Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / MS / Internal / IO / Zip / WriteTimeStream.cs / 1305600 / WriteTimeStream.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // WriteTimeStream - wraps the ArchiveStream in Streaming generation scenarios so that we // can determine current archive stream offset even when working on a stream that is non-seekable // because the Position property is unusable on such streams. // // History: // 03/25/2002: BruceMac: Created. // //----------------------------------------------------------------------------- using System; using System.IO; using System.Windows; using MS.Internal.WindowsBase; namespace MS.Internal.IO.Zip { internal class WriteTimeStream : Stream { //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- ////// CanRead - never /// override public bool CanRead { get { return false; } } ////// CanSeek - never /// override public bool CanSeek{ get { return false; } } ////// CanWrite - only if we are not disposed /// override public bool CanWrite { get { return (_baseStream != null); } } ////// Same as Position /// override public long Length { get { CheckDisposed(); return _position; } } ////// Get is supported even on Write-only stream /// override public long Position { get { CheckDisposed(); return _position; } set { CheckDisposed(); IllegalAccess(); // throw exception } } //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- public override void SetLength(long newLength) { IllegalAccess(); // throw exception } override public long Seek(long offset, SeekOrigin origin) { IllegalAccess(); // throw exception return -1; // keep compiler happy } override public int Read(byte[] buffer, int offset, int count) { IllegalAccess(); // throw exception return -1; // keep compiler happy } override public void Write(byte[] buffer, int offset, int count) { CheckDisposed(); _baseStream.Write(buffer, offset, count); checked{_position += count;} } override public void Flush() { CheckDisposed(); _baseStream.Flush(); } //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ internal WriteTimeStream(Stream baseStream) { if (baseStream == null) { throw new ArgumentNullException("baseStream"); } _baseStream = baseStream; // must be based on writable stream if (!_baseStream.CanWrite) throw new ArgumentException(SR.Get(SRID.WriteNotSupported), "baseStream"); } //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ protected override void Dispose(bool disposing) { try { if (disposing && (_baseStream != null)) { _baseStream.Close(); } } finally { _baseStream = null; base.Dispose(disposing); } } //----------------------------------------------------- // // Private Methods // //----------------------------------------------------- private static void IllegalAccess() { throw new NotSupportedException(SR.Get(SRID.WriteOnlyStream)); } private void CheckDisposed() { if (_baseStream == null) throw new ObjectDisposedException("Stream"); } // _baseStream doubles as our disposed indicator - it's null if we are disposed private Stream _baseStream; // stream we wrap - needs to only support Write private long _position; // current position } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // WriteTimeStream - wraps the ArchiveStream in Streaming generation scenarios so that we // can determine current archive stream offset even when working on a stream that is non-seekable // because the Position property is unusable on such streams. // // History: // 03/25/2002: BruceMac: Created. // //----------------------------------------------------------------------------- using System; using System.IO; using System.Windows; using MS.Internal.WindowsBase; namespace MS.Internal.IO.Zip { internal class WriteTimeStream : Stream { //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- ////// CanRead - never /// override public bool CanRead { get { return false; } } ////// CanSeek - never /// override public bool CanSeek{ get { return false; } } ////// CanWrite - only if we are not disposed /// override public bool CanWrite { get { return (_baseStream != null); } } ////// Same as Position /// override public long Length { get { CheckDisposed(); return _position; } } ////// Get is supported even on Write-only stream /// override public long Position { get { CheckDisposed(); return _position; } set { CheckDisposed(); IllegalAccess(); // throw exception } } //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- public override void SetLength(long newLength) { IllegalAccess(); // throw exception } override public long Seek(long offset, SeekOrigin origin) { IllegalAccess(); // throw exception return -1; // keep compiler happy } override public int Read(byte[] buffer, int offset, int count) { IllegalAccess(); // throw exception return -1; // keep compiler happy } override public void Write(byte[] buffer, int offset, int count) { CheckDisposed(); _baseStream.Write(buffer, offset, count); checked{_position += count;} } override public void Flush() { CheckDisposed(); _baseStream.Flush(); } //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ internal WriteTimeStream(Stream baseStream) { if (baseStream == null) { throw new ArgumentNullException("baseStream"); } _baseStream = baseStream; // must be based on writable stream if (!_baseStream.CanWrite) throw new ArgumentException(SR.Get(SRID.WriteNotSupported), "baseStream"); } //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ protected override void Dispose(bool disposing) { try { if (disposing && (_baseStream != null)) { _baseStream.Close(); } } finally { _baseStream = null; base.Dispose(disposing); } } //----------------------------------------------------- // // Private Methods // //----------------------------------------------------- private static void IllegalAccess() { throw new NotSupportedException(SR.Get(SRID.WriteOnlyStream)); } private void CheckDisposed() { if (_baseStream == null) throw new ObjectDisposedException("Stream"); } // _baseStream doubles as our disposed indicator - it's null if we are disposed private Stream _baseStream; // stream we wrap - needs to only support Write private long _position; // current position } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
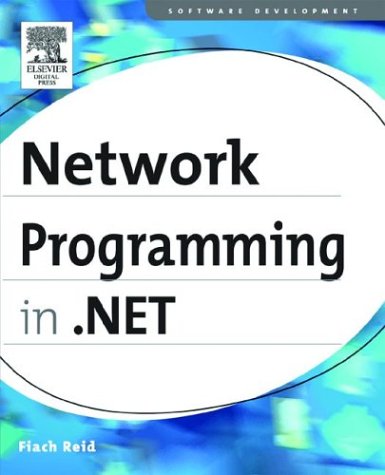
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BitmapSource.cs
- ConnectionInterfaceCollection.cs
- DetailsViewInsertEventArgs.cs
- TreeNodeSelectionProcessor.cs
- HttpRawResponse.cs
- OleDbDataAdapter.cs
- CachedFontFamily.cs
- DeferredRunTextReference.cs
- XmlQueryCardinality.cs
- DropShadowBitmapEffect.cs
- LinkButton.cs
- DataObjectAttribute.cs
- KeyFrames.cs
- ListItemParagraph.cs
- SchemaElementDecl.cs
- StrokeSerializer.cs
- SqlEnums.cs
- SettingsAttributes.cs
- ListViewGroup.cs
- StateMachineWorkflowInstance.cs
- WebResponse.cs
- DockPanel.cs
- XmlLanguage.cs
- Rotation3DAnimationBase.cs
- BooleanConverter.cs
- ListViewCommandEventArgs.cs
- ThicknessAnimation.cs
- NewExpression.cs
- DataGridPageChangedEventArgs.cs
- MinMaxParagraphWidth.cs
- GlobalItem.cs
- Evaluator.cs
- ConfigXmlReader.cs
- ButtonFlatAdapter.cs
- SQLUtility.cs
- CompoundFileDeflateTransform.cs
- oledbconnectionstring.cs
- TypeResolver.cs
- PermissionAttributes.cs
- WindowsGraphicsWrapper.cs
- CqlParserHelpers.cs
- XPathNodeInfoAtom.cs
- ButtonBase.cs
- PathSegment.cs
- DataGridViewDataErrorEventArgs.cs
- ProfileWorkflowElement.cs
- DBDataPermission.cs
- WorkerRequest.cs
- XmlDataProvider.cs
- WindowsToolbar.cs
- EditingMode.cs
- XhtmlTextWriter.cs
- WebServiceHandler.cs
- DecimalMinMaxAggregationOperator.cs
- UpdateManifestForBrowserApplication.cs
- DockPattern.cs
- XmlTextReader.cs
- PhysicalAddress.cs
- DesignerActionUIStateChangeEventArgs.cs
- RSAOAEPKeyExchangeFormatter.cs
- ContractMapping.cs
- DbProviderSpecificTypePropertyAttribute.cs
- AspNetSynchronizationContext.cs
- EntityCommandExecutionException.cs
- EventKeyword.cs
- DelegatedStream.cs
- WebPartMenu.cs
- WorkflowInstanceProvider.cs
- QilGeneratorEnv.cs
- ResourceWriter.cs
- Native.cs
- DetailsViewDeleteEventArgs.cs
- Int32KeyFrameCollection.cs
- QueryStringConverter.cs
- SineEase.cs
- CollectionViewGroup.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- Button.cs
- ServiceMemoryGates.cs
- WindowsEditBox.cs
- TransactionInterop.cs
- HttpCapabilitiesEvaluator.cs
- ArraySubsetEnumerator.cs
- Crypto.cs
- EpmSyndicationContentSerializer.cs
- X509SecurityTokenProvider.cs
- UnicodeEncoding.cs
- HyperLinkField.cs
- RepeaterItemCollection.cs
- EncryptedKeyIdentifierClause.cs
- RecordsAffectedEventArgs.cs
- DataGridTableCollection.cs
- StyleXamlParser.cs
- SoapExtensionStream.cs
- SqlDataSourceParameterParser.cs
- TemplateLookupAction.cs
- ContentFilePart.cs
- XslAstAnalyzer.cs
- VarRemapper.cs
- InputScope.cs