Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / TileBrush.cs / 1 / TileBrush.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: TileBrush.cs // // Description: This file contains the implementation of TileBrush. // The TileBrush is an abstract class of Brushes which describes // a way to fill a region by tiling. The contents of the tiles // are described by classes derived from TileBrush. // // History: // 04/29/2003 : adsmith - Created it. // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Diagnostics; using System.Security; using System.Windows; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using MS.Internal; using System.Runtime.InteropServices; namespace System.Windows.Media { ////// TileBrush /// The TileBrush is an abstract class of Brushes which describes /// a way to fill a region by tiling. The contents of the tiles /// are described by classes derived from TileBrush. /// public abstract partial class TileBrush : Brush { #region Constructors ////// Protected constructor for TileBrush. /// Sets all values to their defaults. /// To set property values, use the constructor which accepts paramters /// protected TileBrush() { } #endregion Constructors ////// Obtains the current bounds of the brush's content /// /// Output bounds of content protected abstract void GetContentBounds(out Rect contentBounds); ////// Obtains a matrix that maps the TileBrush's content to the coordinate /// space of the shape it is filling. /// /// /// Fill-bounds of the shape this brush is stroking/filling /// /// /// Output matrix that maps the TileBrush's content to the coordinate /// space of the shape it is filling /// ////// Critical as this code calls UnsafeNativeMethods.MilUtility_GetTileBrushMapping /// and MILUtilities.ConvertFromD3DMATRIX. /// Treat as safe because the first is simply a math utility function with no /// critical data exposure, and the second is passed pointers to type-correct data. /// [SecurityCritical,SecurityTreatAsSafe] internal void GetTileBrushMapping( Rect shapeFillBounds, out Matrix tileBrushMapping ) { Rect contentBounds = Rect.Empty; BrushMappingMode viewboxUnits = ViewboxUnits; bool brushIsEmpty = false; // Initialize out-param tileBrushMapping = Matrix.Identity; // Obtain content bounds for RelativeToBoundingBox ViewboxUnits // // If ViewboxUnits is RelativeToBoundingBox, then the tile-brush // transform is also dependent on the bounds of the content. if (viewboxUnits == BrushMappingMode.RelativeToBoundingBox) { GetContentBounds(out contentBounds); // If contentBounds is Rect.Empty then this brush renders nothing. // Set the empty flag & early-out. if (contentBounds == Rect.Empty) { brushIsEmpty = true; } } // // Pass the properties to MilUtility_GetTileBrushMapping to calculate // the mapping, unless the brush is already determined to be empty // if (!brushIsEmpty) { // // Obtain properties that must be set into local variables // Rect viewport = Viewport; Rect viewbox = Viewbox; Matrix transformValue; Matrix relativeTransformValue; Transform.GetTransformValue( Transform, out transformValue ); Transform.GetTransformValue( RelativeTransform, out relativeTransformValue ); unsafe { D3DMATRIX d3dTransform; D3DMATRIX d3dRelativeTransform; D3DMATRIX d3dContentToShape; int brushIsEmptyBOOL; // Call MilUtility_GetTileBrushMapping, converting Matrix's to // D3DMATRIX's when needed. MILUtilities.ConvertToD3DMATRIX(&transformValue, &d3dTransform); MILUtilities.ConvertToD3DMATRIX(&relativeTransformValue, &d3dRelativeTransform); MS.Win32.PresentationCore.UnsafeNativeMethods.MilCoreApi.MilUtility_GetTileBrushMapping( &d3dTransform, &d3dRelativeTransform, Stretch, AlignmentX, AlignmentY, ViewportUnits, viewboxUnits, &shapeFillBounds, &contentBounds, ref