Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Wmi / managed / System / Management / PropertySet.cs / 1305376 / PropertySet.cs
using System.Collections; using System.Diagnostics; using System.Runtime.InteropServices; using WbemClient_v1; using System.ComponentModel; namespace System.Management { //CCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCC// ////// ///Represents the set of properties of a WMI object. ////// //CCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCC// public class PropertyDataCollection : ICollection, IEnumerable { private ManagementBaseObject parent; bool isSystem; internal PropertyDataCollection(ManagementBaseObject parent, bool isSystem) : base() { this.parent = parent; this.isSystem = isSystem; } // //ICollection // ///using System; /// using System.Management; /// /// // This sample demonstrates how to enumerate properties /// // in a ManagementObject object. /// class Sample_PropertyDataCollection /// { /// public static int Main(string[] args) { /// ManagementObject disk = new ManagementObject("win32_logicaldisk.deviceid = \"c:\""); /// PropertyDataCollection diskProperties = disk.Properties; /// foreach (PropertyData diskProperty in diskProperties) { /// Console.WriteLine("Property = " + diskProperty.Name); /// } /// return 0; /// } /// } ///
///Imports System /// Imports System.Management /// /// ' This sample demonstrates how to enumerate properties /// ' in a ManagementObject object. /// Class Sample_PropertyDataCollection /// Overloads Public Shared Function Main(args() As String) As Integer /// Dim disk As New ManagementObject("win32_logicaldisk.deviceid=""c:""") /// Dim diskProperties As PropertyDataCollection = disk.Properties /// Dim diskProperty As PropertyData /// For Each diskProperty In diskProperties /// Console.WriteLine("Property = " & diskProperty.Name) /// Next diskProperty /// Return 0 /// End Function /// End Class ///
////// ///Gets or sets the number of objects in the ///. /// public int Count { get { string[] propertyNames = null; object qualVal = null; int flag; if (isSystem) flag = (int)tag_WBEM_CONDITION_FLAG_TYPE.WBEM_FLAG_SYSTEM_ONLY; else flag = (int)tag_WBEM_CONDITION_FLAG_TYPE.WBEM_FLAG_NONSYSTEM_ONLY; flag |= (int)tag_WBEM_CONDITION_FLAG_TYPE.WBEM_FLAG_ALWAYS; int status = parent.wbemObject.GetNames_(null, flag, ref qualVal, out propertyNames); if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } return propertyNames.Length; } } ///The number of objects in the collection. ////// ///Gets or sets a value indicating whether the object is synchronized. ////// public bool IsSynchronized { get { return false; } } ////// if the object is synchronized; /// otherwise, . /// ///Gets or sets the object to be used for synchronization. ////// public object SyncRoot { get { return this; } } ///The object to be used for synchronization. ////// ///Copies the ///into an array. /// /// The array to which to copy theCopies the ///into an array. . /// The index from which to start copying. public void CopyTo(Array array, Int32 index) { if (null == array) throw new ArgumentNullException("array"); if ((index < array.GetLowerBound(0)) || (index > array.GetUpperBound(0))) throw new ArgumentOutOfRangeException("index"); // Get the names of the properties string[] nameArray = null; object dummy = null; int flag = 0; if (isSystem) flag |= (int)tag_WBEM_CONDITION_FLAG_TYPE.WBEM_FLAG_SYSTEM_ONLY; else flag |= (int)tag_WBEM_CONDITION_FLAG_TYPE.WBEM_FLAG_NONSYSTEM_ONLY; flag |= (int)tag_WBEM_CONDITION_FLAG_TYPE.WBEM_FLAG_ALWAYS; int status = this.parent.wbemObject.GetNames_(null, flag, ref dummy, out nameArray); if (status >= 0) { if ((index + nameArray.Length) > array.Length) throw new ArgumentException(null,"index"); foreach (string propertyName in nameArray) array.SetValue(new PropertyData(parent, propertyName), index++); } if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } return; } /// /// /// The destination array to contain the copiedCopies the ///to a specialized object /// array. . /// The index in the