Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / clr / src / BCL / System / Diagnostics / log.cs / 1 / log.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== namespace System.Diagnostics { using System.Runtime.Remoting; using System; using System.Security.Permissions; using System.IO; using System.Collections; using System.Runtime.CompilerServices; using Encoding = System.Text.Encoding; using System.Runtime.Versioning; // LogMessageEventHandlers are triggered when a message is generated which is // "on" per its switch. // // By default, the debugger (if attached) is the only event handler. // There is also a "built-in" console device which can be enabled // programatically, by registry (specifics....) or environment // variables. [Serializable()] [HostProtection(Synchronization=true, ExternalThreading=true)] internal delegate void LogMessageEventHandler(LoggingLevels level, LogSwitch category, String message, StackTrace location); // LogSwitchLevelHandlers are triggered when the level of a LogSwitch is modified // NOTE: These are NOT triggered when the log switch setting is changed from the // attached debugger. // [Serializable()] internal delegate void LogSwitchLevelHandler(LogSwitch ls, LoggingLevels newLevel); internal static class Log { // Switches allow relatively fine level control of which messages are // actually shown. Normally most debugging messages are not shown - the // user will typically enable those which are relevant to what is being // investigated. // // An attached debugger can enable or disable which messages will // actually be reported to the user through the COM+ debugger // services API. This info is communicated to the runtime so only // desired events are actually reported to the debugger. internal static Hashtable m_Hashtable; private static bool m_fConsoleDeviceEnabled; private static Stream[] m_rgStream; private static int m_iNumOfStreamDevices; private static int m_iStreamArraySize; //private static StreamWriter pConsole; internal static int iNumOfSwitches; //private static int iNumOfMsgHandlers; //private static int iMsgHandlerArraySize; private static LogMessageEventHandler _LogMessageEventHandler; private static LogSwitchLevelHandler _LogSwitchLevelHandler; private static Object locker; // Constant representing the global switch public static readonly LogSwitch GlobalSwitch; static Log() { m_Hashtable = new Hashtable(); m_fConsoleDeviceEnabled = false; m_rgStream = null; m_iNumOfStreamDevices = 0; m_iStreamArraySize = 0; //pConsole = null; //iNumOfMsgHandlers = 0; //iMsgHandlerArraySize = 0; locker = new Object(); // allocate the GlobalSwitch object GlobalSwitch = new LogSwitch ("Global", "Global Switch for this log"); GlobalSwitch.MinimumLevel = LoggingLevels.ErrorLevel; } public static void AddOnLogMessage(LogMessageEventHandler handler) { lock (locker) _LogMessageEventHandler = (LogMessageEventHandler) MulticastDelegate.Combine(_LogMessageEventHandler, handler); } public static void RemoveOnLogMessage(LogMessageEventHandler handler) { lock (locker) _LogMessageEventHandler = (LogMessageEventHandler) MulticastDelegate.Remove(_LogMessageEventHandler, handler); } public static void AddOnLogSwitchLevel(LogSwitchLevelHandler handler) { lock (locker) _LogSwitchLevelHandler = (LogSwitchLevelHandler) MulticastDelegate.Combine(_LogSwitchLevelHandler, handler); } public static void RemoveOnLogSwitchLevel(LogSwitchLevelHandler handler) { lock (locker) _LogSwitchLevelHandler = (LogSwitchLevelHandler) MulticastDelegate.Remove(_LogSwitchLevelHandler, handler); } internal static void InvokeLogSwitchLevelHandlers (LogSwitch ls, LoggingLevels newLevel) { LogSwitchLevelHandler handler = _LogSwitchLevelHandler; if (handler != null) handler(ls, newLevel); } // Property to Enable/Disable ConsoleDevice. Enabling the console device // adds the console device as a log output, causing any // log messages which make it through filters to be written to the // application console. The console device is enabled by default if the // ??? registry entry or ??? environment variable is set. public static bool IsConsoleEnabled { get { return m_fConsoleDeviceEnabled; } set { m_fConsoleDeviceEnabled = value; } } // AddStream uses the given stream to create and add a new log // device. Any log messages which make it through filters will be written // to the stream. // public static void AddStream(Stream stream) { if (stream==null) throw new ArgumentNullException("stream"); if (m_iStreamArraySize <= m_iNumOfStreamDevices) { // increase array size in chunks of 4 Stream[] newArray = new Stream [m_iStreamArraySize+4]; // copy the old array objects into the new one. if (m_iNumOfStreamDevices > 0) Array.Copy(m_rgStream, newArray, m_iNumOfStreamDevices); m_iStreamArraySize += 4; m_rgStream = newArray; } m_rgStream [m_iNumOfStreamDevices++] = stream; } // Generates