Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Input / Stylus / StylusPointProperties.cs / 1 / StylusPointProperties.cs
//------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Input; using System.Windows.Media; using System.Collections.Generic; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ////// StylusPointProperties /// public static class StylusPointProperties { ////// X /// public static readonly StylusPointProperty X = new StylusPointProperty( StylusPointPropertyIds.X, false); ////// Y /// public static readonly StylusPointProperty Y = new StylusPointProperty( StylusPointPropertyIds.Y, false); ////// Z /// public static readonly StylusPointProperty Z = new StylusPointProperty( StylusPointPropertyIds.Z, false); ////// Width /// public static readonly StylusPointProperty Width = new StylusPointProperty(StylusPointPropertyIds.Width, false); ////// Height /// public static readonly StylusPointProperty Height = new StylusPointProperty(StylusPointPropertyIds.Height, false); ////// SystemContact /// public static readonly StylusPointProperty SystemTouch = new StylusPointProperty(StylusPointPropertyIds.SystemTouch, false); ////// PacketStatus /// public static readonly StylusPointProperty PacketStatus = new StylusPointProperty( StylusPointPropertyIds.PacketStatus, false); ////// SerialNumber /// public static readonly StylusPointProperty SerialNumber = new StylusPointProperty(StylusPointPropertyIds.SerialNumber, false); ////// NormalPressure /// public static readonly StylusPointProperty NormalPressure = new StylusPointProperty( StylusPointPropertyIds.NormalPressure, false); ////// TangentPressure /// public static readonly StylusPointProperty TangentPressure = new StylusPointProperty( StylusPointPropertyIds.TangentPressure, false); ////// ButtonPressure /// public static readonly StylusPointProperty ButtonPressure = new StylusPointProperty( StylusPointPropertyIds.ButtonPressure, false); ////// XTiltOrientation /// public static readonly StylusPointProperty XTiltOrientation = new StylusPointProperty( StylusPointPropertyIds.XTiltOrientation, false); ////// YTiltOrientation /// public static readonly StylusPointProperty YTiltOrientation = new StylusPointProperty( StylusPointPropertyIds.YTiltOrientation, false); ////// AzimuthOrientation /// public static readonly StylusPointProperty AzimuthOrientation = new StylusPointProperty( StylusPointPropertyIds.AzimuthOrientation, false); ////// AltitudeOrientation /// public static readonly StylusPointProperty AltitudeOrientation = new StylusPointProperty( StylusPointPropertyIds.AltitudeOrientation, false); ////// TwistOrientation /// public static readonly StylusPointProperty TwistOrientation = new StylusPointProperty( StylusPointPropertyIds.TwistOrientation, false); ////// PitchRotation /// public static readonly StylusPointProperty PitchRotation = new StylusPointProperty( StylusPointPropertyIds.PitchRotation, false); ////// RollRotation /// public static readonly StylusPointProperty RollRotation = new StylusPointProperty(StylusPointPropertyIds.RollRotation, false); ////// YawRotation /// public static readonly StylusPointProperty YawRotation = new StylusPointProperty(StylusPointPropertyIds.YawRotation, false); ////// TipButton /// public static readonly StylusPointProperty TipButton = new StylusPointProperty(StylusPointPropertyIds.TipButton, true); ////// BarrelButton /// public static readonly StylusPointProperty BarrelButton = new StylusPointProperty(StylusPointPropertyIds.BarrelButton, true); ////// SecondaryTipButton /// public static readonly StylusPointProperty SecondaryTipButton = new StylusPointProperty(StylusPointPropertyIds.SecondaryTipButton, true); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Input; using System.Windows.Media; using System.Collections.Generic; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ////// StylusPointProperties /// public static class StylusPointProperties { ////// X /// public static readonly StylusPointProperty X = new StylusPointProperty( StylusPointPropertyIds.X, false); ////// Y /// public static readonly StylusPointProperty Y = new StylusPointProperty( StylusPointPropertyIds.Y, false); ////// Z /// public static readonly StylusPointProperty Z = new StylusPointProperty( StylusPointPropertyIds.Z, false); ////// Width /// public static readonly StylusPointProperty Width = new StylusPointProperty(StylusPointPropertyIds.Width, false); ////// Height /// public static readonly StylusPointProperty Height = new StylusPointProperty(StylusPointPropertyIds.Height, false); ////// SystemContact /// public static readonly StylusPointProperty SystemTouch = new StylusPointProperty(StylusPointPropertyIds.SystemTouch, false); ////// PacketStatus /// public static readonly StylusPointProperty PacketStatus = new StylusPointProperty( StylusPointPropertyIds.PacketStatus, false); ////// SerialNumber /// public static readonly StylusPointProperty SerialNumber = new