Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Sys / System / IO / compression / Deflater.cs / 1 / Deflater.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // zlib.h -- interface of the 'zlib' general purpose compression library // version 1.2.1, November 17th, 2003 // // Copyright (C) 1995-2003 Jean-loup Gailly and Mark Adler // // This software is provided 'as-is', without any express or implied // warranty. In no event will the authors be held liable for any damages // arising from the use of this software. // // Permission is granted to anyone to use this software for any purpose, // including commercial applications, and to alter it and redistribute it // freely, subject to the following restrictions: // // 1. The origin of this software must not be misrepresented; you must not // claim that you wrote the original software. If you use this software // in a product, an acknowledgment in the product documentation would be // appreciated but is not required. // 2. Altered source versions must be plainly marked as such, and must not be // misrepresented as being the original software. // 3. This notice may not be removed or altered from any source distribution. // // // ==--== // Compression engine namespace System.IO.Compression { using System; using System.Diagnostics; internal class Deflater { private FastEncoder encoder; public Deflater(bool doGZip) { encoder = new FastEncoder(doGZip); } public void SetInput(byte[] input, int startIndex, int count) { encoder.SetInput(input, startIndex, count); } public int GetDeflateOutput(byte[] output) { Debug.Assert(output != null, "Can't pass in a null output buffer!"); return encoder.GetCompressedOutput(output); } public bool NeedsInput() { return encoder.NeedsInput(); } public int Finish(byte[] output) { Debug.Assert(output != null, "Can't pass in a null output buffer!"); return encoder.Finish(output); } } }
Link Menu
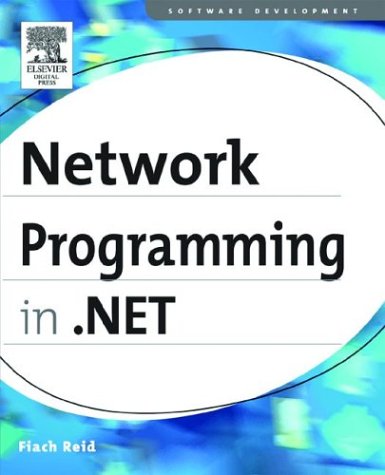
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ObjectDataSourceSelectingEventArgs.cs
- NullableBoolConverter.cs
- Vector3DAnimation.cs
- SafeNativeMethodsCLR.cs
- InternalConfigConfigurationFactory.cs
- WebRequest.cs
- TdsEnums.cs
- XmlDownloadManager.cs
- ObfuscateAssemblyAttribute.cs
- VideoDrawing.cs
- HostedTransportConfigurationManager.cs
- ExpressionBuilder.cs
- RadioButtonAutomationPeer.cs
- infer.cs
- DataGridViewTextBoxColumn.cs
- ProgressPage.cs
- DataFormat.cs
- TerminateSequence.cs
- BamlLocalizer.cs
- Metadata.cs
- MutexSecurity.cs
- EpmTargetPathSegment.cs
- DispatcherProcessingDisabled.cs
- IPAddress.cs
- AnchoredBlock.cs
- WsdlImporterElement.cs
- IPHostEntry.cs
- TreeNodeCollectionEditorDialog.cs
- HtmlInputText.cs
- QueryAsyncResult.cs
- CacheChildrenQuery.cs
- SchemaMapping.cs
- SystemResourceKey.cs
- SafeProcessHandle.cs
- DataMisalignedException.cs
- EditorZoneBase.cs
- SwitchCase.cs
- PointConverter.cs
- OwnerDrawPropertyBag.cs
- CacheHelper.cs
- MergablePropertyAttribute.cs
- AppSecurityManager.cs
- Encoder.cs
- MissingMemberException.cs
- HiddenField.cs
- TabRenderer.cs
- ConstraintManager.cs
- Simplifier.cs
- XmlRootAttribute.cs
- XmlElementCollection.cs
- DataGrid.cs
- SqlConnectionStringBuilder.cs
- SmiContext.cs
- ToolStripSplitButton.cs
- SmiGettersStream.cs
- OracleNumber.cs
- SqlFlattener.cs
- BindableTemplateBuilder.cs
- WebPartConnectionsCancelVerb.cs
- BinaryWriter.cs
- SvcMapFileSerializer.cs
- DocumentApplicationJournalEntry.cs
- Brushes.cs
- ToolStripScrollButton.cs
- TrackPoint.cs
- SupportsEventValidationAttribute.cs
- ExpressionsCollectionConverter.cs
- sqlser.cs
- CompositionAdorner.cs
- HandleRef.cs
- ResourceBinder.cs
- PrintController.cs
- EnumMemberAttribute.cs
- ipaddressinformationcollection.cs
- SoapExtensionReflector.cs
- MutableAssemblyCacheEntry.cs
- ClassValidator.cs
- TransformConverter.cs
- RewritingPass.cs
- Image.cs
- ReferencedCollectionType.cs
- ProgressiveCrcCalculatingStream.cs
- AnonymousIdentificationSection.cs
- SwitchElementsCollection.cs
- InvalidDataContractException.cs
- HttpConfigurationSystem.cs
- httpapplicationstate.cs
- SqlNodeTypeOperators.cs
- TextServicesDisplayAttributePropertyRanges.cs
- NumberSubstitution.cs
- PnrpPermission.cs
- MasterPageCodeDomTreeGenerator.cs
- CodeCommentStatementCollection.cs
- TextFormatterHost.cs
- EventItfInfo.cs
- DbConnectionPoolCounters.cs
- ButtonRenderer.cs
- DependencyProperty.cs
- RelOps.cs
- DbParameterHelper.cs