Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / Routing / RouteValueDictionary.cs / 1305376 / RouteValueDictionary.cs
namespace System.Web.Routing { using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Runtime.CompilerServices; [TypeForwardedFrom("System.Web.Routing, Version=3.5.0.0, Culture=Neutral, PublicKeyToken=31bf3856ad364e35")] public class RouteValueDictionary : IDictionary{ private Dictionary _dictionary; public RouteValueDictionary() { _dictionary = new Dictionary (StringComparer.OrdinalIgnoreCase); } public RouteValueDictionary(object values) { _dictionary = new Dictionary (StringComparer.OrdinalIgnoreCase); AddValues(values); } public RouteValueDictionary(IDictionary dictionary) { _dictionary = new Dictionary (dictionary, StringComparer.OrdinalIgnoreCase); } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public int Count { get { return _dictionary.Count; } } public Dictionary .KeyCollection Keys { get { return _dictionary.Keys; } } public Dictionary .ValueCollection Values { get { return _dictionary.Values; } } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public object this[string key] { get { object value; TryGetValue(key, out value); return value; } set { _dictionary[key] = value; } } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public void Add(string key, object value) { _dictionary.Add(key, value); } private void AddValues(object values) { if (values != null) { PropertyDescriptorCollection props = TypeDescriptor.GetProperties(values); foreach (PropertyDescriptor prop in props) { object val = prop.GetValue(values); Add(prop.Name, val); } } } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public void Clear() { _dictionary.Clear(); } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public bool ContainsKey(string key) { return _dictionary.ContainsKey(key); } public bool ContainsValue(object value) { return _dictionary.ContainsValue(value); } public Dictionary .Enumerator GetEnumerator() { return _dictionary.GetEnumerator(); } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public bool Remove(string key) { return _dictionary.Remove(key); } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public bool TryGetValue(string key, out object value) { return _dictionary.TryGetValue(key, out value); } #region IDictionary Members [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] ICollection IDictionary .Keys { get { return _dictionary.Keys; } } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] ICollection
Link Menu
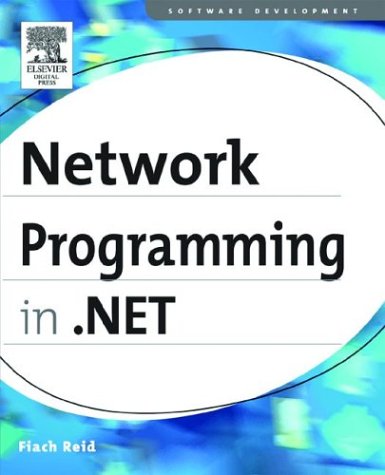
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConnectionStringsExpressionBuilder.cs
- RangeBaseAutomationPeer.cs
- XmlNodeChangedEventManager.cs
- FamilyCollection.cs
- HttpRequestWrapper.cs
- ClrPerspective.cs
- RegexGroupCollection.cs
- SqlDataSourceStatusEventArgs.cs
- EntityReference.cs
- XamlWriterExtensions.cs
- PackageRelationshipCollection.cs
- DiagnosticTraceSource.cs
- URLIdentityPermission.cs
- ViewStateChangedEventArgs.cs
- UriSchemeKeyedCollection.cs
- FormViewRow.cs
- LinearGradientBrush.cs
- WebControl.cs
- WsiProfilesElementCollection.cs
- XmlUtil.cs
- NativeCompoundFileAPIs.cs
- MailSettingsSection.cs
- Binding.cs
- DrawingAttributes.cs
- Nullable.cs
- FontStyleConverter.cs
- SimpleMailWebEventProvider.cs
- CreateUserErrorEventArgs.cs
- BlockCollection.cs
- HttpHeaderCollection.cs
- URLAttribute.cs
- ProviderConnectionPoint.cs
- DocumentViewerBaseAutomationPeer.cs
- brushes.cs
- DesignTimeParseData.cs
- MembershipUser.cs
- EncoderParameters.cs
- TdsParserStaticMethods.cs
- WebPartHeaderCloseVerb.cs
- SystemWebCachingSectionGroup.cs
- arabicshape.cs
- BaseTemplateParser.cs
- FileSystemInfo.cs
- RuleElement.cs
- MethodRental.cs
- MessageParameterAttribute.cs
- Help.cs
- QuaternionIndependentAnimationStorage.cs
- HybridObjectCache.cs
- PointAnimationUsingPath.cs
- MaxMessageSizeStream.cs
- Thread.cs
- TagPrefixAttribute.cs
- ConversionContext.cs
- TimeSpanConverter.cs
- TableHeaderCell.cs
- RefreshEventArgs.cs
- ContextStack.cs
- SafeMemoryMappedFileHandle.cs
- XmlAnyElementAttributes.cs
- FigureParaClient.cs
- TypeDelegator.cs
- LeftCellWrapper.cs
- HttpResponseHeader.cs
- ResourceDisplayNameAttribute.cs
- HtmlProps.cs
- TokenBasedSetEnumerator.cs
- MasterPageBuildProvider.cs
- GeometryGroup.cs
- AnnouncementInnerClientCD1.cs
- SortQuery.cs
- RestHandlerFactory.cs
- ThreadSafeList.cs
- GestureRecognizer.cs
- LinqDataSourceDisposeEventArgs.cs
- XmlSchemaChoice.cs
- RequestCache.cs
- CheckBoxStandardAdapter.cs
- OleDbConnectionFactory.cs
- ElementsClipboardData.cs
- TypeInitializationException.cs
- ProcessModuleCollection.cs
- IPHostEntry.cs
- SqlConnectionString.cs
- HtmlEmptyTagControlBuilder.cs
- login.cs
- OfTypeExpression.cs
- TreeViewCancelEvent.cs
- DataRecordInternal.cs
- EmbeddedMailObject.cs
- DataSourceSelectArguments.cs
- KeyedHashAlgorithm.cs
- ImageConverter.cs
- Domain.cs
- XmlWrappingReader.cs
- TargetFrameworkUtil.cs
- ArglessEventHandlerProxy.cs
- RemotingAttributes.cs
- TableFieldsEditor.cs
- EndpointDispatcher.cs