Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media3D / Generated / Model3DCollection.cs / 1305600 / Model3DCollection.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Text; using System.Windows.Markup; using System.Windows.Media.Media3D.Converters; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Windows.Media.Imaging; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Media3D { ////// A collection of Model3D objects. /// public sealed partial class Model3DCollection : Animatable, IList, IList{ //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods /// /// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new Model3DCollection Clone() { return (Model3DCollection)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new Model3DCollection CloneCurrentValue() { return (Model3DCollection)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region IList/// /// Adds "value" to the list /// public void Add(Model3D value) { AddHelper(value); } ////// Removes all elements from the list /// public void Clear() { WritePreamble(); // As part of Clear()'ing the collection, we will iterate it and call // OnFreezablePropertyChanged and OnRemove for each item. // However, OnRemove assumes that the item to be removed has already been // pulled from the underlying collection. To statisfy this condition, // we store the old collection and clear _collection before we call these methods. // As Clear() semantics do not include TrimToFit behavior, we create the new // collection storage at the same size as the previous. This is to provide // as close as possible the same perf characteristics as less complicated collections. FrugalStructListoldCollection = _collection; _collection = new FrugalStructList (_collection.Capacity); for (int i = oldCollection.Count - 1; i >= 0; i--) { OnFreezablePropertyChanged(/* oldValue = */ oldCollection[i], /* newValue = */ null); // Fire the OnRemove handlers for each item. We're not ensuring that // all OnRemove's get called if a resumable exception is thrown. // At this time, these call-outs are not public, so we do not handle exceptions. OnRemove( /* oldValue */ oldCollection[i]); } ++_version; WritePostscript(); } /// /// Determines if the list contains "value" /// public bool Contains(Model3D value) { ReadPreamble(); return _collection.Contains(value); } ////// Returns the index of "value" in the list /// public int IndexOf(Model3D value) { ReadPreamble(); return _collection.IndexOf(value); } ////// Inserts "value" into the list at the specified position /// public void Insert(int index, Model3D value) { if (value == null) { throw new System.ArgumentException(SR.Get(SRID.Collection_NoNull)); } WritePreamble(); OnFreezablePropertyChanged(/* oldValue = */ null, /* newValue = */ value); _collection.Insert(index, value); OnInsert(value); ++_version; WritePostscript(); } ////// Removes "value" from the list /// public bool Remove(Model3D value) { WritePreamble(); // By design collections "succeed silently" if you attempt to remove an item // not in the collection. Therefore we need to first verify the old value exists // before calling OnFreezablePropertyChanged. Since we already need to locate // the item in the collection we keep the index and use RemoveAt(...) to do // the work. (Windows OS #1016178) // We use the public IndexOf to guard our UIContext since OnFreezablePropertyChanged // is only called conditionally. IList.IndexOf returns -1 if the value is not found. int index = IndexOf(value); if (index >= 0) { Model3D oldValue = _collection[index]; OnFreezablePropertyChanged(oldValue, null); _collection.RemoveAt(index); OnRemove(oldValue); ++_version; WritePostscript(); return true; } // Collection_Remove returns true, calls WritePostscript, // increments version, and does UpdateResource if it succeeds return false; } ////// Removes the element at the specified index /// public void RemoveAt(int index) { RemoveAtWithoutFiringPublicEvents(index); // RemoveAtWithoutFiringPublicEvents incremented the version WritePostscript(); } ////// Removes the element at the specified index without firing /// the public Changed event. /// The caller - typically a public method - is responsible for calling /// WritePostscript if appropriate. /// internal void RemoveAtWithoutFiringPublicEvents(int index) { WritePreamble(); Model3D oldValue = _collection[ index ]; OnFreezablePropertyChanged(oldValue, null); _collection.RemoveAt(index); OnRemove(oldValue); ++_version; // No WritePostScript to avoid firing the Changed event. } ////// Indexer for the collection /// public Model3D this[int index] { get { ReadPreamble(); return _collection[index]; } set { if (value == null) { throw new System.ArgumentException(SR.Get(SRID.Collection_NoNull)); } WritePreamble(); if (!Object.ReferenceEquals(_collection[ index ], value)) { Model3D oldValue = _collection[ index ]; OnFreezablePropertyChanged(oldValue, value); _collection[ index ] = value; OnSet(oldValue, value); } ++_version; WritePostscript(); } } #endregion #region ICollection/// /// The number of elements contained in the collection. /// public int Count { get { ReadPreamble(); return _collection.Count; } } ////// Copies the elements of the collection into "array" starting at "index" /// public void CopyTo(Model3D[] array, int index) { ReadPreamble(); if (array == null) { throw new ArgumentNullException("array"); } // This will not throw in the case that we are copying // from an empty collection. This is consistent with the // BCL Collection implementations. (Windows 1587365) if (index < 0 || (index + _collection.Count) > array.Length) { throw new ArgumentOutOfRangeException("index"); } _collection.CopyTo(array, index); } bool ICollection.IsReadOnly { get { ReadPreamble(); return IsFrozen; } } #endregion #region IEnumerable /// /// Returns an enumerator for the collection /// public Enumerator GetEnumerator() { ReadPreamble(); return new Enumerator(this); } IEnumeratorIEnumerable .GetEnumerator() { return this.GetEnumerator(); } #endregion #region IList bool IList.IsReadOnly { get { return ((ICollection )this).IsReadOnly; } } bool IList.IsFixedSize { get { ReadPreamble(); return IsFrozen; } } object IList.this[int index] { get { return this[index]; } set { // Forwards to typed implementation this[index] = Cast(value); } } int IList.Add(object value) { // Forward to typed helper return AddHelper(Cast(value)); } bool IList.Contains(object value) { return Contains(value as Model3D); } int IList.IndexOf(object value) { return IndexOf(value as Model3D); } void IList.Insert(int index, object value) { // Forward to IList Insert Insert(index, Cast(value)); } void IList.Remove(object value) { Remove(value as Model3D); } #endregion #region ICollection void ICollection.CopyTo(Array array, int index) { ReadPreamble(); if (array == null) { throw new ArgumentNullException("array"); } // This will not throw in the case that we are copying // from an empty collection. This is consistent with the // BCL Collection implementations. (Windows 1587365) if (index < 0 || (index + _collection.Count) > array.Length) { throw new ArgumentOutOfRangeException("index"); } if (array.Rank != 1) { throw new ArgumentException(SR.Get(SRID.Collection_BadRank)); } // Elsewhere in the collection we throw an AE when the type is // bad so we do it here as well to be consistent try { int count = _collection.Count; for (int i = 0; i < count; i++) { array.SetValue(_collection[i], index + i); } } catch (InvalidCastException e) { throw new ArgumentException(SR.Get(SRID.Collection_BadDestArray, this.GetType().Name), e); } } bool ICollection.IsSynchronized { get { ReadPreamble(); return IsFrozen || Dispatcher != null; } } object ICollection.SyncRoot { get { ReadPreamble(); return this; } } #endregion #region IEnumerable IEnumerator IEnumerable.GetEnumerator() { return this.GetEnumerator(); } #endregion #region Internal Helpers /// /// A frozen empty Model3DCollection. /// internal static Model3DCollection Empty { get { if (s_empty == null) { Model3DCollection collection = new Model3DCollection(); collection.Freeze(); s_empty = collection; } return s_empty; } } ////// Helper to return read only access. /// internal Model3D Internal_GetItem(int i) { return _collection[i]; } ////// Freezable collections need to notify their contained Freezables /// about the change in the InheritanceContext /// internal override void OnInheritanceContextChangedCore(EventArgs args) { base.OnInheritanceContextChangedCore(args); for (int i=0; i.Add does not. This // is called by both Adds and IList 's just ignores the // integer private int AddHelper(Model3D value) { int index = AddWithoutFiringPublicEvents(value); // AddAtWithoutFiringPublicEvents incremented the version WritePostscript(); return index; } internal