viewport, ref viewbox, out d3dContentToShape, out brushIsEmptyBOOL ); // Convert the brushIsEmpty flag from BOOL to a bool. brushIsEmpty = (brushIsEmptyBOOL != 0); // Set output matrix if the brush isn't empty. Otherwise, the // output of MilUtility_GetTileBrushMapping must be ignored. if (!brushIsEmpty) { Matrix contentToShape; MILUtilities.ConvertFromD3DMATRIX(&d3dContentToShape, &contentToShape); // Set the out-param to the computed tile brush mapping tileBrushMapping = contentToShape; } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: TileBrush.cs // // Description: This file contains the implementation of TileBrush. // The TileBrush is an abstract class of Brushes which describes // a way to fill a region by tiling. The contents of the tiles // are described by classes derived from TileBrush. // // History: // 04/29/2003 : adsmith - Created it. // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Diagnostics; using System.Security; using System.Windows; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using MS.Internal; using System.Runtime.InteropServices; namespace System.Windows.Media { ////// TileBrush /// The TileBrush is an abstract class of Brushes which describes /// a way to fill a region by tiling. The contents of the tiles /// are described by classes derived from TileBrush. /// public abstract partial class TileBrush : Brush { #region Constructors ////// Protected constructor for TileBrush. /// Sets all values to their defaults. /// To set property values, use the constructor which accepts paramters /// protected TileBrush() { } #endregion Constructors ////// Obtains the current bounds of the brush's content /// /// Output bounds of content protected abstract void GetContentBounds(out Rect contentBounds); ////// Obtains a matrix that maps the TileBrush's content to the coordinate /// space of the shape it is filling. /// /// /// Fill-bounds of the shape this brush is stroking/filling /// /// /// Output matrix that maps the TileBrush's content to the coordinate /// space of the shape it is filling /// ////// Critical as this code calls UnsafeNativeMethods.MilUtility_GetTileBrushMapping /// and MILUtilities.ConvertFromD3DMATRIX. /// Treat as safe because the first is simply a math utility function with no /// critical data exposure, and the second is passed pointers to type-correct data. /// [SecurityCritical,SecurityTreatAsSafe] internal void GetTileBrushMapping( Rect shapeFillBounds, out Matrix tileBrushMapping ) { Rect contentBounds = Rect.Empty; BrushMappingMode viewboxUnits = ViewboxUnits; bool brushIsEmpty = false; // Initialize out-param tileBrushMapping = Matrix.Identity; // Obtain content bounds for RelativeToBoundingBox ViewboxUnits // // If ViewboxUnits is RelativeToBoundingBox, then the tile-brush // transform is also dependent on the bounds of the content. if (viewboxUnits == BrushMappingMode.RelativeToBoundingBox) { GetContentBounds(out contentBounds); // If contentBounds is Rect.Empty then this brush renders nothing. // Set the empty flag & early-out. if (contentBounds == Rect.Empty) { brushIsEmpty = true; } } // // Pass the properties to MilUtility_GetTileBrushMapping to calculate // the mapping, unless the brush is already determined to be empty // if (!brushIsEmpty) { // // Obtain properties that must be set into local variables // Rect viewport = Viewport; Rect viewbox = Viewbox; Matrix transformValue; Matrix relativeTransformValue; Transform.GetTransformValue( Transform, out transformValue ); Transform.GetTransformValue( RelativeTransform, out relativeTransformValue ); unsafe { D3DMATRIX d3dTransform; D3DMATRIX d3dRelativeTransform; D3DMATRIX d3dContentToShape; int brushIsEmptyBOOL; // Call MilUtility_GetTileBrushMapping, converting Matrix's to // D3DMATRIX's when needed. MILUtilities.ConvertToD3DMATRIX(&transformValue, &d3dTransform); MILUtilities.ConvertToD3DMATRIX(&relativeTransformValue, &d3dRelativeTransform); MS.Win32.PresentationCore.UnsafeNativeMethods.MilCoreApi.MilUtility_GetTileBrushMapping( &d3dTransform, &d3dRelativeTransform, Stretch, AlignmentX, AlignmentY, ViewportUnits, viewboxUnits, &shapeFillBounds, &contentBounds, ref viewport, ref viewbox, out d3dContentToShape, out brushIsEmptyBOOL ); // Convert the brushIsEmpty flag from BOOL to a bool. brushIsEmpty = (brushIsEmptyBOOL != 0); // Set output matrix if the brush isn't empty. Otherwise, the // output of MilUtility_GetTileBrushMapping must be ignored. if (!brushIsEmpty) { Matrix contentToShape; MILUtilities.ConvertFromD3DMATRIX(&d3dContentToShape, &contentToShape); // Set the out-param to the computed tile brush mapping tileBrushMapping = contentToShape; } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
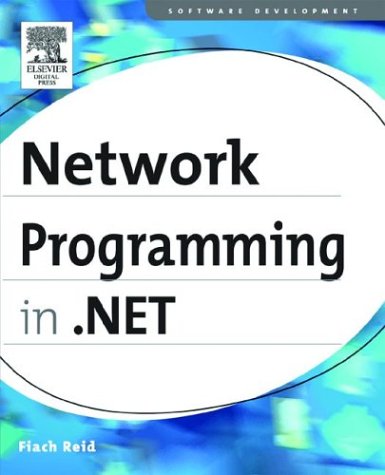
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Underline.cs
- BezierSegment.cs
- XmlSchemaType.cs
- ParallelSeparator.xaml.cs
- SecureStringHasher.cs
- AlternationConverter.cs
- OdbcDataAdapter.cs
- KeyTimeConverter.cs
- InternalRelationshipCollection.cs
- SqlVersion.cs
- SerialReceived.cs
- VisualTarget.cs
- BitmapPalette.cs
- NativeConfigurationLoader.cs
- CodePageUtils.cs
- TextUtf8RawTextWriter.cs
- StopStoryboard.cs
- Int16.cs
- CryptoConfig.cs
- OleDbConnectionInternal.cs
- TableSectionStyle.cs
- Int32AnimationUsingKeyFrames.cs
- DataGridViewCellParsingEventArgs.cs
- JpegBitmapDecoder.cs
- TaskFormBase.cs
- AttributeProviderAttribute.cs
- ACE.cs
- WebEventTraceProvider.cs
- HttpCapabilitiesEvaluator.cs
- ZoneLinkButton.cs
- RequestUriProcessor.cs
- FragmentNavigationEventArgs.cs
- XmlWriterTraceListener.cs
- DataGridRelationshipRow.cs
- ReachPrintTicketSerializerAsync.cs
- PositiveTimeSpanValidator.cs
- Cursor.cs
- TextFindEngine.cs
- DbException.cs
- DBConnectionString.cs
- EntityKey.cs
- EventProvider.cs
- TrackBar.cs
- StyleBamlTreeBuilder.cs
- SqlUtil.cs
- StrokeNodeOperations2.cs
- AnnouncementClient.cs
- TraceHwndHost.cs
- hebrewshape.cs
- ObservableCollectionDefaultValueFactory.cs
- MethodAccessException.cs
- TextOptions.cs
- EndEvent.cs
- WebEvents.cs
- AuthenticatedStream.cs
- DurableInstanceProvider.cs
- XPathParser.cs
- Monitor.cs
- CodeTypeParameter.cs
- Root.cs
- MouseEvent.cs
- HtmlWindowCollection.cs
- BamlVersionHeader.cs
- ReferencedType.cs
- WebPartDisplayModeCancelEventArgs.cs
- SecurityKeyUsage.cs
- SQLChars.cs
- AppDomainShutdownMonitor.cs
- PolyBezierSegmentFigureLogic.cs
- SmiGettersStream.cs
- ButtonDesigner.cs
- UnsafeNativeMethodsMilCoreApi.cs
- TextTreeObjectNode.cs
- XmlSchemaAnnotated.cs
- HtmlHead.cs
- TextSelection.cs
- ControllableStoryboardAction.cs
- WebPartEditorCancelVerb.cs
- MemberRelationshipService.cs
- ActivityInterfaces.cs
- ResourcesBuildProvider.cs
- CachedPathData.cs
- DataGridViewComboBoxEditingControl.cs
- PropertyItemInternal.cs
- QilXmlWriter.cs
- Assembly.cs
- ExecutionContext.cs
- GridEntry.cs
- QilBinary.cs
- AuthenticationException.cs
- RightsManagementPermission.cs
- AttributeCollection.cs
- FilterEventArgs.cs
- VectorAnimationBase.cs
- OleDbErrorCollection.cs
- WebPartManager.cs
- Point.cs
- AutomationPropertyInfo.cs
- BitmapCache.cs
- TabItemWrapperAutomationPeer.cs