Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Security / Cryptography / ManifestSignatureInformation.cs / 1305376 / ManifestSignatureInformation.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Deployment.Internal; using System.Diagnostics; using System.IO; using System.Runtime.InteropServices.ComTypes; using System.Security; using System.Security.Cryptography.X509Certificates; using System.Security.Cryptography.Xml; using System.Xml; namespace System.Security.Cryptography { ////// Wrapper for information about the various signatures that can be applied to a manifest /// [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class ManifestSignatureInformation { private ManifestKinds m_manifest; private StrongNameSignatureInformation m_strongNameSignature; private AuthenticodeSignatureInformation m_authenticodeSignature; internal ManifestSignatureInformation(ManifestKinds manifest, StrongNameSignatureInformation strongNameSignature, AuthenticodeSignatureInformation authenticodeSignature) { Debug.Assert(manifest == ManifestKinds.Application || manifest == ManifestKinds.Deployment, "Invalid manifest for signature information"); m_manifest = manifest; m_strongNameSignature = strongNameSignature; m_authenticodeSignature = authenticodeSignature; } ////// Authenticode signature of the manifest /// public AuthenticodeSignatureInformation AuthenticodeSignature { get { return m_authenticodeSignature; } } ////// Manifest the signature information is for /// public ManifestKinds Manifest { get { return m_manifest; } } ////// Details about the strong name signature of the manifest /// public StrongNameSignatureInformation StrongNameSignature { get { return m_strongNameSignature; } } ////// Load the XML from the specified manifest into an XmlDocument /// //// [System.Security.SecurityCritical] private static XmlDocument GetManifestXml(ActivationContext application, ManifestKinds manifest) { Debug.Assert(application != null, "application != null"); IStream manifestStream = null; if (manifest == ManifestKinds.Application) { manifestStream = InternalActivationContextHelper.GetApplicationComponentManifest(application) as IStream; } else if (manifest == ManifestKinds.Deployment) { manifestStream = InternalActivationContextHelper.GetDeploymentComponentManifest(application) as IStream; } Debug.Assert(manifestStream != null, "Cannot get stream for manifest"); using (MemoryStream manifestContent = new MemoryStream()) { byte[] buffer = new byte[4096]; int bytesRead = 0; do { unsafe { manifestStream.Read(buffer, buffer.Length, new IntPtr(&bytesRead)); } manifestContent.Write(buffer, 0, bytesRead); } while (bytesRead == buffer.Length); manifestContent.Position = 0; XmlDocument manifestXml = new XmlDocument(); manifestXml.PreserveWhitespace = true; manifestXml.Load(manifestContent); return manifestXml; } } ///// /// Verify and gather information about the signatures of the specified manifests /// public static ManifestSignatureInformationCollection VerifySignature(ActivationContext application) { return VerifySignature(application, ManifestKinds.ApplicationAndDeployment); } ////// Verify and gather information about the signatures of the specified manifests /// public static ManifestSignatureInformationCollection VerifySignature(ActivationContext application, ManifestKinds manifests) { return VerifySignature(application, manifests, X509RevocationFlag.ExcludeRoot, X509RevocationMode.Online); } ////// Verify and gather information about the signatures of the specified manifests /// //// [System.Security.SecurityCritical] public static ManifestSignatureInformationCollection VerifySignature(ActivationContext application, ManifestKinds manifests, X509RevocationFlag revocationFlag, X509RevocationMode revocationMode) { if (application == null) { throw new ArgumentNullException("application"); } if (revocationFlag < X509RevocationFlag.EndCertificateOnly || X509RevocationFlag.ExcludeRoot < revocationFlag) { throw new ArgumentOutOfRangeException("revocationFlag"); } if (revocationMode < X509RevocationMode.NoCheck || X509RevocationMode.Offline < revocationMode) { throw new ArgumentOutOfRangeException("revocationMode"); } List// signatures = new List (); if ((manifests & ManifestKinds.Deployment) == ManifestKinds.Deployment) { XmlDocument deploymentManifest = GetManifestXml(application, ManifestKinds.Deployment); ManifestSignedXml deploymentSignature = new ManifestSignedXml(deploymentManifest, ManifestKinds.Deployment); signatures.Add(deploymentSignature.VerifySignature(revocationFlag, revocationMode)); } if ((manifests & ManifestKinds.Application) == ManifestKinds.Application) { XmlDocument applicationManifest = GetManifestXml(application, ManifestKinds.Application); ManifestSignedXml applicationSignature = new ManifestSignedXml(applicationManifest, ManifestKinds.Application); signatures.Add(applicationSignature.VerifySignature(revocationFlag, revocationMode)); } return new ManifestSignatureInformationCollection(signatures); } } [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class ManifestSignatureInformationCollection : ReadOnlyCollection { internal ManifestSignatureInformationCollection(IList signatureInformation) : base(signatureInformation) { return; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Deployment.Internal; using System.Diagnostics; using System.IO; using System.Runtime.InteropServices.ComTypes; using System.Security; using System.Security.Cryptography.X509Certificates; using System.Security.Cryptography.Xml; using System.Xml; namespace System.Security.Cryptography { /// /// Wrapper for information about the various signatures that can be applied to a manifest /// [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class ManifestSignatureInformation { private ManifestKinds m_manifest; private StrongNameSignatureInformation m_strongNameSignature; private AuthenticodeSignatureInformation m_authenticodeSignature; internal ManifestSignatureInformation(ManifestKinds manifest, StrongNameSignatureInformation strongNameSignature, AuthenticodeSignatureInformation authenticodeSignature) { Debug.Assert(manifest == ManifestKinds.Application || manifest == ManifestKinds.Deployment, "Invalid manifest for signature information"); m_manifest = manifest; m_strongNameSignature = strongNameSignature; m_authenticodeSignature = authenticodeSignature; } ////// Authenticode signature of the manifest /// public AuthenticodeSignatureInformation AuthenticodeSignature { get { return m_authenticodeSignature; } } ////// Manifest the signature information is for /// public ManifestKinds Manifest { get { return m_manifest; } } ////// Details about the strong name signature of the manifest /// public StrongNameSignatureInformation StrongNameSignature { get { return m_strongNameSignature; } } ////// Load the XML from the specified manifest into an XmlDocument /// //// [System.Security.SecurityCritical] private static XmlDocument GetManifestXml(ActivationContext application, ManifestKinds manifest) { Debug.Assert(application != null, "application != null"); IStream manifestStream = null; if (manifest == ManifestKinds.Application) { manifestStream = InternalActivationContextHelper.GetApplicationComponentManifest(application) as IStream; } else if (manifest == ManifestKinds.Deployment) { manifestStream = InternalActivationContextHelper.GetDeploymentComponentManifest(application) as IStream; } Debug.Assert(manifestStream != null, "Cannot get stream for manifest"); using (MemoryStream manifestContent = new MemoryStream()) { byte[] buffer = new byte[4096]; int bytesRead = 0; do { unsafe { manifestStream.Read(buffer, buffer.Length, new IntPtr(&bytesRead)); } manifestContent.Write(buffer, 0, bytesRead); } while (bytesRead == buffer.Length); manifestContent.Position = 0; XmlDocument manifestXml = new XmlDocument(); manifestXml.PreserveWhitespace = true; manifestXml.Load(manifestContent); return manifestXml; } } ///// /// Verify and gather information about the signatures of the specified manifests /// public static ManifestSignatureInformationCollection VerifySignature(ActivationContext application) { return VerifySignature(application, ManifestKinds.ApplicationAndDeployment); } ////// Verify and gather information about the signatures of the specified manifests /// public static ManifestSignatureInformationCollection VerifySignature(ActivationContext application, ManifestKinds manifests) { return VerifySignature(application, manifests, X509RevocationFlag.ExcludeRoot, X509RevocationMode.Online); } ////// Verify