Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / Compilation / CompilerTypeWithParams.cs / 1 / CompilerTypeWithParams.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Compilation { using System; using System.Security.Permissions; using System.IO; using System.Collections; using System.Globalization; using System.CodeDom; using System.CodeDom.Compiler; using System.Web.Hosting; using System.Web.Util; using System.Web.UI; using System.Web.Configuration; /* * This class describes a CodeDom compiler, along with the parameters that it uses. * The reason we need this class is that if two files both use the same language, * but ask for different command line options (e.g. debug vs retail), we will not * be able to compile them together. So effectively, we need to treat them as * different languages. */ [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class CompilerType { private Type _codeDomProviderType; public Type CodeDomProviderType { get { return _codeDomProviderType; } } private CompilerParameters _compilParams; public CompilerParameters CompilerParameters { get { return _compilParams; } } internal CompilerType(Type codeDomProviderType, CompilerParameters compilParams) { Debug.Assert(codeDomProviderType != null); _codeDomProviderType = codeDomProviderType; if (compilParams == null) _compilParams = new CompilerParameters(); else _compilParams = compilParams; } internal CompilerType Clone() { // Clone the CompilerParameters to make sure the original is untouched return new CompilerType(_codeDomProviderType, CloneCompilerParameters()); } private CompilerParameters CloneCompilerParameters() { CompilerParameters copy = new CompilerParameters(); copy.IncludeDebugInformation = _compilParams.IncludeDebugInformation; copy.TreatWarningsAsErrors = _compilParams.TreatWarningsAsErrors; copy.WarningLevel = _compilParams.WarningLevel; copy.CompilerOptions = _compilParams.CompilerOptions; return copy; } public override int GetHashCode() { return _codeDomProviderType.GetHashCode(); } public override bool Equals(Object o) { CompilerType other = o as CompilerType; if (o == null) return false; return _codeDomProviderType == other._codeDomProviderType && _compilParams.WarningLevel == other._compilParams.WarningLevel && _compilParams.IncludeDebugInformation == other._compilParams.IncludeDebugInformation && _compilParams.CompilerOptions == other._compilParams.CompilerOptions; } internal AssemblyBuilder CreateAssemblyBuilder(CompilationSection compConfig, ICollection referencedAssemblies) { return CreateAssemblyBuilder(compConfig, referencedAssemblies, null /*generatedFilesDir*/, null /*outputAssemblyName*/); } internal AssemblyBuilder CreateAssemblyBuilder(CompilationSection compConfig, ICollection referencedAssemblies, string generatedFilesDir, string outputAssemblyName) { // Create a special AssemblyBuilder when we're only supposed to generate // source files but not compile them (for ClientBuildManager.GetCodeDirectoryInformation) if (generatedFilesDir != null) { return new CbmCodeGeneratorBuildProviderHost(compConfig, referencedAssemblies, this, generatedFilesDir, outputAssemblyName); } return new AssemblyBuilder(compConfig, referencedAssemblies, this, outputAssemblyName); } private static CompilerType GetDefaultCompilerTypeWithParams( CompilationSection compConfig, VirtualPath configPath) { // By default, use C# when no provider is asking for a specific language return CompilationUtil.GetCSharpCompilerInfo(compConfig, configPath); } internal static AssemblyBuilder GetDefaultAssemblyBuilder(CompilationSection compConfig, ICollection referencedAssemblies, VirtualPath