Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / Serialization / System / Runtime / Serialization / xmlformatgeneratorstatics.cs / 2 / xmlformatgeneratorstatics.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Runtime.Serialization { using System; using System.Reflection; using System.Security; using System.Xml; using System.Collections; using System.Collections.Generic; ////// Critical - Class holds static instances used for code generation during serialization. /// Static fields are marked SecurityCritical or readonly to prevent /// data from being modified or leaked to other components in appdomain. /// Safe - All get-only properties marked safe since they only need to be protected for write. /// internal static class XmlFormatGeneratorStatics { [SecurityCritical] static MethodInfo writeStartElementMethod2; internal static MethodInfo WriteStartElementMethod2 { [SecurityCritical, SecurityTreatAsSafe] get { if (writeStartElementMethod2 == null) writeStartElementMethod2 = typeof(XmlWriterDelegator).GetMethod("WriteStartElement", Globals.ScanAllMembers, null, new Type[] { typeof(XmlDictionaryString), typeof(XmlDictionaryString) }, null); return writeStartElementMethod2; } } [SecurityCritical] static MethodInfo writeStartElementMethod3; internal static MethodInfo WriteStartElementMethod3 { [SecurityCritical, SecurityTreatAsSafe] get { if (writeStartElementMethod3 == null) writeStartElementMethod3 = typeof(XmlWriterDelegator).GetMethod("WriteStartElement", Globals.ScanAllMembers, null, new Type[] { typeof(string), typeof(XmlDictionaryString), typeof(XmlDictionaryString) }, null); return writeStartElementMethod3; } } [SecurityCritical] static MethodInfo writeEndElementMethod; internal static MethodInfo WriteEndElementMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (writeEndElementMethod == null) writeEndElementMethod = typeof(XmlWriterDelegator).GetMethod("WriteEndElement", Globals.ScanAllMembers, null, new Type[] { }, null); return writeEndElementMethod; } } [SecurityCritical] static MethodInfo writeNamespaceDeclMethod; internal static MethodInfo WriteNamespaceDeclMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (writeNamespaceDeclMethod == null) writeNamespaceDeclMethod = typeof(XmlWriterDelegator).GetMethod("WriteNamespaceDecl", Globals.ScanAllMembers, null, new Type[] { typeof(XmlDictionaryString) }, null); return writeNamespaceDeclMethod; } } [SecurityCritical] static PropertyInfo extensionDataProperty; internal static PropertyInfo ExtensionDataProperty { [SecurityCritical, SecurityTreatAsSafe] get { if (extensionDataProperty == null) extensionDataProperty = typeof(IExtensibleDataObject).GetProperty("ExtensionData"); return extensionDataProperty; } } [SecurityCritical] static MethodInfo boxPointer; internal static MethodInfo BoxPointer { [SecurityCritical, SecurityTreatAsSafe] get { if (boxPointer == null) boxPointer = typeof(Pointer).GetMethod("Box"); return boxPointer; } } [SecurityCritical] static ConstructorInfo dictionaryEnumeratorCtor; internal static ConstructorInfo DictionaryEnumeratorCtor { [SecurityCritical, SecurityTreatAsSafe] get { if (dictionaryEnumeratorCtor == null) dictionaryEnumeratorCtor = Globals.TypeOfDictionaryEnumerator.GetConstructor(Globals.ScanAllMembers, null, new Type[] { Globals.TypeOfIDictionaryEnumerator }, null); return dictionaryEnumeratorCtor; } } [SecurityCritical] static MethodInfo ienumeratorMoveNextMethod; internal static MethodInfo MoveNextMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (ienumeratorMoveNextMethod == null) ienumeratorMoveNextMethod = typeof(IEnumerator).GetMethod("MoveNext"); return ienumeratorMoveNextMethod; } } [SecurityCritical] static MethodInfo ienumeratorGetCurrentMethod; internal static MethodInfo GetCurrentMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (ienumeratorGetCurrentMethod == null) ienumeratorGetCurrentMethod = typeof(IEnumerator).GetProperty("Current").GetGetMethod(); return ienumeratorGetCurrentMethod; } } [SecurityCritical] static