destination array from which to start copying. public void CopyTo(PropertyData[] propertyArray, Int32 index) { CopyTo((Array)propertyArray, index); } // // IEnumerable // IEnumerator IEnumerable.GetEnumerator() { return (IEnumerator)(new PropertyDataEnumerator(parent, isSystem)); } /// /// ///Returns the enumerator for this ///. /// public PropertyDataEnumerator GetEnumerator() { return new PropertyDataEnumerator(parent, isSystem); } //Enumerator class ///An ////// that can be used to iterate through the collection. /// ///Represents the enumerator for ////// objects in the . /// public class PropertyDataEnumerator : IEnumerator { private ManagementBaseObject parent; private string[] propertyNames; private int index; internal PropertyDataEnumerator(ManagementBaseObject parent, bool isSystem) { this.parent = parent; propertyNames = null; index = -1; int flag; object qualVal = null; if (isSystem) flag = (int)tag_WBEM_CONDITION_FLAG_TYPE.WBEM_FLAG_SYSTEM_ONLY; else flag = (int)tag_WBEM_CONDITION_FLAG_TYPE.WBEM_FLAG_NONSYSTEM_ONLY; flag |= (int)tag_WBEM_CONDITION_FLAG_TYPE.WBEM_FLAG_ALWAYS; int status = parent.wbemObject.GetNames_(null, flag, ref qualVal, out propertyNames); if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } } ///using System; /// using System.Management; /// /// // This sample demonstrates how to enumerate all properties in a /// // ManagementObject using the PropertyDataEnumerator object. /// class Sample_PropertyDataEnumerator /// { /// public static int Main(string[] args) { /// ManagementObject disk = new ManagementObject("Win32_LogicalDisk.DeviceID='C:'"); /// PropertyDataCollection.PropertyDataEnumerator propertyEnumerator = disk.Properties.GetEnumerator(); /// while(propertyEnumerator.MoveNext()) { /// PropertyData p = (PropertyData)propertyEnumerator.Current; /// Console.WriteLine("Property found: " + p.Name); /// } /// return 0; /// } /// } ///
///Imports System /// Imports System.Management /// /// ' This sample demonstrates how to enumerate all properties in a /// ' ManagementObject using PropertyDataEnumerator object. /// Class Sample_PropertyDataEnumerator /// Overloads Public Shared Function Main(args() As String) As Integer /// Dim disk As New ManagementObject("Win32_LogicalDisk.DeviceID='C:'") /// Dim propertyEnumerator As _ /// PropertyDataCollection.PropertyDataEnumerator = disk.Properties.GetEnumerator() /// While propertyEnumerator.MoveNext() /// Dim p As PropertyData = _ /// CType(propertyEnumerator.Current, PropertyData) /// Console.WriteLine("Property found: " & p.Name) /// End While /// Return 0 /// End Function /// End Class ///
///object IEnumerator.Current { get { return (object)this.Current; } } /// /// ///Gets the current ///in the enumeration. /// The current public PropertyData Current { get { if ((index == -1) || (index == propertyNames.Length)) throw new InvalidOperationException(); else return new PropertyData(parent, propertyNames[index]); } } ////// element in the collection. /// /// ///Moves to the next element in the ////// enumeration. /// public bool MoveNext() { if (index == propertyNames.Length) //passed the end of the array return false; //don't advance the index any more index++; return (index == propertyNames.Length) ? false : true; } ////// if the enumerator was successfully advanced to the next element; /// if the enumerator has passed the end of the collection. /// public void Reset() { index = -1; } }//PropertyDataEnumerator // // Methods // ///Resets the enumerator to the beginning of the ////// enumeration. /// /// The name of the property to retrieve. ///Returns the specified property from the ///, using [] syntax. /// ///A ///, based on /// the name specified. /// public virtual PropertyData this[string propertyName] { get { if (null == propertyName) throw new ArgumentNullException("propertyName"); return new PropertyData(parent, propertyName); } } ///ManagementObject o = new ManagementObject("Win32_LogicalDisk.Name = 'C:'"); /// Console.WriteLine("Free space on C: drive is: ", c.Properties["FreeSpace"].Value); ///
