Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataWeb / Server / System / Data / Services / DelegateBodyWriter.cs / 1 / DelegateBodyWriter.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a BodyWriter that invokes a callback on writing. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services { #region Namespaces. using System; using System.Data.Services.Providers; using System.Diagnostics; using System.IO; using System.ServiceModel.Channels; using System.Xml; #endregion Namespaces. ///Use this class to handle writing body contents using a callback. internal class DelegateBodyWriter : BodyWriter { #region Fields. ///Service to dispose data source from once the response is written. private readonly IDataService service; ///Callback. private readonly ActionwriterAction; #endregion Fields. /// Initializes a new /// Callback for writing. /// Service to dispose data source from once the response is written. internal DelegateBodyWriter(Actioninstance. writer, IDataService service) : base(false) { Debug.Assert(service != null, "service != null"); Debug.Assert(writer != null, "writer != null"); this.writerAction = writer; this.service = service; } /// Called when the message body is written to an XML file. /// /// Anthat is used to write this /// message body to an XML file. /// protected override void OnWriteBodyContents(XmlDictionaryWriter writer) { Debug.Assert(writer != null, "writer != null"); try { writer.WriteStartElement(XmlConstants.WcfBinaryElementName); using (XmlWriterStream stream = new XmlWriterStream(writer)) { this.writerAction(stream); } writer.WriteEndElement(); } finally { if (this.service != null) { this.service.DisposeDataSource(); HttpContextServiceHost host = this.service.Host as HttpContextServiceHost; if (host != null) { if (host.ErrorFound) { var ctx = System.ServiceModel.OperationContext.Current; if (ctx != null) { ctx.Channel.Abort(); } } } } } } #region Inner types. /// Use this class to write to an internal class XmlWriterStream : Stream { ///. Target writer. private XmlDictionaryWriter innerWriter; ///Initializes a new /// Target writer. internal XmlWriterStream(XmlDictionaryWriter xmlWriter) { Debug.Assert(xmlWriter != null, "xmlWriter != null"); this.innerWriter = xmlWriter; } ///instance. Gets a value indicating whether the current stream supports reading. public override bool CanRead { get { return false; } } ///Gets a value indicating whether the current stream supports seeking. public override bool CanSeek { get { return false; } } ///Gets a value indicating whether the current stream supports writing. public override bool CanWrite { get { return true; } } ///Gets the length in bytes of the stream. public override long Length { get { throw Error.NotSupported(); } } ///Gets or sets the position within the current stream. public override long Position { get { throw Error.NotSupported(); } set { throw Error.NotSupported(); } } ////// Clears all buffers for this stream and causes any buffered /// data to be written to the underlying device. /// public override void Flush() { this.innerWriter.Flush(); } ////// Reads a sequence of bytes from the current stream and /// advances the position within the stream by the number of bytes read. /// /// /// An array of bytes. When this method returns, the buffer contains /// the specified byte array with the values between/// and ( + - 1) replaced /// by the bytes read from the current source. /// /// /// The zero-based byte offset in at which to /// begin storing the data read from the current stream. /// /// /// The maximum number of bytes to be read from the current stream. /// /// The total number of bytes read into the buffer. public override int Read(byte[] buffer, int offset, int count) { throw Error.NotSupported(); } ///Sets the position within the current stream. /// /// A byte offset relative to theparameter. /// /// /// A value of type indicating the reference /// point used to obtain the new position. /// /// The new position within the current stream. public