Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / Tools / WSATConfig / Configuration / RegistryExceptionHelper.cs / 1305376 / RegistryExceptionHelper.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.Tools.ServiceModel.WsatConfig { using Microsoft.Win32; using System; class RegistryExceptionHelper { string registryKey; public RegistryExceptionHelper(string registryKey) { this.registryKey = registryKey; EnsureEndsWithSlash(ref this.registryKey); } public RegistryExceptionHelper(string machineName, RegistryHive registryHive, string registryKeyRelativeToHive) : this(RegistryExceptionHelper.GetRegistryKeyBase(machineName, registryHive) + registryKeyRelativeToHive) { } public static void EnsureEndsWithSlash(ref string str) { if (!string.IsNullOrEmpty(str)) { if (!str.EndsWith("\\", StringComparison.OrdinalIgnoreCase)) { str += '\\'; } } } static string GetRegistryKeyBase(string machineName, RegistryHive registryHive) { string registryBase = Utilities.IsLocalMachineName(machineName) ? string.Empty : SR.GetString(SR.RemoteRegistryFormat, machineName); switch (registryHive) { case RegistryHive.ClassesRoot: registryBase += Registry.ClassesRoot.Name; break; case RegistryHive.CurrentUser: registryBase += Registry.CurrentUser.Name; break; case RegistryHive.LocalMachine: registryBase += Registry.LocalMachine.Name; break; default: // We do not support other values here System.Diagnostics.Debug.Assert(false, "registryHive is not supported"); break; } RegistryExceptionHelper.EnsureEndsWithSlash(ref registryBase); return registryBase; } public WsatAdminException CreateRegistryAccessException(int errorCode) { return CreateRegistryAccessException(unchecked((uint)errorCode)); } public WsatAdminException CreateRegistryAccessException(uint errorCode) { return new WsatAdminException(WsatAdminErrorCode.REGISTRY_ACCESS, SR.GetString(SR.ErrorRegistryAccess, registryKey, errorCode)); } public WsatAdminException CreateRegistryAccessException(Exception innerException) { return DoCreateRegistryAccessException(registryKey, innerException); } public WsatAdminException CreateRegistryAccessException(string subRegistryKey, Exception innerException) { return DoCreateRegistryAccessException(registryKey + subRegistryKey, innerException); } static WsatAdminException DoCreateRegistryAccessException(string regKey, Exception innerException) { if (innerException == null) { return new WsatAdminException(WsatAdminErrorCode.REGISTRY_ACCESS, SR.GetString(SR.ErrorRegistryAccessNoErrorCode, regKey)); } return new WsatAdminException(WsatAdminErrorCode.REGISTRY_ACCESS, SR.GetString(SR.ErrorRegistryAccessNoErrorCode, regKey), innerException); } public WsatAdminException CreateRegistryWriteException(Exception innerException) { return DoCreateRegistryWriteException(registryKey, innerException); } public WsatAdminException CreateRegistryWriteException(string subRegistryKey, Exception innerException) { return DoCreateRegistryWriteException(registryKey + subRegistryKey, innerException); } static WsatAdminException DoCreateRegistryWriteException(string regKey, Exception innerException) { if (innerException == null) { return new WsatAdminException(WsatAdminErrorCode.REGISTRY_WRITE, SR.GetString(SR.ErrorRegistryWrite, regKey)); } return new WsatAdminException(WsatAdminErrorCode.REGISTRY_WRITE, SR.GetString(SR.ErrorRegistryWrite, regKey), innerException); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
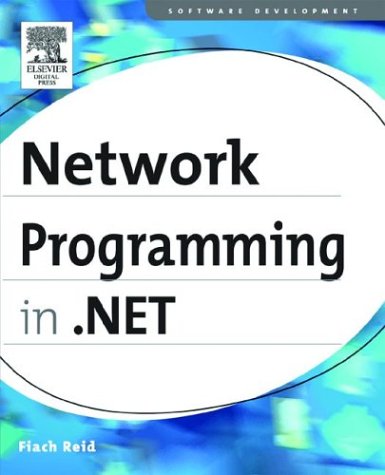
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ObjectDataSource.cs
- UriSection.cs
- DynamicRendererThreadManager.cs
- ToolboxItemAttribute.cs
- CompModSwitches.cs
- SqlConnectionString.cs
- PerformanceCounterManager.cs
- StrokeNodeOperations2.cs
- Vector3DKeyFrameCollection.cs
- CounterCreationDataCollection.cs
- TextEffectCollection.cs
- AppDomainProtocolHandler.cs
- NameTable.cs
- DataGrid.cs
- ComNativeDescriptor.cs
- NullableDecimalAverageAggregationOperator.cs
- WebConfigurationHostFileChange.cs
- Point3DConverter.cs
- SplayTreeNode.cs
- UnitySerializationHolder.cs
- XmlSchemaDatatype.cs
- State.cs
- SimpleMailWebEventProvider.cs
- WindowsButton.cs
- BitVector32.cs
- JournalEntryListConverter.cs
- EntityProviderServices.cs
- SystemGatewayIPAddressInformation.cs
- ZipPackage.cs
- WebPartDisplayMode.cs
- DbParameterCollectionHelper.cs
- HtmlControl.cs
- PackageStore.cs
- NativeMethods.cs
- Configuration.cs
- ZipIOCentralDirectoryBlock.cs
- ToolStripPanelCell.cs
- HotSpot.cs
- SecurityUtils.cs
- TraceContextEventArgs.cs
- TemplateBamlRecordReader.cs
- SmiConnection.cs
- ViewCellRelation.cs
- Int16AnimationUsingKeyFrames.cs
- autovalidator.cs
- SettingsPropertyCollection.cs
- SQLSingle.cs
- RepeatBehaviorConverter.cs
- DesignerSelectionListAdapter.cs
- AutomationPropertyInfo.cs
- OrderedParallelQuery.cs
- ColumnMapProcessor.cs
- ListViewSelectEventArgs.cs
- AutoGeneratedFieldProperties.cs
- TraceLevelHelper.cs
- ScriptDescriptor.cs
- ResXResourceWriter.cs
- assemblycache.cs
- TemplatedWizardStep.cs
- XmlSchemaSimpleType.cs
- SiteMembershipCondition.cs
- CalculatedColumn.cs
- ToolBar.cs
- BevelBitmapEffect.cs
- LoginCancelEventArgs.cs
- PersistenceContextEnlistment.cs
- MediaPlayerState.cs
- KeyboardEventArgs.cs
- TypeCollectionDesigner.xaml.cs
- InvariantComparer.cs
- KnownAssemblyEntry.cs
- RemotingService.cs
- SqlInternalConnectionSmi.cs
- TypeInitializationException.cs
- RoleService.cs
- VirtualPathData.cs
- InputScope.cs
- CommandPlan.cs
- FontResourceCache.cs
- VersionedStreamOwner.cs
- TdsValueSetter.cs
- TableLayoutSettings.cs
- EditorZoneBase.cs
- DesignSurfaceEvent.cs
- BuildResult.cs
- BrowserDefinition.cs
- formatter.cs
- SiteMapDesignerDataSourceView.cs
- CaretElement.cs
- GroupBoxDesigner.cs
- SamlAttribute.cs
- BitmapPalettes.cs
- MailWriter.cs
- documentsequencetextpointer.cs
- Int16.cs
- EtwTrace.cs
- ClientRuntimeConfig.cs
- ThreadExceptionEvent.cs
- SqlGatherConsumedAliases.cs
- RestHandler.cs