Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Net / System / Net / _OSSOCK.cs / 2 / _OSSOCK.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System.Net.Sockets; using System.Runtime.InteropServices; // // Argument structure for IP_ADD_MEMBERSHIP and IP_DROP_MEMBERSHIP. // [StructLayout(LayoutKind.Sequential)] internal struct IPMulticastRequest { internal int MulticastAddress; // IP multicast address of group internal int InterfaceAddress; // local IP address of interface internal static readonly int Size = Marshal.SizeOf(typeof(IPMulticastRequest)); } [StructLayout(LayoutKind.Sequential)] internal struct Linger { internal short OnOff; // option on/off internal short Time; // linger time } [StructLayout(LayoutKind.Sequential)] internal struct WSABuffer { internal int Length; // Length of Buffer internal IntPtr Pointer;// Pointer to Buffer } [StructLayout(LayoutKind.Sequential)] internal class TransmitFileBuffers { internal IntPtr preBuffer;// Pointer to Buffer internal int preBufferLength; // Length of Buffer internal IntPtr postBuffer;// Pointer to Buffer internal int postBufferLength; // Length of Buffer } [StructLayout(LayoutKind.Sequential)] internal struct WSAData { internal short wVersion; internal short wHighVersion; [MarshalAs(UnmanagedType.ByValTStr, SizeConst=257)] internal string szDescription; [MarshalAs(UnmanagedType.ByValTStr, SizeConst=129)] internal string szSystemStatus; internal short iMaxSockets; internal short iMaxUdpDg; internal IntPtr lpVendorInfo; } // data structures and types needed for getaddrinfo calls. [StructLayout(LayoutKind.Sequential, CharSet=CharSet.Ansi)] internal unsafe struct AddressInfo { internal AddressInfoHints ai_flags; internal AddressFamily ai_family; internal SocketType ai_socktype; internal ProtocolFamily ai_protocol; internal int ai_addrlen; internal sbyte* ai_canonname; // Ptr to the cannonical name - check for NULL internal byte* ai_addr; // Ptr to the sockaddr structure internal AddressInfo* ai_next; // Ptr to the next AddressInfo structure } [Flags] internal enum AddressInfoHints { AI_PASSIVE = 0x01, /* Socket address will be used in bind() call */ AI_CANONNAME = 0x02, /* Return canonical name in first ai_canonname */ AI_NUMERICHOST = 0x04, /* Nodename must be a numeric address string */ } [Flags] internal enum NameInfoFlags { NI_NOFQDN = 0x01, /* Only return nodename portion for local hosts */ NI_NUMERICHOST = 0x02, /* Return numeric form of the host's address */ NI_NAMEREQD = 0x04, /* Error if the host's name not in DNS */ NI_NUMERICSERV = 0x08, /* Return numeric form of the service (port #) */ NI_DGRAM = 0x10, /* Service is a datagram service */ } // Argument structure for IPV6_ADD_MEMBERSHIP and IPV6_DROP_MEMBERSHIP. [StructLayout(LayoutKind.Sequential)] internal struct IPv6MulticastRequest { [MarshalAs(UnmanagedType.ByValArray,SizeConst=16)] internal byte[] MulticastAddress; // IP address of group internal int InterfaceIndex; // local interface index internal static readonly int Size = Marshal.SizeOf(typeof(IPv6MulticastRequest)); } // // used as last parameter to WSASocket call // [Flags] internal enum SocketConstructorFlags { WSA_FLAG_OVERLAPPED = 0x01, WSA_FLAG_MULTIPOINT_C_ROOT = 0x02, WSA_FLAG_MULTIPOINT_C_LEAF = 0x04, WSA_FLAG_MULTIPOINT_D_ROOT = 0x08, WSA_FLAG_MULTIPOINT_D_LEAF = 0x10, } } // namespace System.Net
