Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / Providers / ServiceOperation.cs / 1305376 / ServiceOperation.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a type to represent custom operations on services. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Providers { using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; ///Use this class to represent a custom service operation. [DebuggerVisualizer("ServiceOperation={Name}")] public class ServiceOperation { ///Protocol (for example HTTP) method the service operation responds to. private readonly string method; ///In-order parameters for this operation. private readonly ReadOnlyCollectionparameters; /// Kind of result expected from this operation. private readonly ServiceOperationResultKind resultKind; ///Type of element of the method result. private readonly ResourceType resultType; ///Empty parameter collection. private static ReadOnlyCollectionemptyParameterCollection = new ReadOnlyCollection (new ServiceOperationParameter[0]); /// MIME type specified on primitive results, possibly null. private string mimeType; ///Entity set from which entities are read, if applicable. private ResourceSet resourceSet; ///name of the service operation. private string name; ///Is true, if the service operation is set to readonly i.e. fully initialized and validated. No more changes can be made, /// after the service operation is set to readonly. private bool isReadOnly; ////// Initializes a new /// name of the service operation. /// Kind of result expected from this operation. /// Type of element of the method result. /// EntitySet of the result expected from this operation. /// Protocol (for example HTTP) method the service operation responds to. /// In-order parameters for this operation. public ServiceOperation( string name, ServiceOperationResultKind resultKind, ResourceType resultType, ResourceSet resultSet, string method, IEnumerableinstance. /// parameters) { WebUtil.CheckStringArgumentNull(name, "name"); WebUtil.CheckServiceOperationResultKind(resultKind, "resultKind"); WebUtil.CheckStringArgumentNull(method, "method"); if ((resultKind == ServiceOperationResultKind.Void && resultType != null) || (resultKind != ServiceOperationResultKind.Void && resultType == null)) { throw new ArgumentException(Strings.ServiceOperation_ResultTypeAndKindMustMatch("resultKind", "resultType", ServiceOperationResultKind.Void)); } if ((resultType == null || resultType.ResourceTypeKind != ResourceTypeKind.EntityType) && resultSet != null) { throw new ArgumentException(Strings.ServiceOperation_ResultSetMustBeNull("resultSet", "resultType")); } if (resultType != null && resultType.ResourceTypeKind == ResourceTypeKind.EntityType && (resultSet == null || !resultSet.ResourceType.IsAssignableFrom(resultType))) { throw new ArgumentException(Strings.ServiceOperation_ResultTypeAndResultSetMustMatch("resultType", "resultSet")); } if (method != XmlConstants.HttpMethodGet && method != XmlConstants.HttpMethodPost) { throw new ArgumentException(Strings.ServiceOperation_NotSupportedProtocolMethod(method, name)); } this.name = name; this.resultKind = resultKind; this.resultType = resultType; this.resourceSet = resultSet; this.method = method; if (parameters == null) { this.parameters = ServiceOperation.emptyParameterCollection; } else { this.parameters = new ReadOnlyCollection (new List (parameters)); HashSet paramNames = new HashSet (StringComparer.Ordinal); foreach (ServiceOperationParameter p in this.parameters) { if (!paramNames.Add(p.Name)) { throw new ArgumentException(Strings.ServiceOperation_DuplicateParameterName(p.Name), "parameters"); } } } } /// Protocol (for example HTTP) method the service operation responds to. public string Method { get { return this.method; } } ///MIME type specified on primitive results, possibly null. public string MimeType { get { return this.mimeType; } set { this.ThrowIfSealed(); if (String.IsNullOrEmpty(value)) { throw new InvalidOperationException(Strings.ServiceOperation_MimeTypeCannotBeEmpty(this.Name)); } if (!WebUtil.IsValidMimeType(value)) { throw new InvalidOperationException(Strings.ServiceOperation_MimeTypeNotValid(value, this.Name)); } this.mimeType = value; } } ///Name of the service operation. public string Name { get { return this.name; } } ///Returns all the parameters for the given service operations./// public ReadOnlyCollectionParameters { get { return this.parameters; } } /// Kind of result expected from this operation. public ServiceOperationResultKind ResultKind { get { return this.resultKind; } } ///Element