///Dim o As New ManagementObject("Win32_LogicalDisk.Name=""C:""") /// Console.WriteLine("Free space on C: drive is: " & c.Properties("FreeSpace").Value) ///
////// /// The name of the property to be removed. ///Removes a ///from the . /// ///Properties can only be removed from class definitions, /// not from instances. This method is only valid when invoked on a property /// collection in a ///. /// public virtual void Remove(string propertyName) { // On instances, reset the property to the default value for the class. if (parent.GetType() == typeof(ManagementObject)) { ManagementClass cls = new ManagementClass(parent.ClassPath); parent.SetPropertyValue(propertyName, cls.GetPropertyValue(propertyName)); } else { int status = parent.wbemObject.Delete_(propertyName); if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } } } ///ManagementClass c = new ManagementClass("MyClass"); /// c.Properties.Remove("PropThatIDontWantOnThisClass"); ///
///Dim c As New ManagementClass("MyClass") /// c.Properties.Remove("PropThatIDontWantOnThisClass") ///
////// ///Adds a new ///with the specified value. /// /// The name of the new property. /// The value of the property (cannot be null). ///Adds a new ///with the specified value. The value cannot /// be null and must be convertable to a CIM type. /// public virtual void Add(string propertyName, Object propertyValue) { if (null == propertyValue) throw new ArgumentNullException("propertyValue"); if (parent.GetType() == typeof(ManagementObject)) //can't add properties to instance throw new InvalidOperationException(); CimType cimType = 0; bool isArray = false; object wmiValue = PropertyData.MapValueToWmiValue(propertyValue, out isArray, out cimType); int wmiCimType = (int)cimType; if (isArray) wmiCimType |= (int)tag_CIMTYPE_ENUMERATION.CIM_FLAG_ARRAY; int status = parent.wbemObject.Put_(propertyName, 0, ref wmiValue, wmiCimType); if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } } ///Properties can only be added to class definitions, not /// to instances. This method is only valid when invoked on a ////// in /// a . /// /// The name of the property. /// The value of the property (which can be null). /// The CIM type of the property. ///Adds a new ///with the specified value and CIM type. /// public void Add(string propertyName, Object propertyValue, CimType propertyType) { if (null == propertyName) throw new ArgumentNullException("propertyName"); if (parent.GetType() == typeof(ManagementObject)) //can't add properties to instance throw new InvalidOperationException(); int wmiCimType = (int)propertyType; bool isArray = false; if ((null != propertyValue) && propertyValue.GetType().IsArray) { isArray = true; wmiCimType = (wmiCimType | (int)tag_CIMTYPE_ENUMERATION.CIM_FLAG_ARRAY); } object wmiValue = PropertyData.MapValueToWmiValue(propertyValue, propertyType, isArray); int status = parent.wbemObject.Put_(propertyName, 0, ref wmiValue, wmiCimType); if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } } ///Properties can only be added to class definitions, not /// to instances. This method is only valid when invoked on a ////// in /// a . /// /// The name of the property. /// The CIM type of the property. ///Adds a new ///with no assigned value. to specify that the property is an array type; otherwise, . /// /// public void Add(string propertyName, CimType propertyType, bool isArray) { if (null == propertyName) throw new ArgumentNullException(propertyName); if (parent.GetType() == typeof(ManagementObject)) //can't add properties to instance throw new InvalidOperationException(); int wmiCimType = (int)propertyType; if (isArray) wmiCimType = (wmiCimType | (int)tag_CIMTYPE_ENUMERATION.CIM_FLAG_ARRAY); object dummyObj = System.DBNull.Value; int status = parent.wbemObject.Put_(propertyName, 0, ref dummyObj, wmiCimType); if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } } }//PropertyDataCollection } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System.Collections; using System.Diagnostics; using