MethodInfo getItemContractMethod; internal static MethodInfo GetItemContractMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (getItemContractMethod == null) getItemContractMethod = typeof(CollectionDataContract).GetProperty("ItemContract", Globals.ScanAllMembers).GetGetMethod(true/*nonPublic*/); return getItemContractMethod; } } [SecurityCritical] static MethodInfo isStartElementMethod2; internal static MethodInfo IsStartElementMethod2 { [SecurityCritical, SecurityTreatAsSafe] get { if (isStartElementMethod2 == null) isStartElementMethod2 = typeof(XmlReaderDelegator).GetMethod("IsStartElement", Globals.ScanAllMembers, null, new Type[] { typeof(XmlDictionaryString), typeof(XmlDictionaryString) }, null); return isStartElementMethod2; } } [SecurityCritical] static MethodInfo isStartElementMethod0; internal static MethodInfo IsStartElementMethod0 { [SecurityCritical, SecurityTreatAsSafe] get { if (isStartElementMethod0 == null) isStartElementMethod0 = typeof(XmlReaderDelegator).GetMethod("IsStartElement", Globals.ScanAllMembers, null, new Type[] { }, null); return isStartElementMethod0; } } [SecurityCritical] static MethodInfo getUninitializedObjectMethod; internal static MethodInfo GetUninitializedObjectMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (getUninitializedObjectMethod == null) getUninitializedObjectMethod = typeof(XmlFormatReaderGenerator).GetMethod("UnsafeGetUninitializedObject", Globals.ScanAllMembers, null, new Type[] { typeof(int) }, null); return getUninitializedObjectMethod; } } [SecurityCritical] static MethodInfo onDeserializationMethod; internal static MethodInfo OnDeserializationMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (onDeserializationMethod == null) onDeserializationMethod = typeof(IDeserializationCallback).GetMethod("OnDeserialization"); return onDeserializationMethod; } } [SecurityCritical] static MethodInfo unboxPointer; internal static MethodInfo UnboxPointer { [SecurityCritical, SecurityTreatAsSafe] get { if (unboxPointer == null) unboxPointer = typeof(Pointer).GetMethod("Unbox"); return unboxPointer; } } [SecurityCritical] static PropertyInfo nodeTypeProperty; internal static PropertyInfo NodeTypeProperty { [SecurityCritical, SecurityTreatAsSafe] get { if (nodeTypeProperty == null) nodeTypeProperty = typeof(XmlReaderDelegator).GetProperty("NodeType", Globals.ScanAllMembers); return nodeTypeProperty; } } [SecurityCritical] static ConstructorInfo serializationExceptionCtor; internal static ConstructorInfo SerializationExceptionCtor { [SecurityCritical, SecurityTreatAsSafe] get { if (serializationExceptionCtor == null) serializationExceptionCtor = typeof(SerializationException).GetConstructor(new Type[] { typeof(string) }); return serializationExceptionCtor; } } [SecurityCritical] static ConstructorInfo extensionDataObjectCtor; internal static ConstructorInfo ExtensionDataObjectCtor { [SecurityCritical, SecurityTreatAsSafe] get { if (extensionDataObjectCtor == null) extensionDataObjectCtor = typeof(ExtensionDataObject).GetConstructor(Globals.ScanAllMembers, null, new Type[] { }, null); return extensionDataObjectCtor; } } [SecurityCritical] static ConstructorInfo hashtableCtor; internal static ConstructorInfo HashtableCtor { [SecurityCritical, SecurityTreatAsSafe] get { if (hashtableCtor == null) hashtableCtor = Globals.TypeOfHashtable.GetConstructor(Globals.ScanAllMembers, null, Globals.EmptyTypeArray, null); return hashtableCtor; } } [SecurityCritical] static MethodInfo getStreamingContextMethod; internal static MethodInfo GetStreamingContextMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (getStreamingContextMethod == null) getStreamingContextMethod = typeof(XmlObjectSerializerContext).GetMethod("GetStreamingContext", Globals.ScanAllMembers); return getStreamingContextMethod; } } [SecurityCritical] static MethodInfo getCollectionMemberMethod; internal static MethodInfo GetCollectionMemberMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (getCollectionMemberMethod == null) getCollectionMemberMethod = typeof(XmlObjectSerializerReadContext).GetMethod("GetCollectionMember", Globals.ScanAllMembers); return getCollectionMemberMethod; } } [SecurityCritical] static MethodInfo storeCollectionMemberInfoMethod; internal static MethodInfo StoreCollectionMemberInfoMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (storeCollectionMemberInfoMethod == null) storeCollectionMemberInfoMethod = typeof(XmlObjectSerializerReadContext).GetMethod("StoreCollectionMemberInfo", Globals.ScanAllMembers, null, new Type[] { typeof(object) }, null); return storeCollectionMemberInfoMethod; } } [SecurityCritical] static MethodInfo storeIsGetOnlyCollectionMethod; internal static MethodInfo StoreIsGetOnlyCollectionMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (storeIsGetOnlyCollectionMethod == null) storeIsGetOnlyCollectionMethod = typeof(XmlObjectSerializerWriteContext).GetMethod("StoreIsGetOnlyCollection", Globals.ScanAllMembers); return storeIsGetOnlyCollectionMethod; } } [SecurityCritical] static MethodInfo throwNullValueReturnedForGetOnlyCollectionExceptionMethod; internal static MethodInfo ThrowNullValueReturnedForGetOnlyCollectionExceptionMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (throwNullValueReturnedForGetOnlyCollectionExceptionMethod == null) throwNullValueReturnedForGetOnlyCollectionExceptionMethod = typeof(XmlObjectSerializerReadContext).GetMethod("ThrowNullValueReturnedForGetOnlyCollectionException", Globals.ScanAllMembers); return throwNullValueReturnedForGetOnlyCollectionExceptionMethod; } } static MethodInfo throwArrayExceededSizeExceptionMethod; internal static MethodInfo ThrowArrayExceededSizeExceptionMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (throwArrayExceededSizeExceptionMethod == null) throwArrayExceededSizeExceptionMethod = typeof(XmlObjectSerializerReadContext).GetMethod("ThrowArrayExceededSizeException", Globals.ScanAllMembers); return throwArrayExceededSizeExceptionMethod; } } [SecurityCritical] static MethodInfo incrementItemCountMethod; internal static MethodInfo IncrementItemCountMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (incrementItemCountMethod == null) incrementItemCountMethod = typeof(XmlObjectSerializerContext).GetMethod("IncrementItemCount", Globals.ScanAllMembers); return incrementItemCountMethod; } } ////// Critical - holds instance of SecurityPermission that we will Demand for SerializationFormatter /// should not be modified to something else /// [SecurityCritical] static MethodInfo demandSerializationFormatterPermissionMethod; internal static MethodInfo DemandSerializationFormatterPermissionMethod { ////// Critical - Demands SerializationFormatter permission. Demanding the right permission is critical /// Safe - no data or control leaks in or out, must be callable from transparent generated IL /// [SecurityCritical, SecurityTreatAsSafe] get { if (demandSerializationFormatterPermissionMethod == null) demandSerializationFormatterPermissionMethod = typeof(XmlObjectSerializerContext).GetMethod("DemandSerializationFormatterPermission", Globals.ScanAllMembers); return demandSerializationFormatterPermissionMethod; } } ////// Critical - holds instance of SecurityPermission that we will Demand for MemberAccess /// should not be modified to something else /// [SecurityCritical] static MethodInfo demandMemberAccessPermissionMethod; internal static MethodInfo DemandMemberAccessPermissionMethod { ////// Critical - Demands MemberAccess permission. Demanding the right permission is critical /// Safe - no data or control leaks in or out, must be callable from transparent generated IL /// [SecurityCritical, SecurityTreatAsSafe] get { if (demandMemberAccessPermissionMethod == null) demandMemberAccessPermissionMethod = typeof(XmlObjectSerializerContext).GetMethod("DemandMemberAccessPermission", Globals.ScanAllMembers); return demandMemberAccessPermissionMethod; } } [SecurityCritical] static MethodInfo internalDeserializeMethod; internal static