Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / WinForms / Managed / System / WinForms / ListViewGroupItemCollection.cs / 1 / ListViewGroupItemCollection.cs
using System.ComponentModel; using System.Diagnostics; using System; using System.Security.Permissions; using System.Drawing; using System.Windows.Forms; using System.ComponentModel.Design; using System.Collections; using Microsoft.Win32; using System.Globalization; namespace System.Windows.Forms { // A collection of items in a ListViewGroup. // internal class ListViewGroupItemCollection : ListView.ListViewItemCollection.IInnerList { private ListViewGroup group; private ArrayList items; public ListViewGroupItemCollection(ListViewGroup group) { this.group = group; } public int Count { get { return Items.Count; } } private ArrayList Items { get { if (items == null) { items = new ArrayList(); } return items; } } public bool OwnerIsVirtualListView { get { if (this.group.ListView != null) { return group.ListView.VirtualMode; } else { return false; } } } public bool OwnerIsDesignMode { get { if (this.group.ListView != null) { ISite s = group.ListView.Site; return(s == null) ? false : s.DesignMode; } else { return false; } } } public ListViewItem this[int index] { get { return (ListViewItem)Items[index]; } set { if (value != Items[index]) { MoveToGroup((ListViewItem)Items[index], null); Items[index] = value; MoveToGroup((ListViewItem)Items[index], this.group); } } } public ListViewItem Add(ListViewItem value) { CheckListViewItem(value); MoveToGroup(value, this.group); Items.Add(value); return value; } public void AddRange(ListViewItem[] items) { for (int i = 0; i < items.Length; i ++) { CheckListViewItem(items[i]); } Items.AddRange(items); for(int i=0; i < items.Length; i++) { MoveToGroup(items[i], this.group); } } ////// throws an ArgumentException if the listViewItem is in another listView already. /// private void CheckListViewItem(ListViewItem item) { if (item.ListView != null && item.ListView != this.group.ListView) { // [....]: maybe we should throw an InvalidOperationException when we add an item from another listView // into this group's collection. // But in a similar situation, ListViewCollection throws an ArgumentException. This is the v1.* behavior throw new ArgumentException(SR.GetString(SR.OnlyOneControl, item.Text), "item"); } } public void Clear() { for(int i=0; i < this.Count; i++) { MoveToGroup(this[i], null); } Items.Clear(); } public bool Contains(ListViewItem item) { return Items.Contains(item); } public void CopyTo(Array dest, int index) { Items.CopyTo(dest, index); } public IEnumerator GetEnumerator() { return Items.GetEnumerator(); } public int IndexOf(ListViewItem item) { return Items.IndexOf(item); } public ListViewItem Insert(int index, ListViewItem item) { CheckListViewItem(item); MoveToGroup(item, this.group); Items.Insert(index, item); return item; } private void MoveToGroup(ListViewItem item, ListViewGroup newGroup) { ListViewGroup oldGroup = item.Group; if (oldGroup != newGroup) { item.group = newGroup; if (oldGroup != null) { oldGroup.Items.Remove(item); } UpdateNativeListViewItem(item); } } public void Remove(ListViewItem item) { Items.Remove(item); if (item.group == this.group) { item.group = null; UpdateNativeListViewItem(item); } } public void RemoveAt(int index) { Remove(this[index]); } private void UpdateNativeListViewItem(ListViewItem item) { if (item.ListView != null && item.ListView.IsHandleCreated && !item.ListView.InsertingItemsNatively) { item.UpdateStateToListView(item.Index); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System.ComponentModel; using System.Diagnostics; using System; using System.Security.Permissions; using System.Drawing; using System.Windows.Forms; using System.ComponentModel.Design; using System.Collections; using Microsoft.Win32; using System.Globalization; namespace System.Windows.Forms { // A collection of items in a ListViewGroup. // internal class ListViewGroupItemCollection : ListView.ListViewItemCollection.IInnerList { private ListViewGroup group; private ArrayList items; public ListViewGroupItemCollection(ListViewGroup group) { this.group = group; } public int Count { get { return Items.Count; } } private ArrayList Items { get { if (items == null) { items = new ArrayList(); } return items; } } public bool OwnerIsVirtualListView { get { if (this.group.ListView != null) { return group.ListView.VirtualMode; } else { return false; } } } public bool OwnerIsDesignMode { get { if (this.group.ListView != null) { ISite s = group.ListView.Site; return(s == null) ? false : s.DesignMode; } else { return false; } } } public ListViewItem this[int index] { get { return (ListViewItem)Items[index]; } set { if (value != Items[index]) { MoveToGroup((ListViewItem)Items[index], null); Items[index] = value; MoveToGroup((ListViewItem)Items[index], this.group); } } } public ListViewItem Add(ListViewItem value) { CheckListViewItem(value); MoveToGroup(value, this.group); Items.Add(value); return value; } public void AddRange(ListViewItem[] items) { for (int i = 0; i < items.Length; i ++) { CheckListViewItem(items[i]); } Items.AddRange(items); for(int i=0; i < items.Length; i++) { MoveToGroup(items[i], this.group); } } ////// throws an ArgumentException if the listViewItem is in another listView already. /// private void CheckListViewItem(ListViewItem item) { if (item.ListView != null && item.ListView != this.group.ListView) { // [....]: maybe we should throw an InvalidOperationException when we add an item from another listView // into this group's collection. // But in a similar situation, ListViewCollection throws an ArgumentException. This is the v1.* behavior throw new ArgumentException(SR.GetString(SR.OnlyOneControl, item.Text), "item"); } } public void Clear() { for(int i=0; i < this.Count; i++) { MoveToGroup(this[i], null); } Items.Clear(); } public bool Contains(ListViewItem item) { return Items.Contains(item); } public void CopyTo(Array dest, int index) { Items.CopyTo(dest, index); } public IEnumerator GetEnumerator() { return Items.GetEnumerator(); } public int IndexOf(ListViewItem item) { return Items.IndexOf(item); } public ListViewItem Insert(int index, ListViewItem item) { CheckListViewItem(item); MoveToGroup(item, this.group); Items.Insert(index, item); return item; } private void MoveToGroup(ListViewItem item, ListViewGroup newGroup) { ListViewGroup oldGroup = item.Group; if (oldGroup != newGroup) { item.group = newGroup; if (oldGroup != null) { oldGroup.Items.Remove(item); } UpdateNativeListViewItem(item); } } public void Remove(ListViewItem item) { Items.Remove(item); if (item.group == this.group) { item.group = null; UpdateNativeListViewItem(item); } } public void RemoveAt(int index) { Remove(this[index]); } private void UpdateNativeListViewItem(ListViewItem item) { if (item.ListView != null && item.ListView.IsHandleCreated && !item.ListView.InsertingItemsNatively) { item.UpdateStateToListView(item.Index); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
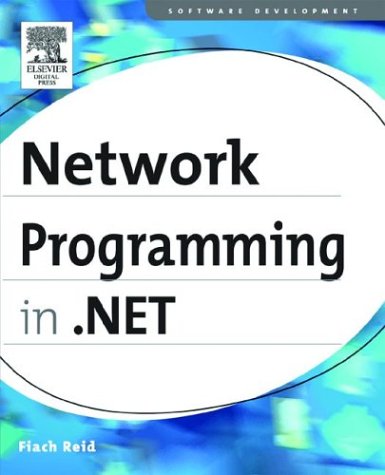
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ObjectListItem.cs
- ObjectTypeMapping.cs
- DefaultPrintController.cs
- LicFileLicenseProvider.cs
- EndpointAddressMessageFilter.cs
- WindowsListBox.cs
- HttpHandlerAction.cs
- OutputCacheSettingsSection.cs
- SchemaContext.cs
- HttpWriter.cs
- StagingAreaInputItem.cs
- RegexWorker.cs
- ListMarkerSourceInfo.cs
- LinqDataSourceDeleteEventArgs.cs
- OneOfScalarConst.cs
- X509Certificate2.cs
- Memoizer.cs
- DrawingCollection.cs
- XmlWellformedWriter.cs
- SortedDictionary.cs
- ListParagraph.cs
- BookmarkTable.cs
- MemoryStream.cs
- SchemaConstraints.cs
- BaseCollection.cs
- ClientTargetSection.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- WindowsFormsDesignerOptionService.cs
- BitmapDownload.cs
- RoleGroup.cs
- TextStore.cs
- CodeComment.cs
- DataGridViewLinkColumn.cs
- CaseStatement.cs
- SEHException.cs
- DataGridDefaultColumnWidthTypeConverter.cs
- Figure.cs
- CharacterBuffer.cs
- Main.cs
- EqualityComparer.cs
- SecurityUtils.cs
- ExitEventArgs.cs
- SystemBrushes.cs
- ZipIOLocalFileHeader.cs
- SafeFileMappingHandle.cs
- MultiPropertyDescriptorGridEntry.cs
- CapabilitiesAssignment.cs
- KeyboardDevice.cs
- CollectionEditorDialog.cs
- SubstitutionDesigner.cs
- SafeViewOfFileHandle.cs
- FixUp.cs
- RandomNumberGenerator.cs
- mda.cs
- InvalidWMPVersionException.cs
- CngKey.cs
- AutoFocusStyle.xaml.cs
- ContentPlaceHolder.cs
- SchemaInfo.cs
- ThreadAttributes.cs
- ObjectResult.cs
- ImmutableClientRuntime.cs
- CollectionEditorDialog.cs
- Span.cs
- FunctionNode.cs
- ToolboxItemFilterAttribute.cs
- Padding.cs
- CompilerGlobalScopeAttribute.cs
- ArrayElementGridEntry.cs
- StreamAsIStream.cs
- AQNBuilder.cs
- ConnectionInterfaceCollection.cs
- EntityCommandCompilationException.cs
- CalloutQueueItem.cs
- ScrollChangedEventArgs.cs
- TemporaryBitmapFile.cs
- XmlMemberMapping.cs
- ErrorsHelper.cs
- DataGridViewRowPostPaintEventArgs.cs
- HttpApplicationFactory.cs
- HashCodeCombiner.cs
- KeyValuePair.cs
- InfoCardClaimCollection.cs
- PrincipalPermission.cs
- VScrollProperties.cs
- AdobeCFFWrapper.cs
- BamlLocalizationDictionary.cs
- WindowsScroll.cs
- WindowsAuthenticationModule.cs
- ToolStripItem.cs
- WindowsListViewGroupSubsetLink.cs
- AnnotationService.cs
- CommonObjectSecurity.cs
- Schema.cs
- BitmapEffectDrawingContextWalker.cs
- AppliesToBehaviorDecisionTable.cs
- DesignerDataConnection.cs
- GeneratedContractType.cs
- MSG.cs
- ManipulationStartingEventArgs.cs