System.Runtime.InteropServices; using WbemClient_v1; using System.ComponentModel; namespace System.Management { //CCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCC// ///Properties can only be added to class definitions, not /// to instances. This method is only valid when invoked on a ////// in /// a . /// ///Represents the set of properties of a WMI object. ////// //CCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCC// public class PropertyDataCollection : ICollection, IEnumerable { private ManagementBaseObject parent; bool isSystem; internal PropertyDataCollection(ManagementBaseObject parent, bool isSystem) : base() { this.parent = parent; this.isSystem = isSystem; } // //ICollection // ///using System; /// using System.Management; /// /// // This sample demonstrates how to enumerate properties /// // in a ManagementObject object. /// class Sample_PropertyDataCollection /// { /// public static int Main(string[] args) { /// ManagementObject disk = new ManagementObject("win32_logicaldisk.deviceid = \"c:\""); /// PropertyDataCollection diskProperties = disk.Properties; /// foreach (PropertyData diskProperty in diskProperties) { /// Console.WriteLine("Property = " + diskProperty.Name); /// } /// return 0; /// } /// } ///
///Imports System /// Imports System.Management /// /// ' This sample demonstrates how to enumerate properties /// ' in a ManagementObject object. /// Class Sample_PropertyDataCollection /// Overloads Public Shared Function Main(args() As String) As Integer /// Dim disk As New ManagementObject("win32_logicaldisk.deviceid=""c:""") /// Dim diskProperties As PropertyDataCollection = disk.Properties /// Dim diskProperty As PropertyData /// For Each diskProperty In diskProperties /// Console.WriteLine("Property = " & diskProperty.Name) /// Next diskProperty /// Return 0 /// End Function /// End Class ///
////// ///Gets or sets the number of objects in the ///. /// public int Count { get { string[] propertyNames = null; object qualVal = null; int flag; if (isSystem) flag = (int)tag_WBEM_CONDITION_FLAG_TYPE.WBEM_FLAG_SYSTEM_ONLY; else flag = (int)tag_WBEM_CONDITION_FLAG_TYPE.WBEM_FLAG_NONSYSTEM_ONLY; flag |= (int)tag_WBEM_CONDITION_FLAG_TYPE.WBEM_FLAG_ALWAYS; int status = parent.wbemObject.GetNames_(null, flag, ref qualVal, out propertyNames); if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } return propertyNames.Length; } } ///The number of objects in the collection. ////// ///Gets or sets a value indicating whether the object is synchronized. ////// public bool IsSynchronized { get { return false; } } ////// if the object is synchronized; /// otherwise, . /// ///Gets or sets the object to be used for synchronization. ////// public object SyncRoot { get { return this; } } ///The object to be used for synchronization. ////// ///Copies the ///into an array. /// /// The array to which to copy theCopies the ///into an array. . /// The index from which to start copying. public void CopyTo(Array array, Int32 index) { if (null == array) throw new ArgumentNullException("array"); if ((index < array.GetLowerBound(0)) || (index > array.GetUpperBound(0))) throw new ArgumentOutOfRangeException("index"); // Get the names of the properties string[] nameArray = null; object dummy = null; int flag = 0; if (isSystem) flag |= (int)tag_WBEM_CONDITION_FLAG_TYPE.WBEM_FLAG_SYSTEM_ONLY; else flag |= (int)tag_WBEM_CONDITION_FLAG_TYPE.WBEM_FLAG_NONSYSTEM_ONLY; flag |= (int)tag_WBEM_CONDITION_FLAG_TYPE.WBEM_FLAG_ALWAYS; int status = this.parent.wbemObject.GetNames_(null, flag, ref dummy, out nameArray); if (status >= 0) { if ((index + nameArray.Length) > array.Length) throw new ArgumentException(null,"index"); foreach (string propertyName in nameArray) array.SetValue(new PropertyData(parent, propertyName), index++); } if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } return; } /// /// /// The destination array to contain the copiedCopies the ///to a specialized object /// array. . /// The index in the destination array from which to start copying. public void