.MobileControls.Adapters; using System.Web.UI.Design.MobileControls.Adapters; ////// ////// Provides a designer for the ////// control. /// [ System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode) ] [Obsolete("The System.Web.Mobile.dll assembly has been deprecated and should no longer be used. For information about how to develop ASP.NET mobile applications, see http://go.microsoft.com/fwlink/?LinkId=157231.")] internal class TextViewDesigner : MobileControlDesigner { private System.Web.UI.MobileControls.TextView _textView; /// /// /// /// The control element for design. /// ////// Initializes the designer with the component for design. /// ////// ////// This is called by the designer host to establish the component for /// design. /// ///public override void Initialize(IComponent component) { Debug.Assert(component is System.Web.UI.MobileControls.TextView, "TextViewDesigner.Initialize - Invalid TextView Control"); _textView = (System.Web.UI.MobileControls.TextView) component; base.Initialize(component); } private bool ValidContainment { get { return ( ContainmentStatus == ContainmentStatus.InForm || ContainmentStatus == ContainmentStatus.InPanel || ContainmentStatus == ContainmentStatus.InTemplateFrame); } } /// /// ////// Returns the design-time HTML of the ////// mobile control /// /// ////// The HTML of the control. /// ///protected override String GetDesignTimeNormalHtml() { Debug.Assert (_textView.Text != null); DesignerTextWriter tw; Control[] children = null; String originalText = _textView.Text; bool blankText = (originalText.Trim().Length == 0); bool hasControls = _textView.HasControls(); if (blankText) { if (hasControls) { children = new Control[_textView.Controls.Count]; _textView.Controls.CopyTo(children, 0); } _textView.Text = "[" + _textView.ID + "]"; } try { tw = new DesignerTextWriter(); _textView.Adapter.Render(tw); } finally { if (blankText) { _textView.Text = originalText; if (hasControls) { foreach (Control c in children) { _textView.Controls.Add(c); } } } } return tw.ToString(); } protected override String GetErrorMessage(out bool infoMode) { infoMode = false; if (DesignerAdapterUtil.InMobileUserControl(_textView)) { return null; } if (DesignerAdapterUtil.InUserControl(_textView)) { infoMode = true; return MobileControlDesigner._userControlWarningMessage; } if (!DesignerAdapterUtil.InMobilePage(_textView)) { return MobileControlDesigner._mobilePageErrorMessage; } if (!ValidContainment) { return MobileControlDesigner._formPanelContainmentErrorMessage; } // Containment is valid, return null; return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
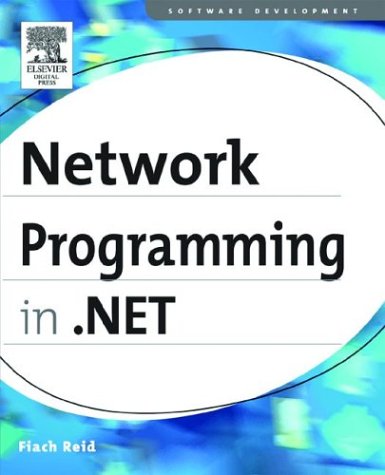
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TabletCollection.cs
- NullableDecimalMinMaxAggregationOperator.cs
- FactoryId.cs
- StrokeNode.cs
- exports.cs
- DataControlPagerLinkButton.cs
- infer.cs
- TearOffProxy.cs
- ZipIOLocalFileDataDescriptor.cs
- EntitySqlException.cs
- Rules.cs
- EntryIndex.cs
- WaitForChangedResult.cs
- MULTI_QI.cs
- Decorator.cs
- SignedInfo.cs
- Model3D.cs
- BindingCompleteEventArgs.cs
- CodeCommentStatementCollection.cs
- Token.cs
- NetTcpSection.cs
- TextEditorThreadLocalStore.cs
- SoapProtocolImporter.cs
- OwnerDrawPropertyBag.cs
- SQLInt16Storage.cs
- TextReader.cs
- RequestCacheManager.cs
- ArrangedElement.cs
- XmlSchemaSimpleTypeUnion.cs
- ErrorRuntimeConfig.cs
- SponsorHelper.cs
- FixUp.cs
- Inflater.cs
- BinaryHeap.cs
- HandlerFactoryWrapper.cs
- ContextMenuStrip.cs
- FrameworkObject.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- ContainerActivationHelper.cs
- MetadataCollection.cs
- FileSystemWatcher.cs
- Color.cs
- SimplePropertyEntry.cs
- CqlGenerator.cs
- Evidence.cs
- PrimitiveType.cs
- ZoneLinkButton.cs
- InfoCardRSAPKCS1SignatureFormatter.cs
- DesignerSerializerAttribute.cs
- AsyncDataRequest.cs
- DockEditor.cs
- SequenceDesigner.cs
- IntSecurity.cs
- DispatcherTimer.cs
- LoginUtil.cs
- XsdBuilder.cs
- _HelperAsyncResults.cs
- sqlstateclientmanager.cs
- ParameterReplacerVisitor.cs
- OracleInternalConnection.cs
- DependencyPropertyAttribute.cs
- dtdvalidator.cs
- ProcessModuleDesigner.cs
- DiscreteKeyFrames.cs
- DoubleCollection.cs
- SQLInt16.cs
- ExceptionWrapper.cs
- DictionaryEntry.cs
- SqlDataSourceQueryConverter.cs
- ConfigXmlSignificantWhitespace.cs
- Pen.cs
- KerberosRequestorSecurityTokenAuthenticator.cs
- AtomServiceDocumentSerializer.cs
- HwndHostAutomationPeer.cs
- ConfigurationManagerInternalFactory.cs
- AnimationStorage.cs
- ConnectionPoint.cs
- FederatedMessageSecurityOverHttp.cs
- DefaultTraceListener.cs
- GeneratedCodeAttribute.cs
- TextBlockAutomationPeer.cs
- IdentityManager.cs
- HelpEvent.cs
- IUnknownConstantAttribute.cs
- EndpointAddress.cs
- RequiredFieldValidator.cs
- ViewGenResults.cs
- CollectionDataContract.cs
- WebPartPersonalization.cs
- FontResourceCache.cs
- JoinCqlBlock.cs
- WsdlInspector.cs
- WebPageTraceListener.cs
- DataKey.cs
- Environment.cs
- AppDomainProtocolHandler.cs
- EUCJPEncoding.cs
- TableLayoutSettingsTypeConverter.cs
- HwndHostAutomationPeer.cs
- XmlSchemas.cs