Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Documents / TableRowCollection.cs / 1305600 / TableRowCollection.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: TableRowCollection.cs // // Description: Collection of TableRow objects. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using MS.Internal.Documents; namespace System.Windows.Documents { ////// A TableRowCollection is an ordered collection of TableRows. /// ////// TableRowCollection provides public access for TableRow /// reading and manipulating. /// public sealed class TableRowCollection : IList, IList { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors internal TableRowCollection(TableRowGroup owner) { _rowCollectionInternal = new TableTextElementCollectionInternal (owner); } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods /// /// ////// /// ////// /// ////// /// ////// /// public void CopyTo(Array array, int index) { _rowCollectionInternal.CopyTo(array, index); } /// /// Strongly typed version of ICollection.CopyTo. /// ////// ////// /// ////// /// ////// /// /// /// public void CopyTo(TableRow[] array, int index) { _rowCollectionInternal.CopyTo(array, index); } ////// /// IEnumerator IEnumerable.GetEnumerator() { return _rowCollectionInternal.GetEnumerator(); } ////// /// IEnumerator/// IEnumerable .GetEnumerator() { return ((IEnumerable )_rowCollectionInternal).GetEnumerator(); } /// /// Appends a TableRow to the end of the TableRowCollection. /// /// The TableRow to be added to the end of the TableRowCollection. ///The TableRowCollection index at which the TableRow has been added. ///Adding a null is prohibited. ////// If the ///item value is null. ////// If the new child already has a parent. /// public void Add(TableRow item) { _rowCollectionInternal.Add(item); } ////// Removes all elements from the TableRowCollection. /// ////// Count is set to zero. Capacity remains unchanged. /// To reset the capacity of the TableRowCollection, call TrimToSize /// or set the Capacity property directly. /// public void Clear() { _rowCollectionInternal.Clear(); } ////// Determines whether a TableRow is in the TableRowCollection. /// /// The TableRow to locate in the TableRowCollection. /// The value can be a null reference. ///true if TableRow is found in the TableRowCollection; /// otherwise, false. public bool Contains(TableRow item) { return _rowCollectionInternal.Contains(item); } ////// Returns the zero-based index of the TableRow. If the TableRow is not /// in the TableRowCollection, -1 is returned. /// /// The TableRow to locate in the TableRowCollection. public int IndexOf(TableRow item) { return _rowCollectionInternal.IndexOf(item); } ////// Inserts a TableRow into the TableRowCollection at the specified index. /// /// The zero-based index at which value should be inserted. /// The TableRow to insert. ////// ///index c> is less than zero. /// -or- ///index is greater than Count. ////// If the ///item value is null. ////// If Count already equals Capacity, the capacity of the /// TableRowCollection is increased before the new TableRow is inserted. /// /// If index is equal to Count, TableRow is added to the /// end of TableRowCollection. /// /// The TableRows that follow the insertion point move down to /// accommodate the new TableRow. The indexes of the TableRows that are /// moved are also updated. /// public void Insert(int index, TableRow item) { _rowCollectionInternal.Insert(index, item); } ////// Removes the specified TableRow from the TableRowCollection. /// /// The TableRow to remove from the TableRowCollection. ////// If the ///item value is null. ////// If the specified TableRow is not in this collection. /// ////// The TableRows that follow the removed TableRow move up to occupy /// the vacated spot. The indices of the TableRows that are moved /// also updated. /// public bool Remove(TableRow item) { return _rowCollectionInternal.Remove(item); } ////// Removes the TableRow at the specified index. /// /// The zero-based index of the TableRow to remove. ////// ///index is less than zero /// - or - ///index is equal or greater than count. ////// The TableRows that follow the removed TableRow move up to occupy /// the vacated spot. The indices of the TableRows that are moved /// also updated. /// public void RemoveAt(int index) { _rowCollectionInternal.RemoveAt(index); } ////// Removes a range of TableRows from the TableRowCollection. /// /// The zero-based index