MethodInfo InternalDeserializeMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (internalDeserializeMethod == null) internalDeserializeMethod = typeof(XmlObjectSerializerReadContext).GetMethod("InternalDeserialize", Globals.ScanAllMembers, null, new Type[] { typeof(XmlReaderDelegator), typeof(int), typeof(RuntimeTypeHandle), typeof(string), typeof(string) }, null); return internalDeserializeMethod; } } [SecurityCritical] static MethodInfo moveToNextElementMethod; internal static MethodInfo MoveToNextElementMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (moveToNextElementMethod == null) moveToNextElementMethod = typeof(XmlObjectSerializerReadContext).GetMethod("MoveToNextElement", Globals.ScanAllMembers); return moveToNextElementMethod; } } [SecurityCritical] static MethodInfo getMemberIndexMethod; internal static MethodInfo GetMemberIndexMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (getMemberIndexMethod == null) getMemberIndexMethod = typeof(XmlObjectSerializerReadContext).GetMethod("GetMemberIndex", Globals.ScanAllMembers); return getMemberIndexMethod; } } [SecurityCritical] static MethodInfo getMemberIndexWithRequiredMembersMethod; internal static MethodInfo GetMemberIndexWithRequiredMembersMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (getMemberIndexWithRequiredMembersMethod == null) getMemberIndexWithRequiredMembersMethod = typeof(XmlObjectSerializerReadContext).GetMethod("GetMemberIndexWithRequiredMembers", Globals.ScanAllMembers); return getMemberIndexWithRequiredMembersMethod; } } [SecurityCritical] static MethodInfo throwRequiredMemberMissingExceptionMethod; internal static MethodInfo ThrowRequiredMemberMissingExceptionMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (throwRequiredMemberMissingExceptionMethod == null) throwRequiredMemberMissingExceptionMethod = typeof(XmlObjectSerializerReadContext).GetMethod("ThrowRequiredMemberMissingException", Globals.ScanAllMembers); return throwRequiredMemberMissingExceptionMethod; } } [SecurityCritical] static MethodInfo skipUnknownElementMethod; internal static MethodInfo SkipUnknownElementMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (skipUnknownElementMethod == null) skipUnknownElementMethod = typeof(XmlObjectSerializerReadContext).GetMethod("SkipUnknownElement", Globals.ScanAllMembers); return skipUnknownElementMethod; } } [SecurityCritical] static MethodInfo readIfNullOrRefMethod; internal static MethodInfo ReadIfNullOrRefMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (readIfNullOrRefMethod == null) readIfNullOrRefMethod = typeof(XmlObjectSerializerReadContext).GetMethod("ReadIfNullOrRef", Globals.ScanAllMembers, null, new Type[] { typeof(XmlReaderDelegator), typeof(Type), typeof(bool) }, null); return readIfNullOrRefMethod; } } [SecurityCritical] static MethodInfo readAttributesMethod; internal static MethodInfo ReadAttributesMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (readAttributesMethod == null) readAttributesMethod = typeof(XmlObjectSerializerReadContext).GetMethod("ReadAttributes", Globals.ScanAllMembers); return readAttributesMethod; } } [SecurityCritical] static MethodInfo resetAttributesMethod; internal static MethodInfo ResetAttributesMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (resetAttributesMethod == null) resetAttributesMethod = typeof(XmlObjectSerializerReadContext).GetMethod("ResetAttributes", Globals.ScanAllMembers); return resetAttributesMethod; } } [SecurityCritical] static MethodInfo getObjectIdMethod; internal static MethodInfo GetObjectIdMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (getObjectIdMethod == null) getObjectIdMethod = typeof(XmlObjectSerializerReadContext).GetMethod("GetObjectId", Globals.ScanAllMembers); return getObjectIdMethod; } } [SecurityCritical] static MethodInfo getArraySizeMethod; internal static MethodInfo GetArraySizeMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (getArraySizeMethod == null) getArraySizeMethod = typeof(XmlObjectSerializerReadContext).GetMethod("GetArraySize", Globals.ScanAllMembers); return getArraySizeMethod; } } [SecurityCritical] static MethodInfo addNewObjectMethod; internal static MethodInfo AddNewObjectMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (addNewObjectMethod == null) addNewObjectMethod = typeof(XmlObjectSerializerReadContext).GetMethod("AddNewObject", Globals.ScanAllMembers); return addNewObjectMethod; } } [SecurityCritical] static MethodInfo addNewObjectWithIdMethod; internal static MethodInfo AddNewObjectWithIdMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (addNewObjectWithIdMethod == null) addNewObjectWithIdMethod = typeof(XmlObjectSerializerReadContext).GetMethod("AddNewObjectWithId", Globals.ScanAllMembers); return addNewObjectWithIdMethod; } } [SecurityCritical] static MethodInfo replaceDeserializedObjectMethod; internal static MethodInfo ReplaceDeserializedObjectMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (replaceDeserializedObjectMethod == null) replaceDeserializedObjectMethod = typeof(XmlObjectSerializerReadContext).GetMethod("ReplaceDeserializedObject", Globals.ScanAllMembers); return replaceDeserializedObjectMethod; } } [SecurityCritical] static MethodInfo getExistingObjectMethod; internal static MethodInfo GetExistingObjectMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (getExistingObjectMethod == null) getExistingObjectMethod = typeof(XmlObjectSerializerReadContext).GetMethod("GetExistingObject", Globals.ScanAllMembers); return getExistingObjectMethod; } } [SecurityCritical] static MethodInfo getRealObjectMethod; internal static MethodInfo GetRealObjectMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (getRealObjectMethod == null) getRealObjectMethod = typeof(XmlObjectSerializerReadContext).GetMethod("GetRealObject", Globals.ScanAllMembers); return getRealObjectMethod; } } [SecurityCritical] static MethodInfo readMethod; internal static MethodInfo ReadMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (readMethod == null) readMethod = typeof(XmlObjectSerializerReadContext).GetMethod("Read", Globals.ScanAllMembers); return readMethod; } } [SecurityCritical] static MethodInfo ensureArraySizeMethod; internal static MethodInfo EnsureArraySizeMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (ensureArraySizeMethod == null) ensureArraySizeMethod = typeof(XmlObjectSerializerReadContext).GetMethod("EnsureArraySize", Globals.ScanAllMembers); return ensureArraySizeMethod; } } [SecurityCritical] static MethodInfo trimArraySizeMethod; internal static MethodInfo TrimArraySizeMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (trimArraySizeMethod == null) trimArraySizeMethod = typeof(XmlObjectSerializerReadContext).GetMethod("TrimArraySize", Globals.ScanAllMembers); return trimArraySizeMethod; } } [SecurityCritical] static MethodInfo checkEndOfArrayMethod; internal static MethodInfo CheckEndOfArrayMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (checkEndOfArrayMethod == null) checkEndOfArrayMethod = typeof(XmlObjectSerializerReadContext).GetMethod("CheckEndOfArray", Globals.ScanAllMembers); return checkEndOfArrayMethod; } } [SecurityCritical] static MethodInfo getArrayLengthMethod; internal static MethodInfo GetArrayLengthMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (getArrayLengthMethod == null) getArrayLengthMethod = Globals.TypeOfArray.GetProperty("Length").GetGetMethod(); return getArrayLengthMethod; } } [SecurityCritical] static MethodInfo readSerializationInfoMethod; internal static MethodInfo ReadSerializationInfoMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (readSerializationInfoMethod == null) readSerializationInfoMethod = typeof(XmlObjectSerializerReadContext).GetMethod("ReadSerializationInfo", Globals.ScanAllMembers); return readSerializationInfoMethod; } } [SecurityCritical] static MethodInfo createUnexpectedStateExceptionMethod; internal static MethodInfo CreateUnexpectedStateExceptionMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (createUnexpectedStateExceptionMethod == null) createUnexpectedStateExceptionMethod = typeof(XmlObjectSerializerReadContext).GetMethod("CreateUnexpectedStateException", Globals.ScanAllMembers, null, new Type[] { typeof(XmlNodeType), typeof(XmlReaderDelegator) }, null); return createUnexpectedStateExceptionMethod; } } [SecurityCritical] static MethodInfo internalSerializeReferenceMethod; internal static MethodInfo InternalSerializeReferenceMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (internalSerializeReferenceMethod == null) internalSerializeReferenceMethod = typeof(XmlObjectSerializerWriteContext).GetMethod("InternalSerializeReference", Globals.ScanAllMembers); return internalSerializeReferenceMethod; } } [SecurityCritical] static MethodInfo internalSerializeMethod; internal static MethodInfo InternalSerializeMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (internalSerializeMethod == null) internalSerializeMethod = typeof(XmlObjectSerializerWriteContext).GetMethod("InternalSerialize", Globals.ScanAllMembers); return internalSerializeMethod; } } [SecurityCritical] static MethodInfo writeNullMethod; internal static MethodInfo WriteNullMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (writeNullMethod == null) writeNullMethod = typeof(XmlObjectSerializerWriteContext).GetMethod("WriteNull", Globals.ScanAllMembers, null, new Type[] { typeof(XmlWriterDelegator), typeof(Type), typeof(bool) }, null); return writeNullMethod; } } [SecurityCritical] static MethodInfo incrementArrayCountMethod; internal static MethodInfo IncrementArrayCountMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (incrementArrayCountMethod == null) incrementArrayCountMethod = typeof(XmlObjectSerializerWriteContext).GetMethod("IncrementArrayCount", Globals.ScanAllMembers); return incrementArrayCountMethod; } } [SecurityCritical] static MethodInfo incrementCollectionCountMethod; internal static MethodInfo IncrementCollectionCountMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (incrementCollectionCountMethod == null) incrementCollectionCountMethod = typeof(XmlObjectSerializerWriteContext).GetMethod("IncrementCollectionCount", Globals.ScanAllMembers, null, new Type[] { typeof(XmlWriterDelegator), typeof(ICollection) }, null); return incrementCollectionCountMethod; } } [SecurityCritical] static MethodInfo incrementCollectionCountGenericMethod; internal static MethodInfo IncrementCollectionCountGenericMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (incrementCollectionCountGenericMethod == null) incrementCollectionCountGenericMethod = typeof(XmlObjectSerializerWriteContext).GetMethod("IncrementCollectionCountGeneric", Globals.ScanAllMembers); return incrementCollectionCountGenericMethod; } } [SecurityCritical] static MethodInfo getDefaultValueMethod; internal static MethodInfo GetDefaultValueMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (getDefaultValueMethod == null) getDefaultValueMethod = typeof(XmlObjectSerializerWriteContext).GetMethod("GetDefaultValue", Globals.ScanAllMembers); return getDefaultValueMethod; } } [SecurityCritical] static MethodInfo getNullableValueMethod; internal static MethodInfo GetNullableValueMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (getNullableValueMethod == null) getNullableValueMethod = typeof(XmlObjectSerializerWriteContext).GetMethod("GetNullableValue", Globals.ScanAllMembers); return getNullableValueMethod; } } [SecurityCritical] static MethodInfo throwRequiredMemberMustBeEmittedMethod; internal static MethodInfo ThrowRequiredMemberMustBeEmittedMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (throwRequiredMemberMustBeEmittedMethod == null) throwRequiredMemberMustBeEmittedMethod = typeof(XmlObjectSerializerWriteContext).GetMethod("ThrowRequiredMemberMustBeEmitted", Globals.ScanAllMembers); return throwRequiredMemberMustBeEmittedMethod; } } [SecurityCritical] static MethodInfo getHasValueMethod; internal static MethodInfo GetHasValueMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (getHasValueMethod == null) getHasValueMethod = typeof(XmlObjectSerializerWriteContext).GetMethod("GetHasValue", Globals.ScanAllMembers); return getHasValueMethod; } } [SecurityCritical] static MethodInfo writeISerializableMethod; internal static MethodInfo WriteISerializableMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (writeISerializableMethod == null) writeISerializableMethod = typeof(XmlObjectSerializerWriteContext).GetMethod("WriteISerializable", Globals.ScanAllMembers); return writeISerializableMethod; } } [SecurityCritical] static MethodInfo writeExtensionDataMethod; internal static MethodInfo WriteExtensionDataMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (writeExtensionDataMethod == null) writeExtensionDataMethod = typeof(XmlObjectSerializerWriteContext).GetMethod("WriteExtensionData", Globals.ScanAllMembers); return writeExtensionDataMethod; } } [SecurityCritical] static MethodInfo writeXmlValueMethod; internal static MethodInfo WriteXmlValueMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (writeXmlValueMethod == null) writeXmlValueMethod = typeof(DataContract).GetMethod("WriteXmlValue", Globals.ScanAllMembers); return writeXmlValueMethod; } } [SecurityCritical] static MethodInfo readXmlValueMethod; internal static MethodInfo ReadXmlValueMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (readXmlValueMethod == null) readXmlValueMethod = typeof(DataContract).GetMethod("ReadXmlValue", Globals.ScanAllMembers); return readXmlValueMethod; } } [SecurityCritical] static MethodInfo throwTypeNotSerializableMethod; internal static MethodInfo ThrowTypeNotSerializableMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (throwTypeNotSerializableMethod == null) throwTypeNotSerializableMethod = typeof(DataContract).GetMethod("ThrowTypeNotSerializable", Globals.ScanAllMembers); return throwTypeNotSerializableMethod; } } [SecurityCritical] static PropertyInfo namespaceProperty; internal static PropertyInfo NamespaceProperty { [SecurityCritical, SecurityTreatAsSafe] get { if (namespaceProperty == null) namespaceProperty = typeof(DataContract).GetProperty("Namespace", Globals.ScanAllMembers); return namespaceProperty; } } [SecurityCritical] static FieldInfo contractNamespacesField; internal static FieldInfo ContractNamespacesField { [SecurityCritical, SecurityTreatAsSafe] get { if (contractNamespacesField == null) contractNamespacesField = typeof(ClassDataContract).GetField("ContractNamespaces", Globals.ScanAllMembers); return contractNamespacesField; } } [SecurityCritical] static FieldInfo memberNamesField; internal static FieldInfo MemberNamesField { [SecurityCritical, SecurityTreatAsSafe] get { if (memberNamesField == null) memberNamesField = typeof(ClassDataContract).GetField("MemberNames", Globals.ScanAllMembers); return memberNamesField; } } [SecurityCritical] static MethodInfo extensionDataSetExplicitMethodInfo; internal static MethodInfo ExtensionDataSetExplicitMethodInfo { [SecurityCritical, SecurityTreatAsSafe] get { if (extensionDataSetExplicitMethodInfo == null) extensionDataSetExplicitMethodInfo = typeof(IExtensibleDataObject).GetMethod(Globals.ExtensionDataSetMethod); return extensionDataSetExplicitMethodInfo; } } [SecurityCritical] static PropertyInfo childElementNamespacesProperty; internal static PropertyInfo ChildElementNamespacesProperty { [SecurityCritical, SecurityTreatAsSafe] get { if (childElementNamespacesProperty == null) childElementNamespacesProperty = typeof(ClassDataContract).GetProperty("ChildElementNamespaces", Globals.ScanAllMembers); return childElementNamespacesProperty; } } [SecurityCritical] static PropertyInfo collectionItemNameProperty; internal static PropertyInfo CollectionItemNameProperty { [SecurityCritical, SecurityTreatAsSafe] get { if (collectionItemNameProperty == null) collectionItemNameProperty = typeof(CollectionDataContract).GetProperty("CollectionItemName", Globals.ScanAllMembers); return collectionItemNameProperty; } } [SecurityCritical] static PropertyInfo