Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Extensions / UI / WebControls / Expressions / RangeExpression.cs / 1305376 / RangeExpression.cs
#if ORYX_VNEXT namespace Microsoft.Web.Data.UI.WebControls.Expressions { using System.Web; #else namespace System.Web.UI.WebControls.Expressions { #endif using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Linq; using System.Linq.Expressions; using System.Web.Resources; using System.Web.UI; using System.Web.UI.WebControls; public class RangeExpression : ParameterDataSourceExpression { public string DataField { get { return (string)ViewState["DataField"] ?? String.Empty; } set { ViewState["DataField"] = value; } } public RangeType MinType { get { object o = ViewState["MinType"]; return o != null ? (RangeType)o : RangeType.None; } set { ViewState["MinType"] = value; } } public RangeType MaxType { get { object o = ViewState["MaxType"]; return o != null ? (RangeType)o : RangeType.None; } set { ViewState["MaxType"] = value; } } internal virtual new IOrderedDictionary GetValues() { return Parameters.GetValues(Context, Owner); } public override IQueryable GetQueryable(IQueryable source) { if (source == null) { return null; } if (String.IsNullOrEmpty(DataField)) { throw new InvalidOperationException(AtlasWeb.Expressions_DataFieldRequired); } IOrderedDictionary values = GetValues(); ParameterExpression parameterExpression = Expression.Parameter(source.ElementType, String.Empty); Expression properyExpression = ExpressionHelper.GetValue(ExpressionHelper.CreatePropertyExpression(parameterExpression, DataField)); if (MinType == RangeType.None && MaxType == RangeType.None) { throw new InvalidOperationException(AtlasWeb.RangeExpression_RangeTypeMustBeSpecified); } Expression minExpression = null; Expression maxExpression = null; if (MinType != RangeType.None) { if (values.Count == 0) { throw new InvalidOperationException(AtlasWeb.RangeExpression_MinimumValueRequired); } if (values[0] != null) { minExpression = GetMinRangeExpression(properyExpression, values[0], MinType); } } if (MaxType != RangeType.None) { if (values.Count == 0 || ((minExpression != null) && (values.Count == 1))) { throw new InvalidOperationException(AtlasWeb.RangeExpression_MaximumValueRequired); } object maxValue = minExpression == null ? values[0] : values[1]; if (maxValue != null) { maxExpression = GetMaxRangeExpression(properyExpression, maxValue, MaxType); } } if ((maxExpression == null) && (minExpression == null)) { return null; } Expression rangeExpression = CreateRangeExpressionBody(minExpression, maxExpression); return ExpressionHelper.Where(source, Expression.Lambda(rangeExpression, parameterExpression)); } private static Expression GetMinRangeExpression(Expression propertyExpression, object value, RangeType rangeType) { ConstantExpression constantValue = Expression.Constant(ExpressionHelper.BuildObjectValue(value, propertyExpression.Type)); switch (rangeType) { case RangeType.Exclusive: return Expression.GreaterThan(propertyExpression, constantValue); case RangeType.None: return null; case RangeType.Inclusive: return Expression.GreaterThanOrEqual(propertyExpression, constantValue); default: Debug.Fail("shouldn't get here!"); return null; } } private static Expression GetMaxRangeExpression(Expression propertyExpression, object value, RangeType rangeType) { ConstantExpression