Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.ServiceModel.Routing / System / ServiceModel / Routing / ClientFactory.cs / 1305376 / ClientFactory.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.ServiceModel.Routing { using System; using System.Collections.Generic; using System.Runtime; using System.ServiceModel; using System.ServiceModel.Channels; using System.ServiceModel.Description; using System.Transactions; static class ClientFactory { public static IRoutingClient Create(RoutingEndpointTrait endpointTrait, RoutingService service, bool impersonating) { Type contractType = endpointTrait.RouterContract; IRoutingClient client; if (contractType == typeof(ISimplexDatagramRouter)) { client = new SimplexDatagramClient(endpointTrait, service.RoutingConfig, impersonating); } else if (contractType == typeof(IRequestReplyRouter)) { client = new RequestReplyClient(endpointTrait, service.RoutingConfig, impersonating); } else if (contractType == typeof(ISimplexSessionRouter)) { client = new SimplexSessionClient(endpointTrait, service.RoutingConfig, impersonating); } else //if (contractType == typeof(IDuplexSessionRouter)) { Fx.Assert(contractType == typeof(IDuplexSessionRouter), "Only one contract type remaining."); client = new DuplexSessionClient(service, endpointTrait, impersonating); } return client; } abstract class RoutingClientBase: ClientBase , IRoutingClient where TChannel : class { bool openCompleted; object thisLock; Queue waiters; protected RoutingClientBase(RoutingEndpointTrait endpointTrait, RoutingConfiguration routingConfig, bool impersonating) : base(endpointTrait.Endpoint.Binding, endpointTrait.Endpoint.Address) { Initialize(endpointTrait, routingConfig, impersonating); } protected RoutingClientBase(RoutingEndpointTrait endpointTrait, RoutingConfiguration routingConfig, object callbackInstance, bool impersonating) : base(new InstanceContext(callbackInstance), endpointTrait.Endpoint.Binding, endpointTrait.Endpoint.Address) { Initialize(endpointTrait, routingConfig, impersonating); } public RoutingEndpointTrait Key { get; private set; } public event EventHandler Faulted; static void ConfigureImpersonation(ServiceEndpoint endpoint, bool impersonating) { // Used for both impersonation and ASP.NET Compatibilty Mode. Both currently require // everything to be synchronous. if (impersonating) { CustomBinding binding = endpoint.Binding as CustomBinding; if (binding == null) { binding = new CustomBinding(endpoint.Binding); } SynchronousSendBindingElement syncSend = binding.Elements.Find (); if (syncSend == null) { binding.Elements.Insert(0, new SynchronousSendBindingElement()); endpoint.Binding = binding; } } } static void ConfigureTransactionFlow(ServiceEndpoint endpoint) { CustomBinding binding = endpoint.Binding as CustomBinding; if (binding == null) { binding = new CustomBinding(endpoint.Binding); } TransactionFlowBindingElement transactionFlow = binding.Elements.Find (); if (transactionFlow != null) { transactionFlow.AllowWildcardAction = true; endpoint.Binding = binding; } } void Initialize(RoutingEndpointTrait endpointTrait, RoutingConfiguration routingConfig, bool impersonating) { this.thisLock = new object(); this.Key = endpointTrait; if (TD.RoutingServiceCreatingClientForEndpointIsEnabled()) { TD.RoutingServiceCreatingClientForEndpoint(this.Key.ToString()); } ServiceEndpoint clientEndpoint = endpointTrait.Endpoint; ServiceEndpoint endpoint = this.Endpoint; KeyedByTypeCollection behaviors = endpoint.Behaviors; endpoint.ListenUri = clientEndpoint.ListenUri; endpoint.ListenUriMode = clientEndpoint.ListenUriMode; endpoint.Name = clientEndpoint.Name; foreach (IEndpointBehavior behavior in clientEndpoint.Behaviors) { // Remove if present, ok to call if not there (will simply return false) behaviors.Remove(behavior.GetType()); behaviors.Add(behavior); } // If the configuration