CopyTo(PropertyData[] propertyArray, Int32 index) { CopyTo((Array)propertyArray, index); } // // IEnumerable // IEnumerator IEnumerable.GetEnumerator() { return (IEnumerator)(new PropertyDataEnumerator(parent, isSystem)); } /// /// ///Returns the enumerator for this ///. /// public PropertyDataEnumerator GetEnumerator() { return new PropertyDataEnumerator(parent, isSystem); } //Enumerator class ///An ////// that can be used to iterate through the collection. /// ///Represents the enumerator for ////// objects in the . /// public class PropertyDataEnumerator : IEnumerator { private ManagementBaseObject parent; private string[] propertyNames; private int index; internal PropertyDataEnumerator(ManagementBaseObject parent, bool isSystem) { this.parent = parent; propertyNames = null; index = -1; int flag; object qualVal = null; if (isSystem) flag = (int)tag_WBEM_CONDITION_FLAG_TYPE.WBEM_FLAG_SYSTEM_ONLY; else flag = (int)tag_WBEM_CONDITION_FLAG_TYPE.WBEM_FLAG_NONSYSTEM_ONLY; flag |= (int)tag_WBEM_CONDITION_FLAG_TYPE.WBEM_FLAG_ALWAYS; int status = parent.wbemObject.GetNames_(null, flag, ref qualVal, out propertyNames); if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } } ///using System; /// using System.Management; /// /// // This sample demonstrates how to enumerate all properties in a /// // ManagementObject using the PropertyDataEnumerator object. /// class Sample_PropertyDataEnumerator /// { /// public static int Main(string[] args) { /// ManagementObject disk = new ManagementObject("Win32_LogicalDisk.DeviceID='C:'"); /// PropertyDataCollection.PropertyDataEnumerator propertyEnumerator = disk.Properties.GetEnumerator(); /// while(propertyEnumerator.MoveNext()) { /// PropertyData p = (PropertyData)propertyEnumerator.Current; /// Console.WriteLine("Property found: " + p.Name); /// } /// return 0; /// } /// } ///
///Imports System /// Imports System.Management /// /// ' This sample demonstrates how to enumerate all properties in a /// ' ManagementObject using PropertyDataEnumerator object. /// Class Sample_PropertyDataEnumerator /// Overloads Public Shared Function Main(args() As String) As Integer /// Dim disk As New ManagementObject("Win32_LogicalDisk.DeviceID='C:'") /// Dim propertyEnumerator As _ /// PropertyDataCollection.PropertyDataEnumerator = disk.Properties.GetEnumerator() /// While propertyEnumerator.MoveNext() /// Dim p As PropertyData = _ /// CType(propertyEnumerator.Current, PropertyData) /// Console.WriteLine("Property found: " & p.Name) /// End While /// Return 0 /// End Function /// End Class ///
///object IEnumerator.Current { get { return (object)this.Current; } } /// /// ///Gets the current ///in the enumeration. /// The current public PropertyData Current { get { if ((index == -1) || (index == propertyNames.Length)) throw new InvalidOperationException(); else return new PropertyData(parent, propertyNames[index]); } } ////// element in the collection. /// /// ///Moves to the next element in the ////// enumeration. /// public bool MoveNext() { if (index == propertyNames.Length) //passed the end of the array return false; //don't advance the index any more index++; return (index == propertyNames.Length) ? false : true; } ////// if the enumerator was successfully advanced to the next element; /// if the enumerator has passed the end of the collection. /// public void Reset() { index = -1; } }//PropertyDataEnumerator // // Methods // ///Resets the enumerator to the beginning of the ////// enumeration. /// /// The name of the property to retrieve. ///Returns the specified property from the ///, using [] syntax. /// ///A ///, based on /// the name specified. /// public virtual PropertyData this[string propertyName] { get { if (null == propertyName) throw new ArgumentNullException("propertyName"); return new PropertyData(parent, propertyName); } } ///ManagementObject o = new ManagementObject("Win32_LogicalDisk.Name = 'C:'"); /// Console.WriteLine("Free space on C: drive is: ", c.Properties["FreeSpace"].Value); ///
