Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / MS / Internal / documents / DocumentApplication.cs / 1 / DocumentApplication.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // DocumentApplication is an internal application that is used to show // Xps Documents. It inherits from Application and hosts DocumentViewer. // // History: // 12/07/04 - twillie created // //--------------------------------------------------------------------------- // Used to support the warnings disabled below #pragma warning disable 1634, 1691 using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.IO; // Stream using System.Security; using System.Security.Permissions; using System.Text; // StringBuilder using System.Windows; using System.Windows.Controls; // DocumentViewer using System.Windows.Documents; using System.Windows.Interop; using System.Windows.Media; // NumberSubstitution (localization) using System.Windows.Navigation; using System.Windows.Markup; using System.Windows.Threading; using MS.Internal; using MS.Internal.AppModel; using MS.Internal.Documents.Application; using MS.Internal.PresentationFramework; using MS.Internal.Utility; using MS.Win32; namespace MS.Internal.Documents { ////// /// /// internal sealed class DocumentApplication : System.Windows.Application { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Critical 1) Audits that we will not construct in a FullTrust environment. /// 2) Sets HostedController.Navigate which expects only /// DocumentApplication.Navigate /// TreatAsSafe 1) This check is only critical because we need to ensure it will /// be tracked, and cannot be removed without review. /// 2) HostedController.Navigate is correctly being assigned with /// the expected value of BrowserInteropHelper.Navigate /// [SecurityCritical, SecurityTreatAsSafe] public DocumentApplication() : base() { // If true throw, since we shouldn't be in FullTrust. if (SecurityHelper.CallerHasPermissionWithAppDomainOptimization(new SecurityPermission(SecurityPermissionFlag.UnmanagedCode))) { throw new ApplicationException(SR.Get(SRID.DocumentApplicationNotInFullTrust)); } // Add style to application resources. SetupNavigationWindowStyle(); // this is done because the functionality to navigate is internal to Framework // and PresentationUI is not allowed direct access to the internals of Framework // here we are specifically allowing the NavigationHelper to navigate the root browser // on the frameworks behalf MS.Internal.Documents.Application.NavigationHelper.Navigate = Navigate; // Get a reference to the DigSig Provider. // (this is a our means to interact with reach) _docSigManager = DocumentSignatureManager.Current; // Get a reference to the RM Provider. // (this is a our means to interact with reach) _docRMManager = DocumentRightsManagementManager.Current; // Setup mime handler for type checking. SetupMimeHandlers(); } #endregion Constructors //------------------------------------------------------ // // Protected Methods // //----------------------------------------------------- #region Protected Methods ////// Critical: VerifySignatures is defined in a non-APTCA assembly. /// TreatAsSafe: call is safe, does not have security implications. /// [SecurityCritical, SecurityTreatAsSafe] protected override void OnLoadCompleted(NavigationEventArgs e) { base.OnLoadCompleted(e); // On the first load of the UI initialize the DocumentViewer if (_docViewer == null) { InitUI(); // DocumentSignature setup _docSigManager.VerifySignatures(); InitializeDocumentApplicationDocumentViewer(); // Run an initial evaluate to set the default infobar text. _docSigManager.Evaluate(); _docRMManager.Evaluate(); } } ////// Critical: GetCurrentState is defined in a non-APTCA assembly. /// TreatAsSafe: method is not intrinsically unsafe; it's safe to call it. Also, /// returned value is not disclosed. /// [SecurityCritical, SecurityTreatAsSafe] protected override void OnNavigating(NavigatingCancelEventArgs e) { base.OnNavigating(e); // Save the current view state if (_docViewer != null) { System.Diagnostics.Debug.Assert(e.ContentStateToSave == null); // There shouldn't be anything to overwrite. e.ContentStateToSave = new DocumentApplicationJournalEntry(_docViewer.GetCurrentState()); } } #endregion Protected Methods //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods ////// Critical: /// 1) Gets Uri which has path info we do not want to leak /// [SecurityCritical] private void Navigate(SecurityCriticalDatauri) { // ensuring that navigation happens on browser thread Dispatcher.BeginInvoke( DispatcherPriority.Send, new DispatcherOperationCallback(NavigateCallback), uri); } /// /// Critical: /// 1) Gets Uri which has path info we do not want to leak /// [SecurityCritical] private object NavigateCallback(object data) { SecurityCriticalDatauri = (SecurityCriticalData )data; (SecurityHelper.CreateUserInitiatedNavigationPermission()).Assert(); //BlessedAssert try { // Use the DemandWhenUnsafe navigation call to make it clear that // we could be navigating to an external Uri or another document. AppSecurityManager.SafeLaunchBrowserDemandWhenUnsafe( null, uri.Value, true); } finally { CodeAccessPermission.RevertAssert(); } return null; } /// /// Constructs the appropriate NavigationWindow styles, and assign them to the resources. /// private void SetupNavigationWindowStyle() { // Add the UI styles to the application resources. // For performance we build the basic NavWindow style here and insert it into // the resources (avoiding a FindResource call). // Setup the ContentPresenter that will hold the DocumentViewer FrameworkElementFactory docViewer = new FrameworkElementFactory(typeof(DocumentApplicationDocumentViewer), "PUIDocumentApplicationDocumentViewer"); DynamicResourceExtension styleKey = new DynamicResourceExtension(new ComponentResourceKey( typeof(PresentationUIStyleResources), "PUIDocumentApplicationDocumentViewerStyleKey")); docViewer.SetValue(FrameworkElement.StyleProperty, styleKey); docViewer.SetValue(FrameworkElement.OverridesDefaultStyleProperty, true); docViewer.SetValue(FrameworkElement.NameProperty, "PUIDocumentApplicationDocumentViewer"); docViewer.SetValue(NumberSubstitution.CultureSourceProperty, NumberCultureSource.User); docViewer.SetValue(DocumentViewer.DocumentProperty, new TemplateBindingExtension(Window.ContentProperty)); // Setup the ControlTemplate ControlTemplate controlTemplate = new ControlTemplate(typeof(NavigationWindow)); controlTemplate.VisualTree = docViewer; controlTemplate.Seal(); // Construct the NavigationWindow style and assign the control template. Style style = new Style(); style.Setters.Add(new Setter(Control.TemplateProperty, controlTemplate)); style.Seal(); // Assign the style to both of the Navigation Style Keys. Resources.Add(SystemParameters.NavigationChromeStyleKey, style); Resources.Add(SystemParameters.NavigationChromeDownLevelStyleKey, style); } ////// Registers the mime handlers for the appropriate mime types. /// private void SetupMimeHandlers() { // Setup the fixed document mime handler. StreamToObjectFactoryDelegate docFactory = new StreamToObjectFactoryDelegate(S0ConverterDoc); MimeObjectFactory.Register(MimeTypeMapper.FixedDocumentSequenceMime, docFactory); // Setup the resource dictionary mime handler. StreamToObjectFactoryDelegate resDictFactory = new StreamToObjectFactoryDelegate(S0ConverterResDict); MimeObjectFactory.Register(MimeTypeMapper.ResourceDictionaryMime, resDictFactory); // Setup the mime handler for invalid mime types StreamToObjectFactoryDelegate notSupportedFactory = new StreamToObjectFactoryDelegate(NotSupportedConverter); // dangerous types MimeObjectFactory.Register(MimeTypeMapper.BamlMime, notSupportedFactory); MimeObjectFactory.Register(MimeTypeMapper.XamlMime, notSupportedFactory); // out of spec types MimeObjectFactory.Register(MimeTypeMapper.FixedDocumentMime, notSupportedFactory); MimeObjectFactory.Register(MimeTypeMapper.FixedPageMime, notSupportedFactory); } ////// /// ////// Critical - 1) This code uses the rootbrowserwindow which is critical. /// 2) This method calls InitializeUI and passes in a reference to the /// DocumentRightsManagementManager. /// TreatAsSafe - 1) Since the window reference is only used to find the DocumentViewer. /// 2) Since the DocumentRightsManagementManager is constructed locally within /// this