Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / CompMod / System / ComponentModel / Design / Serialization / CodeDomSerializerException.cs / 1 / CodeDomSerializerException.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel.Design.Serialization { using System; using System.CodeDom; using System.Runtime.Serialization; ////// /// [Serializable] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1032:ImplementStandardExceptionConstructors")] public class CodeDomSerializerException : SystemException { private CodeLinePragma linePragma; ///The exception that is thrown when the code dom serializer experiences an error. /// ////// /// public CodeDomSerializerException(string message, CodeLinePragma linePragma) : base(message) { this.linePragma = linePragma; } ///Initializes a new instance of the CodeDomSerializerException class. ////// /// public CodeDomSerializerException(Exception ex, CodeLinePragma linePragma) : base(ex.Message, ex) { this.linePragma = linePragma; } ///Initializes a new instance of the CodeDomSerializerException class. ////// /// public CodeDomSerializerException(string message, IDesignerSerializationManager manager) : base(message) { FillLinePragmaFromContext(manager); } ///Initializes a new instance of the CodeDomSerializerException class. ////// /// public CodeDomSerializerException(Exception ex, IDesignerSerializationManager manager) : base(ex.Message, ex) { FillLinePragmaFromContext(manager); } ///Initializes a new instance of the CodeDomSerializerException class. ///protected CodeDomSerializerException(SerializationInfo info, StreamingContext context) : base (info, context) { linePragma = (CodeLinePragma)info.GetValue("linePragma", typeof(CodeLinePragma)); } /// /// /// public CodeLinePragma LinePragma { get { return linePragma; } } ///Gets the line pragma object that is related to this error. ////// Sniffs around in the context looking for a code statement. if it finds one, it will add the statement's /// line # information to the exception. /// private void FillLinePragmaFromContext(IDesignerSerializationManager manager) { if (manager == null) throw new ArgumentNullException("manager"); CodeStatement statement = (CodeStatement)manager.Context[typeof(CodeStatement)]; CodeLinePragma linePragma = null; if (statement != null) { linePragma = statement.LinePragma; } } ///public override void GetObjectData(SerializationInfo info, StreamingContext context) { if (info==null) { throw new ArgumentNullException("info"); } info.AddValue("linePragma", linePragma); base.GetObjectData(info, context); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
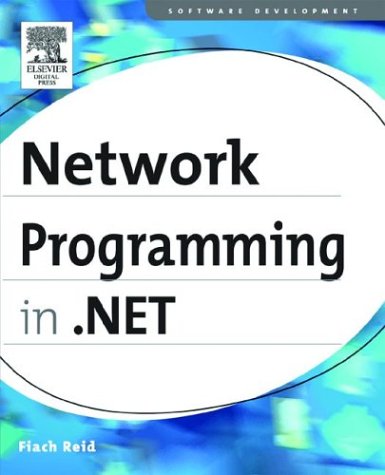
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- webeventbuffer.cs
- WindowsGraphicsCacheManager.cs
- OdbcHandle.cs
- AuthStoreRoleProvider.cs
- DummyDataSource.cs
- BezierSegment.cs
- ChtmlMobileTextWriter.cs
- ToolboxSnapDragDropEventArgs.cs
- TrayIconDesigner.cs
- XmlUnspecifiedAttribute.cs
- SecurityHelper.cs
- XmlWriter.cs
- AssemblyCollection.cs
- WindowProviderWrapper.cs
- ApplicationException.cs
- DataGridPagerStyle.cs
- SByteConverter.cs
- DateTimePicker.cs
- PrincipalPermission.cs
- ExpressionNormalizer.cs
- ReadOnlyHierarchicalDataSourceView.cs
- ButtonAutomationPeer.cs
- CfgArc.cs
- Stack.cs
- ClonableStack.cs
- ValidateNames.cs
- LocalizeDesigner.cs
- MultiSelector.cs
- Column.cs
- CalendarDesigner.cs
- RenderContext.cs
- ValidateNames.cs
- ComponentChangingEvent.cs
- Int16AnimationUsingKeyFrames.cs
- SingleStorage.cs
- EncryptedPackage.cs
- EntityDataSourceSelectedEventArgs.cs
- TimeStampChecker.cs
- FilterRepeater.cs
- WindowsFormsHostAutomationPeer.cs
- Inflater.cs
- XmlDesigner.cs
- DataGridViewAccessibleObject.cs
- Ipv6Element.cs
- SchemaTypeEmitter.cs
- TypeDescriptionProvider.cs
- TreeNodeMouseHoverEvent.cs
- CellTreeNodeVisitors.cs
- latinshape.cs
- OrderedDictionary.cs
- regiisutil.cs
- FolderLevelBuildProviderCollection.cs
- Panel.cs
- SafeNativeMethods.cs
- DataGridViewRowPostPaintEventArgs.cs
- BufferedStream2.cs
- SqlGenericUtil.cs
- DbExpressionVisitor.cs
- XmlDocumentFragment.cs
- WizardStepBase.cs
- CodeAccessPermission.cs
- TraceHandlerErrorFormatter.cs
- DataGridViewMethods.cs
- RangeContentEnumerator.cs
- HostProtectionException.cs
- NativeMethods.cs
- returneventsaver.cs
- GridView.cs
- SignatureHelper.cs
- PassportAuthentication.cs
- DateRangeEvent.cs
- Tuple.cs
- HttpWebRequest.cs
- FilterElement.cs
- ActivityValidationServices.cs
- EraserBehavior.cs
- EncoderParameters.cs
- Rotation3D.cs
- GreenMethods.cs
- XmlSchemaGroup.cs
- MetadataUtil.cs
- RoleServiceManager.cs
- ProcessHostMapPath.cs
- ImageSource.cs
- DbConnectionClosed.cs
- SqlUDTStorage.cs
- XmlSchemaComplexType.cs
- BezierSegment.cs
- PenThread.cs
- PolicyLevel.cs
- Collection.cs
- ApplicationSecurityManager.cs
- UMPAttributes.cs
- JournalNavigationScope.cs
- VerificationAttribute.cs
- WebContext.cs
- HostingEnvironmentSection.cs
- BaseCAMarshaler.cs
- OleDbRowUpdatedEvent.cs
- RowUpdatingEventArgs.cs