Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / ScrollBarRenderer.cs / 1305376 / ScrollBarRenderer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Windows.Forms.VisualStyles; using System.Diagnostics.CodeAnalysis; using Microsoft.Win32; ////// /// public sealed class ScrollBarRenderer { //Make this per-thread, so that different threads can safely use these methods. [ThreadStatic] private static VisualStyleRenderer visualStyleRenderer = null; //cannot instantiate private ScrollBarRenderer() { } ////// This is a rendering class for the ScrollBar control. /// ////// /// public static bool IsSupported { get { return VisualStyleRenderer.IsSupported; // no downlevel support } } ////// Returns true if this class is supported for the current OS and user/application settings, /// otherwise returns false. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawArrowButton(Graphics g, Rectangle bounds, ScrollBarArrowButtonState state) { InitializeRenderer(VisualStyleElement.ScrollBar.ArrowButton.LeftNormal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a ScrollBar arrow button. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawHorizontalThumb(Graphics g, Rectangle bounds, ScrollBarState state) { InitializeRenderer(VisualStyleElement.ScrollBar.ThumbButtonHorizontal.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a horizontal ScrollBar thumb. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawVerticalThumb(Graphics g, Rectangle bounds, ScrollBarState state) { InitializeRenderer(VisualStyleElement.ScrollBar.ThumbButtonVertical.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a vertical ScrollBar thumb. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawHorizontalThumbGrip(Graphics g, Rectangle bounds, ScrollBarState state) { InitializeRenderer(VisualStyleElement.ScrollBar.GripperHorizontal.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a horizontal ScrollBar thumb grip. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawVerticalThumbGrip(Graphics g, Rectangle bounds, ScrollBarState state) { InitializeRenderer(VisualStyleElement.ScrollBar.GripperVertical.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a vertical ScrollBar thumb grip. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawRightHorizontalTrack(Graphics g, Rectangle bounds, ScrollBarState state) { InitializeRenderer(VisualStyleElement.ScrollBar.RightTrackHorizontal.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a horizontal ScrollBar thumb. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawLeftHorizontalTrack(Graphics g, Rectangle bounds, ScrollBarState state) { InitializeRenderer(VisualStyleElement.ScrollBar.LeftTrackHorizontal.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a horizontal ScrollBar thumb. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawUpperVerticalTrack(Graphics g, Rectangle bounds, ScrollBarState state) { InitializeRenderer(VisualStyleElement.ScrollBar.UpperTrackVertical.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a vertical ScrollBar thumb in the center of the given bounds. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawLowerVerticalTrack(Graphics g, Rectangle bounds, ScrollBarState state) { InitializeRenderer(VisualStyleElement.ScrollBar.LowerTrackVertical.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a vertical ScrollBar thumb in the center of the given bounds. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawSizeBox(Graphics g, Rectangle bounds, ScrollBarSizeBoxState state) { InitializeRenderer(VisualStyleElement.ScrollBar.SizeBox.LeftAlign, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a ScrollBar size box in the center of the given bounds. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static Size GetThumbGripSize(Graphics g, ScrollBarState state) { InitializeRenderer(VisualStyleElement.ScrollBar.GripperHorizontal.Normal, (int)state); return visualStyleRenderer.GetPartSize(g, ThemeSizeType.True); } ////// Returns the size of the ScrollBar thumb grip. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static Size GetSizeBoxSize(Graphics g, ScrollBarState state) { InitializeRenderer(VisualStyleElement.ScrollBar.SizeBox.LeftAlign, (int)state); return visualStyleRenderer.GetPartSize(g, ThemeSizeType.True); } private static void InitializeRenderer(VisualStyleElement element, int state) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(element.ClassName, element.Part, state); } else { visualStyleRenderer.SetParameters(element.ClassName, element.Part, state); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Returns the size of the ScrollBar size box. /// ///
Link Menu
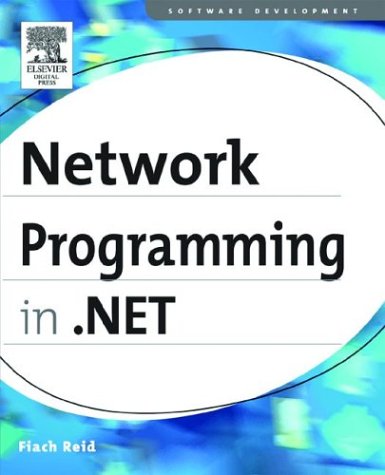
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CompiledRegexRunnerFactory.cs
- CodeObjectCreateExpression.cs
- ContainerControl.cs
- PagedDataSource.cs
- LoginUtil.cs
- DateTimeFormatInfoScanner.cs
- MonthChangedEventArgs.cs
- AnnotationResource.cs
- RijndaelManaged.cs
- XmlDeclaration.cs
- DataTemplateKey.cs
- ConfigurationStrings.cs
- ClosableStream.cs
- IntegerFacetDescriptionElement.cs
- DataGridViewColumnCollection.cs
- TransformPattern.cs
- TableRowGroup.cs
- InputBuffer.cs
- DrawingContextDrawingContextWalker.cs
- ToolboxItemSnapLineBehavior.cs
- ProjectionNode.cs
- FileInfo.cs
- ConfigurationValue.cs
- DiagnosticTrace.cs
- Size3D.cs
- ChtmlImageAdapter.cs
- InfoCardX509Validator.cs
- DocumentSchemaValidator.cs
- XmlText.cs
- Main.cs
- DataSourceCache.cs
- Metadata.cs
- APCustomTypeDescriptor.cs
- WSFederationHttpBindingElement.cs
- SerializationObjectManager.cs
- AstNode.cs
- BufferBuilder.cs
- WindowsGraphics.cs
- XmlSchemaComplexType.cs
- HwndSource.cs
- BCLDebug.cs
- WebPartsPersonalizationAuthorization.cs
- ExtensibleClassFactory.cs
- AuthorizationSection.cs
- XmlSerializerVersionAttribute.cs
- PingOptions.cs
- DefaultValueTypeConverter.cs
- BrowserCapabilitiesCompiler.cs
- DivideByZeroException.cs
- TextTrailingCharacterEllipsis.cs
- NamedPipeHostedTransportConfiguration.cs
- Int16Animation.cs
- DialogWindow.cs
- Debugger.cs
- TransactionManager.cs
- Pen.cs
- MLangCodePageEncoding.cs
- FontSizeConverter.cs
- Subtree.cs
- SqlBinder.cs
- WebPartAuthorizationEventArgs.cs
- TogglePattern.cs
- XmlSchemaException.cs
- Globals.cs
- DbExpressionBuilder.cs
- TabControlEvent.cs
- VSWCFServiceContractGenerator.cs
- DesignerAttribute.cs
- RtType.cs
- DataGridBoolColumn.cs
- LabelLiteral.cs
- BooleanConverter.cs
- Msec.cs
- DefaultBinder.cs
- ContextQuery.cs
- ToolStripKeyboardHandlingService.cs
- UnsafeNativeMethodsMilCoreApi.cs
- RepeatEnumerable.cs
- PresentationSource.cs
- BackgroundWorker.cs
- Route.cs
- RuntimeConfigLKG.cs
- PerfService.cs
- LingerOption.cs
- MetadataCache.cs
- InheritanceContextChangedEventManager.cs
- RoutedEvent.cs
- IteratorDescriptor.cs
- _UncName.cs
- DataSet.cs
- GeneralTransform3DGroup.cs
- DiscoveryServiceExtension.cs
- CssStyleCollection.cs
- UriSectionReader.cs
- HtmlInputImage.cs
- ToolStripComboBox.cs
- BindingListCollectionView.cs
- RoleGroup.cs
- QuarticEase.cs
- TreeView.cs