StylusPointProperty(StylusPointPropertyIds.SerialNumber, false); ////// NormalPressure /// public static readonly StylusPointProperty NormalPressure = new StylusPointProperty( StylusPointPropertyIds.NormalPressure, false); ////// TangentPressure /// public static readonly StylusPointProperty TangentPressure = new StylusPointProperty( StylusPointPropertyIds.TangentPressure, false); ////// ButtonPressure /// public static readonly StylusPointProperty ButtonPressure = new StylusPointProperty( StylusPointPropertyIds.ButtonPressure, false); ////// XTiltOrientation /// public static readonly StylusPointProperty XTiltOrientation = new StylusPointProperty( StylusPointPropertyIds.XTiltOrientation, false); ////// YTiltOrientation /// public static readonly StylusPointProperty YTiltOrientation = new StylusPointProperty( StylusPointPropertyIds.YTiltOrientation, false); ////// AzimuthOrientation /// public static readonly StylusPointProperty AzimuthOrientation = new StylusPointProperty( StylusPointPropertyIds.AzimuthOrientation, false); ////// AltitudeOrientation /// public static readonly StylusPointProperty AltitudeOrientation = new StylusPointProperty( StylusPointPropertyIds.AltitudeOrientation, false); ////// TwistOrientation /// public static readonly StylusPointProperty TwistOrientation = new StylusPointProperty( StylusPointPropertyIds.TwistOrientation, false); ////// PitchRotation /// public static readonly StylusPointProperty PitchRotation = new StylusPointProperty( StylusPointPropertyIds.PitchRotation, false); ////// RollRotation /// public static readonly StylusPointProperty RollRotation = new StylusPointProperty(StylusPointPropertyIds.RollRotation, false); ////// YawRotation /// public static readonly StylusPointProperty YawRotation = new StylusPointProperty(StylusPointPropertyIds.YawRotation, false); ////// TipButton /// public static readonly StylusPointProperty TipButton = new StylusPointProperty(StylusPointPropertyIds.TipButton, true); ////// BarrelButton /// public static readonly StylusPointProperty BarrelButton = new StylusPointProperty(StylusPointPropertyIds.BarrelButton, true); ////// SecondaryTipButton /// public static readonly StylusPointProperty SecondaryTipButton = new StylusPointProperty(StylusPointPropertyIds.SecondaryTipButton, true); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
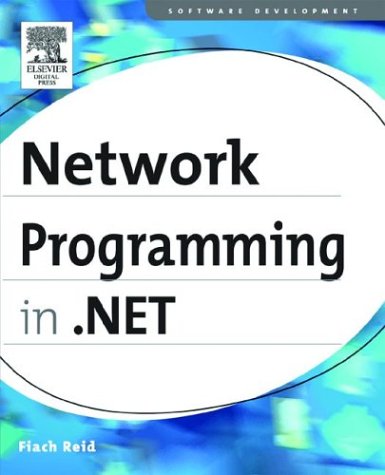
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartConnectVerb.cs
- OracleSqlParser.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- CompoundFileDeflateTransform.cs
- BitmapFrameEncode.cs
- ContentPlaceHolder.cs
- Random.cs
- StorageFunctionMapping.cs
- ExpressionCopier.cs
- XPathScanner.cs
- XmlnsPrefixAttribute.cs
- JoinCqlBlock.cs
- ObjectManager.cs
- BitmapPalettes.cs
- UidManager.cs
- NodeLabelEditEvent.cs
- InternalBufferOverflowException.cs
- TPLETWProvider.cs
- DictionaryKeyPropertyAttribute.cs
- Rect3D.cs
- ISFTagAndGuidCache.cs
- QueryOutputWriterV1.cs
- HttpStreamMessageEncoderFactory.cs
- MenuItem.cs
- KeyInstance.cs
- RayMeshGeometry3DHitTestResult.cs
- ProvidersHelper.cs
- IgnoreDeviceFilterElementCollection.cs
- AppDomainUnloadedException.cs
- TimeStampChecker.cs
- AdornerLayer.cs
- DurableMessageDispatchInspector.cs
- DocumentViewerHelper.cs
- AuthenticationSection.cs
- DataGridCommandEventArgs.cs
- HighlightVisual.cs
- EnumValAlphaComparer.cs
- SerializationStore.cs
- ReachSerializerAsync.cs
- ProfileSettings.cs
- SettingsContext.cs
- Attributes.cs
- CRYPTPROTECT_PROMPTSTRUCT.cs
- XmlElementAttribute.cs
- WhitespaceSignificantCollectionAttribute.cs
- PartialList.cs
- XhtmlTextWriter.cs
- ScopedKnownTypes.cs
- DateTimeConverter2.cs
- ColorMatrix.cs
- PackageRelationshipSelector.cs
- SystemFonts.cs
- WebPartDisplayModeEventArgs.cs
- SmtpNetworkElement.cs
- LocalizationCodeDomSerializer.cs
- StrictModeSecurityHeaderElementInferenceEngine.cs
- OciHandle.cs
- WebPartDisplayMode.cs
- RuntimeWrappedException.cs
- MatrixStack.cs
- PageRanges.cs
- GeneralTransform3D.cs
- SiteMapNodeItem.cs
- PEFileEvidenceFactory.cs
- CompoundFileStorageReference.cs
- RemoteWebConfigurationHostStream.cs
- SqlUtils.cs
- AttributeCollection.cs
- ClientSideQueueItem.cs
- RemotingConfigParser.cs
- FontUnit.cs
- CodeNamespaceImport.cs
- SiteMapNodeItem.cs
- SqlDataSourceCache.cs
- ToolboxComponentsCreatedEventArgs.cs
- RelatedView.cs
- ContractsBCL.cs
- ElementHostPropertyMap.cs
- GeometryGroup.cs
- RTLAwareMessageBox.cs
- PropertyGridEditorPart.cs
- EntityDataSourceState.cs
- DisableDpiAwarenessAttribute.cs
- TemplateControlBuildProvider.cs
- LexicalChunk.cs
- BinaryReader.cs
- VisualBasic.cs
- ExpandCollapseProviderWrapper.cs
- ViewRendering.cs
- UriParserTemplates.cs
- MulticastIPAddressInformationCollection.cs
- ConvertersCollection.cs
- RegisteredArrayDeclaration.cs
- MetaColumn.cs
- SingleConverter.cs
- Int64.cs
- Application.cs
- HttpTransportBindingElement.cs
- OptimalBreakSession.cs
- TagNameToTypeMapper.cs