class. /// [SecurityCritical, SecurityTreatAsSafe] private void InitializeDocumentApplicationDocumentViewer() { // Set references to UI objects. NavigationWindow appWin = GetAppWindow(); // Ensure NavigationWindow is valid if (appWin == null) { throw new NotSupportedException(SR.Get(SRID.DocumentApplicationCannotInitializeUI)); } // This will guarantee that the required styles have been loaded, and that all // required controls will be available appWin.ApplyTemplate(); // Find the DocumentViewer in the current style. This will be used by // DocumentApplicationUI to find all UI controls. The appWin would work // here, however, for security the reference to the appWin (RootBrowserWindow) // is released at the end of this method. _docViewer = appWin.Template.FindName("PUIDocumentApplicationDocumentViewer", appWin) as DocumentApplicationDocumentViewer; if (_docViewer == null) { throw new NotSupportedException(SR.Get(SRID.DocumentApplicationCannotInitializeUI)); } // Setup DocumentApplicationDocumentViewer's IDocumentScrollInfo for viewing modes. IDocumentScrollInfo idsi = _docViewer.DocumentScrollInfo; idsi.LockViewModes = true; // Initialize and verify the UI try { _docViewer.InitializeUI(_docSigManager, _docRMManager); } // Allow empty catch statements. #pragma warning disable 56500 catch (Exception exceptionArgs) { // Throw assert with the exception details Invariant.Assert(false, exceptionArgs.Message, exceptionArgs.ToString()); } // Disallow empty catch statements. #pragma warning restore 56500 // Setup a handler to add custom journal entries _docViewer.AddJournalEntry += OnAddJournalEntry; } #endregion Private Methods #region Internal Methods ////// This is called when the Text dependency property changes in the Window. /// ////// Critical - calls SetStatusText which is SUC'ed. /// TreatAsSafe - setting the status bar text of the browser considered safe. /// IE/MSRC does not consider hostile setting of text ( even for hyperlink spoofing) /// to be an exploit. /// [SecurityCritical, SecurityTreatAsSafe] internal void InitUI() { // Set Statusbar BrowserCallbackServices.SetStatusText(SR.Get(SRID.DocumentApplicationStatusLoaded)); } ////// This will convert the incomming stream to a FixedDocument or FixedDocumentSequence. /// It uses the XpsS0SchemaValiator if the stream is not valid it will throw. /// NOTE: Method is not strongly types to match StreamToObjectFactoryDelegate /// /// Stream to process /// baseUri to resolve to /// parameter indicating that navigation can use the top level browser if we fail to produce an object /// provide secure, isolated content if possible /// Should we allow async loading of this content /// Was this navigation the result of a journal operation /// ///A FixedDocument or FixedDocumentSequence. private object S0ConverterDoc(Stream stream, Uri baseUri, bool canUseTopLevelBrowser, bool sandboxExternalContent, bool allowAsync, bool isJournalNavigation, out XamlReader asyncObjectConverter) { ParserContext pc = new ParserContext(); pc.BaseUri = baseUri; pc.SkipJournaledProperties = false; // assuming that the S0 schema will never have any state that needs to be journaled pc.XamlTypeMapper = XmlParserDefaults.DefaultMapper; XpsValidatingLoader.AssertDocumentMode(); XpsValidatingLoader loader = new XpsValidatingLoader(); object document = loader.Load(stream, null, pc, MimeTypeMapper.FixedDocumentSequenceMime); asyncObjectConverter = null; if (document is FixedDocumentSequence) { // Since a FixedDocumentSequence was found allow FixedDocuments to be loaded. _isInitialDocSeqLoaded = true; } // Check if FixedDocument is allowed, if so attempt to load it // This case will filter the invalid document load attempts. else if (!_isInitialDocSeqLoaded || !