///Dim o As New ManagementObject("Win32_LogicalDisk.Name=""C:""") /// Console.WriteLine("Free space on C: drive is: " & c.Properties("FreeSpace").Value) ///
////// /// The name of the property to be removed. ///Removes a ///from the . /// ///Properties can only be removed from class definitions, /// not from instances. This method is only valid when invoked on a property /// collection in a ///. /// public virtual void Remove(string propertyName) { // On instances, reset the property to the default value for the class. if (parent.GetType() == typeof(ManagementObject)) { ManagementClass cls = new ManagementClass(parent.ClassPath); parent.SetPropertyValue(propertyName, cls.GetPropertyValue(propertyName)); } else { int status = parent.wbemObject.Delete_(propertyName); if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } } } ///ManagementClass c = new ManagementClass("MyClass"); /// c.Properties.Remove("PropThatIDontWantOnThisClass"); ///
///Dim c As New ManagementClass("MyClass") /// c.Properties.Remove("PropThatIDontWantOnThisClass") ///
////// ///Adds a new ///with the specified value. /// /// The name of the new property. /// The value of the property (cannot be null). ///Adds a new ///with the specified value. The value cannot /// be null and must be convertable to a CIM type. /// public virtual void Add(string propertyName, Object propertyValue) { if (null == propertyValue) throw new ArgumentNullException("propertyValue"); if (parent.GetType() == typeof(ManagementObject)) //can't add properties to instance throw new InvalidOperationException(); CimType cimType = 0; bool isArray = false; object wmiValue = PropertyData.MapValueToWmiValue(propertyValue, out isArray, out cimType); int wmiCimType = (int)cimType; if (isArray) wmiCimType |= (int)tag_CIMTYPE_ENUMERATION.CIM_FLAG_ARRAY; int status = parent.wbemObject.Put_(propertyName, 0, ref wmiValue, wmiCimType); if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } } ///Properties can only be added to class definitions, not /// to instances. This method is only valid when invoked on a ////// in /// a . /// /// The name of the property. /// The value of the property (which can be null). /// The CIM type of the property. ///Adds a new ///with the specified value and CIM type. /// public void Add(string propertyName, Object propertyValue, CimType propertyType) { if (null == propertyName) throw new ArgumentNullException("propertyName"); if (parent.GetType() == typeof(ManagementObject)) //can't add properties to instance throw new InvalidOperationException(); int wmiCimType = (int)propertyType; bool isArray = false; if ((null != propertyValue) && propertyValue.GetType().IsArray) { isArray = true; wmiCimType = (wmiCimType | (int)tag_CIMTYPE_ENUMERATION.CIM_FLAG_ARRAY); } object wmiValue = PropertyData.MapValueToWmiValue(propertyValue, propertyType, isArray); int status = parent.wbemObject.Put_(propertyName, 0, ref wmiValue, wmiCimType); if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } } ///Properties can only be added to class definitions, not /// to instances. This method is only valid when invoked on a ////// in /// a . /// /// The name of the property. /// The CIM type of the property. ///Adds a new ///with no assigned value. to specify that the property is an array type; otherwise, . /// /// public void Add(string propertyName, CimType propertyType, bool isArray) { if (null == propertyName) throw new ArgumentNullException(propertyName); if (parent.GetType() == typeof(ManagementObject)) //can't add properties to instance throw new InvalidOperationException(); int wmiCimType = (int)propertyType; if (isArray) wmiCimType = (wmiCimType | (int)tag_CIMTYPE_ENUMERATION.CIM_FLAG_ARRAY); object dummyObj = System.DBNull.Value; int status = parent.wbemObject.Put_(propertyName, 0, ref dummyObj, wmiCimType); if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } } }//PropertyDataCollection } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.Properties can only be added to class definitions, not /// to instances. This method is only valid when invoked on a ////// in /// a .
