Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / Compilation / SimpleHandlerBuildProvider.cs / 2 / SimpleHandlerBuildProvider.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Compilation { using System; using System.IO; using System.Collections; using System.Reflection; using System.CodeDom; using System.CodeDom.Compiler; using System.Web.Configuration; using System.Web.Util; using System.Web.UI; #if ORCAS using System.Web.UI.Imaging; #endif [BuildProviderAppliesTo(BuildProviderAppliesTo.Web)] internal abstract class SimpleHandlerBuildProvider: InternalBuildProvider { private SimpleWebHandlerParser _parser; internal override IAssemblyDependencyParser AssemblyDependencyParser { get { return _parser; } } protected abstract SimpleWebHandlerParser CreateParser(); public override CompilerType CodeCompilerType { get { Debug.Assert(_parser == null); _parser = CreateParser(); _parser.SetBuildProvider(this); _parser.IgnoreParseErrors = IgnoreParseErrors; _parser.Parse(ReferencedAssemblies); return _parser.CompilerType; } } protected internal override CodeCompileUnit GetCodeCompileUnit(out IDictionary linePragmasTable) { Debug.Assert(_parser != null); CodeCompileUnit ccu = _parser.GetCodeModel(); linePragmasTable = _parser.GetLinePragmasTable(); return ccu; } public override void GenerateCode(AssemblyBuilder assemblyBuilder) { CodeCompileUnit codeCompileUnit = _parser.GetCodeModel(); // Bail if we have nothing we need to compile if (codeCompileUnit == null) return; assemblyBuilder.AddCodeCompileUnit(this, codeCompileUnit); // Add all the assemblies if (_parser.AssemblyDependencies != null) { foreach (Assembly assembly in _parser.AssemblyDependencies) { assemblyBuilder.AddAssemblyReference(assembly, codeCompileUnit); } } // NOTE: we can't actually generate the fast factory because it would give // a really bad error if the user specifies a classname which doesn't match // the actual class they define. A bit unfortunate, but not that big a deal... // tell the host to generate a fast factory for this type (if any) //string generatedTypeName = _parser.GeneratedTypeName; //if (generatedTypeName != null) // assemblyBuilder.GenerateTypeFactory(generatedTypeName); } public override Type GetGeneratedType(CompilerResults results) { Type t; if (_parser.HasInlineCode) { // This is the case where the asmx/ashx has code in the file, and it // has been compiled. Debug.Assert(results != null); t = _parser.GetTypeToCache(results.CompiledAssembly); } else { // This is the case where the asmx/ashx has no code and is simply // pointing to an existing assembly. Set the UsesExistingAssembly // flag accordingly. t = _parser.GetTypeToCache(null); } return t; } public override ICollection VirtualPathDependencies { get { return _parser.SourceDependencies; } } internal CompilerType GetDefaultCompilerTypeForLanguageInternal(string language) { return GetDefaultCompilerTypeForLanguage(language); } internal CompilerType GetDefaultCompilerTypeInternal() { return GetDefaultCompilerType(); } internal TextReader OpenReaderInternal() { return OpenReader(); } internal override ICollection GetGeneratedTypeNames() { // Note that _parser.TypeName does not necessarily point to the type defined in the handler file, // it could be any type that can be referenced at runtime, App_Code for example. return new SingleObjectCollection(_parser.TypeName); } } #if ORCAS internal class ImageGeneratorBuildProvider: SimpleHandlerBuildProvider { private