configPath, string outputAssemblyName) { return GetDefaultAssemblyBuilder(compConfig, referencedAssemblies, configPath, null /*generatedFilesDir*/, outputAssemblyName); } internal static AssemblyBuilder GetDefaultAssemblyBuilder(CompilationSection compConfig, ICollection referencedAssemblies, VirtualPath configPath, string generatedFilesDir, string outputAssemblyName) { CompilerType ctwp = GetDefaultCompilerTypeWithParams(compConfig, configPath); return ctwp.CreateAssemblyBuilder(compConfig, referencedAssemblies, generatedFilesDir, outputAssemblyName); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Compilation { using System; using System.Security.Permissions; using System.IO; using System.Collections; using System.Globalization; using System.CodeDom; using System.CodeDom.Compiler; using System.Web.Hosting; using System.Web.Util; using System.Web.UI; using System.Web.Configuration; /* * This class describes a CodeDom compiler, along with the parameters that it uses. * The reason we need this class is that if two files both use the same language, * but ask for different command line options (e.g. debug vs retail), we will not * be able to compile them together. So effectively, we need to treat them as * different languages. */ [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class CompilerType { private Type _codeDomProviderType; public Type CodeDomProviderType { get { return _codeDomProviderType; } } private CompilerParameters _compilParams; public CompilerParameters CompilerParameters { get { return _compilParams; } } internal CompilerType(Type codeDomProviderType, CompilerParameters compilParams) { Debug.Assert(codeDomProviderType != null); _codeDomProviderType = codeDomProviderType; if (compilParams == null) _compilParams = new CompilerParameters(); else _compilParams = compilParams; } internal CompilerType Clone() { // Clone the CompilerParameters to make sure the original is untouched return new CompilerType(_codeDomProviderType, CloneCompilerParameters()); } private CompilerParameters CloneCompilerParameters() { CompilerParameters copy = new CompilerParameters(); copy.IncludeDebugInformation = _compilParams.IncludeDebugInformation; copy.TreatWarningsAsErrors = _compilParams.TreatWarningsAsErrors; copy.WarningLevel = _compilParams.WarningLevel; copy.CompilerOptions = _compilParams.CompilerOptions; return copy; } public override int GetHashCode() { return _codeDomProviderType.GetHashCode(); } public override bool Equals(Object o) { CompilerType other = o as CompilerType; if (o == null) return false; return _codeDomProviderType == other._codeDomProviderType && _compilParams.WarningLevel == other._compilParams.WarningLevel && _compilParams.IncludeDebugInformation == other._compilParams.IncludeDebugInformation && _compilParams.CompilerOptions == other._compilParams.CompilerOptions; } internal AssemblyBuilder CreateAssemblyBuilder(CompilationSection compConfig, ICollection referencedAssemblies) { return CreateAssemblyBuilder(compConfig, referencedAssemblies, null /*generatedFilesDir*/, null /*outputAssemblyName*/); } internal AssemblyBuilder CreateAssemblyBuilder(CompilationSection compConfig, ICollection referencedAssemblies, string generatedFilesDir, string outputAssemblyName) { // Create a special AssemblyBuilder when we're only supposed to generate // source files but not compile them (for ClientBuildManager.GetCodeDirectoryInformation) if (generatedFilesDir != null) { return new CbmCodeGeneratorBuildProviderHost(compConfig, referencedAssemblies, this, generatedFilesDir, outputAssemblyName); } return new