Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / MessageEncodingBindingElement.cs / 1 / MessageEncodingBindingElement.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Channels { using System.Collections.Generic; using System.ServiceModel.Description; using System.Runtime.Serialization; using System.ServiceModel; using System.ServiceModel.Diagnostics; using System.Xml; public abstract class MessageEncodingBindingElement : BindingElement { protected MessageEncodingBindingElement() { } protected MessageEncodingBindingElement(MessageEncodingBindingElement elementToBeCloned) : base(elementToBeCloned) { } public abstract MessageVersion MessageVersion { get; set; } internal IChannelFactoryInternalBuildChannelFactory (BindingContext context) { if (context == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("context")); } #pragma warning suppress 56506 // [....], BindingContext.BindingParameters never be null context.BindingParameters.Add(this); return context.BuildInnerChannelFactory (); } internal bool InternalCanBuildChannelFactory (BindingContext context) { if (context == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("context")); } #pragma warning suppress 56506 // [....], BindingContext.BindingParameters never be null context.BindingParameters.Add(this); return context.CanBuildInnerChannelFactory (); } internal IChannelListener InternalBuildChannelListener (BindingContext context) where TChannel : class, IChannel { if (context == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("context")); } #pragma warning suppress 56506 // [....], BindingContext.BindingParameters never be null context.BindingParameters.Add(this); return context.BuildInnerChannelListener (); } internal bool InternalCanBuildChannelListener (BindingContext context) where TChannel : class, IChannel { if (context == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("context")); } #pragma warning suppress 56506 // [....], BindingContext.BindingParameters never be null context.BindingParameters.Add(this); return context.CanBuildInnerChannelListener (); } public abstract MessageEncoderFactory CreateMessageEncoderFactory(); public override T GetProperty (BindingContext context) { if (context == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("context"); } if (typeof(T) == typeof(MessageVersion)) { return (T)(object)this.MessageVersion; } else { return context.GetInnerProperty (); } } internal virtual bool CheckEncodingVersion(EnvelopeVersion version) { return false; } internal override bool IsMatch(BindingElement b) { if (b == null) return false; MessageEncodingBindingElement encoding = b as MessageEncodingBindingElement; if (encoding == null) return false; return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
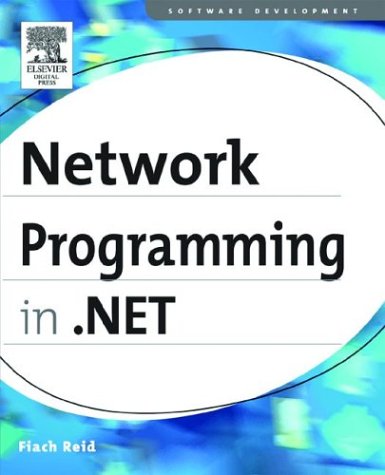
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UnsafeNativeMethods.cs
- CollectionBase.cs
- BindingSource.cs
- GlyphInfoList.cs
- AppDomainProtocolHandler.cs
- ParameterSubsegment.cs
- QilBinary.cs
- Codec.cs
- JoinGraph.cs
- ViewBox.cs
- SQLStringStorage.cs
- Size3DConverter.cs
- PasswordBoxAutomationPeer.cs
- InternalResources.cs
- EdmMember.cs
- Terminate.cs
- X509CertificateCollection.cs
- OdbcUtils.cs
- sortedlist.cs
- InstancePersistence.cs
- WSFederationHttpSecurityElement.cs
- PrivilegedConfigurationManager.cs
- DataGridViewCheckBoxColumn.cs
- MediaElementAutomationPeer.cs
- initElementDictionary.cs
- SerializableAttribute.cs
- XPathNavigator.cs
- DataGridViewLinkCell.cs
- PingReply.cs
- Pen.cs
- ToggleButton.cs
- HtmlElementErrorEventArgs.cs
- TextSchema.cs
- EmptyEnumerator.cs
- PreviewKeyDownEventArgs.cs
- PointValueSerializer.cs
- CodeGenHelper.cs
- HandlerBase.cs
- PublisherIdentityPermission.cs
- ScanQueryOperator.cs
- XmlSerializerSection.cs
- ServerIdentity.cs
- NamedPipeProcessProtocolHandler.cs
- ByteStack.cs
- Internal.cs
- TypedReference.cs
- SQLInt16Storage.cs
- ByteViewer.cs
- BindingList.cs
- _HelperAsyncResults.cs
- SqlReferenceCollection.cs
- ClientRuntimeConfig.cs
- CustomErrorsSectionWrapper.cs
- shaper.cs
- GlyphElement.cs
- UrlMapping.cs
- TrustManager.cs
- CodeDelegateInvokeExpression.cs
- UnsafeNativeMethodsTablet.cs
- NotCondition.cs
- HelpProvider.cs
- ConnectionOrientedTransportBindingElement.cs
- Paragraph.cs
- DataBindingHandlerAttribute.cs
- AudioLevelUpdatedEventArgs.cs
- TableLayoutPanel.cs
- ContainsRowNumberChecker.cs
- HGlobalSafeHandle.cs
- FilteredDataSetHelper.cs
- SafeThemeHandle.cs
- DataListCommandEventArgs.cs
- Int32CollectionConverter.cs
- TypeDependencyAttribute.cs
- SchemaCollectionCompiler.cs
- LayoutUtils.cs
- DistinctQueryOperator.cs
- XsltException.cs
- LassoSelectionBehavior.cs
- XsltInput.cs
- UrlRoutingModule.cs
- XmlReflectionMember.cs
- XamlStyleSerializer.cs
- SingleAnimation.cs
- LiteralControl.cs
- CodeNamespaceImportCollection.cs
- ChineseLunisolarCalendar.cs
- DocumentApplicationJournalEntry.cs
- DecimalConstantAttribute.cs
- Quad.cs
- DataGrid.cs
- ToolStripContainer.cs
- AvTraceFormat.cs
- M3DUtil.cs
- Underline.cs
- Transform3DCollection.cs
- PerformanceCounterPermissionEntry.cs
- TransportBindingElementImporter.cs
- CacheEntry.cs
- BamlBinaryReader.cs
- RequestCacheManager.cs