childElementNamespaceProperty; internal static PropertyInfo ChildElementNamespaceProperty { [SecurityCritical, SecurityTreatAsSafe] get { if (childElementNamespaceProperty == null) childElementNamespaceProperty = typeof(CollectionDataContract).GetProperty("ChildElementNamespace", Globals.ScanAllMembers); return childElementNamespaceProperty; } } [SecurityCritical] static MethodInfo getDateTimeOffsetMethod; internal static MethodInfo GetDateTimeOffsetMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (getDateTimeOffsetMethod == null) getDateTimeOffsetMethod = typeof(DateTimeOffsetAdapter).GetMethod("GetDateTimeOffset", Globals.ScanAllMembers); return getDateTimeOffsetMethod; } } [SecurityCritical] static MethodInfo getDateTimeOffsetAdapterMethod; internal static MethodInfo GetDateTimeOffsetAdapterMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (getDateTimeOffsetAdapterMethod == null) getDateTimeOffsetAdapterMethod = typeof(DateTimeOffsetAdapter).GetMethod("GetDateTimeOffsetAdapter", Globals.ScanAllMembers); return getDateTimeOffsetAdapterMethod; } } [SecurityCritical] static MethodInfo traceInstructionMethod; internal static MethodInfo TraceInstructionMethod { [SecurityCritical, SecurityTreatAsSafe] get { if (traceInstructionMethod == null) traceInstructionMethod = typeof(SerializationTrace).GetMethod("TraceInstruction", Globals.ScanAllMembers); return traceInstructionMethod; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
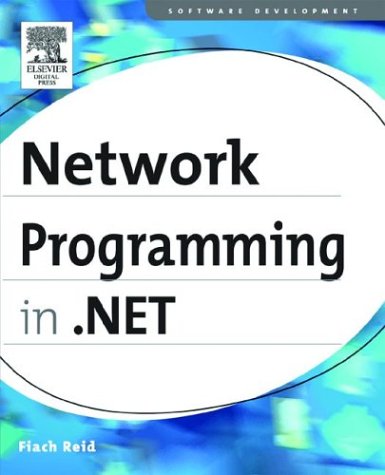
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IndexerNameAttribute.cs
- NetDataContractSerializer.cs
- Encoding.cs
- ExpressionEditorAttribute.cs
- PathSegment.cs
- SmtpNtlmAuthenticationModule.cs
- BrowserCapabilitiesCodeGenerator.cs
- DodSequenceMerge.cs
- ListParaClient.cs
- DataControlPagerLinkButton.cs
- CapabilitiesSection.cs
- FrameworkElementFactoryMarkupObject.cs
- ScriptingProfileServiceSection.cs
- ResourceAttributes.cs
- ToolStripControlHost.cs
- IMembershipProvider.cs
- Substitution.cs
- GB18030Encoding.cs
- DescendantOverDescendantQuery.cs
- LicFileLicenseProvider.cs
- UrlMappingsSection.cs
- SqlCacheDependency.cs
- ObjectPersistData.cs
- RedirectionProxy.cs
- ServicesUtilities.cs
- D3DImage.cs
- SchemaLookupTable.cs
- ItemCheckedEvent.cs
- PropertyConverter.cs
- ToolStripDropTargetManager.cs
- XmlNodeComparer.cs
- Deserializer.cs
- ResourceBinder.cs
- WebPartDisplayModeCancelEventArgs.cs
- HtmlTable.cs
- ActivityExecutorDelegateInfo.cs
- PDBReader.cs
- Util.cs
- TextStore.cs
- HtmlMeta.cs
- SchemaImporter.cs
- SizeAnimationBase.cs
- BamlTreeUpdater.cs
- AttributeAction.cs
- DSASignatureDeformatter.cs
- StackSpiller.Temps.cs
- StructuralType.cs
- Operand.cs
- Privilege.cs
- XmlSignatureProperties.cs
- PrintPreviewGraphics.cs
- DataServiceBehavior.cs
- InputBindingCollection.cs
- EntityDataSourceWrapper.cs
- ReferentialConstraint.cs
- QilIterator.cs
- ListViewDeletedEventArgs.cs
- CmsInterop.cs
- ReflectionTypeLoadException.cs
- SubMenuStyle.cs
- SessionStateContainer.cs
- IdentityManager.cs
- initElementDictionary.cs
- TrackingCondition.cs
- SqlPersonalizationProvider.cs
- QuaternionKeyFrameCollection.cs
- RegexCompilationInfo.cs
- UpdatePanelTriggerCollection.cs
- OrderedDictionary.cs
- PlacementWorkspace.cs
- RSACryptoServiceProvider.cs
- SemaphoreSecurity.cs
- UniqueEventHelper.cs
- SqlDataSourceFilteringEventArgs.cs
- WSAddressing10ProblemHeaderQNameFault.cs
- MultiSelectRootGridEntry.cs
- SQLBoolean.cs
- ConnectionStringSettingsCollection.cs
- StringUtil.cs
- DataStreamFromComStream.cs
- MutexSecurity.cs
- FileAuthorizationModule.cs
- WebControl.cs
- DataErrorValidationRule.cs
- RuntimeCompatibilityAttribute.cs
- GroupByQueryOperator.cs
- ByteAnimation.cs
- DataSourceXmlSerializationAttribute.cs
- GridEntry.cs
- x509store.cs
- Range.cs
- SqlProvider.cs
- NavigationProperty.cs
- XamlFxTrace.cs
- DiscoveryDocumentReference.cs
- ParameterCollection.cs
- HttpClientCertificate.cs
- ColorPalette.cs
- MenuAdapter.cs
- AsmxEndpointPickerExtension.cs