Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / View / Win32Interop.cs / 1484997 / Win32Interop.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.View { using System; using System.Runtime.InteropServices; using System.Diagnostics.CodeAnalysis; static class Win32Interop { public const int WM_SETICON = 0x80; public const int WM_NCHITTEST = 0x84; public const int WM_SYSCOMMAND = 0x0112; public const int GWL_STYLE = -16; public const int WS_MAXIMIZEBOX = 0x00010000; public const int WS_MINIMIZEBOX = 0x00020000; public const int GWL_EXSTYLE = -20; public const int WS_EX_DLGMODALFRAME = 0x00000001; public const int WS_EX_CONTEXTHELP = 0x00000400; public const int SC_CONTEXTHELP = 0xf180; public const int ICON_SMALL = 0; public const int ICON_BIG = 1; [DllImport("User32", EntryPoint = "ScreenToClient", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)] public static extern int ScreenToClient(IntPtr hWnd, [In, Out] POINT pt); [StructLayout(LayoutKind.Sequential)] public sealed class POINT { public int x; public int y; public POINT() { this.x = 0; this.y = 0; } public POINT(int x, int y) { this.x = x; this.y = y; } } // This static method is required because legacy OSes do not support // SetWindowLongPtr internal static IntPtr SetWindowLongPtr(HandleRef hWnd, int nIndex, IntPtr dwNewLong) { if (IntPtr.Size == 8) return SetWindowLongPtr64(hWnd, nIndex, dwNewLong); else return new IntPtr(SetWindowLong32(hWnd, nIndex, dwNewLong.ToInt32())); } // This static method is required because Win32 does not support // GetWindowLongPtr directly public static IntPtr GetWindowLongPtr(IntPtr hWnd, int nIndex) { if (IntPtr.Size == 8) return GetWindowLongPtr64(hWnd, nIndex); else return GetWindowLongPtr32(hWnd, nIndex); } [DllImport("user32.dll", EntryPoint = "SetWindowLong")] private static extern int SetWindowLong32(HandleRef hWnd, int nIndex, int dwNewLong); [DllImport("user32.dll", EntryPoint = "SetWindowLongPtr")] private static extern IntPtr SetWindowLongPtr64(HandleRef hWnd, int nIndex, IntPtr dwNewLong); [SuppressMessage("Microsoft.Portability", "CA1901:PInvokeDeclarationsShouldBePortable", MessageId = "return", Justification = "Calling code is expected to handle the different size of IntPtr")] [DllImport("user32.dll", EntryPoint = "GetWindowLong")] private static extern IntPtr GetWindowLongPtr32(IntPtr hWnd, int nIndex); [DllImport("user32.dll", EntryPoint = "GetWindowLongPtr")] private static extern IntPtr GetWindowLongPtr64(IntPtr hWnd, int nIndex); [DllImport("user32.dll")] public static extern IntPtr SendMessage(IntPtr hWnd, int Msg, IntPtr wParam, IntPtr lParam); [DllImport("user32.dll", CharSet = CharSet.Auto, ExactSpelling = true)] public static extern IntPtr GetActiveWindow(); [System.Runtime.InteropServices.DllImport("gdi32.dll")] public static extern bool DeleteObject(IntPtr hObject); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
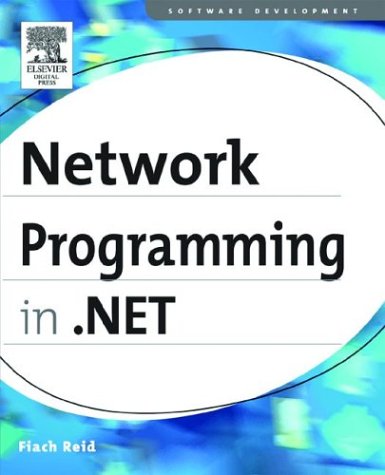
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HtmlSelectionListAdapter.cs
- SizeFConverter.cs
- ContextProperty.cs
- TypeConverter.cs
- ImageListUtils.cs
- CqlWriter.cs
- DesignerAutoFormatStyle.cs
- VariableBinder.cs
- ClockController.cs
- IERequestCache.cs
- AnnotationDocumentPaginator.cs
- AttachedPropertyBrowsableAttribute.cs
- NameObjectCollectionBase.cs
- State.cs
- Stopwatch.cs
- TemplateBindingExpressionConverter.cs
- MetadataExporter.cs
- DataGridBoundColumn.cs
- CustomAttributeFormatException.cs
- AlternateViewCollection.cs
- WsatServiceAddress.cs
- SqlCommandBuilder.cs
- Attribute.cs
- ComplexPropertyEntry.cs
- AppDomain.cs
- TemporaryBitmapFile.cs
- tibetanshape.cs
- PerformanceCounters.cs
- WebPartConnectionsEventArgs.cs
- CreateSequence.cs
- VectorAnimationBase.cs
- COM2PictureConverter.cs
- XmlNamespaceManager.cs
- MatrixTransform3D.cs
- Activity.cs
- Part.cs
- RayHitTestParameters.cs
- ControlCachePolicy.cs
- DataGridViewRowDividerDoubleClickEventArgs.cs
- Timer.cs
- ConvertEvent.cs
- oledbmetadatacollectionnames.cs
- StorageInfo.cs
- VerificationAttribute.cs
- WebServiceBindingAttribute.cs
- Subtree.cs
- ToolStripStatusLabel.cs
- ColumnReorderedEventArgs.cs
- SecurityTokenAttachmentMode.cs
- CompilerLocalReference.cs
- RecordsAffectedEventArgs.cs
- EventKeyword.cs
- DataBindEngine.cs
- StoragePropertyMapping.cs
- ContentPropertyAttribute.cs
- HelpKeywordAttribute.cs
- EntityDataSourceContextDisposingEventArgs.cs
- SqlExpressionNullability.cs
- Event.cs
- ElapsedEventArgs.cs
- TimeSpanOrInfiniteConverter.cs
- SendMailErrorEventArgs.cs
- ProcessModelSection.cs
- WebPartConnectionsDisconnectVerb.cs
- ModelTreeEnumerator.cs
- EventItfInfo.cs
- SecureConversationSecurityTokenParameters.cs
- CustomActivityDesigner.cs
- TextRangeAdaptor.cs
- ModelItemCollection.cs
- FullTextBreakpoint.cs
- ChannelTokenTypeConverter.cs
- TypeLibConverter.cs
- UpdateCommand.cs
- XmlSchemaCollection.cs
- RunWorkerCompletedEventArgs.cs
- TypeForwardedToAttribute.cs
- EncryptedXml.cs
- Utilities.cs
- CodeGotoStatement.cs
- ErrorCodes.cs
- DataBoundControlHelper.cs
- InfoCard.cs
- SmiEventSink.cs
- ConfigXmlAttribute.cs
- Rotation3D.cs
- Label.cs
- UpDownBase.cs
- ConfigurationCollectionAttribute.cs
- XmlExpressionDumper.cs
- TemplatedMailWebEventProvider.cs
- _NativeSSPI.cs
- ReachDocumentSequenceSerializerAsync.cs
- XmlValueConverter.cs
- InvokeBinder.cs
- CachingHintValidation.cs
- StringResourceManager.cs
- Slider.cs
- BinaryExpression.cs
- RegistrationServices.cs