Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Dispatcher / ErrorBehavior.cs / 1 / ErrorBehavior.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System; using System.ServiceModel.Channels; using System.ServiceModel; using System.Collections.ObjectModel; using System.Collections.Generic; using System.ServiceModel.Diagnostics; class ErrorBehavior { IErrorHandler[] handlers; bool debug; bool isOnServer; MessageVersion messageVersion; internal ErrorBehavior(ChannelDispatcher channelDispatcher) { this.handlers = EmptyArray.ToArray(channelDispatcher.ErrorHandlers); this.debug = channelDispatcher.IncludeExceptionDetailInFaults; this.isOnServer = channelDispatcher.IsOnServer; this.messageVersion = channelDispatcher.MessageVersion; } void InitializeFault(ref MessageRpc rpc) { Exception error = rpc.Error; FaultException fault = error as FaultException; if (fault != null) { string action; MessageFault messageFault = rpc.Operation.FaultFormatter.Serialize(fault, out action); if (action == null) action = rpc.RequestVersion.Addressing.DefaultFaultAction; if (messageFault != null) rpc.FaultInfo.Fault = Message.CreateMessage(rpc.RequestVersion, messageFault, action); } } internal IErrorHandler[] Handlers { get { return handlers; } } internal void ProvideMessageFault(ref MessageRpc rpc) { if (rpc.Error != null) { ProvideMessageFaultCore(ref rpc); } } void ProvideMessageFaultCore(ref MessageRpc rpc) { if (this.messageVersion != rpc.RequestVersion) { DiagnosticUtility.DebugAssert("System.ServiceModel.Dispatcher.ErrorBehavior.ProvideMessageFaultCore(): (this.messageVersion != rpc.RequestVersion)"); } this.InitializeFault(ref rpc); this.ProvideFault(rpc.Error, rpc.Channel.GetProperty (), ref rpc.FaultInfo); this.ProvideMessageFaultCoreCoda(ref rpc); } void ProvideFaultOfLastResort(Exception error, ref ErrorHandlerFaultInfo faultInfo) { if (faultInfo.Fault == null) { FaultCode code = new FaultCode(FaultCodeConstants.Codes.InternalServiceFault, FaultCodeConstants.Namespaces.NetDispatch); code = FaultCode.CreateReceiverFaultCode(code); string action = FaultCodeConstants.Actions.NetDispatcher; MessageFault fault; if (this.debug) { faultInfo.DefaultFaultAction = action; fault = MessageFault.CreateFault(code, new FaultReason(error.Message), new ExceptionDetail(error)); } else { string reason = this.isOnServer ? SR.GetString(SR.SFxInternalServerError) : SR.GetString(SR.SFxInternalCallbackError); fault = MessageFault.CreateFault(code, new FaultReason(reason)); } faultInfo.IsConsideredUnhandled = true; faultInfo.Fault = Message.CreateMessage(this.messageVersion, fault, action); } } void ProvideMessageFaultCoreCoda(ref MessageRpc rpc) { if (rpc.FaultInfo.Fault.Headers.Action == null) { rpc.FaultInfo.Fault.Headers.Action = rpc.RequestVersion.Addressing.DefaultFaultAction; } rpc.Reply = rpc.FaultInfo.Fault; } internal void ProvideOnlyFaultOfLastResort(ref MessageRpc rpc) { this.ProvideFaultOfLastResort(rpc.Error, ref rpc.FaultInfo); this.ProvideMessageFaultCoreCoda(ref rpc); } internal void ProvideFault(Exception e, FaultConverter faultConverter, ref ErrorHandlerFaultInfo faultInfo) { ProvideWellKnownFault(e, faultConverter, ref faultInfo); for (int i=0; i
Link Menu
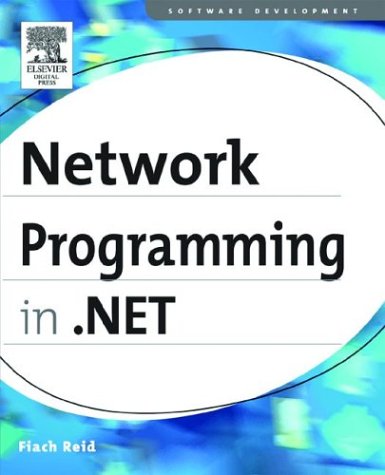
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DigestTraceRecordHelper.cs
- UnsafeNativeMethods.cs
- sapiproxy.cs
- GenericRootAutomationPeer.cs
- Normalization.cs
- Type.cs
- ListenerElementsCollection.cs
- InputEventArgs.cs
- XmlEncoding.cs
- ReplyAdapterChannelListener.cs
- XmlEncodedRawTextWriter.cs
- RestHandler.cs
- RegistrationProxy.cs
- Menu.cs
- ProviderException.cs
- ClientData.cs
- KeyGestureValueSerializer.cs
- LabelAutomationPeer.cs
- PropagatorResult.cs
- DependencyPropertyValueSerializer.cs
- Compilation.cs
- XmlEntityReference.cs
- MulticastDelegate.cs
- XmlIlVisitor.cs
- GenericTextProperties.cs
- SwitchElementsCollection.cs
- ActivityExecutionFilter.cs
- PerformanceCounterPermissionEntryCollection.cs
- DataTableCollection.cs
- UnknownWrapper.cs
- SchemaCollectionCompiler.cs
- SoapHeaders.cs
- externdll.cs
- XmlTextReaderImplHelpers.cs
- HwndSubclass.cs
- StreamUpdate.cs
- XmlIterators.cs
- RewritingSimplifier.cs
- FileAuthorizationModule.cs
- XdrBuilder.cs
- AppDomain.cs
- sqlmetadatafactory.cs
- WebPartZone.cs
- ResourceSet.cs
- CollectionBuilder.cs
- MenuScrollingVisibilityConverter.cs
- ExpressionParser.cs
- RepeaterItemEventArgs.cs
- DoWhileDesigner.xaml.cs
- AutomationPatternInfo.cs
- PingReply.cs
- HttpDebugHandler.cs
- InvalidComObjectException.cs
- DiscoveryEndpoint.cs
- Pair.cs
- IInstanceTable.cs
- Helper.cs
- Variant.cs
- StructuralObject.cs
- webproxy.cs
- SqlTransaction.cs
- XmlName.cs
- EdmRelationshipRoleAttribute.cs
- InkCollectionBehavior.cs
- DesignerTransaction.cs
- XPathParser.cs
- TreeWalkHelper.cs
- MissingMemberException.cs
- DeclaredTypeElementCollection.cs
- versioninfo.cs
- ListViewUpdateEventArgs.cs
- TextProviderWrapper.cs
- FrameworkElement.cs
- MouseOverProperty.cs
- SqlXml.cs
- FactoryMaker.cs
- FontCacheUtil.cs
- BreakRecordTable.cs
- AddingNewEventArgs.cs
- SoundPlayerAction.cs
- SelectorAutomationPeer.cs
- SqlCommandSet.cs
- TimeSpanValidator.cs
- StatusBarItem.cs
- EntityFunctions.cs
- HeaderPanel.cs
- ConfigXmlText.cs
- SocketCache.cs
- ContractMapping.cs
- RoleServiceManager.cs
- StreamGeometry.cs
- localization.cs
- TreeNodeBindingCollection.cs
- EventDescriptor.cs
- MsmqElementBase.cs
- DataRowChangeEvent.cs
- LinqDataSourceHelper.cs
- VisualStyleInformation.cs
- EdmFunctions.cs
- MsmqInputSessionChannel.cs