(document is FixedDocument)) { // Did not throw directly as this will // a. increase block coverage ;) // b. consolidate behavior to one place NotSupportedConverter(null, null, false, false, allowAsync, false, out asyncObjectConverter); } return document; } ////// This will convert the incomming stream to a ResourceDictionary. /// It uses the XpsS0SchemaValiator if the stream is not valid it will throw. /// NOTE: Method is not strongly types to match StreamToObjectFactoryDelegate /// /// Stream to process /// baseUri to resolve to /// parameter indicating that navigation can use the top level browser if we fail to produce an object /// provide secure, isolated content if possible /// Should we allow async loading of this content /// Was this navigation the result of a journal operation /// ///A ResourceDictionary. private object S0ConverterResDict(Stream stream, Uri baseUri, bool canUseTopLevelBrowser, bool sandboxExternalContent, bool allowAsync, bool isJournalNavigation, out XamlReader asyncObjectConverter) { ParserContext pc = new ParserContext(); pc.BaseUri = baseUri; pc.SkipJournaledProperties = false; // assuming that the S0 schema will never have any state that needs to be journaled pc.XamlTypeMapper = XmlParserDefaults.DefaultMapper; XpsValidatingLoader loader = new XpsValidatingLoader(); ResourceDictionary resDict = loader.Load(stream, baseUri, pc, MimeTypeMapper.ResourceDictionaryMime) as ResourceDictionary; asyncObjectConverter = null; if (resDict == null) { // Did not throw directly as this will // a. increase block coverage ;) // b. consolidate behavior to one place NotSupportedConverter(null, null, false, false, allowAsync, false, out asyncObjectConverter); } return resDict; } ////// This will throw an exception, since the document type is not supported. /// /// Stream to process /// baseUri to resolve to /// parameter indicating that navigation can use the top level browser if we fail to produce an object /// provide secure, isolated content if possible /// Should we allow async loading of this content /// Was this navigation the result of a journal operation /// ///private object NotSupportedConverter(Stream stream, Uri baseUri, bool canUseTopLevelBrowser, bool sandboxExternalContent, bool allowAsync, bool isJournalNavigation, out XamlReader asyncObjectConverter) { asyncObjectConverter = null; // Since the document type contained in the stream is not supported, this will throw. throw new NotSupportedException(SR.Get(SRID.DocumentApplicationUnknownFileFormat)); } /// /// Creates a new journal entry on the NavWindow's backstack that stores the current /// UI state. /// /// /// ////// Critical - This code uses the rootbrowserwindow which is critical /// TreatAsSafe - Since the window reference is only used to add a journal entry. /// [SecurityCritical, SecurityTreatAsSafe] private void OnAddJournalEntry(object sender, DocumentApplicationJournalEntryEventArgs e) { NavigationWindow appWin = GetAppWindow(); if ((appWin != null) && (e != null)) { // Create a new DocumentApplicationJournalEntry and add to back stack appWin.AddBackEntry(new DocumentApplicationJournalEntry(e.State)); } } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private DocumentApplicationDocumentViewer _docViewer; private DocumentSignatureManager _docSigManager; private DocumentRightsManagementManager _docRMManager; private bool _isInitialDocSeqLoaded; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // DocumentApplication is an internal application that is used to show // Xps Documents. It inherits from Application and hosts DocumentViewer. // // History: // 12/07/04 - twillie created // //--------------------------------------------------------------------------- // Used to support the warnings disabled below #pragma warning disable 1634, 1691 using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.IO; // Stream using System.Security; using System.Security.Permissions; using System.Text; // StringBuilder using System.Windows; using System.Windows.Controls; // DocumentViewer using System.Windows.Documents; using System.Windows.Interop; using System.Windows.Media; // NumberSubstitution (localization) using System.Windows.Navigation; using System.Windows.Markup; using System.Windows.Threading; using MS.Internal; using MS.Internal.AppModel; using MS.Internal.Documents.Application; using MS.Internal.PresentationFramework; using MS.Internal.Utility; using MS.Win32; namespace MS.Internal.Documents { ////// /// /// internal sealed class DocumentApplication : System.Windows.Application { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Critical 