int AddWithoutFiringPublicEvents(Model3D value) { int index = -1; if (value == null) { throw new System.ArgumentException(SR.Get(SRID.Collection_NoNull)); } WritePreamble(); Model3D newValue = value; OnFreezablePropertyChanged(/* oldValue = */ null, newValue); index = _collection.Add(newValue); OnInsert(newValue); ++_version; // No WritePostScript to avoid firing the Changed event. return index; } internal event ItemInsertedHandler ItemInserted; internal event ItemRemovedHandler ItemRemoved; private void OnInsert(object item) { if (ItemInserted != null) { ItemInserted(this, item); } } private void OnRemove(object oldValue) { if (ItemRemoved != null) { ItemRemoved(this, oldValue); } } private void OnSet(object oldValue, object newValue) { OnInsert(newValue); OnRemove(oldValue); } #endregion Private Helpers private static Model3DCollection s_empty; #region Public Properties #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods /// /// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new Model3DCollection(); } ////// Implementation of Freezable.CloneCore() /// protected override void CloneCore(Freezable source) { Model3DCollection sourceModel3DCollection = (Model3DCollection) source; base.CloneCore(source); int count = sourceModel3DCollection._collection.Count; _collection = new FrugalStructList(count); for (int i = 0; i < count; i++) { Model3D newValue = (Model3D) sourceModel3DCollection._collection[i].Clone(); OnFreezablePropertyChanged(/* oldValue = */ null, newValue); _collection.Add(newValue); OnInsert(newValue); } } /// /// Implementation of Freezable.CloneCurrentValueCore() /// protected override void CloneCurrentValueCore(Freezable source) { Model3DCollection sourceModel3DCollection = (Model3DCollection) source; base.CloneCurrentValueCore(source); int count = sourceModel3DCollection._collection.Count; _collection = new FrugalStructList(count); for (int i = 0; i < count; i++) { Model3D newValue = (Model3D) sourceModel3DCollection._collection[i].CloneCurrentValue(); OnFreezablePropertyChanged(/* oldValue = */ null, newValue); _collection.Add(newValue); OnInsert(newValue); } } /// /// Implementation of Freezable.GetAsFrozenCore() /// protected override void GetAsFrozenCore(Freezable source) { Model3DCollection sourceModel3DCollection = (Model3DCollection) source; base.GetAsFrozenCore(source); int count = sourceModel3DCollection._collection.Count; _collection = new FrugalStructList(count); for (int i = 0; i < count; i++) { Model3D newValue = (Model3D) sourceModel3DCollection._collection[i].GetAsFrozen(); OnFreezablePropertyChanged(/* oldValue = */ null, newValue); _collection.Add(newValue); OnInsert(newValue); } } /// /// Implementation of Freezable.GetCurrentValueAsFrozenCore() /// protected override void GetCurrentValueAsFrozenCore(Freezable source) { Model3DCollection sourceModel3DCollection = (Model3DCollection) source; base.GetCurrentValueAsFrozenCore(source); int count = sourceModel3DCollection._collection.Count; _collection = new FrugalStructList(count); for (int i = 0; i < count; i++) { Model3D newValue = (Model3D) sourceModel3DCollection._collection[i].GetCurrentValueAsFrozen(); OnFreezablePropertyChanged(/* oldValue = */ null, newValue); _collection.Add(newValue); OnInsert(newValue); } } /// /// Implementation of protected override bool FreezeCore(bool isChecking) { bool canFreeze = base.FreezeCore(isChecking); int count = _collection.Count; for (int i = 0; i < count && canFreeze; i++) { canFreeze &= Freezable.Freeze(_collection[i], isChecking); } return canFreeze; } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal FrugalStructListFreezable.FreezeCore . ///_collection; internal uint _version = 0; #endregion Internal Fields #region Enumerator /// /// Enumerates the items in a Model3DCollection /// public struct Enumerator : IEnumerator, IEnumerator{ #region Constructor internal Enumerator(Model3DCollection list) { Debug.Assert(list != null, "list may not be null."); _list = list; _version = list._version; _index = -1; _current = default(Model3D); } #endregion #region Methods void IDisposable.Dispose() { } /// /// Advances the enumerator to the next element of the collection. /// ////// true if the enumerator was successfully advanced to the next element, /// false if the enumerator has passed the end of the collection. /// public bool MoveNext() { _list.ReadPreamble(); if (_version == _list._version) { if (_index > -2 && _index < _list._collection.Count - 1) { _current = _list._collection[++_index]; return true; } else { _index = -2; // -2 indicates "past the end" return false; } } else { throw new InvalidOperationException(SR.Get(SRID.Enumerator_CollectionChanged)); } } ////// Sets the enumerator to its initial position, which is before the /// first element in the collection. /// public void Reset() { _list.ReadPreamble(); if (_version == _list._version) { _index = -1; } else { throw new InvalidOperationException(SR.Get(SRID.Enumerator_CollectionChanged)); } } #endregion #region Properties object IEnumerator.Current { get { return this.Current; } } ////// Current element /// /// The behavior of IEnumerable<T>.Current is undefined /// before the first MoveNext and after we have walked /// off the end of the list. However, the IEnumerable.Current /// contract requires that we throw exceptions /// public Model3D Current { get { if (_index > -1) { return _current; } else if (_index == -1) { throw new InvalidOperationException(SR.Get(SRID.Enumerator_NotStarted)); } else { Debug.Assert(_index == -2, "expected -2, got " + _index + "\n"); throw new InvalidOperationException(SR.Get(SRID.Enumerator_ReachedEnd)); } } } #endregion #region Data private Model3D _current; private Model3DCollection _list; private uint _version; private int _index; #endregion } #endregion #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- ////// Initializes a new instance that is empty. /// public Model3DCollection() { _collection = new FrugalStructList(); } /// /// Initializes a new instance that is empty and has the specified initial capacity. /// /// int - The number of elements that the new list is initially capable of storing. public Model3DCollection(int capacity) { _collection = new FrugalStructList(capacity); } /// /// Creates a Model3DCollection with all of the same elements as collection /// public Model3DCollection(IEnumerablecollection) { // The WritePreamble and WritePostscript aren't technically necessary // in the constructor as of 1/20/05 but they are put here in case // their behavior changes at a later date WritePreamble(); if (collection != null) { bool needsItemValidation = true; ICollection icollectionOfT = collection as ICollection ; if (icollectionOfT != null) { _collection = new FrugalStructList (icollectionOfT); } else { ICollection icollection = collection as ICollection; if (icollection != null) // an IC but not and IC { _collection = new FrugalStructList (icollection); } else // not a IC or IC so fall back to the slower Add { _collection = new FrugalStructList (); foreach (Model3D item in collection) { if (item == null) { throw new System.ArgumentException(SR.Get(SRID.Collection_NoNull)); } Model3D newValue = item; OnFreezablePropertyChanged(/* oldValue = */ null, newValue); _collection.Add(newValue); OnInsert(newValue); } needsItemValidation = false; } } if (needsItemValidation) { foreach (Model3D item in collection) { if (item == null) { throw new System.ArgumentException(SR.Get(SRID.Collection_NoNull)); } OnFreezablePropertyChanged(/* oldValue = */ null, item); OnInsert(item); } } WritePostscript(); } else { throw new ArgumentNullException("collection"); } } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Text; using System.Windows.Markup; using System.Windows.Media.Media3D.Converters; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Windows.Media.Imaging; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Media3D { ////// A collection of Model3D objects. /// public sealed partial class Model3DCollection : Animatable, IList, IList{ //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods /// /// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new Model3DCollection Clone() { return (Model3DCollection)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new Model3DCollection CloneCurrentValue() { return (Model3DCollection)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region IList/// /// Adds "value" to the list /// public void Add(Model3D value) { AddHelper(value); } ////// Removes all elements from the list /// public void Clear() { WritePreamble(); // As part of Clear()'ing the collection, we will iterate it and call // OnFreezablePropertyChanged and OnRemove for each item. // However, OnRemove assumes that the item to be removed has already been // pulled from