Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Services / Web / System / Web / Services / Discovery / DiscoveryReference.cs / 1305376 / DiscoveryReference.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Services.Discovery { using System; using System.Xml.Serialization; using System.Text.RegularExpressions; using System.IO; using System.Text; using System.Threading; using System.Collections; using System.Diagnostics; using System.Web.Services.Diagnostics; ////// /// public abstract class DiscoveryReference { private DiscoveryClientProtocol clientProtocol; ///[To be supplied.] ////// /// [XmlIgnore] public DiscoveryClientProtocol ClientProtocol { get { return clientProtocol; } set { clientProtocol = value; } } ///[To be supplied.] ////// /// [XmlIgnore] public virtual string DefaultFilename { get { return FilenameFromUrl(Url); } } ///[To be supplied.] ////// /// public abstract void WriteDocument(object document, Stream stream); ///[To be supplied.] ////// /// public abstract object ReadDocument(Stream stream); ///[To be supplied.] ////// /// [XmlIgnore] public abstract string Url { get; set; } internal virtual void LoadExternals(Hashtable loadedExternals) { } ///[To be supplied.] ////// /// public static string FilenameFromUrl(string url) { // get everything after the last /, not including the one at the end of the string int lastSlash = url.LastIndexOf('/', url.Length - 1); if (lastSlash >= 0) url = url.Substring(lastSlash + 1); // get everything up to the first dot (the filename) int firstDot = url.IndexOf('.'); if (firstDot >= 0) url = url.Substring(0, firstDot); // make sure we don't include the question mark and stuff that follows it int question = url.IndexOf('?'); if (question >= 0) url = url.Substring(0, question); if (url == null || url.Length == 0) return "item"; return MakeValidFilename(url); } private static bool FindChar(char ch, char[] chars) { for (int i = 0; i < chars.Length; i++) { if (ch == chars[i]) return true; } return false; } internal static string MakeValidFilename(string filename) { if (filename == null) return null; StringBuilder sb = new StringBuilder(filename.Length); for (int i = 0; i < filename.Length; i++) { char c = filename[i]; if (!FindChar(c, Path.InvalidPathChars)) sb.Append(c); } string name = sb.ToString(); if (name.Length == 0) name="item"; return Path.GetFileName(name); } ///[To be supplied.] ////// /// public void Resolve() { if (ClientProtocol == null) throw new InvalidOperationException(Res.GetString(Res.WebResolveMissingClientProtocol)); if (ClientProtocol.Documents[Url] != null) return; if (ClientProtocol.InlinedSchemas[Url] != null) return; string newUrl = Url; string oldUrl = Url; string contentType = null; Stream stream = ClientProtocol.Download(ref newUrl, ref contentType); if (ClientProtocol.Documents[newUrl] != null) { Url = newUrl; return; } try { Url = newUrl; Resolve(contentType, stream); } catch { Url = oldUrl; throw; } finally { stream.Close(); } } internal Exception AttemptResolve(string contentType, Stream stream) { try { Resolve(contentType, stream); return