1) Audits that we will not construct in a FullTrust environment. /// 2) Sets HostedController.Navigate which expects only /// DocumentApplication.Navigate /// TreatAsSafe 1) This check is only critical because we need to ensure it will /// be tracked, and cannot be removed without review. /// 2) HostedController.Navigate is correctly being assigned with /// the expected value of BrowserInteropHelper.Navigate /// [SecurityCritical, SecurityTreatAsSafe] public DocumentApplication() : base() { // If true throw, since we shouldn't be in FullTrust. if (SecurityHelper.CallerHasPermissionWithAppDomainOptimization(new SecurityPermission(SecurityPermissionFlag.UnmanagedCode))) { throw new ApplicationException(SR.Get(SRID.DocumentApplicationNotInFullTrust)); } // Add style to application resources. SetupNavigationWindowStyle(); // this is done because the functionality to navigate is internal to Framework // and PresentationUI is not allowed direct access to the internals of Framework // here we are specifically allowing the NavigationHelper to navigate the root browser // on the frameworks behalf MS.Internal.Documents.Application.NavigationHelper.Navigate = Navigate; // Get a reference to the DigSig Provider. // (this is a our means to interact with reach) _docSigManager = DocumentSignatureManager.Current; // Get a reference to the RM Provider. // (this is a our means to interact with reach) _docRMManager = DocumentRightsManagementManager.Current; // Setup mime handler for type checking. SetupMimeHandlers(); } #endregion Constructors //------------------------------------------------------ // // Protected Methods // //----------------------------------------------------- #region Protected Methods ////// Critical: VerifySignatures is defined in a non-APTCA assembly. /// TreatAsSafe: call is safe, does not have security implications. /// [SecurityCritical, SecurityTreatAsSafe] protected override void OnLoadCompleted(NavigationEventArgs e) { base.OnLoadCompleted(e); // On the first load of the UI initialize the DocumentViewer if (_docViewer == null) { InitUI(); // DocumentSignature setup _docSigManager.VerifySignatures(); InitializeDocumentApplicationDocumentViewer(); // Run an initial evaluate to set the default infobar text. _docSigManager.Evaluate(); _docRMManager.Evaluate(); } } ////// Critical: GetCurrentState is defined in a non-APTCA assembly. /// TreatAsSafe: method is not intrinsically unsafe; it's safe to call it. Also, /// returned value is not disclosed. /// [SecurityCritical, SecurityTreatAsSafe] protected override void OnNavigating(NavigatingCancelEventArgs e) { base.OnNavigating(e); // Save the current view state if (_docViewer != null) { System.Diagnostics.Debug.Assert(e.ContentStateToSave == null); // There shouldn't be anything to overwrite. e.ContentStateToSave = new DocumentApplicationJournalEntry(_docViewer.GetCurrentState()); } } #endregion Protected Methods //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods ////// Critical: /// 1) Gets Uri which has path info we do not want to leak /// [SecurityCritical] private void Navigate(SecurityCriticalDatauri) { // ensuring that navigation happens on browser thread Dispatcher.BeginInvoke( DispatcherPriority.Send, new DispatcherOperationCallback(NavigateCallback), uri); } /// /// Critical: /// 1) Gets Uri which has path info we do not want to leak /// [SecurityCritical] private object NavigateCallback(object data) { SecurityCriticalDatauri = (SecurityCriticalData )data; (SecurityHelper.CreateUserInitiatedNavigationPermission()).Assert(); //BlessedAssert try { // Use the DemandWhenUnsafe navigation call to make it clear that // we could be navigating to an external Uri or another document. AppSecurityManager.SafeLaunchBrowserDemandWhenUnsafe( null, uri.Value, true); } finally { CodeAccessPermission.RevertAssert(); } return null; } /// /// Constructs the appropriate NavigationWindow styles, and assign them to the resources. /// private void SetupNavigationWindowStyle() { // Add the UI styles to the application resources. // For performance we build the basic NavWindow style here and insert it into // the resources (avoiding a FindResource call). // Setup the ContentPresenter that will hold the DocumentViewer FrameworkElementFactory docViewer = new FrameworkElementFactory(typeof(DocumentApplicationDocumentViewer), "PUIDocumentApplicationDocumentViewer"); DynamicResourceExtension styleKey = new DynamicResourceExtension(new ComponentResourceKey( typeof(PresentationUIStyleResources), "PUIDocumentApplicationDocumentViewerStyleKey")); docViewer.SetValue(FrameworkElement.StyleProperty, styleKey); docViewer.SetValue(FrameworkElement.OverridesDefaultStyleProperty, true); docViewer.SetValue(FrameworkElement.NameProperty, "PUIDocumentApplicationDocumentViewer"); docViewer.SetValue(NumberSubstitution.CultureSourceProperty, NumberCultureSource.User); docViewer.SetValue(DocumentViewer.DocumentProperty, new TemplateBindingExtension(Window.ContentProperty)); // Setup the ControlTemplate ControlTemplate controlTemplate = new ControlTemplate(typeof(NavigationWindow)); controlTemplate.VisualTree = docViewer; controlTemplate.Seal(); // Construct the NavigationWindow style and assign the control template. Style style = new Style(); style.Setters.Add(new Setter(Control.TemplateProperty, controlTemplate)); style.Seal(); // Assign the style to both of the Navigation Style Keys. Resources.Add(SystemParameters.NavigationChromeStyleKey, style); Resources.Add(SystemParameters.NavigationChromeDownLevelStyleKey, style); } ////// Registers the mime handlers for the appropriate mime types. /// private void SetupMimeHandlers() { // Setup the fixed document mime handler. StreamToObjectFactoryDelegate docFactory = new StreamToObjectFactoryDelegate(S0ConverterDoc); MimeObjectFactory.Register(MimeTypeMapper.FixedDocumentSequenceMime, docFactory); // Setup the resource dictionary mime handler. StreamToObjectFactoryDelegate resDictFactory = new StreamToObjectFactoryDelegate(S0ConverterResDict); MimeObjectFactory.Register(MimeTypeMapper.ResourceDictionaryMime, resDictFactory); // Setup the mime handler for invalid mime types StreamToObjectFactoryDelegate notSupportedFactory = new StreamToObjectFactoryDelegate(NotSupportedConverter); // dangerous types MimeObjectFactory.Register(MimeTypeMapper.BamlMime, notSupportedFactory); MimeObjectFactory.Register(MimeTypeMapper.XamlMime, notSupportedFactory); // out of spec types MimeObjectFactory.Register(MimeTypeMapper.FixedDocumentMime, notSupportedFactory); MimeObjectFactory.Register(MimeTypeMapper.FixedPageMime, notSupportedFactory); } ////// /// ////// Critical - 1) This code uses the rootbrowserwindow which is critical. /// 2) This method calls InitializeUI and passes in a reference to the /// DocumentRightsManagementManager. /// TreatAsSafe - 1) Since the window reference is only used to find the DocumentViewer. /// 2) Since the DocumentRightsManagementManager is constructed locally within /// this class. /// [SecurityCritical, SecurityTreatAsSafe] private void InitializeDocumentApplicationDocumentViewer() { // Set references to UI objects. NavigationWindow appWin = GetAppWindow(); // Ensure NavigationWindow is valid if (appWin == null) { throw new NotSupportedException(SR.Get(SRID.DocumentApplicationCannotInitializeUI)); } // This will guarantee that the required styles have been loaded, and that all // required controls will be available appWin.ApplyTemplate(); // Find the DocumentViewer in the current style. This will be used by // DocumentApplicationUI to find all UI controls. The appWin would work // here, however, for security the reference to the appWin (RootBrowserWindow) // is released at the end of this method. _docViewer = appWin.Template.FindName("PUIDocumentApplicationDocumentViewer", appWin) as DocumentApplicationDocumentViewer; if (_docViewer == null) { throw new NotSupportedException(SR.Get(SRID.DocumentApplicationCannotInitializeUI)); } // Setup DocumentApplicationDocumentViewer's IDocumentScrollInfo for viewing modes. IDocumentScrollInfo idsi = _docViewer.DocumentScrollInfo; idsi.LockViewModes = true; // Initialize and verify the UI try { _docViewer.InitializeUI(_docSigManager, _docRMManager); } // Allow empty catch statements. #pragma warning disable 56500 catch (Exception