Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Xml / System / Xml / Dom / XmlSignificantWhitespace.cs / 1 / XmlSignificantWhitespace.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml { using System; using System.Xml.XPath; using System.Text; using System.Diagnostics; // Represents the text content of an element or attribute. public class XmlSignificantWhitespace : XmlCharacterData { protected internal XmlSignificantWhitespace( string strData, XmlDocument doc ) : base( strData, doc ) { if ( !doc.IsLoading && !base.CheckOnData( strData ) ) throw new ArgumentException(Res.GetString(Res.Xdom_WS_Char)); } // Gets the name of the node. public override String Name { get { return OwnerDocument.strSignificantWhitespaceName; } } // Gets the name of the current node without the namespace prefix. public override String LocalName { get { return OwnerDocument.strSignificantWhitespaceName; } } // Gets the type of the current node. public override XmlNodeType NodeType { get { return XmlNodeType.SignificantWhitespace; } } public override XmlNode ParentNode { get { switch (parentNode.NodeType) { case XmlNodeType.Document: return base.ParentNode; case XmlNodeType.Text: case XmlNodeType.CDATA: case XmlNodeType.Whitespace: case XmlNodeType.SignificantWhitespace: XmlNode parent = parentNode.parentNode; while (parent.IsText) { parent = parent.parentNode; } return parent; default: return parentNode; } } } // Creates a duplicate of this node. public override XmlNode CloneNode(bool deep) { Debug.Assert( OwnerDocument != null ); return OwnerDocument.CreateSignificantWhitespace( Data ); } public override String Value { get { return Data; } set { if ( CheckOnData( value ) ) Data = value; else throw new ArgumentException(Res.GetString(Res.Xdom_WS_Char)); } } // Saves the node to the specified XmlWriter. public override void WriteTo(XmlWriter w) { w.WriteString(Data); } // Saves all the children of the node to the specified XmlWriter. public override void WriteContentTo(XmlWriter w) { // Intentionally do nothing } internal override XPathNodeType XPNodeType { get { XPathNodeType xnt = XPathNodeType.SignificantWhitespace; DecideXPNodeTypeForTextNodes( this, ref xnt ); return xnt; } } internal override bool IsText { get { return true; } } internal override XmlNode PreviousText { get { if (parentNode.IsText) { return parentNode; } return null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
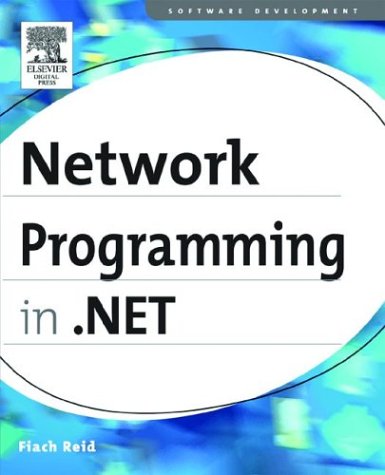
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConnectionPoint.cs
- SHA512Managed.cs
- GroupLabel.cs
- Constants.cs
- FlowchartSizeFeature.cs
- Int32CAMarshaler.cs
- SessionPageStateSection.cs
- PropertyChangeTracker.cs
- OutputCacheModule.cs
- ProtectedConfiguration.cs
- SegmentTree.cs
- XmlSchemaObjectCollection.cs
- SafeNativeMethods.cs
- Point3DKeyFrameCollection.cs
- XmlNodeList.cs
- LocalizationParserHooks.cs
- BamlBinaryReader.cs
- ToolStripPanelCell.cs
- Misc.cs
- MailMessage.cs
- LogStore.cs
- MenuItemCollection.cs
- Substitution.cs
- FaultFormatter.cs
- ReachSerializerAsync.cs
- MultiViewDesigner.cs
- SemanticResultValue.cs
- ImageFormatConverter.cs
- ResourceReferenceKeyNotFoundException.cs
- ScriptManagerProxy.cs
- FieldCollectionEditor.cs
- SqlClientMetaDataCollectionNames.cs
- StreamResourceInfo.cs
- ChangeTracker.cs
- ReadOnlyTernaryTree.cs
- WarningException.cs
- EFAssociationProvider.cs
- EventNotify.cs
- ListViewSortEventArgs.cs
- AspNetHostingPermission.cs
- SystemIPv4InterfaceProperties.cs
- UnsafeNativeMethods.cs
- BindingContext.cs
- IsolatedStorageFile.cs
- GenerateHelper.cs
- CollectionsUtil.cs
- SafeCryptContextHandle.cs
- DiagnosticsConfiguration.cs
- SplashScreen.cs
- DataControlFieldsEditor.cs
- SubMenuStyleCollection.cs
- ModuleBuilderData.cs
- UnsafeNetInfoNativeMethods.cs
- ScrollBarAutomationPeer.cs
- XhtmlBasicPanelAdapter.cs
- mediaeventargs.cs
- PackWebRequestFactory.cs
- OdbcConnectionString.cs
- XPathConvert.cs
- DataGridViewCellStateChangedEventArgs.cs
- Asn1IntegerConverter.cs
- TypeBuilderInstantiation.cs
- PageThemeCodeDomTreeGenerator.cs
- StringValidator.cs
- ObjectDisposedException.cs
- SqlDataSourceCustomCommandPanel.cs
- TextTreeTextBlock.cs
- DefaultTraceListener.cs
- ParseHttpDate.cs
- RenderingEventArgs.cs
- SubstitutionResponseElement.cs
- TextBreakpoint.cs
- Misc.cs
- ToolStripDropDownClosedEventArgs.cs
- SHA256Managed.cs
- SmtpNtlmAuthenticationModule.cs
- MulticastIPAddressInformationCollection.cs
- PenContext.cs
- DataFieldCollectionEditor.cs
- CompiledRegexRunnerFactory.cs
- KeyGesture.cs
- RangeContentEnumerator.cs
- StringAnimationUsingKeyFrames.cs
- TypedColumnHandler.cs
- MarkupWriter.cs
- GlyphManager.cs
- SinglePhaseEnlistment.cs
- Win32Exception.cs
- ArcSegment.cs
- BindToObject.cs
- SoapSchemaMember.cs
- WebPartActionVerb.cs
- ScriptResourceAttribute.cs
- DataRelationPropertyDescriptor.cs
- BaseParaClient.cs
- PriorityBindingExpression.cs
- DateTimeFormatInfo.cs
- Region.cs
- ControlOperationInvoker.cs
- RadioButton.cs