Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Services / Web / System / Web / Services / WebServiceBindingAttribute.cs / 1305376 / WebServiceBindingAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Services { using System; using System.ComponentModel; using System.Web.Services.Protocols; using System.Runtime.InteropServices; ////// /// [AttributeUsage(AttributeTargets.Class | AttributeTargets.Interface, AllowMultiple=true)] public sealed class WebServiceBindingAttribute : Attribute { string name; string ns; string location; WsiProfiles claims = WsiProfiles.None; bool emitClaims; ///[To be supplied.] ////// /// public WebServiceBindingAttribute() { } ///[To be supplied.] ////// /// public WebServiceBindingAttribute(string name) { this.name = name; } ///[To be supplied.] ////// /// public WebServiceBindingAttribute(string name, string ns) { this.name = name; this.ns = ns; } ///[To be supplied.] ////// /// public WebServiceBindingAttribute(string name, string ns, string location) { this.name = name; this.ns = ns; this.location = location; } ///[To be supplied.] ////// /// public WsiProfiles ConformsTo { get { return claims; } set { claims = value; } } ///[To be supplied.] ////// /// public bool EmitConformanceClaims { get { return emitClaims; } set { emitClaims = value; } } ///[To be supplied.] ////// /// public string Location { get { return location == null ? string.Empty : location; } set { location = value; } } ///[To be supplied.] ////// /// public string Name { get { return name == null ? string.Empty : name; } set { name = value; } } ///[To be supplied.] ////// /// public string Namespace { get { return ns == null ? string.Empty : ns; } set { ns = value; } } } internal class WebServiceBindingReflector { private WebServiceBindingReflector() {} internal static WebServiceBindingAttribute GetAttribute(Type type) { for (; type != null; type = type.BaseType) { object[] attrs = type.GetCustomAttributes(typeof(WebServiceBindingAttribute), false); if (attrs.Length == 0) continue; if (attrs.Length > 1) throw new ArgumentException(Res.GetString(Res.OnlyOneWebServiceBindingAttributeMayBeSpecified1, type.FullName), "type"); return (WebServiceBindingAttribute)attrs[0]; } return null; } internal static WebServiceBindingAttribute GetAttribute(LogicalMethodInfo methodInfo, string binding) { if (methodInfo.Binding != null) { if (binding.Length > 0 && methodInfo.Binding.Name != binding) throw new InvalidOperationException(Res.GetString(Res.WebInvalidBindingName, binding, methodInfo.Binding.Name)); return methodInfo.Binding; } Type type = methodInfo.DeclaringType; object[] attrs = type.GetCustomAttributes(typeof(WebServiceBindingAttribute), false); WebServiceBindingAttribute webAttr = null; foreach (WebServiceBindingAttribute attr in attrs) { if (attr.Name == binding) { if (webAttr != null) throw new ArgumentException(Res.GetString(Res.MultipleBindingsWithSameName2, type.FullName, binding, "methodInfo")); webAttr = attr; } } if (webAttr == null && binding != null && binding.Length > 0) throw new ArgumentException(Res.GetString(Res.TypeIsMissingWebServiceBindingAttributeThat2, type.FullName, binding), "methodInfo"); return webAttr; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
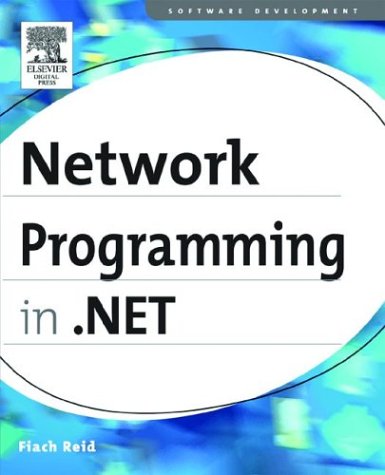
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WindowsFormsHost.cs
- ToolboxComponentsCreatedEventArgs.cs
- UpdatePanel.cs
- CodeObject.cs
- DomainUpDown.cs
- listitem.cs
- SessionPageStateSection.cs
- KeysConverter.cs
- EtwTrace.cs
- Pkcs9Attribute.cs
- Journaling.cs
- MetadataCache.cs
- ResourceDefaultValueAttribute.cs
- OleDbConnectionFactory.cs
- ProcessingInstructionAction.cs
- SiteMapPath.cs
- XmlRawWriter.cs
- VerificationAttribute.cs
- SourceFileBuildProvider.cs
- HGlobalSafeHandle.cs
- SiteMapDataSourceView.cs
- SoapFault.cs
- SqlResolver.cs
- File.cs
- ProtocolsConfigurationEntry.cs
- CheckoutException.cs
- AnnouncementEndpointElement.cs
- UnsafeNativeMethods.cs
- SelectionItemProviderWrapper.cs
- QualificationDataAttribute.cs
- RootBuilder.cs
- RequestCacheEntry.cs
- EdgeProfileValidation.cs
- ColorConverter.cs
- UserMapPath.cs
- SpecialNameAttribute.cs
- ExpressionBindings.cs
- InfoCardRequestException.cs
- XmlSerializationGeneratedCode.cs
- AndCondition.cs
- XmlException.cs
- LoginView.cs
- BindingOperations.cs
- DefaultHttpHandler.cs
- Animatable.cs
- SecurityTokenSerializer.cs
- MissingMemberException.cs
- MatrixCamera.cs
- SizeConverter.cs
- OleDbEnumerator.cs
- DrawingServices.cs
- EmbeddedObject.cs
- Ipv6Element.cs
- DataSpaceManager.cs
- DataGridTextBoxColumn.cs
- Int32CAMarshaler.cs
- ProcessThreadCollection.cs
- DomainUpDown.cs
- _Events.cs
- DbResourceAllocator.cs
- GuidelineCollection.cs
- ProfileProvider.cs
- DeviceContext.cs
- ItemsPanelTemplate.cs
- TimeSpanConverter.cs
- DataTable.cs
- FileDialogCustomPlacesCollection.cs
- VerificationException.cs
- CompositeCollectionView.cs
- ElementHostAutomationPeer.cs
- InkCanvasAutomationPeer.cs
- DataGridViewRowPostPaintEventArgs.cs
- NumericExpr.cs
- CodeAccessSecurityEngine.cs
- BufferedGraphicsManager.cs
- ResourceExpressionBuilder.cs
- WebPartDeleteVerb.cs
- BamlVersionHeader.cs
- ProgressBar.cs
- DesignerCommandAdapter.cs
- ObjectStateEntry.cs
- CodePageEncoding.cs
- ClientFormsIdentity.cs
- CacheDict.cs
- SqlClientWrapperSmiStreamChars.cs
- CodeExporter.cs
- SafeMILHandle.cs
- DetailsView.cs
- DataPagerFieldCollection.cs
- EncoderReplacementFallback.cs
- IISMapPath.cs
- MetabaseServerConfig.cs
- ChannelServices.cs
- path.cs
- AssemblySettingAttributes.cs
- WebPartDescription.cs
- ModelItemCollection.cs
- AddInIpcChannel.cs
- SinglePageViewer.cs
- SecurityContext.cs