ImageGeneratorParser _parser; protected override SimpleWebHandlerParser CreateParser() { _parser = new ImageGeneratorParser(VirtualPath); return _parser; } internal override BuildResultCompiledType CreateBuildResult(Type t) { return new ImageGeneratorBuildResultCompiledType(t); } internal override BuildResult CreateBuildResult(CompilerResults results) { ImageGeneratorBuildResultCompiledType result = (ImageGeneratorBuildResultCompiledType)base.CreateBuildResult(results); result.OutputCacheInfo = _parser.OutputCacheDirective; result.CustomErrorImageUrl = _parser.CustomErrorImageUrl; return result; } } #endif internal class WebServiceBuildProvider: SimpleHandlerBuildProvider { protected override SimpleWebHandlerParser CreateParser() { return new WebServiceParser(VirtualPath); } } internal class WebHandlerBuildProvider: SimpleHandlerBuildProvider { protected override SimpleWebHandlerParser CreateParser() { return new WebHandlerParser(VirtualPath); } } }
Link Menu
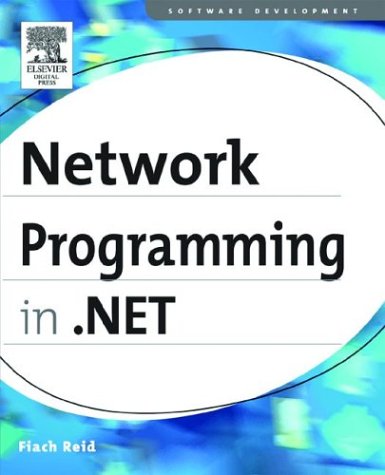
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HWStack.cs
- DataGridViewCell.cs
- XsltOutput.cs
- UnsafeNativeMethods.cs
- RelationshipFixer.cs
- MachineKeyValidationConverter.cs
- XPathMultyIterator.cs
- BaseTreeIterator.cs
- LinearGradientBrush.cs
- ScrollableControl.cs
- DoubleAnimationUsingKeyFrames.cs
- ImageAutomationPeer.cs
- DataGridViewRowsAddedEventArgs.cs
- DSACryptoServiceProvider.cs
- PartialTrustVisibleAssembliesSection.cs
- InternalBufferOverflowException.cs
- MetadataCollection.cs
- COM2IProvidePropertyBuilderHandler.cs
- FactoryId.cs
- AssemblyFilter.cs
- TraceSource.cs
- UriWriter.cs
- SetterBase.cs
- Internal.cs
- Misc.cs
- autovalidator.cs
- DataBindingsDialog.cs
- HandledMouseEvent.cs
- SymDocumentType.cs
- altserialization.cs
- SplitterPanel.cs
- RewritingPass.cs
- HttpPostedFile.cs
- TemplateParser.cs
- Triplet.cs
- ButtonFieldBase.cs
- DBCommand.cs
- PropertyValueUIItem.cs
- RemotingService.cs
- ControlPropertyNameConverter.cs
- OledbConnectionStringbuilder.cs
- FrameworkTextComposition.cs
- PagerStyle.cs
- SqlCacheDependencySection.cs
- Knowncolors.cs
- ToolStrip.cs
- ObjectIDGenerator.cs
- BigInt.cs
- DataGridCaption.cs
- TextAnchor.cs
- ReadOnlyCollection.cs
- MatrixAnimationBase.cs
- SafeNativeMethodsMilCoreApi.cs
- WorkflowViewService.cs
- Visual3D.cs
- ConstructorExpr.cs
- TreeBuilder.cs
- StrokeRenderer.cs
- QueryContext.cs
- Section.cs
- CacheOutputQuery.cs
- XPathAncestorIterator.cs
- ObjectStateEntryOriginalDbUpdatableDataRecord.cs
- IdentifierElement.cs
- AsymmetricKeyExchangeDeformatter.cs
- TransformPattern.cs
- InvalidProgramException.cs
- TextServicesCompartmentEventSink.cs
- CodeMemberEvent.cs
- RelationshipEndMember.cs
- AsyncResult.cs
- MulticastNotSupportedException.cs
- KoreanCalendar.cs
- Merger.cs
- DesignTimeVisibleAttribute.cs
- UserNameSecurityTokenParameters.cs
- XmlResolver.cs
- NavigationPropertyEmitter.cs
- XmlSchemaSubstitutionGroup.cs
- TableCellCollection.cs
- EdmTypeAttribute.cs
- DynamicDiscoveryDocument.cs
- LicenseContext.cs
- AndAlso.cs
- InkPresenterAutomationPeer.cs
- SelectQueryOperator.cs
- EntityReference.cs
- XMLSyntaxException.cs
- EventTask.cs
- ListCollectionView.cs
- RightNameExpirationInfoPair.cs
- XPathChildIterator.cs
- BigInt.cs
- CodeMethodReturnStatement.cs
- RegexStringValidator.cs
- FacetDescriptionElement.cs
- X509SubjectKeyIdentifierClause.cs
- ErrorInfoXmlDocument.cs
- LogicalCallContext.cs
- EntityTypeBase.cs