doesn't explicitly add MustUnderstandBehavior (to override us) // add it here, with mustunderstand = false. if (behaviors.Find () == null) { behaviors.Add(new MustUnderstandBehavior(false)); } // If the configuration doesn't explicitly turn off marshaling we add it here. if (routingConfig.SoapProcessingEnabled && behaviors.Find () == null) { behaviors.Add(new SoapProcessingBehavior()); } ConfigureTransactionFlow(endpoint); ConfigureImpersonation(endpoint, impersonating); } protected override TChannel CreateChannel() { TChannel channel = base.CreateChannel(); ((ICommunicationObject)channel).Faulted += this.InnerChannelFaulted; return channel; } public IAsyncResult BeginOperation(Message message, Transaction transaction, AsyncCallback callback, object state) { return new OperationAsyncResult(this, message, transaction, callback, state); } public Message EndOperation(IAsyncResult result) { return OperationAsyncResult.End(result); } protected abstract IAsyncResult OnBeginOperation(Message message, AsyncCallback callback, object state); protected abstract Message OnEndOperation(IAsyncResult asyncResult); void InnerChannelFaulted(object sender, EventArgs args) { EventHandler handlers = this.Faulted; if (handlers != null) { handlers(this, args); } } class OperationAsyncResult : AsyncResult { static AsyncCompletion openComplete = OpenComplete; static AsyncCompletion operationComplete = OperationComplete; static Action
Link Menu
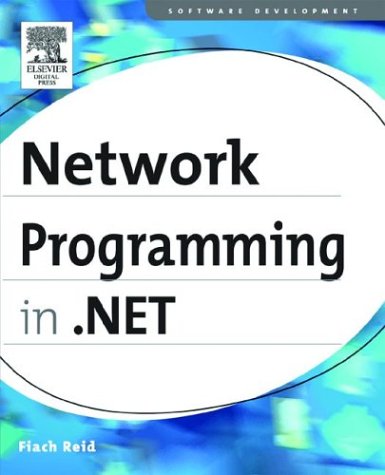
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DelayedRegex.cs
- OleDbDataReader.cs
- GetMemberBinder.cs
- RegionInfo.cs
- GridViewDeletedEventArgs.cs
- OleDbReferenceCollection.cs
- BasicHttpBindingCollectionElement.cs
- ExcCanonicalXml.cs
- SqlPersonalizationProvider.cs
- EventHandlersDesigner.cs
- PeerNameRegistration.cs
- DataSourceXmlTextReader.cs
- NativeRightsManagementAPIsStructures.cs
- DocumentViewerHelper.cs
- GC.cs
- SubtreeProcessor.cs
- DataAdapter.cs
- HttpServerUtilityBase.cs
- CompositionAdorner.cs
- HttpRawResponse.cs
- TargetConverter.cs
- StyleHelper.cs
- QilFactory.cs
- XmlFileEditor.cs
- SendSecurityHeaderElement.cs
- ParagraphResult.cs
- CaseStatement.cs
- LabelLiteral.cs
- EditorPartChrome.cs
- PrtCap_Public_Simple.cs
- ExtentJoinTreeNode.cs
- ConstructorBuilder.cs
- MILUtilities.cs
- SecurityDescriptor.cs
- JoinTreeNode.cs
- DateTimeConverter2.cs
- XPathConvert.cs
- SecurityContext.cs
- WindowsSysHeader.cs
- RelationHandler.cs
- SQLByte.cs
- TabControlAutomationPeer.cs
- LZCodec.cs
- SourceChangedEventArgs.cs
- XmlAttributeCache.cs
- ConstraintConverter.cs
- ReturnType.cs
- EntitySqlQueryBuilder.cs
- DerivedKeySecurityToken.cs
- smtpconnection.cs
- CommandEventArgs.cs
- ZoneMembershipCondition.cs
- CodeStatement.cs
- FacetEnabledSchemaElement.cs
- InvokeBinder.cs
- LassoSelectionBehavior.cs
- X509Certificate2.cs
- KeyTime.cs
- Point4DConverter.cs
- ValidatorCollection.cs
- BasicExpressionVisitor.cs
- TypeDelegator.cs
- CategoryAttribute.cs
- FileDetails.cs
- FontStretch.cs
- LocationUpdates.cs
- SemanticResolver.cs
- ReadOnlyObservableCollection.cs
- StreamAsIStream.cs
- WindowsGraphicsCacheManager.cs
- Grammar.cs
- GenerateScriptTypeAttribute.cs
- PolicyStatement.cs
- DataColumnCollection.cs
- SerializationStore.cs
- EventManager.cs
- IIS7UserPrincipal.cs
- ClonableStack.cs
- ToolStripItemCollection.cs
- NameSpaceEvent.cs
- PrimitiveSchema.cs
- WebPartVerbsEventArgs.cs
- _OverlappedAsyncResult.cs
- CurrencyWrapper.cs
- Literal.cs
- _UriSyntax.cs
- BitStack.cs
- Wow64ConfigurationLoader.cs
- DocumentOrderQuery.cs
- ColumnBinding.cs
- Base64Encoder.cs
- NullableLongSumAggregationOperator.cs
- EnumConverter.cs
- CodeGenerationManager.cs
- OneToOneMappingSerializer.cs
- TypeLoader.cs
- ExtendedProtectionPolicy.cs
- TreeNodeStyle.cs
- ReachPrintTicketSerializer.cs
- X509CertificateValidationMode.cs