of the range /// of TableRows to remove /// The number of TableRows to remove. ////// ///index is less than zero. /// -or- ///count is less than zero. ////// ///index andcount do not denote a valid range of TableRows in the TableRowCollection. ////// The TableRows that follow the removed TableRows move up to occupy /// the vacated spot. The indices of the TableRows that are moved are /// also updated. /// public void RemoveRange(int index, int count) { _rowCollectionInternal.RemoveRange(index, count); } ////// Sets the capacity to the actual number of elements in the TableRowCollection. /// ////// This method can be used to minimize a TableRowCollection's memory overhead /// if no new elements will be added to the collection. /// /// To completely clear all elements in a TableRowCollection, call the Clear method /// before calling TrimToSize. /// public void TrimToSize() { _rowCollectionInternal.TrimToSize(); } #endregion Public Methods //-------------------------------------------------------------------- // // IList Members // //-------------------------------------------------------------------- #region IList Members int IList.Add(object value) { return ((IList)_rowCollectionInternal).Add(value); } void IList.Clear() { this.Clear(); } bool IList.Contains(object value) { return ((IList)_rowCollectionInternal).Contains(value); } int IList.IndexOf(object value) { return ((IList)_rowCollectionInternal).IndexOf(value); } void IList.Insert(int index, object value) { ((IList)_rowCollectionInternal).Insert(index, value); } bool IList.IsFixedSize { get { return ((IList)_rowCollectionInternal).IsFixedSize; } } bool IList.IsReadOnly { get { return ((IList)_rowCollectionInternal).IsReadOnly; } } void IList.Remove(object value) { ((IList)_rowCollectionInternal).Remove(value); } void IList.RemoveAt(int index) { ((IList)_rowCollectionInternal).RemoveAt(index); } object IList.this[int index] { get { return ((IList)_rowCollectionInternal)[index]; } set { ((IList)_rowCollectionInternal)[index] = value; } } #endregion IList Members //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ////// public int Count { get { return _rowCollectionInternal.Count; } } ////// /// public bool IsReadOnly { get { return ((IList)_rowCollectionInternal).IsReadOnly; } } ////// /// public bool IsSynchronized { get { return ((IList)_rowCollectionInternal).IsSynchronized; } } ////// /// Always returns false. /// ////// public object SyncRoot { get { return ((IList)_rowCollectionInternal).SyncRoot; } } ////// /// Gets or sets the number of elements that the TableRowCollection can contain. /// ////// The number of elements that the TableRowCollection can contain. /// ////// Capacity is the number of elements that the TableRowCollection is capable of storing. /// Count is the number of Visuals that are actually in the TableRowCollection. /// /// Capacity is always greater than or equal to Count. If Count exceeds /// Capacity while adding elements, the capacity of the TableRowCollection is increased. /// /// By default the capacity is 8. /// ////// Capacity is set to a value that is less than Count. /// ///public int Capacity { get { return _rowCollectionInternal.Capacity; } set { _rowCollectionInternal.Capacity = value; } } /// /// Indexer for the TableRowCollection. Gets the TableRow stored at the /// zero-based index of the TableRowCollection. /// ///This property provides the ability to access a specific TableRow in the /// TableRowCollection by using the following systax: ///TableRow myTableRow = myTableRowCollection[index] . ////// public TableRow this[int index] { get { return _rowCollectionInternal[index]; } set { _rowCollectionInternal[index] = value; } } #endregion Public Properties #region Internal Methods ///index is less than zero -or-index is equal to or greater than Count. ////// Performs the actual work of adding the item into the array, and notifying it when it is connected /// internal void InternalAdd(TableRow item) { _rowCollectionInternal.InternalAdd(item); } ////// Performs the actual work of notifying item it is leaving the array, and disconnecting it. /// internal void InternalRemove(TableRow item) { _rowCollectionInternal.InternalRemove(item); } #endregion Internal Methods //----------------------------------------------------- // // Private Methods // //----------------------------------------------------- #region Private Methods ////// Ensures that the capacity of this list