and gather information about the signatures of the specified manifests /// //// [System.Security.SecurityCritical] public static ManifestSignatureInformationCollection VerifySignature(ActivationContext application, ManifestKinds manifests, X509RevocationFlag revocationFlag, X509RevocationMode revocationMode) { if (application == null) { throw new ArgumentNullException("application"); } if (revocationFlag < X509RevocationFlag.EndCertificateOnly || X509RevocationFlag.ExcludeRoot < revocationFlag) { throw new ArgumentOutOfRangeException("revocationFlag"); } if (revocationMode < X509RevocationMode.NoCheck || X509RevocationMode.Offline < revocationMode) { throw new ArgumentOutOfRangeException("revocationMode"); } List// signatures = new List (); if ((manifests & ManifestKinds.Deployment) == ManifestKinds.Deployment) { XmlDocument deploymentManifest = GetManifestXml(application, ManifestKinds.Deployment); ManifestSignedXml deploymentSignature = new ManifestSignedXml(deploymentManifest, ManifestKinds.Deployment); signatures.Add(deploymentSignature.VerifySignature(revocationFlag, revocationMode)); } if ((manifests & ManifestKinds.Application) == ManifestKinds.Application) { XmlDocument applicationManifest = GetManifestXml(application, ManifestKinds.Application); ManifestSignedXml applicationSignature = new ManifestSignedXml(applicationManifest, ManifestKinds.Application); signatures.Add(applicationSignature.VerifySignature(revocationFlag, revocationMode)); } return new ManifestSignatureInformationCollection(signatures); } } [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class ManifestSignatureInformationCollection : ReadOnlyCollection { internal ManifestSignatureInformationCollection(IList signatureInformation) : base(signatureInformation) { return; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
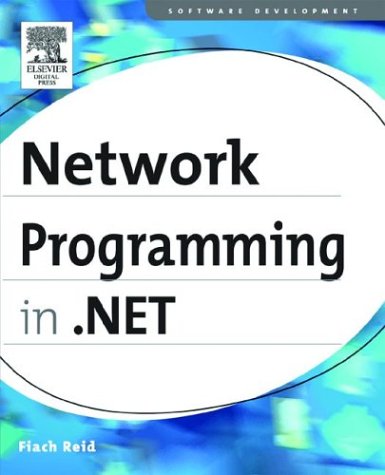
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataDocumentXPathNavigator.cs
- Menu.cs
- XmlAnyAttributeAttribute.cs
- OpCopier.cs
- CommandEventArgs.cs
- SiteMapNodeItemEventArgs.cs
- SafeUserTokenHandle.cs
- ImagingCache.cs
- ValueTypeFixupInfo.cs
- FormCollection.cs
- UserNameSecurityToken.cs
- CodeTypeReferenceExpression.cs
- BufferedReadStream.cs
- DataGridTextBoxColumn.cs
- LZCodec.cs
- BodyGlyph.cs
- PeerApplication.cs
- WebPartDisplayModeCollection.cs
- SerializationInfoEnumerator.cs
- PageBreakRecord.cs
- DbParameterCollection.cs
- TextFormatterHost.cs
- FlowPanelDesigner.cs
- ClientApiGenerator.cs
- AssemblyHash.cs
- BasePropertyDescriptor.cs
- Highlights.cs
- WorkflowInstance.cs
- SiteOfOriginPart.cs
- COM2DataTypeToManagedDataTypeConverter.cs
- CodeGenerator.cs
- EntitySqlQueryState.cs
- filewebrequest.cs
- ResourceLoader.cs
- DbConnectionStringBuilder.cs
- MemoryPressure.cs
- HandleRef.cs
- Registry.cs
- LoginName.cs
- ExpandoClass.cs
- SemaphoreSecurity.cs
- BufferedWebEventProvider.cs
- WebBrowserProgressChangedEventHandler.cs
- EncodingInfo.cs
- StylusDownEventArgs.cs
- ClassImporter.cs
- Types.cs
- DSACryptoServiceProvider.cs
- _StreamFramer.cs
- SchemaAttDef.cs
- AdRotator.cs
- XmlILConstructAnalyzer.cs
- ConditionalAttribute.cs
- RemoveStoryboard.cs
- Int32.cs
- TextParaClient.cs
- OciLobLocator.cs
- _Win32.cs
- BindingList.cs
- CodeCompileUnit.cs
- PrintSchema.cs
- DataGridPagerStyle.cs
- XmlSerializationWriter.cs
- ScrollBarAutomationPeer.cs
- EventLogger.cs
- WebPartMenu.cs
- WorkflowDebuggerSteppingAttribute.cs
- FreeFormPanel.cs
- FontStretch.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- CryptoApi.cs
- EventlogProvider.cs
- SspiHelper.cs
- XmlResolver.cs
- EntityContainerEmitter.cs
- BamlResourceDeserializer.cs
- SmtpDigestAuthenticationModule.cs
- MulticastDelegate.cs
- ResourcesBuildProvider.cs
- FtpWebResponse.cs
- TextComposition.cs
- NamespaceList.cs
- Semaphore.cs
- DiscoveryInnerClientManaged11.cs
- ConvertEvent.cs
- EdmMember.cs
- IgnoreFileBuildProvider.cs
- HyperLinkStyle.cs
- COAUTHINFO.cs
- MetaModel.cs
- LeafCellTreeNode.cs
- FrameworkReadOnlyPropertyMetadata.cs
- BufferAllocator.cs
- ButtonPopupAdapter.cs
- MemoryRecordBuffer.cs
- ElementFactory.cs
- ToolBarTray.cs
- MemberAssignment.cs
- RayHitTestParameters.cs
- SessionStateUtil.cs