Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / SqlClient / SqlGen / SqlBuilder.cs / 1305376 / SqlBuilder.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.IO; using System.Text; using System.Data.SqlClient; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees; namespace System.Data.SqlClient.SqlGen { ////// This class is like StringBuilder. While traversing the tree for the first time, /// we do not know all the strings that need to be appended e.g. things that need to be /// renamed, nested select statements etc. So, we use a builder that can collect /// all kinds of sql fragments. /// internal class SqlBuilder : ISqlFragment { private List
Link Menu
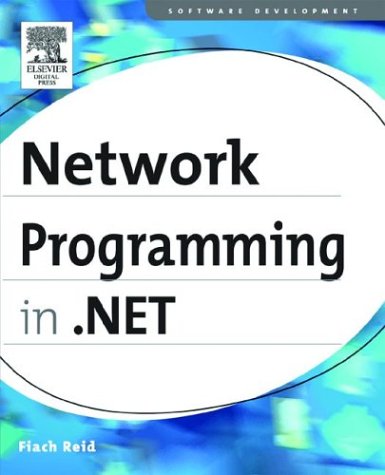
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RegexRunnerFactory.cs
- Double.cs
- AbstractDataSvcMapFileLoader.cs
- MethodImplAttribute.cs
- X509UI.cs
- DataBoundLiteralControl.cs
- StretchValidation.cs
- GC.cs
- IteratorDescriptor.cs
- RangeBaseAutomationPeer.cs
- WebBrowserUriTypeConverter.cs
- TransactionContext.cs
- CodeDOMProvider.cs
- XmlSchemaChoice.cs
- HttpFileCollection.cs
- KnownTypesProvider.cs
- DelayDesigner.cs
- WindowsRegion.cs
- VarRefManager.cs
- NonParentingControl.cs
- SqlDataSource.cs
- TimerElapsedEvenArgs.cs
- ScrollEvent.cs
- ControlParameter.cs
- DescendentsWalkerBase.cs
- SpAudioStreamWrapper.cs
- CfgSemanticTag.cs
- PnrpPeerResolverBindingElement.cs
- URI.cs
- MatrixAnimationBase.cs
- SqlMetaData.cs
- UICuesEvent.cs
- TextEditorThreadLocalStore.cs
- CodeDOMUtility.cs
- JapaneseLunisolarCalendar.cs
- Vector3DConverter.cs
- MethodAccessException.cs
- HtmlHistory.cs
- EventLogWatcher.cs
- ServiceBuildProvider.cs
- EventToken.cs
- XmlSerializerSection.cs
- GacUtil.cs
- MessageBox.cs
- Geometry.cs
- BulletChrome.cs
- PrivilegeNotHeldException.cs
- Schema.cs
- WarningException.cs
- DoWorkEventArgs.cs
- SoapEnumAttribute.cs
- Rfc2898DeriveBytes.cs
- Helper.cs
- ConnectionInterfaceCollection.cs
- WinEventHandler.cs
- InkCanvasInnerCanvas.cs
- Dump.cs
- MulticastDelegate.cs
- _BufferOffsetSize.cs
- ColorMap.cs
- ApplicationFileParser.cs
- printdlgexmarshaler.cs
- XmlNamedNodeMap.cs
- RunInstallerAttribute.cs
- ResourceSetExpression.cs
- SplineKeyFrames.cs
- WebPartExportVerb.cs
- WorkflowIdleElement.cs
- RegexStringValidatorAttribute.cs
- HitTestDrawingContextWalker.cs
- FormsAuthenticationUserCollection.cs
- ObjectContextServiceProvider.cs
- PeerResolverMode.cs
- CfgArc.cs
- StorageFunctionMapping.cs
- ClientSettingsProvider.cs
- AutoGeneratedField.cs
- XamlToRtfParser.cs
- RowType.cs
- SystemIPGlobalStatistics.cs
- Terminate.cs
- SoapExtension.cs
- CryptoConfig.cs
- SqlClientFactory.cs
- Formatter.cs
- OutputCacheProfile.cs
- Hex.cs
- GridViewColumnHeader.cs
- Matrix3DStack.cs
- WebControlParameterProxy.cs
- CompilerError.cs
- XmlSiteMapProvider.cs
- OleDbSchemaGuid.cs
- Group.cs
- TextLineBreak.cs
- AccessViolationException.cs
- PreviewKeyDownEventArgs.cs
- Propagator.ExtentPlaceholderCreator.cs
- DataObjectAttribute.cs
- TypedTableBase.cs