Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Metadata / StoreItemCollection.Loader.cs / 1 / StoreItemCollection.Loader.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Xml; using System.Collections.Generic; using System.Data.EntityModel.SchemaObjectModel; using System.Data.Common; using System.Diagnostics; using System.Data.Common.Utils; using System.Data.Entity; using System.Text; namespace System.Data.Metadata.Edm { public sealed partial class StoreItemCollection { private class Loader { private string _provider = null; private string _providerManifestToken = null; private DbProviderManifest _providerManifest; private DbProviderFactory _providerFactory; IList_errors; IList _schemas; bool _throwOnError; public Loader(IEnumerable xmlReaders, IEnumerable sourceFilePaths, bool throwOnError) { _throwOnError = throwOnError; LoadItems(xmlReaders, sourceFilePaths); } public IList Errors { get { return _errors; } } public IList Schemas { get { return _schemas; } } public DbProviderManifest ProviderManifest { get { return _providerManifest; } } public DbProviderFactory ProviderFactory { get { return _providerFactory; } } public bool HasNonWarningErrors { get { return !MetadataHelper.CheckIfAllErrorsAreWarnings(_errors); } } private void LoadItems(IEnumerable xmlReaders, IEnumerable sourceFilePaths) { Debug.Assert(_errors == null, "we are expecting this to be the location that sets _errors for the first time"); _errors = SchemaManager.ParseAndValidate(xmlReaders, sourceFilePaths, SchemaDataModelOption.ProviderDataModel, OnProviderNotification, OnProviderManifestTokenNotification, OnProviderManifestNeeded, out _schemas ); if (_throwOnError) { ThrowOnNonWarningErrors(); } } internal void ThrowOnNonWarningErrors() { if (!MetadataHelper.CheckIfAllErrorsAreWarnings(_errors)) { //Future Enhancement: if there is an error, we throw exception with error and warnings. //Otherwise the user has no clue to know about warnings. throw EntityUtil.InvalidSchemaEncountered(Helper.CombineErrorMessage(_errors)); } } private void OnProviderNotification(string provider, Action addError) { string expected = _provider; if (_provider == null) { // Even if the Provider is only now being discovered from the first SSDL file, // it must still match the 'implicit' provider that is implied by the DbConnection // or DbProviderFactory that was used to construct this StoreItemCollection. _provider = provider; InitializeProviderManifest(addError); return; } else { // The provider was previously discovered from a preceeding SSDL file; it is an error // if the 'Provider' attributes in all SSDL files are not identical. if (_provider == provider) { return; } } Debug.Assert(expected != null, "Expected provider name not initialized from _provider or _providerFactory?"); addError(Strings.AllArtifactsMustTargetSameProvider_InvariantName(expected, _provider), ErrorCode.InconsistentProvider, EdmSchemaErrorSeverity.Error); } private void InitializeProviderManifest(Action addError) { if (_providerManifest == null && (_providerManifestToken != null && _provider != null)) { DbProviderFactory factory = null; try { factory = DbProviderServices.GetProviderFactory(_provider); } catch (ArgumentException e) { addError(e.Message, ErrorCode.InvalidProvider, EdmSchemaErrorSeverity.Error); return; } try { DbProviderServices services = DbProviderServices.GetProviderServices(factory); _providerManifest = services.GetProviderManifest(_providerManifestToken); _providerFactory = factory; if (_providerManifest is EdmProviderManifest) { if (_throwOnError) { throw EntityUtil.NotSupported(Strings.OnlyStoreConnectionsSupported); } else { addError(Strings.OnlyStoreConnectionsSupported, ErrorCode.InvalidProvider, EdmSchemaErrorSeverity.Error); } return; } } catch (ProviderIncompatibleException e) { if (_throwOnError) { // we want to surface these as ProviderIncompatibleExceptions if we are "allowed" to. throw; } AddProviderIncompatibleError(e, addError); } } } private void OnProviderManifestTokenNotification(string token, Action addError) { if (_providerManifestToken == null) { _providerManifestToken = token; InitializeProviderManifest(addError); return; } if (_providerManifestToken != token) { addError(Strings.AllArtifactsMustTargetSameProvider_ManifestToken(token, _providerManifestToken), ErrorCode.ProviderManifestTokenMismatch, EdmSchemaErrorSeverity.Error); } } private DbProviderManifest OnProviderManifestNeeded(Action addError) { if (_providerManifest == null) { addError(Strings.ProviderManifestTokenNotFound, ErrorCode.ProviderManifestTokenNotFound, EdmSchemaErrorSeverity.Error); } return _providerManifest; } private void AddProviderIncompatibleError(ProviderIncompatibleException provEx, Action addError) { Debug.Assert(provEx != null); Debug.Assert(addError != null); StringBuilder message = new StringBuilder(provEx.Message); if (provEx.InnerException != null && !string.IsNullOrEmpty(provEx.InnerException.Message)) { message.AppendFormat(" {0}", provEx.InnerException.Message); } addError(message.ToString(), ErrorCode.FailedToRetrieveProviderManifest, EdmSchemaErrorSeverity.Error); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Xml; using System.Collections.Generic; using System.Data.EntityModel.SchemaObjectModel; using System.Data.Common; using System.Diagnostics; using System.Data.Common.Utils; using System.Data.Entity; using System.Text; namespace System.Data.Metadata.Edm { public sealed partial class StoreItemCollection { private class Loader { private string _provider = null; private string _providerManifestToken = null; private DbProviderManifest _providerManifest; private DbProviderFactory _providerFactory; IList_errors; IList _schemas; bool _throwOnError; public Loader(IEnumerable xmlReaders, IEnumerable sourceFilePaths, bool throwOnError) { _throwOnError = throwOnError; LoadItems(xmlReaders, sourceFilePaths); } public IList Errors { get { return _errors; } } public IList Schemas { get { return _schemas; } } public DbProviderManifest ProviderManifest { get { return _providerManifest; } } public DbProviderFactory ProviderFactory { get { return _providerFactory; } } public bool HasNonWarningErrors { get { return !MetadataHelper.CheckIfAllErrorsAreWarnings(_errors); } } private void LoadItems(IEnumerable xmlReaders, IEnumerable sourceFilePaths) { Debug.Assert(_errors == null, "we are expecting this to be the location that sets _errors for the first time"); _errors = SchemaManager.ParseAndValidate(xmlReaders, sourceFilePaths, SchemaDataModelOption.ProviderDataModel, OnProviderNotification, OnProviderManifestTokenNotification, OnProviderManifestNeeded, out _schemas ); if (_throwOnError) { ThrowOnNonWarningErrors(); } } internal void ThrowOnNonWarningErrors() { if (!MetadataHelper.CheckIfAllErrorsAreWarnings(_errors)) { //Future Enhancement: if there is an error, we throw exception with error and warnings. //Otherwise the user has no clue to know about warnings. throw EntityUtil.InvalidSchemaEncountered(Helper.CombineErrorMessage(_errors)); } } private void OnProviderNotification(string provider, Action addError) { string expected = _provider; if (_provider == null) { // Even if the Provider is only now being discovered from the first SSDL file, // it must still match the 'implicit' provider that is implied by the DbConnection // or DbProviderFactory that was used to construct this StoreItemCollection. _provider = provider; InitializeProviderManifest(addError); return; } else { // The provider was previously discovered from a preceeding SSDL file; it is an error // if the 'Provider' attributes in all SSDL files are not identical. if (_provider == provider) { return; } } Debug.Assert(expected != null, "Expected provider name not initialized from _provider or _providerFactory?"); addError(Strings.AllArtifactsMustTargetSameProvider_InvariantName(expected, _provider), ErrorCode.InconsistentProvider, EdmSchemaErrorSeverity.Error); } private void InitializeProviderManifest(Action addError) { if (_providerManifest == null && (_providerManifestToken != null && _provider != null)) { DbProviderFactory factory = null; try { factory = DbProviderServices.GetProviderFactory(_provider); } catch (ArgumentException e) { addError(e.Message, ErrorCode.InvalidProvider, EdmSchemaErrorSeverity.Error); return; } try { DbProviderServices services = DbProviderServices.GetProviderServices(factory); _providerManifest = services.GetProviderManifest(_providerManifestToken); _providerFactory = factory; if (_providerManifest is