of result type. ////// Note that if the method returns an IEnumerable<string>, /// this property will be typeof(string). /// public ResourceType ResultType { get { return this.resultType; } } ////// PlaceHolder to hold custom state information about service operation. /// public object CustomState { get; set; } ////// Returns true, if this service operation has been set to read only. Otherwise returns false. /// public bool IsReadOnly { get { return this.isReadOnly; } } ///Entity set from which entities are read (possibly null). public ResourceSet ResourceSet { get { return this.resourceSet; } } ////// Set this service operation to readonly. /// public void SetReadOnly() { if (this.isReadOnly) { return; } foreach (ServiceOperationParameter parameter in this.Parameters) { parameter.SetReadOnly(); } this.isReadOnly = true; } ////// Throws an InvalidOperationException if this service operation is already set to readonly. /// internal void ThrowIfSealed() { if (this.isReadOnly) { throw new InvalidOperationException(Strings.ServiceOperation_Sealed(this.Name)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a type to represent custom operations on services. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Providers { using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; ///Use this class to represent a custom service operation. [DebuggerVisualizer("ServiceOperation={Name}")] public class ServiceOperation { ///Protocol (for example HTTP) method the service operation responds to. private readonly string method; ///In-order parameters for this operation. private readonly ReadOnlyCollectionparameters; /// Kind of result expected from this operation. private readonly ServiceOperationResultKind resultKind; ///Type of element of the method result. private readonly ResourceType resultType; ///Empty parameter collection. private static ReadOnlyCollectionemptyParameterCollection = new ReadOnlyCollection (new ServiceOperationParameter[0]); /// MIME type specified on primitive results, possibly null. private string mimeType; ///Entity set from which entities are read, if applicable. private ResourceSet resourceSet; ///name of the service operation. private string name; ///Is true, if the service operation is set to readonly i.e. fully initialized and validated. No more changes can be made, /// after the service operation is set to readonly. private bool isReadOnly; ////// Initializes a new /// name of the service operation. /// Kind of result expected from this operation. /// Type of element of the method result. /// EntitySet of the result expected from this operation. /// Protocol (for example HTTP) method the service operation responds to. /// In-order parameters for this operation. public ServiceOperation( string name, ServiceOperationResultKind resultKind, ResourceType resultType, ResourceSet resultSet, string method, IEnumerableinstance. /// parameters) { WebUtil.CheckStringArgumentNull(name, "name"); WebUtil.CheckServiceOperationResultKind(resultKind, "resultKind"); WebUtil.CheckStringArgumentNull(method, "method"); if ((resultKind == ServiceOperationResultKind.Void && resultType != null) || (resultKind != ServiceOperationResultKind.Void && resultType == null)) { throw new ArgumentException(Strings.ServiceOperation_ResultTypeAndKindMustMatch("resultKind", "resultType", ServiceOperationResultKind.Void)); } if ((resultType == null || resultType.ResourceTypeKind != ResourceTypeKind.EntityType) && resultSet != null) { throw new ArgumentException(Strings.ServiceOperation_ResultSetMustBeNull("resultSet", "resultType")); } if (resultType != null && resultType.ResourceTypeKind == ResourceTypeKind.EntityType && (resultSet == null || !resultSet.ResourceType.IsAssignableFrom(resultType))) { throw new ArgumentException(Strings.ServiceOperation_ResultTypeAndResultSetMustMatch("resultType", "resultSet")); } if (method != XmlConstants.HttpMethodGet && method != XmlConstants.HttpMethodPost) { throw new ArgumentException(Strings.ServiceOperation_NotSupportedProtocolMethod(method, name)); } this.name = name; this.resultKind = resultKind; this.resultType = resultType; this.resourceSet = resultSet; this.method = method; if (parameters == null) { this.parameters = ServiceOperation.emptyParameterCollection; } else { this.parameters = new ReadOnlyCollection (new List (parameters)); HashSet paramNames = new HashSet (StringComparer.Ordinal); foreach (ServiceOperationParameter p in this.parameters) { if (!paramNames.Add(p.Name)) { throw new ArgumentException(Strings.ServiceOperation_DuplicateParameterName(p.Name), "parameters"); } } } } /// Protocol (for example HTTP) method the service operation responds to. public string Method { get { return this.method; } } ///MIME type specified on primitive results, possibly null. public string MimeType { get { return this.mimeType; } set { this.ThrowIfSealed(); if (String.IsNullOrEmpty(value)) { throw new InvalidOperationException(Strings.ServiceOperation_MimeTypeCannotBeEmpty(this.Name)); } if (!WebUtil.IsValidMimeType(value)) { throw new InvalidOperationException(Strings.ServiceOperation_MimeTypeNotValid(value, this.Name)); } this.mimeType = value; } } ///Name of the service operation. public string Name { get { return this.name; } } ///Returns all the parameters for the given service operations./// public ReadOnlyCollectionParameters { get { return this.parameters; } } /// Kind of result expected from this operation. public ServiceOperationResultKind ResultKind { get { return this.resultKind; } } ///Element of result type. ////// Note that if the method returns an IEnumerable<string>, /// this property will be typeof(string). /// public ResourceType ResultType { get { return this.resultType; } } ////// PlaceHolder to hold custom state information about service operation. /// public object CustomState { get; set; } ////// Returns true, if this service operation has been set to read only. Otherwise returns false. /// public bool IsReadOnly { get { return this.isReadOnly; } } ///Entity set from which entities are read (possibly null). public ResourceSet ResourceSet { get { return this.resourceSet; } } ////// Set this service operation to readonly. /// public void SetReadOnly() { if (this.isReadOnly) { return; } foreach (ServiceOperationParameter parameter in this.Parameters) { parameter.SetReadOnly(); } this.isReadOnly = true; } ////// Throws an InvalidOperationException if this service operation is already set to readonly. /// internal void ThrowIfSealed() { if (this.isReadOnly) { throw new InvalidOperationException(Strings.ServiceOperation_Sealed(this.Name)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
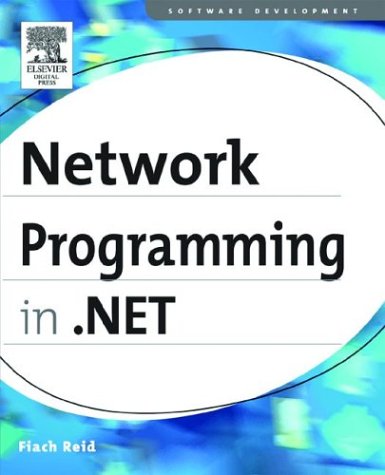
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- wgx_commands.cs
- WindowsListViewSubItem.cs
- cookiecollection.cs
- FixUpCollection.cs
- RankException.cs
- DescendentsWalker.cs
- ConfigurationManagerInternalFactory.cs
- DropDownList.cs
- GenericAuthenticationEventArgs.cs
- ProfileGroupSettings.cs
- SolidColorBrush.cs
- ValueTable.cs
- StateMachineWorkflow.cs
- Metafile.cs
- TearOffProxy.cs
- ByteStream.cs
- CodeConstructor.cs
- FileCodeGroup.cs
- AttributeQuery.cs
- HtmlElementCollection.cs
- GenericPrincipal.cs
- ClientProxyGenerator.cs
- KeyProperty.cs
- TextDecoration.cs
- PreservationFileReader.cs
- XmlAnyElementAttributes.cs
- NetworkInterface.cs
- DataBoundControlHelper.cs
- WebPartConnectionsConfigureVerb.cs
- UrlPath.cs
- GridViewColumnCollectionChangedEventArgs.cs
- SchemaAttDef.cs
- SettingsPropertyValue.cs
- UIElementParagraph.cs
- VirtualizingPanel.cs
- FileLogRecord.cs
- SqlParameter.cs
- ResourcePermissionBaseEntry.cs
- DependencyPropertyKind.cs
- PaperSource.cs
- Dynamic.cs
- CustomActivityDesigner.cs
- PackageRelationshipSelector.cs
- SmtpFailedRecipientException.cs
- TimerEventSubscriptionCollection.cs
- TextDecoration.cs
- KeyGestureConverter.cs
- MetadataArtifactLoader.cs
- FieldMetadata.cs
- BufferedResponseStream.cs
- FilteredDataSetHelper.cs
- TypeLoadException.cs
- DesignerMetadata.cs
- KoreanLunisolarCalendar.cs
- SimpleHandlerBuildProvider.cs
- SmtpSection.cs
- CqlGenerator.cs
- SubtreeProcessor.cs
- InputScopeConverter.cs
- EventProxy.cs
- IsolatedStorageFileStream.cs
- MultiSelectRootGridEntry.cs
- ping.cs
- MembershipUser.cs
- Config.cs
- DictionaryManager.cs
- SafeFileHandle.cs
- XmlDocumentType.cs
- ComponentCollection.cs
- _SecureChannel.cs
- EntityObject.cs
- MenuItemBindingCollection.cs
- BadImageFormatException.cs
- EnumUnknown.cs
- XslException.cs
- DropShadowEffect.cs
- PasswordBoxAutomationPeer.cs
- OracleNumber.cs
- UnSafeCharBuffer.cs
- LoginView.cs
- Convert.cs
- BaseProcessor.cs
- BooleanAnimationUsingKeyFrames.cs
- PageThemeCodeDomTreeGenerator.cs
- ZipIOBlockManager.cs
- TextUtf8RawTextWriter.cs
- LogWriteRestartAreaState.cs
- CryptoHelper.cs
- _BaseOverlappedAsyncResult.cs
- MetadataUtil.cs
- DateTimeValueSerializerContext.cs
- GroupedContextMenuStrip.cs
- TextTreeNode.cs
- TextFragmentEngine.cs
- FileSystemInfo.cs
- InputProcessorProfiles.cs
- AssemblySettingAttributes.cs
- SplineQuaternionKeyFrame.cs
- RC2CryptoServiceProvider.cs
- ValidationHelper.cs