the underlying collection. To statisfy this condition, // we store the old collection and clear _collection before we call these methods. // As Clear() semantics do not include TrimToFit behavior, we create the new // collection storage at the same size as the previous. This is to provide // as close as possible the same perf characteristics as less complicated collections. FrugalStructListoldCollection = _collection; _collection = new FrugalStructList (_collection.Capacity); for (int i = oldCollection.Count - 1; i >= 0; i--) { OnFreezablePropertyChanged(/* oldValue = */ oldCollection[i], /* newValue = */ null); // Fire the OnRemove handlers for each item. We're not ensuring that // all OnRemove's get called if a resumable exception is thrown. // At this time, these call-outs are not public, so we do not handle exceptions. OnRemove( /* oldValue */ oldCollection[i]); } ++_version; WritePostscript(); } /// /// Determines if the list contains "value" /// public bool Contains(Model3D value) { ReadPreamble(); return _collection.Contains(value); } ////// Returns the index of "value" in the list /// public int IndexOf(Model3D value) { ReadPreamble(); return _collection.IndexOf(value); } ////// Inserts "value" into the list at the specified position /// public void Insert(int index, Model3D value) { if (value == null) { throw new System.ArgumentException(SR.Get(SRID.Collection_NoNull)); } WritePreamble(); OnFreezablePropertyChanged(/* oldValue = */ null, /* newValue = */ value); _collection.Insert(index, value); OnInsert(value); ++_version; WritePostscript(); } ////// Removes "value" from the list /// public bool Remove(Model3D value) { WritePreamble(); // By design collections "succeed silently" if you attempt to remove an item // not in the collection. Therefore we need to first verify the old value exists // before calling OnFreezablePropertyChanged. Since we already need to locate // the item in the collection we keep the index and use RemoveAt(...) to do // the work. (Windows OS #1016178) // We use the public IndexOf to guard our UIContext since OnFreezablePropertyChanged // is only called conditionally. IList.IndexOf returns -1 if the value is not found. int index = IndexOf(value); if (index >= 0) { Model3D oldValue = _collection[index]; OnFreezablePropertyChanged(oldValue, null); _collection.RemoveAt(index); OnRemove(oldValue); ++_version; WritePostscript(); return true; } // Collection_Remove returns true, calls WritePostscript, // increments version, and does UpdateResource if it succeeds return false; } ////// Removes the element at the specified index /// public void RemoveAt(int index) { RemoveAtWithoutFiringPublicEvents(index); // RemoveAtWithoutFiringPublicEvents incremented the version WritePostscript(); } ////// Removes the element at the specified index without firing /// the public Changed event. /// The caller - typically a public method - is responsible for calling /// WritePostscript if appropriate. /// internal void RemoveAtWithoutFiringPublicEvents(int index) { WritePreamble(); Model3D oldValue = _collection[ index ]; OnFreezablePropertyChanged(oldValue, null); _collection.RemoveAt(index); OnRemove(oldValue); ++_version; // No WritePostScript to avoid firing the Changed event. } ////// Indexer for the collection /// public Model3D this[int index] { get { ReadPreamble(); return _collection[index]; } set { if (value == null) { throw new System.ArgumentException(SR.Get(SRID.Collection_NoNull)); } WritePreamble(); if (!Object.ReferenceEquals(_collection[ index ], value)) { Model3D oldValue = _collection[ index ]; OnFreezablePropertyChanged(oldValue, value); _collection[ index ] = value; OnSet(oldValue, value); } ++_version; WritePostscript(); } } #endregion #region ICollection/// /// The number of elements contained in the collection. /// public int Count { get { ReadPreamble(); return _collection.Count; } } ////// Copies the elements of the collection into "array" starting at "index" /// public void CopyTo(Model3D[] array, int index) { ReadPreamble(); if (array == null) { throw new ArgumentNullException("array"); } // This will not throw in the case that we are copying // from an empty collection. This is consistent with the // BCL Collection implementations. (Windows 1587365) if (index < 0 || (index + _collection.Count) > array.Length) { throw new ArgumentOutOfRangeException("index"); } _collection.CopyTo(array, index); } bool