override long Seek(long offset, SeekOrigin origin) { throw Error.NotSupported(); } ///Sets the length of the current stream. /// New value for length. public override void SetLength(long value) { throw Error.NotSupported(); } ////// Writes a sequence of bytes to the current stream and advances /// the current position within this stream by the number of /// bytes written. /// /// /// An array of bytes. This method copies/// bytes from to the current stream. /// /// /// The zero-based byte offset in buffer at which to begin copying /// bytes to the current stream. /// /// /// The number of bytes to be written to the current stream. /// public override void Write(byte[] buffer, int offset, int count) { this.innerWriter.WriteBase64(buffer, offset, count); } } #endregion Inner types. } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a BodyWriter that invokes a callback on writing. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services { #region Namespaces. using System; using System.Data.Services.Providers; using System.Diagnostics; using System.IO; using System.ServiceModel.Channels; using System.Xml; #endregion Namespaces. ///Use this class to handle writing body contents using a callback. internal class DelegateBodyWriter : BodyWriter { #region Fields. ///Service to dispose data source from once the response is written. private readonly IDataService service; ///Callback. private readonly ActionwriterAction; #endregion Fields. /// Initializes a new /// Callback for writing. /// Service to dispose data source from once the response is written. internal DelegateBodyWriter(Actioninstance. writer, IDataService service) : base(false) { Debug.Assert(service != null, "service != null"); Debug.Assert(writer != null, "writer != null"); this.writerAction = writer; this.service = service; } /// Called when the message body is written to an XML file. /// /// Anthat is used to write this /// message body to an XML file. /// protected override void OnWriteBodyContents(XmlDictionaryWriter writer) { Debug.Assert(writer != null, "writer != null"); try { writer.WriteStartElement(XmlConstants.WcfBinaryElementName); using (XmlWriterStream stream = new XmlWriterStream(writer)) { this.writerAction(stream); } writer.WriteEndElement(); } finally { if (this.service != null) { this.service.DisposeDataSource(); HttpContextServiceHost host = this.service.Host as HttpContextServiceHost; if (host != null) { if (host.ErrorFound) { var ctx = System.ServiceModel.OperationContext.Current; if (ctx != null) { ctx.Channel.Abort(); } } } } } } #region Inner types. /// Use this class to write to an internal class XmlWriterStream : Stream { ///. Target writer. private XmlDictionaryWriter innerWriter; ///Initializes a new /// Target writer. internal XmlWriterStream(XmlDictionaryWriter xmlWriter) { Debug.Assert(xmlWriter != null, "xmlWriter != null"); this.innerWriter = xmlWriter; } ///instance. Gets a value indicating whether the current stream supports reading. public override bool CanRead { get { return false; } } ///Gets a value indicating whether the current stream supports seeking. public override bool CanSeek { get { return false; } } ///Gets a value indicating whether the current stream supports writing. public override bool CanWrite { get { return true; } } ///Gets the length in bytes of the stream. public override long Length { get { throw Error.NotSupported(); } } ///Gets or sets the position within the current stream. public override long Position { get { throw Error.NotSupported(); } set { throw Error.NotSupported(); } } ////// Clears all buffers for this stream and causes any buffered /// data to be written to the underlying device. /// public override void Flush() { this.innerWriter.Flush(); } ////// Reads a sequence of bytes from the current stream and /// advances the position within the stream by the number of bytes read. /// /// /// An array of bytes. When this method returns, the buffer contains /// the specified byte array with the values between/// and ( + - 1) replaced /// by the bytes read from the current source. /// /// /// The zero-based byte offset in at which to /// begin storing the data read from the current stream. /// /// /// The maximum number of bytes to be read from the current stream. /// /// The total number of bytes read into the buffer. public override int Read(byte[] buffer, int offset, int count) { throw Error.NotSupported(); } ///Sets the position within the current stream. /// /// A byte offset relative to theparameter. /// /// /// A value of type indicating the reference /// point used to obtain the new position. /// /// The new position within the current stream. public override long Seek(long offset, SeekOrigin origin) { throw Error.NotSupported(); } ///Sets the length of the current stream. /// New value for length. public override void SetLength(long value) { throw Error.NotSupported(); } ////// Writes a sequence of bytes to the current stream and advances /// the current position within this stream by the number of /// bytes written. /// /// /// An array of bytes. This method copies/// bytes from to the current stream. /// /// /// The zero-based byte offset in buffer at which to begin copying /// bytes to the current stream. /// /// /// The number of bytes to be written to the current stream. /// public override void Write(byte[] buffer, int offset, int count) { this.innerWriter.WriteBase64(buffer, offset, count); } } #endregion Inner types. } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
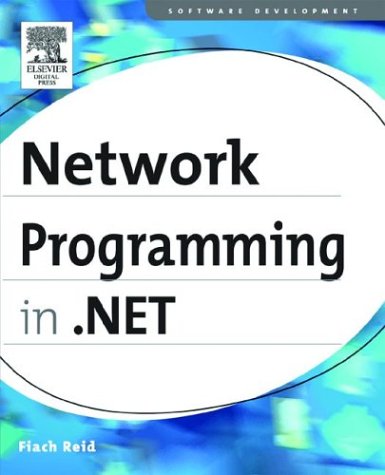
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ErrorLog.cs
- CodeAttachEventStatement.cs
- NamespaceMapping.cs
- SynchronizedKeyedCollection.cs
- TraceProvider.cs
- OracleBinary.cs
- RangeEnumerable.cs
- DocumentSchemaValidator.cs
- ResourceProviderFactory.cs
- TemplatePropertyEntry.cs
- InternalBase.cs
- Propagator.JoinPropagator.cs
- OpenTypeLayout.cs
- SqlRowUpdatingEvent.cs
- EasingFunctionBase.cs
- MessageFormatterConverter.cs
- BinaryMethodMessage.cs
- StreamInfo.cs
- XmlSchemaSequence.cs
- InputScopeConverter.cs
- TableSectionStyle.cs
- DropShadowEffect.cs
- UrlMappingCollection.cs
- XmlNodeChangedEventArgs.cs
- HwndStylusInputProvider.cs
- RuntimeHelpers.cs
- ListViewCancelEventArgs.cs
- DataGridViewCellEventArgs.cs
- TableLayoutSettings.cs
- NetworkInformationPermission.cs
- ListParagraph.cs
- Imaging.cs
- SystemDiagnosticsSection.cs
- ClaimComparer.cs
- ProcessRequestArgs.cs
- NativeMsmqMessage.cs
- SerializationStore.cs
- WebPartConnection.cs
- BitStack.cs
- EventDescriptorCollection.cs
- Convert.cs
- WebControlsSection.cs
- EntityDataSourceSelectingEventArgs.cs
- ConsumerConnectionPointCollection.cs
- SQLRoleProvider.cs
- MemberHolder.cs
- UxThemeWrapper.cs
- StringArrayConverter.cs
- FormsAuthenticationUser.cs
- WebPartEventArgs.cs
- XmlComplianceUtil.cs
- ImpersonateTokenRef.cs
- SimpleWebHandlerParser.cs
- Int16Converter.cs
- LOSFormatter.cs
- ControlBuilder.cs
- DataGridRowsPresenter.cs
- BooleanStorage.cs
- FrameworkPropertyMetadata.cs
- SettingsPropertyIsReadOnlyException.cs
- ContractAdapter.cs
- Scene3D.cs
- RTLAwareMessageBox.cs
- PermissionToken.cs
- ModuleElement.cs
- AdapterSwitches.cs
- FontUnitConverter.cs
- DockingAttribute.cs
- CacheSection.cs
- TreeNodeStyle.cs
- EllipticalNodeOperations.cs
- SafeWaitHandle.cs
- QueryContinueDragEvent.cs
- BrushValueSerializer.cs
- OdbcDataReader.cs
- DeclaredTypeElement.cs
- CodeTryCatchFinallyStatement.cs
- GenericAuthenticationEventArgs.cs
- Site.cs
- WebFormDesignerActionService.cs
- CodeMemberField.cs
- TextSearch.cs
- PropertyChangedEventManager.cs
- HtmlUtf8RawTextWriter.cs
- ProcessingInstructionAction.cs
- TypeToTreeConverter.cs
- WhitespaceRuleLookup.cs
- CollectionConverter.cs
- GPPOINTF.cs
- SeverityFilter.cs
- ConvertersCollection.cs
- MinimizableAttributeTypeConverter.cs
- AttachedAnnotation.cs
- UnsafeNativeMethods.cs
- GetKeyedHashRequest.cs
- RemotingServices.cs
- DateTime.cs
- NameValuePermission.cs
- LambdaValue.cs
- TableItemPatternIdentifiers.cs