EdmProviderManifest) { if (_throwOnError) { throw EntityUtil.NotSupported(Strings.OnlyStoreConnectionsSupported); } else { addError(Strings.OnlyStoreConnectionsSupported, ErrorCode.InvalidProvider, EdmSchemaErrorSeverity.Error); } return; } } catch (ProviderIncompatibleException e) { if (_throwOnError) { // we want to surface these as ProviderIncompatibleExceptions if we are "allowed" to. throw; } AddProviderIncompatibleError(e, addError); } } } private void OnProviderManifestTokenNotification(string token, Action addError) { if (_providerManifestToken == null) { _providerManifestToken = token; InitializeProviderManifest(addError); return; } if (_providerManifestToken != token) { addError(Strings.AllArtifactsMustTargetSameProvider_ManifestToken(token, _providerManifestToken), ErrorCode.ProviderManifestTokenMismatch, EdmSchemaErrorSeverity.Error); } } private DbProviderManifest OnProviderManifestNeeded(Action addError) { if (_providerManifest == null) { addError(Strings.ProviderManifestTokenNotFound, ErrorCode.ProviderManifestTokenNotFound, EdmSchemaErrorSeverity.Error); } return _providerManifest; } private void AddProviderIncompatibleError(ProviderIncompatibleException provEx, Action addError) { Debug.Assert(provEx != null); Debug.Assert(addError != null); StringBuilder message = new StringBuilder(provEx.Message); if (provEx.InnerException != null && !string.IsNullOrEmpty(provEx.InnerException.Message)) { message.AppendFormat(" {0}", provEx.InnerException.Message); } addError(message.ToString(), ErrorCode.FailedToRetrieveProviderManifest, EdmSchemaErrorSeverity.Error); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
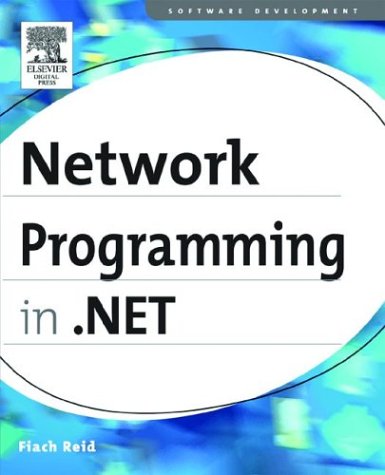
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- xdrvalidator.cs
- ValueTypeFixupInfo.cs
- ResourceDescriptionAttribute.cs
- ProcessThreadCollection.cs
- CoreSwitches.cs
- DynamicObjectAccessor.cs
- ColumnResizeAdorner.cs
- SqlDataSourceStatusEventArgs.cs
- DecimalAnimationUsingKeyFrames.cs
- ColorConverter.cs
- SqlInternalConnectionTds.cs
- ExpressionConverter.cs
- storepermission.cs
- DispatcherObject.cs
- ping.cs
- ChildDocumentBlock.cs
- IssuedTokenServiceCredential.cs
- Publisher.cs
- WebScriptEndpointElement.cs
- OleDbReferenceCollection.cs
- StateFinalizationDesigner.cs
- PerformanceCounterScope.cs
- StreamWithDictionary.cs
- UIElementParaClient.cs
- FixedNode.cs
- TemplateControlBuildProvider.cs
- ListSourceHelper.cs
- OperationInvokerBehavior.cs
- ReadContentAsBinaryHelper.cs
- SimpleHandlerBuildProvider.cs
- DocumentGridContextMenu.cs
- LogicalExpr.cs
- Permission.cs
- DBNull.cs
- SpecularMaterial.cs
- SelectedGridItemChangedEvent.cs
- ContentHostHelper.cs
- XmlTypeAttribute.cs
- DetailsViewInsertedEventArgs.cs
- X509DefaultServiceCertificateElement.cs
- TextElement.cs
- DiscreteKeyFrames.cs
- XmlCharacterData.cs
- ReflectEventDescriptor.cs
- ListControl.cs
- BaseCollection.cs
- DockPatternIdentifiers.cs
- OpenTypeLayoutCache.cs
- BitmapEffectInput.cs
- BitmapEffectState.cs
- CultureTable.cs
- UnsafeNativeMethods.cs
- TaskForm.cs
- CheckoutException.cs
- RadioButtonFlatAdapter.cs
- MultiViewDesigner.cs
- SiteMapSection.cs
- SerializationAttributes.cs
- RadioButtonList.cs
- ExtenderControl.cs
- ReachFixedPageSerializerAsync.cs
- GeneralTransform2DTo3DTo2D.cs
- SqlClientWrapperSmiStreamChars.cs
- BufferBuilder.cs
- DataColumnCollection.cs
- XmlSignatureProperties.cs
- EventMemberCodeDomSerializer.cs
- ScalarOps.cs
- HtmlProps.cs
- WebEvents.cs
- ListMarkerSourceInfo.cs
- DesignerView.Commands.cs
- CodeObjectCreateExpression.cs
- InkCanvasSelectionAdorner.cs
- LookupBindingPropertiesAttribute.cs
- TripleDESCryptoServiceProvider.cs
- DesignerActionItemCollection.cs
- EncoderExceptionFallback.cs
- CompilerInfo.cs
- BuildManagerHost.cs
- OracleMonthSpan.cs
- BaseCollection.cs
- FixedSOMContainer.cs
- DecodeHelper.cs
- ObjectSpanRewriter.cs
- RowToFieldTransformer.cs
- CryptoHelper.cs
- XPathSelfQuery.cs
- AlphabeticalEnumConverter.cs
- URLMembershipCondition.cs
- UnhandledExceptionEventArgs.cs
- TemplateControlParser.cs
- SoapFault.cs
- ThreadAbortException.cs
- TraversalRequest.cs
- UndoManager.cs
- SQLBinaryStorage.cs
- DbParameterHelper.cs
- SerializationObjectManager.cs
- ConfigurationPropertyCollection.cs