Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / InterOp / HwndAppCommandInputProvider.cs / 1305600 / HwndAppCommandInputProvider.cs
using System.Windows.Input; using System.Windows.Media; using System.Windows.Threading; using System.Diagnostics; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using MS.Utility; using MS.Internal; using MS.Internal.Interop; using MS.Win32; namespace System.Windows.Interop { internal sealed class HwndAppCommandInputProvider : DispatcherObject, IInputProvider, IDisposable { ////// Accesses and store critical data. This class is also critical (_site and _source) /// [SecurityCritical] internal HwndAppCommandInputProvider( HwndSource source ) { (new UIPermission(PermissionState.Unrestricted)).Assert(); try { _site = new SecurityCriticalDataClass(InputManager.Current.RegisterInputProvider(this)); } finally { UIPermission.RevertAssert(); } _source = new SecurityCriticalDataClass (source); } /// /// Critical:This class accesses critical data, _site. /// TreatAsSafe: This class does not expose the critical data /// [SecurityCritical, SecurityTreatAsSafe] public void Dispose( ) { if (_site != null) { _site.Value.Dispose(); _site = null; } _source = null; } ////// Critical: As this accesses critical data HwndSource /// TreatAsSafe:Information about whether a given input provider services /// a visual is safe to expose. This method does not expose the critical data either. /// [SecurityCritical, SecurityTreatAsSafe] bool IInputProvider.ProvidesInputForRootVisual( Visual v ) { Debug.Assert(null != _source); return _source.Value.RootVisual == v; } void IInputProvider.NotifyDeactivate() {} ////// Critical: As this accesses critical data HwndSource /// [SecurityCritical] internal IntPtr FilterMessage( IntPtr hwnd, WindowMessage msg, IntPtr wParam, IntPtr lParam, ref bool handled ) { // It is possible to be re-entered during disposal. Just return. if(null == _source || null == _source.Value) { return IntPtr.Zero; } if (msg == WindowMessage.WM_APPCOMMAND) { // WM_APPCOMMAND message notifies a window that the user generated an application command event, // for example, by clicking an application command button using the mouse or typing an application command // key on the keyboard. RawAppCommandInputReport report = new RawAppCommandInputReport( _source.Value, InputMode.Foreground, SafeNativeMethods.GetMessageTime(), GetAppCommand(lParam), GetDevice(lParam), InputType.Command); handled = _site.Value.ReportInput(report); } return handled ? new IntPtr(1) : IntPtr.Zero ; } ////// Implementation of the GET_APPCOMMAND_LPARAM macro defined in Winuser.h /// /// ///private static int GetAppCommand( IntPtr lParam ) { return ((short)(NativeMethods.SignedHIWORD(NativeMethods.IntPtrToInt32(lParam)) & ~NativeMethods.FAPPCOMMAND_MASK)); } /// /// Returns the input device that originated this app command. /// InputType.Hid represents an unspecified device that is neither the /// keyboard nor the mouse. /// /// ///private static InputType GetDevice(IntPtr lParam) { InputType inputType = InputType.Hid; // Implementation of the GET_DEVICE_LPARAM macro defined in Winuser.h // Returns either FAPPCOMMAND_KEY (the user pressed a key), FAPPCOMMAND_MOUSE // (the user clicked a mouse button) or FAPPCOMMAND_OEM (unknown device) ushort deviceId = (ushort)(NativeMethods.SignedHIWORD(NativeMethods.IntPtrToInt32(lParam)) & NativeMethods.FAPPCOMMAND_MASK); switch (deviceId) { case NativeMethods.FAPPCOMMAND_MOUSE: inputType = InputType.Mouse; break; case NativeMethods.FAPPCOMMAND_KEY: inputType = InputType.Keyboard; break; case NativeMethods.FAPPCOMMAND_OEM: default: // Unknown device id or FAPPCOMMAND_OEM. // In either cases we set it to the generic human interface device. inputType=InputType.Hid; break; } return inputType; } /// /// This is got under an elevation and is hence critical. This data is not ok to expose. /// private SecurityCriticalDataClass_source; /// /// This is got under an elevation and is hence critical.This data is not ok to expose. /// private SecurityCriticalDataClass_site; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
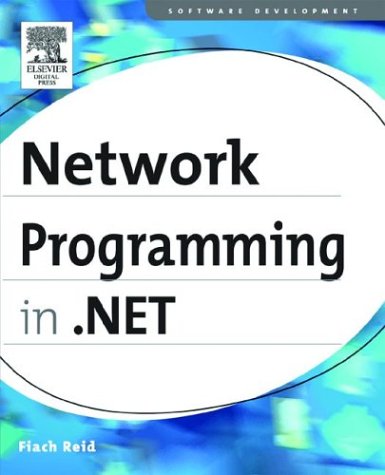
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpApplicationFactory.cs
- RichTextBoxAutomationPeer.cs
- FixedSOMLineCollection.cs
- XsltLoader.cs
- PrimitiveCodeDomSerializer.cs
- ServiceEndpointElementCollection.cs
- EdmType.cs
- ApplicationHost.cs
- Volatile.cs
- DataGridToolTip.cs
- MatrixTransform.cs
- WinEventQueueItem.cs
- CallbackValidator.cs
- PackWebRequestFactory.cs
- SqlTrackingService.cs
- XsdCachingReader.cs
- ExceptionRoutedEventArgs.cs
- WorkflowApplicationUnloadedException.cs
- XmlAutoDetectWriter.cs
- Visual3DCollection.cs
- ArrayEditor.cs
- StorageEndPropertyMapping.cs
- ExceptionDetail.cs
- SequenceNumber.cs
- EntityClassGenerator.cs
- TileBrush.cs
- ConfigurationValues.cs
- PrimitiveXmlSerializers.cs
- Journal.cs
- HtmlElementCollection.cs
- XmlSerializerNamespaces.cs
- DecimalKeyFrameCollection.cs
- FunctionDetailsReader.cs
- ToolStripProgressBar.cs
- ValueQuery.cs
- BindingMAnagerBase.cs
- X509CertificateRecipientServiceCredential.cs
- EntityContainerRelationshipSetEnd.cs
- NavigationPropertyEmitter.cs
- ButtonColumn.cs
- XPathMessageFilterElementCollection.cs
- UnsafeNativeMethods.cs
- OracleCommand.cs
- smtpconnection.cs
- DrawingContextDrawingContextWalker.cs
- OleCmdHelper.cs
- LineSegment.cs
- DesignDataSource.cs
- NotificationContext.cs
- DefaultEventAttribute.cs
- PropertyNames.cs
- LinearKeyFrames.cs
- SmtpTransport.cs
- documentsequencetextpointer.cs
- ClonableStack.cs
- DefaultTraceListener.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- MemberCollection.cs
- PrintControllerWithStatusDialog.cs
- InheritanceUI.cs
- AddInEnvironment.cs
- SortedDictionary.cs
- HtmlInputRadioButton.cs
- WindowsFormsSectionHandler.cs
- SizeChangedEventArgs.cs
- XmlWrappingReader.cs
- DataGridViewTextBoxCell.cs
- SchemaTableOptionalColumn.cs
- ControlIdConverter.cs
- SqlUtils.cs
- CodeMemberMethod.cs
- FigureHelper.cs
- SystemResourceKey.cs
- HttpCapabilitiesBase.cs
- ListViewDeletedEventArgs.cs
- TimeZoneInfo.cs
- ResourceManagerWrapper.cs
- SR.cs
- HashMembershipCondition.cs
- DependencyObject.cs
- ImportCatalogPart.cs
- AnimationException.cs
- TcpChannelHelper.cs
- UTF32Encoding.cs
- WebPartManagerInternals.cs
- DataGridViewRowHeaderCell.cs
- ObjectQuery.cs
- TemplateInstanceAttribute.cs
- LayoutEditorPart.cs
- Win32PrintDialog.cs
- MetadataProperty.cs
- wmiprovider.cs
- ValidatedControlConverter.cs
- EtwTrace.cs
- ExtensionFile.cs
- DataObjectAttribute.cs
- EdmSchemaAttribute.cs
- MetadataPropertyAttribute.cs
- ActiveXMessageFormatter.cs
- UndoUnit.cs