Link Menu
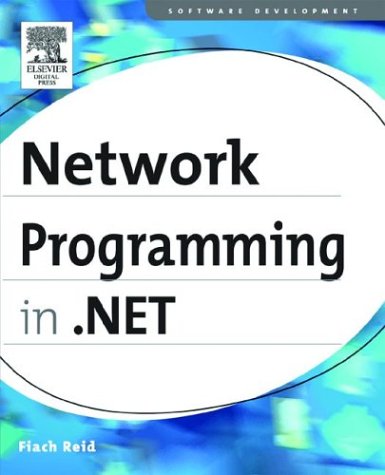
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ActiveXHost.cs
- XXXInfos.cs
- TreeViewImageGenerator.cs
- GeneralTransform3D.cs
- EtwTrackingBehavior.cs
- AttributeAction.cs
- TempFiles.cs
- PropertyInfoSet.cs
- _NTAuthentication.cs
- StyleHelper.cs
- ObfuscateAssemblyAttribute.cs
- _PooledStream.cs
- SingleAnimation.cs
- ConfigurationManagerHelperFactory.cs
- Span.cs
- DragCompletedEventArgs.cs
- Point4DValueSerializer.cs
- TransactionState.cs
- BaseAsyncResult.cs
- DWriteFactory.cs
- PassportAuthenticationModule.cs
- ZoneMembershipCondition.cs
- CacheDict.cs
- ContextMenuStrip.cs
- AdornerHitTestResult.cs
- BamlTreeUpdater.cs
- SQLGuid.cs
- LoginView.cs
- RawTextInputReport.cs
- TransformerConfigurationWizardBase.cs
- DataMemberConverter.cs
- WorkflowMarkupSerializationManager.cs
- CodeSnippetTypeMember.cs
- _ConnectStream.cs
- XmlEncoding.cs
- InstancePersistenceContext.cs
- RepeatButtonAutomationPeer.cs
- PageParser.cs
- StickyNoteAnnotations.cs
- StyleTypedPropertyAttribute.cs
- StateMachineWorkflowInstance.cs
- SqlParameterCollection.cs
- DispatcherProcessingDisabled.cs
- Rule.cs
- SettingsPropertyIsReadOnlyException.cs
- RegexEditorDialog.cs
- OpenTypeCommon.cs
- EventNotify.cs
- TypeToArgumentTypeConverter.cs
- Attributes.cs
- ColumnBinding.cs
- WebRequestModuleElement.cs
- StrokeNode.cs
- SQLMembershipProvider.cs
- QuaternionValueSerializer.cs
- AttributeInfo.cs
- DependencyPropertyKind.cs
- SpellCheck.cs
- NameTable.cs
- Triangle.cs
- PresentationTraceSources.cs
- ProxyManager.cs
- TimeSpanParse.cs
- DrawListViewItemEventArgs.cs
- GeneralTransform2DTo3DTo2D.cs
- RequestSecurityTokenForGetBrowserToken.cs
- ExportOptions.cs
- HMACSHA1.cs
- QueryTask.cs
- ProcessModuleCollection.cs
- SafeFileMappingHandle.cs
- ExpandableObjectConverter.cs
- BinaryConverter.cs
- ScrollViewerAutomationPeer.cs
- MimeReflector.cs
- _ConnectionGroup.cs
- ByteAnimationBase.cs
- Configuration.cs
- TextSyndicationContentKindHelper.cs
- BinaryNode.cs
- querybuilder.cs
- SettingsSection.cs
- StylusPointProperties.cs
- BevelBitmapEffect.cs
- SecurityRuntime.cs
- TextElement.cs
- AssociatedControlConverter.cs
- PropertyToken.cs
- ClientSettingsSection.cs
- OdbcErrorCollection.cs
- CellCreator.cs
- TreeViewHitTestInfo.cs
- DataFormats.cs
- ProfilePropertySettingsCollection.cs
- RadialGradientBrush.cs
- WSSecurityPolicy.cs
- DataGridViewCellStateChangedEventArgs.cs
- SecurityUniqueId.cs
- CharEntityEncoderFallback.cs
- OciEnlistContext.cs