Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / Providers / ServiceOperationParameter.cs / 1305376 / ServiceOperationParameter.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a type to represent parameter information for service // operations. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Providers { using System.Diagnostics; ///Use this type to represent a parameter on a service operation. [DebuggerVisualizer("ServiceOperationParameter={Name}")] public class ServiceOperationParameter { ///Parameter name. private readonly string name; ///Parameter type. private readonly ResourceType type; ///Is true, if the service operation parameter is set to readonly i.e. fully initialized and validated. /// No more changes can be made, after this is set to readonly. private bool isReadOnly; ////// Initializes a new /// Name of parameter. /// resource type of parameter value. public ServiceOperationParameter(string name, ResourceType parameterType) { WebUtil.CheckStringArgumentNull(name, "name"); WebUtil.CheckArgumentNull(parameterType, "parameterType"); if (parameterType.ResourceTypeKind != ResourceTypeKind.Primitive) { throw new ArgumentException(Strings.ServiceOperationParameter_TypeNotSupported(name, parameterType.FullName)); } this.name = name; this.type = parameterType; } ///. /// Name of parameter. public string Name { get { return this.name; } } ///Type of parameter values. public ResourceType ParameterType { get { return this.type; } } ////// PlaceHolder to hold custom state information about service operation parameter. /// public object CustomState { get; set; } ////// Returns true, if this parameter has been set to read only. Otherwise returns false. /// public bool IsReadOnly { get { return this.isReadOnly; } } ////// Sets this service operation parameter to readonly. /// public void SetReadOnly() { if (this.isReadOnly) { return; } this.isReadOnly = true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a type to represent parameter information for service // operations. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Providers { using System.Diagnostics; ///Use this type to represent a parameter on a service operation. [DebuggerVisualizer("ServiceOperationParameter={Name}")] public class ServiceOperationParameter { ///Parameter name. private readonly string name; ///Parameter type. private readonly ResourceType type; ///Is true, if the service operation parameter is set to readonly i.e. fully initialized and validated. /// No more changes can be made, after this is set to readonly. private bool isReadOnly; ////// Initializes a new /// Name of parameter. /// resource type of parameter value. public ServiceOperationParameter(string name, ResourceType parameterType) { WebUtil.CheckStringArgumentNull(name, "name"); WebUtil.CheckArgumentNull(parameterType, "parameterType"); if (parameterType.ResourceTypeKind != ResourceTypeKind.Primitive) { throw new ArgumentException(Strings.ServiceOperationParameter_TypeNotSupported(name, parameterType.FullName)); } this.name = name; this.type = parameterType; } ///. /// Name of parameter. public string Name { get { return this.name; } } ///Type of parameter values. public ResourceType ParameterType { get { return this.type; } } ////// PlaceHolder to hold custom state information about service operation parameter. /// public object CustomState { get; set; } ////// Returns true, if this parameter has been set to read only. Otherwise returns false. /// public bool IsReadOnly { get { return this.isReadOnly; } } ////// Sets this service operation parameter to readonly. /// public void SetReadOnly() { if (this.isReadOnly) { return; } this.isReadOnly = true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
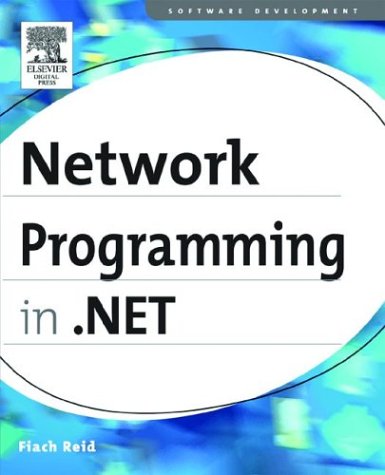
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlLoader.cs
- Deserializer.cs
- PLINQETWProvider.cs
- SystemEvents.cs
- RawUIStateInputReport.cs
- MaskInputRejectedEventArgs.cs
- XamlStream.cs
- DependencyStoreSurrogate.cs
- StringFreezingAttribute.cs
- TdsParameterSetter.cs
- CodeParameterDeclarationExpressionCollection.cs
- WinInet.cs
- SingleObjectCollection.cs
- JoinTreeNode.cs
- StatusBarItemAutomationPeer.cs
- SerializationEventsCache.cs
- CollectionBase.cs
- FontStyles.cs
- GetRecipientRequest.cs
- ProcessManager.cs
- EntityDataSourceContainerNameItem.cs
- DataGridItemEventArgs.cs
- CodeRegionDirective.cs
- MarkupProperty.cs
- XNodeNavigator.cs
- HwndStylusInputProvider.cs
- Normalization.cs
- KeyValueConfigurationElement.cs
- AliasGenerator.cs
- TextEffectCollection.cs
- XamlReader.cs
- HttpChannelBindingToken.cs
- PagedDataSource.cs
- PerspectiveCamera.cs
- ClientApiGenerator.cs
- AssemblySettingAttributes.cs
- selecteditemcollection.cs
- SqlAliaser.cs
- FormsAuthenticationTicket.cs
- SecurityTokenContainer.cs
- RemotingAttributes.cs
- PrivilegedConfigurationManager.cs
- OutOfMemoryException.cs
- HtmlTableRow.cs
- TimeIntervalCollection.cs
- SHA1.cs
- AppearanceEditorPart.cs
- ProviderConnectionPointCollection.cs
- DbMetaDataColumnNames.cs
- LinkAreaEditor.cs
- RegisteredScript.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- URLIdentityPermission.cs
- XmlRawWriter.cs
- SqlReferenceCollection.cs
- OpenTypeCommon.cs
- ObjectTag.cs
- PageParser.cs
- WebPartManagerInternals.cs
- CellParagraph.cs
- ColorBuilder.cs
- WebPartZoneBaseDesigner.cs
- UnsafeNativeMethods.cs
- SqlServer2KCompatibilityAnnotation.cs
- DbDataAdapter.cs
- ArrayItemValue.cs
- DictionaryEntry.cs
- EntityWithKeyStrategy.cs
- BehaviorEditorPart.cs
- GraphicsState.cs
- EditingMode.cs
- EventMappingSettings.cs
- RowUpdatedEventArgs.cs
- XmlLinkedNode.cs
- MLangCodePageEncoding.cs
- SendKeys.cs
- IdentityNotMappedException.cs
- ToolStripScrollButton.cs
- WindowsRebar.cs
- XmlNamespaceMapping.cs
- RoleManagerEventArgs.cs
- EntityDataSourceReferenceGroup.cs
- FlowDocumentFormatter.cs
- OleTxTransaction.cs
- StorageAssociationTypeMapping.cs
- XmlSchemaExporter.cs
- InboundActivityHelper.cs
- TextPatternIdentifiers.cs
- AxisAngleRotation3D.cs
- SequentialActivityDesigner.cs
- SchemaNamespaceManager.cs
- PropertyItem.cs
- RuntimeHelpers.cs
- StateWorkerRequest.cs
- ThreadStateException.cs
- EditorPartChrome.cs
- AnonymousIdentificationSection.cs
- MouseGestureValueSerializer.cs
- Profiler.cs
- CodeDirectoryCompiler.cs