constantValue = Expression.Constant(ExpressionHelper.BuildObjectValue(value, propertyExpression.Type)); switch (rangeType) { case RangeType.Exclusive: return Expression.LessThan(propertyExpression, constantValue); case RangeType.None: return null; case RangeType.Inclusive: return Expression.LessThanOrEqual(propertyExpression, constantValue); default: Debug.Fail("shouldn't get here!"); return null; } } private static Expression CreateRangeExpressionBody(Expression minExpression, Expression maxExpression) { if (minExpression == null && maxExpression == null) { return null; } if (minExpression == null) { return maxExpression; } if (maxExpression == null) { return minExpression; } return Expression.AndAlso(minExpression, maxExpression); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
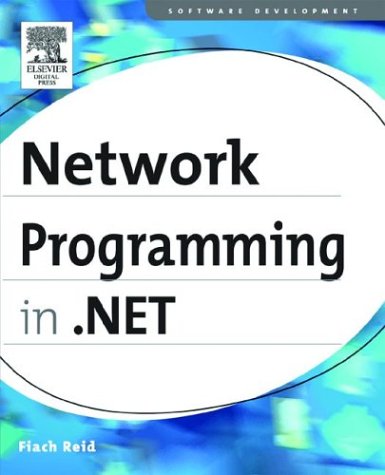
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BlurEffect.cs
- TogglePattern.cs
- PropertyChangedEventArgs.cs
- MetadataWorkspace.cs
- FileLogRecordHeader.cs
- EnumerableCollectionView.cs
- ClientTarget.cs
- PointKeyFrameCollection.cs
- LinqDataSource.cs
- TextBoxLine.cs
- GcHandle.cs
- KeyValueConfigurationElement.cs
- GeometryGroup.cs
- ProcessHostMapPath.cs
- EventBuilder.cs
- BitSet.cs
- FatalException.cs
- AssemblyContextControlItem.cs
- ObservableCollection.cs
- ClientBuildManager.cs
- ValueChangedEventManager.cs
- KeyValueConfigurationCollection.cs
- FileSecurity.cs
- DATA_BLOB.cs
- ResourceKey.cs
- TypeBuilderInstantiation.cs
- DependencyObjectPropertyDescriptor.cs
- DesignerDataParameter.cs
- SoapMessage.cs
- VisualBrush.cs
- MULTI_QI.cs
- CompensationDesigner.cs
- InvalidProgramException.cs
- MulticastDelegate.cs
- DataGridRow.cs
- HttpCapabilitiesSectionHandler.cs
- TemplateBindingExpression.cs
- PerformanceCounterPermissionAttribute.cs
- GetTokenRequest.cs
- BmpBitmapDecoder.cs
- RangeValueProviderWrapper.cs
- TypeNameConverter.cs
- XPathMessageFilterElementComparer.cs
- DataGridViewImageColumn.cs
- LocalValueEnumerator.cs
- CapabilitiesState.cs
- BoundPropertyEntry.cs
- TabControl.cs
- TextControl.cs
- DBConnection.cs
- ServicePointManagerElement.cs
- SqlMethodAttribute.cs
- XmlNodeReader.cs
- IgnoreSection.cs
- XhtmlBasicListAdapter.cs
- PropertySourceInfo.cs
- GridViewEditEventArgs.cs
- PersonalizationEntry.cs
- DataPagerField.cs
- CodeLinePragma.cs
- DispatcherExceptionFilterEventArgs.cs
- GreaterThanOrEqual.cs
- Rule.cs
- PrimitiveDataContract.cs
- wgx_commands.cs
- DataGridViewCellStyleChangedEventArgs.cs
- SignatureToken.cs
- DataReaderContainer.cs
- QueryStringHandler.cs
- ProvidersHelper.cs
- XomlCompilerHelpers.cs
- CollectionConverter.cs
- CorrelationActionMessageFilter.cs
- WorkflowServiceHostFactory.cs
- Types.cs
- ImageListUtils.cs
- ApplicationInterop.cs
- DecimalAverageAggregationOperator.cs
- StreamWriter.cs
- entityreference_tresulttype.cs
- PolyQuadraticBezierSegmentFigureLogic.cs
- MeasureItemEvent.cs
- PtsHost.cs
- CfgParser.cs
- IndexingContentUnit.cs
- DoubleStorage.cs
- BuildProviderAppliesToAttribute.cs
- GroupQuery.cs
- RawStylusInput.cs
- TransactionFlowProperty.cs
- ControlCachePolicy.cs
- XmlAnyAttributeAttribute.cs
- UrlPath.cs
- GregorianCalendarHelper.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- QueryStringHandler.cs
- Win32.cs
- XmlUnspecifiedAttribute.cs
- NopReturnReader.cs
- Rijndael.cs