null; } catch (Exception e) { if (e is ThreadAbortException || e is StackOverflowException || e is OutOfMemoryException) { throw; } if (Tracing.On) Tracing.ExceptionCatch(TraceEventType.Warning, this, "AttemptResolve", e); return e; } } ///[To be supplied.] ////// /// protected internal abstract void Resolve(string contentType, Stream stream); internal static string UriToString(string baseUrl, string relUrl) { return (new Uri(new Uri(baseUrl), relUrl)).GetComponents(UriComponents.AbsoluteUri, UriFormat.SafeUnescaped); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
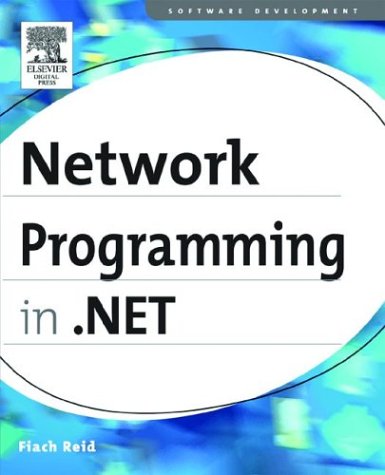
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MSG.cs
- XPathBuilder.cs
- AssemblyInfo.cs
- _SslState.cs
- CatalogPartChrome.cs
- SelectionChangedEventArgs.cs
- SpeechDetectedEventArgs.cs
- WindowsTokenRoleProvider.cs
- QueryInterceptorAttribute.cs
- TagMapCollection.cs
- ConfigXmlDocument.cs
- MailMessageEventArgs.cs
- safex509handles.cs
- WebPartsSection.cs
- SwitchAttribute.cs
- EncryptedPackageFilter.cs
- WebPartEditorApplyVerb.cs
- CLRBindingWorker.cs
- LinqDataView.cs
- GridViewRowPresenterBase.cs
- BufferedWebEventProvider.cs
- XmlSchemaSimpleContentRestriction.cs
- ReferenceEqualityComparer.cs
- SqlPersistenceWorkflowInstanceDescription.cs
- ImageCollectionCodeDomSerializer.cs
- IndicFontClient.cs
- BufferedResponseStream.cs
- TrackingProfileSerializer.cs
- SqlRowUpdatingEvent.cs
- BuilderElements.cs
- FixedTextContainer.cs
- unsafeIndexingFilterStream.cs
- SingleAnimationBase.cs
- WebResourceUtil.cs
- BindingSource.cs
- TypedTableGenerator.cs
- EditorZoneBase.cs
- DSASignatureFormatter.cs
- InkCanvasFeedbackAdorner.cs
- FamilyTypeface.cs
- CompilationUtil.cs
- EntityDataSourceContextCreatingEventArgs.cs
- SplitterDesigner.cs
- DbQueryCommandTree.cs
- TraceContextEventArgs.cs
- ControlParameter.cs
- Odbc32.cs
- HtmlLink.cs
- TextEditorLists.cs
- DesignerProperties.cs
- WindowsRegion.cs
- TripleDES.cs
- LogicalExpressionTypeConverter.cs
- IIS7UserPrincipal.cs
- Schema.cs
- ZoneLinkButton.cs
- RightsManagementInformation.cs
- xsdvalidator.cs
- ViewLoader.cs
- ContextBase.cs
- ScriptReferenceBase.cs
- OdbcPermission.cs
- KeyTimeConverter.cs
- ChangeProcessor.cs
- Point3DKeyFrameCollection.cs
- COAUTHIDENTITY.cs
- ModelItemCollection.cs
- ValueUtilsSmi.cs
- Geometry3D.cs
- ObjectListItem.cs
- PhysicalFontFamily.cs
- TripleDESCryptoServiceProvider.cs
- SoapUnknownHeader.cs
- RemotingServices.cs
- MulticastDelegate.cs
- ColorBlend.cs
- NetMsmqBindingCollectionElement.cs
- ImageAttributes.cs
- IInstanceTable.cs
- HierarchicalDataBoundControl.cs
- InvokeHandlers.cs
- RC2CryptoServiceProvider.cs
- SamlConditions.cs
- SymmetricCryptoHandle.cs
- PartitionResolver.cs
- ToolStripSeparator.cs
- SQLCharsStorage.cs
- WindowsSysHeader.cs
- NativeMethods.cs
- PrintPreviewDialog.cs
- SingleConverter.cs
- SecurityContext.cs
- SqlTopReducer.cs
- TextRangeEditTables.cs
- ClientFormsIdentity.cs
- RawStylusInputCustomData.cs
- QilSortKey.cs
- RawStylusSystemGestureInputReport.cs
- StrongNameUtility.cs
- ExpressionUtilities.cs