Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / MS / Internal / IO / Packaging / contentDescriptor.cs / 1 / contentDescriptor.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Types of keys and data in the element table that is used // by XamlFilter and initialized by the generated function // InitElementDictionary. // // History: // 02/26/2004: [....]: Initial implementation //--------------------------------------------------------------------------- using System; namespace MS.Internal.IO.Packaging { ////// Representation of a fully-qualified XML name for a XAML element. /// internal class ElementTableKey { ////// Constructor. /// internal ElementTableKey(string xmlNamespace, string baseName) { if (xmlNamespace == null) { throw new ArgumentNullException("xmlNamespace"); } if (baseName == null) { throw new ArgumentNullException("baseName"); } _xmlNamespace = xmlNamespace; _baseName = baseName; } ////// Equality test. /// public override bool Equals( object other ) { if (other == null) return false; // Standard behavior. if (other.GetType() != GetType()) return false; // Note that because of the GetType() checking above, the casting must be valid. ElementTableKey otherElement = (ElementTableKey)other; return ( String.CompareOrdinal(BaseName,otherElement.BaseName) == 0 && String.CompareOrdinal(XmlNamespace,otherElement.XmlNamespace) == 0 ); } ////// Hash on all name components. /// public override int GetHashCode() { return XmlNamespace.GetHashCode() ^ BaseName.GetHashCode(); } ////// XML namespace. /// internal string XmlNamespace { get { return _xmlNamespace; } } ////// Local name. /// internal string BaseName { get { return _baseName; } } private string _baseName; private string _xmlNamespace; public static readonly string XamlNamespace = "http://schemas.microsoft.com/winfx/2006/xaml/presentation"; public static readonly string FixedMarkupNamespace = "http://schemas.microsoft.com/xps/2005/06"; } ///Content-location information for an element. internal class ContentDescriptor { ////// The name of the key to the value of _xamlElementContentDescriptorDictionary in the resource file. /// internal const string ResourceKeyName = "Dictionary"; ////// The name of the resource containing the definition of XamlFilter._xamlElementContentDescriptorDictionary. /// internal const string ResourceName = "ElementTable"; ////// Standard constructor. /// internal ContentDescriptor( bool hasIndexableContent, bool isInline, string contentProp, string titleProp) { HasIndexableContent = hasIndexableContent; IsInline = isInline; ContentProp = contentProp; TitleProp = titleProp; } ////// Constructor with default settings for all but HasIndexableContent. /// ////// Currently, this constructor is always passed false, since in this case the other values are "don't care". /// It would make sense to use it with HasIndexableContent=true, however. /// internal ContentDescriptor( bool hasIndexableContent) { HasIndexableContent = hasIndexableContent; IsInline = false; ContentProp = null; TitleProp = null; } ////// Whether indexable at all. /// ////// ContentDescriptor properties are read-write because at table creation time these properties /// are discovered and stored incrementally. /// internal bool HasIndexableContent { get { return _hasIndexableContent; } set { _hasIndexableContent = value; } } ////// Block or inline. /// internal bool IsInline { get { return _isInline; } set { _isInline = value; } } ////// Attribute in which to find content or null. /// internal string ContentProp { get { return _contentProp; } set { _contentProp = value; } } ////// Attribute in which to find a title rather than the real content. /// internal string TitleProp { get { return _titleProp; } set { _titleProp = value; } } private bool _hasIndexableContent; private bool _isInline; private string _contentProp; private string _titleProp; } } // namespace MS.Internal.IO.Packaging // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
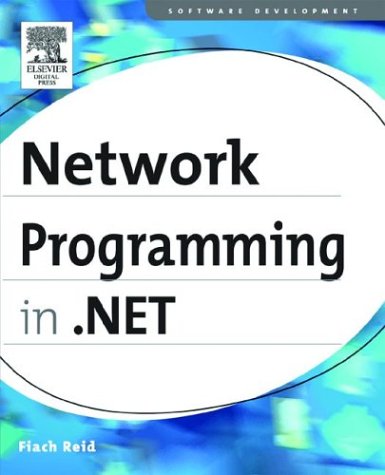
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EntityDataSourceContainerNameConverter.cs
- SmiEventSink_DeferedProcessing.cs
- WorkflowOperationFault.cs
- EndpointConfigContainer.cs
- Keywords.cs
- HybridDictionary.cs
- PanelContainerDesigner.cs
- TextProviderWrapper.cs
- BinaryWriter.cs
- Label.cs
- QilLiteral.cs
- login.cs
- CAGDesigner.cs
- SchemaTableOptionalColumn.cs
- ResourceProviderFactory.cs
- XmlFormatExtensionPointAttribute.cs
- StylusSystemGestureEventArgs.cs
- Utils.cs
- InstanceDescriptor.cs
- CredentialCache.cs
- PieceNameHelper.cs
- SpeechUI.cs
- Identifier.cs
- AppDomainAttributes.cs
- TransformerInfoCollection.cs
- HGlobalSafeHandle.cs
- EmptyEnumerable.cs
- WebPartEditorOkVerb.cs
- ToolStripItemDesigner.cs
- IPipelineRuntime.cs
- DesignerHelpers.cs
- OracleTransaction.cs
- StringDictionary.cs
- RSAPKCS1KeyExchangeFormatter.cs
- PathParser.cs
- ActivityExecutionFilter.cs
- DbProviderFactory.cs
- UTF8Encoding.cs
- OutputCacheSettingsSection.cs
- DecoderNLS.cs
- ObjectSet.cs
- RegexCode.cs
- SymbolPair.cs
- DescendantBaseQuery.cs
- DataGridItem.cs
- EventDrivenDesigner.cs
- ArithmeticException.cs
- WebMessageEncodingElement.cs
- keycontainerpermission.cs
- ISFClipboardData.cs
- LinkArea.cs
- SiteMapDataSource.cs
- CancellationTokenRegistration.cs
- DoubleLinkListEnumerator.cs
- QilStrConcatenator.cs
- GiveFeedbackEventArgs.cs
- ExpressionBindingCollection.cs
- DataGridViewCellEventArgs.cs
- ValidationErrorInfo.cs
- StructuredTypeEmitter.cs
- IndentedWriter.cs
- ConfigurationManagerInternal.cs
- XsltLibrary.cs
- PagePropertiesChangingEventArgs.cs
- DependencyPropertyAttribute.cs
- ProfilePropertyNameValidator.cs
- WorkflowInstance.cs
- ScrollBarRenderer.cs
- XamlSerializationHelper.cs
- EnterpriseServicesHelper.cs
- TCPListener.cs
- PageParser.cs
- DbConnectionInternal.cs
- sqlcontext.cs
- KeyboardNavigation.cs
- BitmapCacheBrush.cs
- Table.cs
- FunctionDetailsReader.cs
- InvalidOleVariantTypeException.cs
- SQLUtility.cs
- ItemMap.cs
- ReferenceAssemblyAttribute.cs
- ReflectEventDescriptor.cs
- TransactionManager.cs
- AnnotationMap.cs
- MarkupExtensionSerializer.cs
- ReadWriteSpinLock.cs
- SkipStoryboardToFill.cs
- StrongBox.cs
- FixedSOMContainer.cs
- Message.cs
- CapabilitiesSection.cs
- ComplexObject.cs
- DrawingServices.cs
- WebPartDisplayModeCancelEventArgs.cs
- DeliveryRequirementsAttribute.cs
- KeyMatchBuilder.cs
- SqlCacheDependencySection.cs
- ContentOperations.cs
- RuntimeWrappedException.cs