a log message. If its switch (or a parent switch) allows the // level for the message, it is "broadcast" to all of the log // devices. // public static void LogMessage(LoggingLevels level, String message) { LogMessage (level, GlobalSwitch, message); } // Generates a log message. If its switch (or a parent switch) allows the // level for the message, it is "broadcast" to all of the log // devices. // [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Process, ResourceScope.Process)] public static void LogMessage(LoggingLevels level, LogSwitch logswitch, String message) { if (logswitch == null) throw new ArgumentNullException ("LogSwitch"); if (level < 0) throw new ArgumentOutOfRangeException("level", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegNum")); // Is logging for this level for this switch enabled? if (logswitch.CheckLevel (level) == true) { // Send message for logging // first send it to the debugger Debugger.Log ((int) level, logswitch.strName, message); // Send to the console device if (m_fConsoleDeviceEnabled) { Console.Write(message); } // Send it to the streams for (int i=0; i0) Array.Copy(m_rgStream, newArray, m_iNumOfStreamDevices); m_iStreamArraySize += 4; m_rgStream = newArray; } m_rgStream [m_iNumOfStreamDevices++] = stream; } // Generates a log message. If its switch (or a parent switch) allows the // level for the message, it is "broadcast" to all of the log // devices. // public static void LogMessage(LoggingLevels level, String message) { LogMessage (level, GlobalSwitch, message); } // Generates a log message. If its switch (or a parent switch) allows the // level for the message, it is "broadcast" to all of the log // devices. // [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Process, ResourceScope.Process)] public static void LogMessage(LoggingLevels level, LogSwitch logswitch, String message) { if (logswitch == null) throw new ArgumentNullException ("LogSwitch"); if (level < 0) throw new ArgumentOutOfRangeException("level", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegNum")); // Is logging for this level for this switch enabled? if (logswitch.CheckLevel (level) == true) { // Send message for logging // first send it to the debugger Debugger.Log ((int) level, logswitch.strName, message); // Send to the console device if (m_fConsoleDeviceEnabled) { Console.Write(message); } // Send it to the streams for (int i=0; i
Link Menu
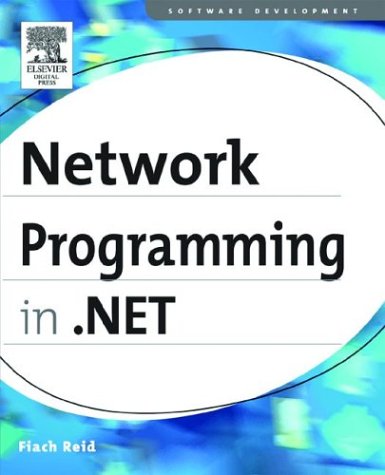
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- KeyboardInputProviderAcquireFocusEventArgs.cs
- MailDefinition.cs
- StaticExtension.cs
- TemplateKey.cs
- SoapSchemaExporter.cs
- AuthenticationModuleElement.cs
- BooleanAnimationBase.cs
- TrailingSpaceComparer.cs
- RefExpr.cs
- XmlObjectSerializerContext.cs
- Rule.cs
- SqlConnectionString.cs
- DataTableNewRowEvent.cs
- RemotingException.cs
- WebMessageEncodingElement.cs
- EventLogSession.cs
- MenuItem.cs
- TypeUtils.cs
- Popup.cs
- AsyncSerializedWorker.cs
- HtmlTableCell.cs
- HScrollProperties.cs
- HostingPreferredMapPath.cs
- CombinedGeometry.cs
- SequenceRange.cs
- SqlProcedureAttribute.cs
- DataGridCellAutomationPeer.cs
- FilterEventArgs.cs
- DispatchWrapper.cs
- DrawingContextDrawingContextWalker.cs
- EventLogger.cs
- SingleAnimationBase.cs
- _SpnDictionary.cs
- BindingMemberInfo.cs
- BuildProvider.cs
- EventMappingSettings.cs
- SqlDataSourceStatusEventArgs.cs
- EntryIndex.cs
- MethodExpr.cs
- HwndSourceKeyboardInputSite.cs
- CodeTypeConstructor.cs
- ClientData.cs
- XmlObjectSerializerContext.cs
- RootNamespaceAttribute.cs
- TreeViewCancelEvent.cs
- DependentList.cs
- PrimaryKeyTypeConverter.cs
- XmlTextWriter.cs
- SpeechUI.cs
- ExtractorMetadata.cs
- WeakRefEnumerator.cs
- DataGridViewDataConnection.cs
- Update.cs
- PointAnimationBase.cs
- QuaternionAnimationBase.cs
- TabItemWrapperAutomationPeer.cs
- XmlObjectSerializerReadContext.cs
- ObjectToIdCache.cs
- RoutedEvent.cs
- ProfileInfo.cs
- Inflater.cs
- BamlMapTable.cs
- TagPrefixInfo.cs
- CustomErrorsSection.cs
- AuditLogLocation.cs
- HeaderElement.cs
- TextTreeRootNode.cs
- SchemaCompiler.cs
- ModifiableIteratorCollection.cs
- HijriCalendar.cs
- EmptyCollection.cs
- WarningException.cs
- WebScriptMetadataFormatter.cs
- PeerTransportElement.cs
- XmlArrayAttribute.cs
- ContentValidator.cs
- connectionpool.cs
- Validator.cs
- NativeMethodsCLR.cs
- DynamicResourceExtensionConverter.cs
- WebScriptMetadataMessage.cs
- DllNotFoundException.cs
- PrintDialog.cs
- Variant.cs
- StreamGeometry.cs
- PersistenceException.cs
- HexParser.cs
- GregorianCalendarHelper.cs
- VScrollBar.cs
- SqlDataSourceCache.cs
- CharEnumerator.cs
- ProtocolsConfiguration.cs
- Drawing.cs
- NameValueFileSectionHandler.cs
- HandledEventArgs.cs
- EntityCommandCompilationException.cs
- ConfigurationConverterBase.cs
- CqlLexerHelpers.cs
- PropertyGeneratedEventArgs.cs
- RequestQueue.cs