Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Common / Utils / Boolean / Vertex.cs / 1305376 / Vertex.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; namespace System.Data.Common.Utils.Boolean { using System.Diagnostics; using System.Globalization; ////// A node in a Reduced Ordered Boolean Decision Diagram. Reads as: /// /// if 'Variable' then 'Then' else 'Else' /// /// Invariant: the Then and Else children must refer to 'deeper' variables, /// or variables with a higher value. Otherwise, the graph is not 'Ordered'. /// All creation of vertices is mediated by the Solver class which ensures /// each vertex is unique. Otherwise, the graph is not 'Reduced'. /// sealed class Vertex : IEquatable{ /// /// Initializes a sink BDD node (zero or one) /// private Vertex() { this.Variable = int.MaxValue; this.Children = new Vertex[] { }; } internal Vertex(int variable, Vertex[] children) { EntityUtil.BoolExprAssert(variable < int.MaxValue, "exceeded number of supported variables"); AssertConstructorArgumentsValid(variable, children); this.Variable = variable; this.Children = children; } [Conditional("DEBUG")] private static void AssertConstructorArgumentsValid(int variable, Vertex[] children) { Debug.Assert(null != children, "internal vertices must define children"); Debug.Assert(2 <= children.Length, "internal vertices must have at least two children"); Debug.Assert(0 < variable, "internal vertices must have 0 < variable"); foreach (Vertex child in children) { Debug.Assert(variable < child.Variable, "children must have greater variable"); } } ////// Sink node representing the Boolean function '1' (true) /// internal static readonly Vertex One = new Vertex(); ////// Sink node representing the Boolean function '0' (false) /// internal static readonly Vertex Zero = new Vertex(); ////// Gets the variable tested by this vertex. If this is a sink node, returns /// int.MaxValue since there is no variable to test (and since this is a leaf, /// this non-existent variable is 'deeper' than any existing variable; the /// variable value is larger than any real variable) /// internal readonly int Variable; ////// Note: do not modify elements. /// Gets the result when Variable evaluates to true. If this is a sink node, /// returns null. /// internal readonly Vertex[] Children; ////// Returns true if this is '1'. /// internal bool IsOne() { return object.ReferenceEquals(Vertex.One, this); } ////// Returns true if this is '0'. /// internal bool IsZero() { return object.ReferenceEquals(Vertex.Zero, this); } ////// Returns true if this is '0' or '1'. /// internal bool IsSink() { return Variable == int.MaxValue; } public bool Equals(Vertex other) { return object.ReferenceEquals(this, other); } public override bool Equals(object obj) { Debug.Fail("used typed Equals"); return base.Equals(obj); } public override int GetHashCode() { return base.GetHashCode(); } public override string ToString() { if (IsOne()) { return "_1_"; } if (IsZero()) { return "_0_"; } return String.Format(CultureInfo.InvariantCulture, "<{0}, {1}>", Variable, StringUtil.ToCommaSeparatedString(Children)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; namespace System.Data.Common.Utils.Boolean { using System.Diagnostics; using System.Globalization; ////// A node in a Reduced Ordered Boolean Decision Diagram. Reads as: /// /// if 'Variable' then 'Then' else 'Else' /// /// Invariant: the Then and Else children must refer to 'deeper' variables, /// or variables with a higher value. Otherwise, the graph is not 'Ordered'. /// All creation of vertices is mediated by the Solver class which ensures /// each vertex is unique. Otherwise, the graph is not 'Reduced'. /// sealed class Vertex : IEquatable{ /// /// Initializes a sink BDD node (zero or one) /// private Vertex() { this.Variable = int.MaxValue; this.Children = new Vertex[] { }; } internal Vertex(int variable, Vertex[] children) { EntityUtil.BoolExprAssert(variable < int.MaxValue, "exceeded number of supported variables"); AssertConstructorArgumentsValid(variable, children); this.Variable = variable; this.Children = children; } [Conditional("DEBUG")] private static void AssertConstructorArgumentsValid(int variable, Vertex[] children) { Debug.Assert(null != children, "internal vertices must define children"); Debug.Assert(2 <= children.Length, "internal