Link Menu
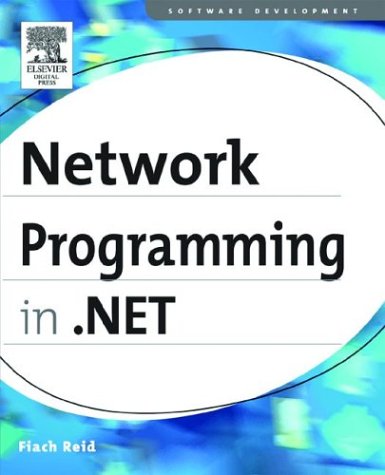
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlAttributeAttribute.cs
- UIAgentRequest.cs
- MatrixAnimationUsingPath.cs
- SystemResourceKey.cs
- ResourceReader.cs
- OdbcEnvironmentHandle.cs
- HtmlSelect.cs
- CollectionContainer.cs
- QueryExpr.cs
- ToolStripContainer.cs
- SessionStateSection.cs
- CharacterBuffer.cs
- Int16.cs
- RequiredFieldValidator.cs
- DataGridViewTopLeftHeaderCell.cs
- ValidatorCollection.cs
- CredentialCache.cs
- ImageMapEventArgs.cs
- CheckBoxField.cs
- MatchingStyle.cs
- DiscardableAttribute.cs
- BaseInfoTable.cs
- FormViewDeleteEventArgs.cs
- Mappings.cs
- Color.cs
- PasswordRecovery.cs
- MimeMapping.cs
- XamlFrame.cs
- CacheMode.cs
- EmbossBitmapEffect.cs
- SQLInt16.cs
- ServicePoint.cs
- CompositeDuplexBindingElement.cs
- EncryptedKey.cs
- TypedReference.cs
- HierarchicalDataSourceControl.cs
- ClassValidator.cs
- ParameterElement.cs
- ImageMapEventArgs.cs
- AuthenticateEventArgs.cs
- FlowLayoutPanel.cs
- BindingContext.cs
- FormViewCommandEventArgs.cs
- PrimitiveXmlSerializers.cs
- Bezier.cs
- SpnegoTokenProvider.cs
- UriScheme.cs
- SymbolDocumentGenerator.cs
- ExpressionWriter.cs
- ProfileModule.cs
- SecurityListenerSettingsLifetimeManager.cs
- XmlLanguage.cs
- PnrpPermission.cs
- SoapSchemaExporter.cs
- MergeFilterQuery.cs
- XPathExpr.cs
- MaxMessageSizeStream.cs
- SortQuery.cs
- ToolStripRenderer.cs
- FileDialogPermission.cs
- Transform3D.cs
- SystemIcmpV4Statistics.cs
- XmlNullResolver.cs
- X509ServiceCertificateAuthentication.cs
- SspiWrapper.cs
- CodeConditionStatement.cs
- InkCanvasAutomationPeer.cs
- CmsInterop.cs
- _ListenerRequestStream.cs
- RemoteWebConfigurationHost.cs
- Sequence.cs
- HMACRIPEMD160.cs
- ContextItemManager.cs
- BuildManager.cs
- SecurityTokenValidationException.cs
- EntityParameter.cs
- StorageEntityContainerMapping.cs
- NavigationProgressEventArgs.cs
- MaterialCollection.cs
- WrappedReader.cs
- UTF8Encoding.cs
- ConstraintStruct.cs
- DaylightTime.cs
- BitmapCodecInfo.cs
- HttpInputStream.cs
- VirtualDirectoryMappingCollection.cs
- SessionState.cs
- NavigationPropertyEmitter.cs
- SchemaElementDecl.cs
- BufferBuilder.cs
- RootAction.cs
- GeneralTransform3DGroup.cs
- EnumUnknown.cs
- TemplateComponentConnector.cs
- NullableDecimalSumAggregationOperator.cs
- DataSourceControl.cs
- IndicShape.cs
- ImageListStreamer.cs
- CellRelation.cs
- TypeDescriptor.cs