AssemblyBuilder(compConfig, referencedAssemblies, this, outputAssemblyName); } private static CompilerType GetDefaultCompilerTypeWithParams( CompilationSection compConfig, VirtualPath configPath) { // By default, use C# when no provider is asking for a specific language return CompilationUtil.GetCSharpCompilerInfo(compConfig, configPath); } internal static AssemblyBuilder GetDefaultAssemblyBuilder(CompilationSection compConfig, ICollection referencedAssemblies, VirtualPath configPath, string outputAssemblyName) { return GetDefaultAssemblyBuilder(compConfig, referencedAssemblies, configPath, null /*generatedFilesDir*/, outputAssemblyName); } internal static AssemblyBuilder GetDefaultAssemblyBuilder(CompilationSection compConfig, ICollection referencedAssemblies, VirtualPath configPath, string generatedFilesDir, string outputAssemblyName) { CompilerType ctwp = GetDefaultCompilerTypeWithParams(compConfig, configPath); return ctwp.CreateAssemblyBuilder(compConfig, referencedAssemblies, generatedFilesDir, outputAssemblyName); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
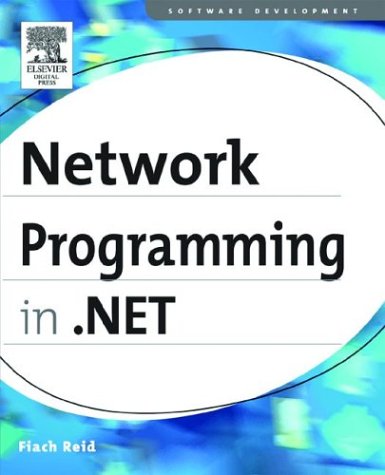
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- QuotedPrintableStream.cs
- CounterCreationData.cs
- RequestUriProcessor.cs
- SmtpDigestAuthenticationModule.cs
- BindingOperations.cs
- FileAuthorizationModule.cs
- RichTextBox.cs
- OperatorExpressions.cs
- CodeSnippetCompileUnit.cs
- CompareValidator.cs
- SubqueryRules.cs
- TablePattern.cs
- NativeActivityTransactionContext.cs
- XmlCharCheckingWriter.cs
- TextFragmentEngine.cs
- regiisutil.cs
- XmlLangPropertyAttribute.cs
- TextEditorLists.cs
- DeclarativeConditionsCollection.cs
- NotifyInputEventArgs.cs
- EmptyReadOnlyDictionaryInternal.cs
- ComboBoxRenderer.cs
- ErrorWebPart.cs
- TextCharacters.cs
- DataGridViewCheckBoxCell.cs
- OdbcUtils.cs
- HierarchicalDataSourceControl.cs
- StrongTypingException.cs
- ConfigurationPropertyAttribute.cs
- TargetControlTypeAttribute.cs
- BindingCompleteEventArgs.cs
- OdbcErrorCollection.cs
- AsyncStreamReader.cs
- Highlights.cs
- CodeTypeParameterCollection.cs
- XmlCharType.cs
- ApplicationGesture.cs
- SqlSupersetValidator.cs
- NavigatorOutput.cs
- DataControlLinkButton.cs
- RadialGradientBrush.cs
- WinEventHandler.cs
- Misc.cs
- CookieParameter.cs
- BufferedReadStream.cs
- MouseEventArgs.cs
- FunctionParameter.cs
- WorkflowValidationFailedException.cs
- IdentityReference.cs
- future.cs
- DataView.cs
- WizardForm.cs
- MarkupWriter.cs
- DesignTimeData.cs
- SharedStatics.cs
- FamilyMap.cs
- ServiceRoute.cs
- XPathDocumentIterator.cs
- FrameworkElementFactoryMarkupObject.cs
- TypeGeneratedEventArgs.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- ConfigXmlCDataSection.cs
- TextEditorSpelling.cs
- NetSectionGroup.cs
- HttpNamespaceReservationInstallComponent.cs
- HtmlInputCheckBox.cs
- WsatServiceAddress.cs
- RepeatBehavior.cs
- AuthenticationSection.cs
- XmlNode.cs
- IgnoreDeviceFilterElementCollection.cs
- ProxyElement.cs
- RNGCryptoServiceProvider.cs
- SQLBinary.cs
- StylusButton.cs
- CodeGenerator.cs
- DataKeyArray.cs
- Condition.cs
- RuntimeUtils.cs
- SiteMembershipCondition.cs
- IxmlLineInfo.cs
- ExpressionVisitorHelpers.cs
- HWStack.cs
- InternalConfigEventArgs.cs
- XmlQualifiedNameTest.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- PermissionListSet.cs
- DuplexChannel.cs
- followingquery.cs
- ServiceNotStartedException.cs
- PolicyStatement.cs
- WpfPayload.cs
- PathFigureCollection.cs
- GlobalAllocSafeHandle.cs
- FromRequest.cs
- Configuration.cs
- WebHttpElement.cs
- HttpHeaderCollection.cs
- DataGridViewComboBoxColumnDesigner.cs
- RoutedEventConverter.cs