Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Services / Messaging / System / Messaging / Cursor.cs / 1305376 / Cursor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Messaging { using System.Messaging.Interop; public sealed class Cursor : IDisposable { private CursorHandle handle; private bool disposed; internal Cursor(MessageQueue queue) { CursorHandle result; int status = SafeNativeMethods.MQCreateCursor(queue.MQInfo.ReadHandle, out result); if (MessageQueue.IsFatalError(status)) throw new MessageQueueException(status); this.handle = result; } internal CursorHandle Handle { get { if (disposed) throw new ObjectDisposedException(GetType().Name); return handle; } } public void Close() { handle.Close(); } public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } void Dispose(bool disposing) { // disposing argument is intentionally unused this.Close(); this.disposed = true; } ~Cursor() { Dispose(false); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Messaging { using System.Messaging.Interop; public sealed class Cursor : IDisposable { private CursorHandle handle; private bool disposed; internal Cursor(MessageQueue queue) { CursorHandle result; int status = SafeNativeMethods.MQCreateCursor(queue.MQInfo.ReadHandle, out result); if (MessageQueue.IsFatalError(status)) throw new MessageQueueException(status); this.handle = result; } internal CursorHandle Handle { get { if (disposed) throw new ObjectDisposedException(GetType().Name); return handle; } } public void Close() { handle.Close(); } public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } void Dispose(bool disposing) { // disposing argument is intentionally unused this.Close(); this.disposed = true; } ~Cursor() { Dispose(false); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
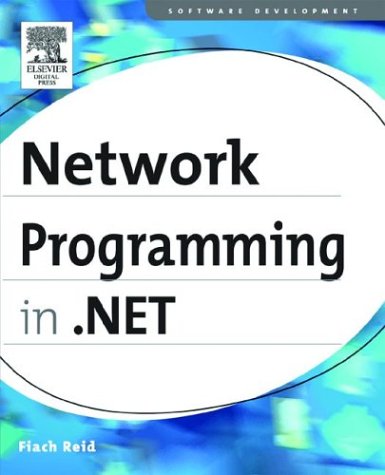
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Properties.cs
- followingsibling.cs
- Cursor.cs
- SafeHandle.cs
- HttpHandlerActionCollection.cs
- ConfigXmlWhitespace.cs
- GiveFeedbackEvent.cs
- IgnoreDataMemberAttribute.cs
- KerberosSecurityTokenProvider.cs
- UpdateManifestForBrowserApplication.cs
- FillErrorEventArgs.cs
- PointAnimationClockResource.cs
- Itemizer.cs
- TrustManagerMoreInformation.cs
- HttpHandlersSection.cs
- TextServicesDisplayAttributePropertyRanges.cs
- SettingsPropertyIsReadOnlyException.cs
- SqlServer2KCompatibilityCheck.cs
- XmlHelper.cs
- KeyTime.cs
- WebPermission.cs
- DrawItemEvent.cs
- OleDbEnumerator.cs
- InputQueueChannel.cs
- Positioning.cs
- ObjectSecurityT.cs
- AnnotationObservableCollection.cs
- ValidatorCollection.cs
- ObjectListTitleAttribute.cs
- UnionCodeGroup.cs
- Properties.cs
- DateTimeFormatInfoScanner.cs
- OpCellTreeNode.cs
- ButtonFieldBase.cs
- TextDpi.cs
- RuntimeCompatibilityAttribute.cs
- NameValuePermission.cs
- PropagatorResult.cs
- CatalogPartCollection.cs
- UdpReplyToBehavior.cs
- ThreadStateException.cs
- SearchExpression.cs
- TreeViewBindingsEditor.cs
- EdmRelationshipRoleAttribute.cs
- TransportBindingElementImporter.cs
- WindowShowOrOpenTracker.cs
- WebPartsPersonalizationAuthorization.cs
- ServiceDeploymentInfo.cs
- Int32Converter.cs
- WinInetCache.cs
- Function.cs
- KeyConverter.cs
- PaperSource.cs
- BinaryFormatter.cs
- DLinqDataModelProvider.cs
- SortedSetDebugView.cs
- FloaterBaseParaClient.cs
- WebPartDeleteVerb.cs
- ErrorCodes.cs
- RectAnimationUsingKeyFrames.cs
- MetadataArtifactLoaderCompositeFile.cs
- DirectoryInfo.cs
- EventHandlersStore.cs
- StreamingContext.cs
- ValueCollectionParameterReader.cs
- Dispatcher.cs
- __Filters.cs
- TracingConnectionInitiator.cs
- WindowsGraphicsCacheManager.cs
- OptimizerPatterns.cs
- FormsAuthenticationUser.cs
- UserMapPath.cs
- ComPersistableTypeElementCollection.cs
- GetPageNumberCompletedEventArgs.cs
- SqlBuffer.cs
- ToolStripCustomTypeDescriptor.cs
- XmlAttributeProperties.cs
- HttpModuleAction.cs
- Unit.cs
- WebPageTraceListener.cs
- StreamDocument.cs
- IndentedWriter.cs
- CellCreator.cs
- ReadOnlyDictionary.cs
- FormViewInsertEventArgs.cs
- ServerIdentity.cs
- TextServicesDisplayAttributePropertyRanges.cs
- SHA384Managed.cs
- XPathNodeHelper.cs
- WindowsFormsHost.cs
- AnnotationAdorner.cs
- ImageListStreamer.cs
- KeyInterop.cs
- ClientSettingsStore.cs
- ErrorInfoXmlDocument.cs
- CommonDialog.cs
- PingOptions.cs
- StreamGeometry.cs
- ConfigXmlSignificantWhitespace.cs
- TextTreeRootTextBlock.cs