exceptionArgs) { // Throw assert with the exception details Invariant.Assert(false, exceptionArgs.Message, exceptionArgs.ToString()); } // Disallow empty catch statements. #pragma warning restore 56500 // Setup a handler to add custom journal entries _docViewer.AddJournalEntry += OnAddJournalEntry; } #endregion Private Methods #region Internal Methods ////// This is called when the Text dependency property changes in the Window. /// ////// Critical - calls SetStatusText which is SUC'ed. /// TreatAsSafe - setting the status bar text of the browser considered safe. /// IE/MSRC does not consider hostile setting of text ( even for hyperlink spoofing) /// to be an exploit. /// [SecurityCritical, SecurityTreatAsSafe] internal void InitUI() { // Set Statusbar BrowserCallbackServices.SetStatusText(SR.Get(SRID.DocumentApplicationStatusLoaded)); } ////// This will convert the incomming stream to a FixedDocument or FixedDocumentSequence. /// It uses the XpsS0SchemaValiator if the stream is not valid it will throw. /// NOTE: Method is not strongly types to match StreamToObjectFactoryDelegate /// /// Stream to process /// baseUri to resolve to /// parameter indicating that navigation can use the top level browser if we fail to produce an object /// provide secure, isolated content if possible /// Should we allow async loading of this content /// Was this navigation the result of a journal operation /// ///A FixedDocument or FixedDocumentSequence. private object S0ConverterDoc(Stream stream, Uri baseUri, bool canUseTopLevelBrowser, bool sandboxExternalContent, bool allowAsync, bool isJournalNavigation, out XamlReader asyncObjectConverter) { ParserContext pc = new ParserContext(); pc.BaseUri = baseUri; pc.SkipJournaledProperties = false; // assuming that the S0 schema will never have any state that needs to be journaled pc.XamlTypeMapper = XmlParserDefaults.DefaultMapper; XpsValidatingLoader.AssertDocumentMode(); XpsValidatingLoader loader = new XpsValidatingLoader(); object document = loader.Load(stream, null, pc, MimeTypeMapper.FixedDocumentSequenceMime); asyncObjectConverter = null; if (document is FixedDocumentSequence) { // Since a FixedDocumentSequence was found allow FixedDocuments to be loaded. _isInitialDocSeqLoaded = true; } // Check if FixedDocument is allowed, if so attempt to load it // This case will filter the invalid document load attempts. else if (!_isInitialDocSeqLoaded || !(document is FixedDocument)) { // Did not throw directly as this will // a. increase block coverage ;) // b. consolidate behavior to one place NotSupportedConverter(null, null, false, false, allowAsync, false, out asyncObjectConverter); } return document; } ////// This will convert the incomming stream to a ResourceDictionary. /// It uses the XpsS0SchemaValiator if the stream is not valid it will throw. /// NOTE: Method is not strongly types to match StreamToObjectFactoryDelegate /// /// Stream to process /// baseUri to resolve to /// parameter indicating that navigation can use the top level browser if we fail to produce an object /// provide secure, isolated content if possible /// Should we allow async loading of this content /// Was this navigation the result of a journal operation /// ///A ResourceDictionary. private object S0ConverterResDict(Stream stream, Uri baseUri, bool canUseTopLevelBrowser, bool sandboxExternalContent, bool allowAsync, bool isJournalNavigation, out XamlReader asyncObjectConverter) { ParserContext pc = new ParserContext(); pc.BaseUri = baseUri; pc.SkipJournaledProperties = false; // assuming that the S0 schema will never have any state that needs to be journaled pc.XamlTypeMapper = XmlParserDefaults.DefaultMapper; XpsValidatingLoader loader = new XpsValidatingLoader(); ResourceDictionary resDict = loader.Load(stream, baseUri, pc, MimeTypeMapper.ResourceDictionaryMime) as ResourceDictionary; asyncObjectConverter = null; if (resDict == null) { // Did not throw directly as this will // a. increase block coverage ;) // b. consolidate behavior to one place NotSupportedConverter(null, null, false, false, allowAsync, false, out asyncObjectConverter); } return resDict; } ////// This will throw an exception, since