is at least the given minimum /// value. If the currect capacity of the list is less than min, the /// capacity is increased to min. /// private void EnsureCapacity(int min) { _rowCollectionInternal.EnsureCapacity(min); } ////// Sets the specified TableRow at the specified index; /// Connects the item to the model tree; /// Notifies the TableRow about the event. /// ////// If the new item has already a parent or if the slot at the specified index is not null. /// ////// Note that the function requires that _item[index] == null and /// it also requires that the passed in item is not included into another TableRowCollection. /// private void PrivateConnectChild(int index, TableRow item) { _rowCollectionInternal.PrivateConnectChild(index, item); } ////// Removes specified TableRow from the TableRowCollection. /// /// TableRow to remove. private void PrivateDisconnectChild(TableRow item) { _rowCollectionInternal.PrivateDisconnectChild(item); } // helper method: return true if the item belongs to the collection's owner private bool BelongsToOwner(TableRow item) { return _rowCollectionInternal.BelongsToOwner(item); } // Helper method - Searches the children collection for the index an item currently exists at - // NOTE - ITEM MUST BE IN TEXT TREE WHEN THIS IS CALLED. private int FindInsertionIndex(TableRow item) { return _rowCollectionInternal.FindInsertionIndex(item); } #endregion Private Methods //----------------------------------------------------- // // Private Properties // //------------------------------------------------------ #region Private Properties ////// PrivateCapacity sets/gets the Capacity of the collection. /// private int PrivateCapacity { get { return _rowCollectionInternal.PrivateCapacity; } set { _rowCollectionInternal.PrivateCapacity = value; } } #endregion Private Properties private TableTextElementCollectionInternal_rowCollectionInternal; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
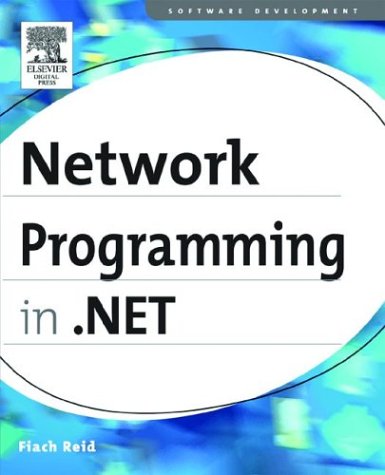
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- QueryExpr.cs
- Vector3D.cs
- PassportIdentity.cs
- ErrorInfoXmlDocument.cs
- CodeCommentStatement.cs
- LazyTextWriterCreator.cs
- SmtpDigestAuthenticationModule.cs
- LOSFormatter.cs
- URIFormatException.cs
- X509ClientCertificateAuthenticationElement.cs
- x509utils.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- QuaternionValueSerializer.cs
- DataBoundLiteralControl.cs
- XmlDocumentFragment.cs
- TagNameToTypeMapper.cs
- ValidationEventArgs.cs
- HtmlTable.cs
- Int16Converter.cs
- TableCellCollection.cs
- Frame.cs
- LabelEditEvent.cs
- ProjectionQueryOptionExpression.cs
- ApplicationInfo.cs
- AllMembershipCondition.cs
- VariableAction.cs
- TimeIntervalCollection.cs
- ControlIdConverter.cs
- CodeComment.cs
- SecurityHeaderLayout.cs
- StreamSecurityUpgradeAcceptorAsyncResult.cs
- PropertyBuilder.cs
- LinkedResource.cs
- ExpandSegment.cs
- ELinqQueryState.cs
- SpinLock.cs
- ToolStripDropDown.cs
- DataListItemEventArgs.cs
- TableParagraph.cs
- Tuple.cs
- InkSerializer.cs
- PropertyChangeTracker.cs
- PseudoWebRequest.cs
- RootBuilder.cs
- GeneralTransform3D.cs
- CheckableControlBaseAdapter.cs
- FlowDocumentPage.cs
- ReflectionHelper.cs
- MessageFault.cs
- DynamicQueryStringParameter.cs
- PenThreadPool.cs
- PointCollection.cs
- TextBox.cs
- GroupQuery.cs
- DataPointer.cs
- ItemsPresenter.cs
- Models.cs
- EventTrigger.cs
- CompositeDataBoundControl.cs
- MergeEnumerator.cs
- AlignmentYValidation.cs
- SafeProcessHandle.cs
- ClientData.cs
- ExpandableObjectConverter.cs
- CustomTokenProvider.cs
- ActivationServices.cs
- CorrelationToken.cs
- AssemblyAssociatedContentFileAttribute.cs
- MulticastDelegate.cs
- XmlSchemaExternal.cs
- WindowVisualStateTracker.cs
- BufferedGraphicsContext.cs
- HtmlInputControl.cs
- OperandQuery.cs
- PersonalizationProviderHelper.cs
- ChannelProtectionRequirements.cs
- BamlBinaryReader.cs
- AppSecurityManager.cs
- DefinitionUpdate.cs
- BufferedWebEventProvider.cs
- CuspData.cs
- FormsAuthenticationCredentials.cs
- DecoderReplacementFallback.cs
- SessionIDManager.cs
- DocumentViewerAutomationPeer.cs
- Int16AnimationUsingKeyFrames.cs
- FreezableOperations.cs
- IdentityNotMappedException.cs
- StreamResourceInfo.cs
- WebControlAdapter.cs
- AstTree.cs
- CmsInterop.cs
- HttpFormatExtensions.cs
- SwitchAttribute.cs
- Automation.cs
- GlyphRunDrawing.cs
- QilLoop.cs
- UnauthorizedAccessException.cs
- RuleInfoComparer.cs
- DiscardableAttribute.cs