ICollection.IsReadOnly { get { ReadPreamble(); return IsFrozen; } } #endregion #region IEnumerable /// /// Returns an enumerator for the collection /// public Enumerator GetEnumerator() { ReadPreamble(); return new Enumerator(this); } IEnumeratorIEnumerable .GetEnumerator() { return this.GetEnumerator(); } #endregion #region IList bool IList.IsReadOnly { get { return ((ICollection )this).IsReadOnly; } } bool IList.IsFixedSize { get { ReadPreamble(); return IsFrozen; } } object IList.this[int index] { get { return this[index]; } set { // Forwards to typed implementation this[index] = Cast(value); } } int IList.Add(object value) { // Forward to typed helper return AddHelper(Cast(value)); } bool IList.Contains(object value) { return Contains(value as Model3D); } int IList.IndexOf(object value) { return IndexOf(value as Model3D); } void IList.Insert(int index, object value) { // Forward to IList Insert Insert(index, Cast(value)); } void IList.Remove(object value) { Remove(value as Model3D); } #endregion #region ICollection void ICollection.CopyTo(Array array, int index) { ReadPreamble(); if (array == null) { throw new ArgumentNullException("array"); } // This will not throw in the case that we are copying // from an empty collection. This is consistent with the // BCL Collection implementations. (Windows 1587365) if (index < 0 || (index + _collection.Count) > array.Length) { throw new ArgumentOutOfRangeException("index"); } if (array.Rank != 1) { throw new ArgumentException(SR.Get(SRID.Collection_BadRank)); } // Elsewhere in the collection we throw an AE when the type is // bad so we do it here as well to be consistent try { int count = _collection.Count; for (int i = 0; i < count; i++) { array.SetValue(_collection[i], index + i); } } catch (InvalidCastException e) { throw new ArgumentException(SR.Get(SRID.Collection_BadDestArray, this.GetType().Name), e); } } bool ICollection.IsSynchronized { get { ReadPreamble(); return IsFrozen || Dispatcher != null; } } object ICollection.SyncRoot { get { ReadPreamble(); return this; } } #endregion #region IEnumerable IEnumerator IEnumerable.GetEnumerator() { return this.GetEnumerator(); } #endregion #region Internal Helpers /// /// A frozen empty Model3DCollection. /// internal static Model3DCollection Empty { get { if (s_empty == null) { Model3DCollection collection = new Model3DCollection(); collection.Freeze(); s_empty = collection; } return s_empty; } } ////// Helper to return read only access. /// internal Model3D Internal_GetItem(int i) { return _collection[i]; } ////// Freezable collections need to notify their contained Freezables /// about the change in the InheritanceContext /// internal override void OnInheritanceContextChangedCore(EventArgs args) { base.OnInheritanceContextChangedCore(args); for (int i=0; i.Add does not. This // is called by both Adds and IList 's just ignores the // integer private int AddHelper(Model3D value) { int index = AddWithoutFiringPublicEvents(value); // AddAtWithoutFiringPublicEvents incremented the version WritePostscript(); return index; } internal int AddWithoutFiringPublicEvents(Model3D value) { int index = -1; if (value == null) { throw new System.ArgumentException(SR.Get(SRID.Collection_NoNull)); } WritePreamble(); Model3D newValue = value; OnFreezablePropertyChanged(/* oldValue = */ null, newValue); index = _collection.Add(newValue); OnInsert(newValue); ++_version; // No WritePostScript to avoid firing the Changed event. return index; } internal event ItemInsertedHandler ItemInserted; internal event ItemRemovedHandler ItemRemoved; private void OnInsert(object item) { if (ItemInserted != null) { ItemInserted(this, item); } } private void OnRemove(object oldValue) { if (ItemRemoved != null) { ItemRemoved(this, oldValue); } } private void OnSet(object oldValue, object newValue) { OnInsert(newValue); OnRemove(oldValue); } #endregion Private Helpers private static Model3DCollection s_empty; #region Public Properties #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods /// /// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new Model3DCollection(); } ////// Implementation of Freezable.CloneCore() /// protected override void CloneCore(Freezable source) { Model3DCollection sourceModel3DCollection = (Model3DCollection) source; base.CloneCore(source); int count = sourceModel3DCollection._collection.Count; _collection = new FrugalStructList(count); for (int i = 0; i < count; i++) { Model3D newValue = (Model3D) sourceModel3DCollection._collection[i].Clone(); OnFreezablePropertyChanged(/* oldValue = */ null, newValue); _collection.Add(newValue); OnInsert(newValue); } } /// /// Implementation of Freezable.CloneCurrentValueCore() /// protected override void CloneCurrentValueCore(Freezable source) { Model3DCollection sourceModel3DCollection = (Model3DCollection) source; base.CloneCurrentValueCore(source); int count = sourceModel3DCollection._collection.Count; _collection = new FrugalStructList(count); for (int i = 0; i < count; i++) { Model3D newValue = (Model3D) sourceModel3DCollection._collection[i].CloneCurrentValue(); OnFreezablePropertyChanged(/* oldValue = */ null, newValue); _collection.Add(newValue); OnInsert(newValue); } } /// /// Implementation of Freezable.GetAsFrozenCore() /// protected override void GetAsFrozenCore(Freezable source) { Model3DCollection sourceModel3DCollection = (Model3DCollection) source; base.GetAsFrozenCore(source); int count = sourceModel3DCollection._collection.Count; _collection = new FrugalStructList(count); for (int i = 0; i < count; i++) { Model3D newValue = (Model3D) sourceModel3DCollection._collection[i].GetAsFrozen(); OnFreezablePropertyChanged(/* oldValue = */ null, newValue); _collection.Add(newValue); OnInsert(newValue); } } /// /// Implementation of Freezable.GetCurrentValueAsFrozenCore() /// protected override void GetCurrentValueAsFrozenCore(Freezable source) { Model3DCollection sourceModel3DCollection = (Model3DCollection) source; base.GetCurrentValueAsFrozenCore(source); int count = sourceModel3DCollection._collection.Count; _collection = new FrugalStructList(count); for (int i = 0; i < count; i++) { Model3D newValue = (Model3D) sourceModel3DCollection._collection[i].GetCurrentValueAsFrozen(); OnFreezablePropertyChanged(/* oldValue = */ null, newValue); _collection.Add(newValue); OnInsert(newValue); } } /// /// Implementation of protected override bool FreezeCore(bool isChecking) { bool canFreeze = base.FreezeCore(isChecking); int count = _collection.Count; for (int i = 0; i < count && canFreeze; i++) { canFreeze &= Freezable.Freeze(_collection[i], isChecking); } return canFreeze; } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal FrugalStructListFreezable.FreezeCore . ///_collection; internal uint _version = 0; #endregion Internal Fields #region Enumerator /// /// Enumerates the items in a Model3DCollection /// public struct Enumerator : IEnumerator, IEnumerator{ #region Constructor internal Enumerator(Model3DCollection list) { Debug.Assert(list != null, "list may not be null."); _list = list; _version = list._version; _index = -1; _current = default(Model3D); } #endregion #region Methods void IDisposable.Dispose() { } /// /// Advances the enumerator to the next element of the collection. /// ////// true if the enumerator was successfully advanced to the next element, /// false if the enumerator has passed the end of the collection. /// public bool MoveNext() { _list.ReadPreamble(); if (_version == _list._version) { if (_index > -2 && _index < _list._collection.Count - 1) { _current = _list._collection[++_index]; return true; } else { _index = -2; // -2 indicates "past the end" return false; } } else { throw new InvalidOperationException(SR.Get(SRID.Enumerator_CollectionChanged)); } } ////// Sets the enumerator to its initial position, which is before the /// first element in the collection. /// public void Reset() { _list.ReadPreamble(); if (_version == _list._version) { _index = -1; } else { throw new InvalidOperationException(SR.Get(SRID.Enumerator_CollectionChanged)); } } #endregion #region Properties object IEnumerator.Current { get { return this.Current; } } ////// Current element /// /// The behavior of IEnumerable<T>.Current is undefined /// before the first MoveNext and after we have walked /// off the end of the list. However, the IEnumerable.Current /// contract requires that we throw exceptions /// public Model3D Current { get { if (_index > -1) { return _current; } else if (_index == -1) { throw new InvalidOperationException(SR.Get(SRID.Enumerator_NotStarted)); } else { Debug.Assert(_index == -2, "expected -2, got " + _index + "\n"); throw new InvalidOperationException(SR.Get(SRID.Enumerator_ReachedEnd)); } } } #endregion #region Data