the document type is not supported. /// /// Stream to process /// baseUri to resolve to /// parameter indicating that navigation can use the top level browser if we fail to produce an object /// provide secure, isolated content if possible /// Should we allow async loading of this content /// Was this navigation the result of a journal operation /// ///private object NotSupportedConverter(Stream stream, Uri baseUri, bool canUseTopLevelBrowser, bool sandboxExternalContent, bool allowAsync, bool isJournalNavigation, out XamlReader asyncObjectConverter) { asyncObjectConverter = null; // Since the document type contained in the stream is not supported, this will throw. throw new NotSupportedException(SR.Get(SRID.DocumentApplicationUnknownFileFormat)); } /// /// Creates a new journal entry on the NavWindow's backstack that stores the current /// UI state. /// /// /// ////// Critical - This code uses the rootbrowserwindow which is critical /// TreatAsSafe - Since the window reference is only used to add a journal entry. /// [SecurityCritical, SecurityTreatAsSafe] private void OnAddJournalEntry(object sender, DocumentApplicationJournalEntryEventArgs e) { NavigationWindow appWin = GetAppWindow(); if ((appWin != null) && (e != null)) { // Create a new DocumentApplicationJournalEntry and add to back stack appWin.AddBackEntry(new DocumentApplicationJournalEntry(e.State)); } } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private DocumentApplicationDocumentViewer _docViewer; private DocumentSignatureManager _docSigManager; private DocumentRightsManagementManager _docRMManager; private bool _isInitialDocSeqLoaded; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
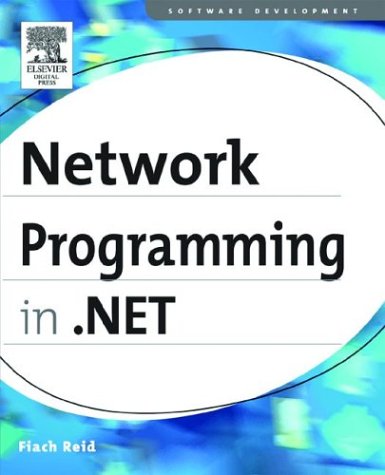
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MessageContractMemberAttribute.cs
- ChangesetResponse.cs
- WebControl.cs
- FontStretches.cs
- HandleCollector.cs
- BooleanKeyFrameCollection.cs
- TextEditorTyping.cs
- DropTarget.cs
- HttpCapabilitiesBase.cs
- CroppedBitmap.cs
- EdmError.cs
- ToolboxItemFilterAttribute.cs
- DesignerCatalogPartChrome.cs
- Configuration.cs
- CheckBox.cs
- CompiledQuery.cs
- EventDescriptor.cs
- ConfigXmlComment.cs
- ReflectPropertyDescriptor.cs
- ContextStaticAttribute.cs
- ChooseAction.cs
- ManipulationDeltaEventArgs.cs
- NativeMethods.cs
- TextViewSelectionProcessor.cs
- MethodCallConverter.cs
- OdbcCommandBuilder.cs
- ChooseAction.cs
- DataGridColumnCollection.cs
- DataGridViewRowPostPaintEventArgs.cs
- ArglessEventHandlerProxy.cs
- IsolatedStorageException.cs
- ScriptingJsonSerializationSection.cs
- FieldDescriptor.cs
- ObjectStateEntry.cs
- ColumnPropertiesGroup.cs
- DataError.cs
- XmlSerializer.cs
- SemanticTag.cs
- TabRenderer.cs
- FlowDocumentScrollViewer.cs
- WebAdminConfigurationHelper.cs
- LogReserveAndAppendState.cs
- RegexStringValidatorAttribute.cs
- XamlStream.cs
- RemotingConfiguration.cs
- TextEndOfParagraph.cs
- XPathScanner.cs
- TextClipboardData.cs
- EntityKey.cs
- MSHTMLHostUtil.cs
- AdobeCFFWrapper.cs
- HtmlInputRadioButton.cs
- DataObjectAttribute.cs
- NameSpaceEvent.cs
- CircleHotSpot.cs
- CollectionContainer.cs
- _BasicClient.cs
- OleDbPropertySetGuid.cs
- OpCopier.cs
- ConfigurationElementProperty.cs
- EncodingInfo.cs
- DesignerActionPropertyItem.cs
- LeftCellWrapper.cs
- RuleInfoComparer.cs
- ScrollChangedEventArgs.cs
- XpsException.cs
- WebPartMenuStyle.cs
- ControlHelper.cs
- PrintDialogDesigner.cs
- Rect3DValueSerializer.cs
- NewArrayExpression.cs
- KeyFrames.cs
- RectAnimation.cs
- FormViewPageEventArgs.cs
- EvidenceBase.cs
- TripleDESCryptoServiceProvider.cs
- UpdateTranslator.cs
- BoundsDrawingContextWalker.cs
- TabControlEvent.cs
- DesignerTextBoxAdapter.cs
- SqlServer2KCompatibilityAnnotation.cs
- ParentQuery.cs
- ResourceDisplayNameAttribute.cs
- PreProcessor.cs
- PathFigureCollectionConverter.cs
- SoapCommonClasses.cs
- SqlUtils.cs
- DbConnectionPoolIdentity.cs
- UrlUtility.cs
- URLAttribute.cs
- ArraySegment.cs
- EntityDesignPluralizationHandler.cs
- TableCellCollection.cs
- MailWriter.cs
- EncryptedXml.cs
- TextEffect.cs
- SqlErrorCollection.cs
- FlowchartDesigner.xaml.cs
- GetUserPreferenceRequest.cs
- BuildManagerHost.cs