vertices must have at least two children"); Debug.Assert(0 < variable, "internal vertices must have 0 < variable"); foreach (Vertex child in children) { Debug.Assert(variable < child.Variable, "children must have greater variable"); } } ////// Sink node representing the Boolean function '1' (true) /// internal static readonly Vertex One = new Vertex(); ////// Sink node representing the Boolean function '0' (false) /// internal static readonly Vertex Zero = new Vertex(); ////// Gets the variable tested by this vertex. If this is a sink node, returns /// int.MaxValue since there is no variable to test (and since this is a leaf, /// this non-existent variable is 'deeper' than any existing variable; the /// variable value is larger than any real variable) /// internal readonly int Variable; ////// Note: do not modify elements. /// Gets the result when Variable evaluates to true. If this is a sink node, /// returns null. /// internal readonly Vertex[] Children; ////// Returns true if this is '1'. /// internal bool IsOne() { return object.ReferenceEquals(Vertex.One, this); } ////// Returns true if this is '0'. /// internal bool IsZero() { return object.ReferenceEquals(Vertex.Zero, this); } ////// Returns true if this is '0' or '1'. /// internal bool IsSink() { return Variable == int.MaxValue; } public bool Equals(Vertex other) { return object.ReferenceEquals(this, other); } public override bool Equals(object obj) { Debug.Fail("used typed Equals"); return base.Equals(obj); } public override int GetHashCode() { return base.GetHashCode(); } public override string ToString() { if (IsOne()) { return "_1_"; } if (IsZero()) { return "_0_"; } return String.Format(CultureInfo.InvariantCulture, "<{0}, {1}>", Variable, StringUtil.ToCommaSeparatedString(Children)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
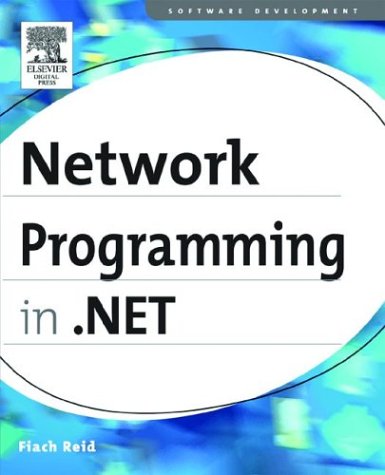
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TimelineClockCollection.cs
- LogLogRecord.cs
- HtmlInputRadioButton.cs
- ExpressionVisitor.cs
- ContentPresenter.cs
- FunctionNode.cs
- recordstatescratchpad.cs
- itemelement.cs
- PropertyChangedEventArgs.cs
- XmlReflectionImporter.cs
- HtmlTableRowCollection.cs
- XmlSerializerFactory.cs
- DefaultPerformanceCounters.cs
- DocobjHost.cs
- StringValidator.cs
- DrawListViewColumnHeaderEventArgs.cs
- NumericUpDown.cs
- ObjectViewListener.cs
- PrtCap_Base.cs
- MobileCapabilities.cs
- IssuedTokenClientBehaviorsElement.cs
- XmlnsPrefixAttribute.cs
- PrivateFontCollection.cs
- ResourceCategoryAttribute.cs
- XmlEnumAttribute.cs
- PngBitmapEncoder.cs
- ServiceBusyException.cs
- QueryOptionExpression.cs
- TemplateLookupAction.cs
- SharedPersonalizationStateInfo.cs
- DesignerCapabilities.cs
- SettingsAttributes.cs
- PartialList.cs
- ResourceExpressionBuilder.cs
- DictionaryManager.cs
- ElementProxy.cs
- HttpResponse.cs
- EndEvent.cs
- xdrvalidator.cs
- RuleProcessor.cs
- XmlDataDocument.cs
- AdjustableArrowCap.cs
- TableRowGroup.cs
- GridItemCollection.cs
- SqlBulkCopyColumnMapping.cs
- TemplateColumn.cs
- TextPointer.cs
- DerivedKeyCachingSecurityTokenSerializer.cs
- DataGridViewHeaderCell.cs
- AccessKeyManager.cs
- localization.cs
- NullableIntAverageAggregationOperator.cs
- RoleGroupCollectionEditor.cs
- ConnectionStringSettingsCollection.cs
- TemplateKey.cs
- PersonalizationState.cs
- TreeView.cs
- EdmToObjectNamespaceMap.cs
- DynamicDocumentPaginator.cs
- ToolStripOverflowButton.cs
- Rect3DValueSerializer.cs
- CorrelationManager.cs
- ProjectionCamera.cs
- SystemException.cs
- Tuple.cs
- SafeLocalMemHandle.cs
- ContactManager.cs
- TypeToArgumentTypeConverter.cs
- EntityKey.cs
- XmlILAnnotation.cs
- Italic.cs
- MimeMapping.cs
- HandlerFactoryCache.cs
- HttpDebugHandler.cs
- FullTrustAssembly.cs
- IntSecurity.cs
- Symbol.cs
- SqlWebEventProvider.cs
- Collection.cs
- XmlQueryTypeFactory.cs
- ObjectConverter.cs
- xamlnodes.cs
- OracleParameterBinding.cs
- TextParaLineResult.cs
- TemplateEditingFrame.cs
- DelegateCompletionCallbackWrapper.cs
- ServiceEndpointAssociationProvider.cs
- Matrix3D.cs
- GenericTypeParameterBuilder.cs
- DecoderFallback.cs
- TextLine.cs
- SystemMulticastIPAddressInformation.cs
- ExecutionScope.cs
- SamlSerializer.cs
- ZipPackage.cs
- WinEventQueueItem.cs
- odbcmetadatacollectionnames.cs
- TextBoxAutomationPeer.cs
- XmlArrayItemAttributes.cs
- Attributes.cs