private Model3D _current; private Model3DCollection _list; private uint _version; private int _index; #endregion } #endregion #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- ////// Initializes a new instance that is empty. /// public Model3DCollection() { _collection = new FrugalStructList(); } /// /// Initializes a new instance that is empty and has the specified initial capacity. /// /// int - The number of elements that the new list is initially capable of storing. public Model3DCollection(int capacity) { _collection = new FrugalStructList(capacity); } /// /// Creates a Model3DCollection with all of the same elements as collection /// public Model3DCollection(IEnumerablecollection) { // The WritePreamble and WritePostscript aren't technically necessary // in the constructor as of 1/20/05 but they are put here in case // their behavior changes at a later date WritePreamble(); if (collection != null) { bool needsItemValidation = true; ICollection icollectionOfT = collection as ICollection ; if (icollectionOfT != null) { _collection = new FrugalStructList (icollectionOfT); } else { ICollection icollection = collection as ICollection; if (icollection != null) // an IC but not and IC { _collection = new FrugalStructList (icollection); } else // not a IC or IC so fall back to the slower Add { _collection = new FrugalStructList (); foreach (Model3D item in collection) { if (item == null) { throw new System.ArgumentException(SR.Get(SRID.Collection_NoNull)); } Model3D newValue = item; OnFreezablePropertyChanged(/* oldValue = */ null, newValue); _collection.Add(newValue); OnInsert(newValue); } needsItemValidation = false; } } if (needsItemValidation) { foreach (Model3D item in collection) { if (item == null) { throw new System.ArgumentException(SR.Get(SRID.Collection_NoNull)); } OnFreezablePropertyChanged(/* oldValue = */ null, item); OnInsert(item); } } WritePostscript(); } else { throw new ArgumentNullException("collection"); } } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
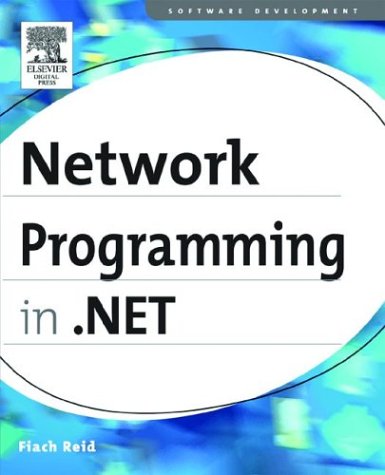
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DetailsViewUpdatedEventArgs.cs
- PageSettings.cs
- TdsParserSessionPool.cs
- DecoderFallback.cs
- EntityUtil.cs
- TextBounds.cs
- wgx_exports.cs
- EntityWrapper.cs
- BamlLocalizabilityResolver.cs
- SevenBitStream.cs
- ManifestResourceInfo.cs
- base64Transforms.cs
- FormsIdentity.cs
- DetailsViewRow.cs
- DynamicQueryableWrapper.cs
- Deflater.cs
- SystemPens.cs
- httpserverutility.cs
- XmlNamespaceMappingCollection.cs
- OdbcPermission.cs
- XPathAxisIterator.cs
- DictionaryBase.cs
- ConfigurationProperty.cs
- ErrorWebPart.cs
- SortedList.cs
- FrameworkObject.cs
- InternalControlCollection.cs
- ToolStripPanelDesigner.cs
- SqlProfileProvider.cs
- GridViewSelectEventArgs.cs
- DecimalAnimationUsingKeyFrames.cs
- PropertyInfoSet.cs
- ListMarkerLine.cs
- Blend.cs
- MergeLocalizationDirectives.cs
- Menu.cs
- AmbientLight.cs
- InvokeProviderWrapper.cs
- Common.cs
- SerializationIncompleteException.cs
- Run.cs
- DataTable.cs
- remotingproxy.cs
- WorkflowPrinting.cs
- RemotingSurrogateSelector.cs
- UnknownBitmapDecoder.cs
- GcHandle.cs
- HybridCollection.cs
- Assembly.cs
- TypeHelper.cs
- HttpGetProtocolImporter.cs
- HttpServerUtilityWrapper.cs
- PolyBezierSegment.cs
- Brush.cs
- WindowsRegion.cs
- DynamicObjectAccessor.cs
- DataControlFieldCell.cs
- BezierSegment.cs
- SortableBindingList.cs
- HttpListenerRequest.cs
- MsmqHostedTransportManager.cs
- SynchronizationContext.cs
- ToolStripContainer.cs
- InvokePattern.cs
- ConfigXmlAttribute.cs
- RealizationContext.cs
- XXXOnTypeBuilderInstantiation.cs
- TabItemWrapperAutomationPeer.cs
- ReferenceSchema.cs
- HttpHandlersSection.cs
- DefaultSerializationProviderAttribute.cs
- ColorContextHelper.cs
- TransactionScopeDesigner.cs
- CLRBindingWorker.cs
- PtsPage.cs
- baseshape.cs
- GridViewRow.cs
- MsmqIntegrationBinding.cs
- QueryOpcode.cs
- ConfigurationConverterBase.cs
- MouseGestureValueSerializer.cs
- StateChangeEvent.cs
- ServiceRoute.cs
- ReadOnlyDictionary.cs
- EnvelopedPkcs7.cs
- IISMapPath.cs
- FrameworkReadOnlyPropertyMetadata.cs
- CounterSample.cs
- NavigatorInput.cs
- ProviderUtil.cs
- PriorityQueue.cs
- RegionData.cs
- ExeConfigurationFileMap.cs
- Privilege.cs
- AttachmentCollection.cs
- CatalogPart.cs
- StringValidator.cs
- FocusTracker.cs
- Sql8ExpressionRewriter.cs
- DataGridColumnHeader.cs