Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Input / Stylus / RawStylusInput.cs / 1 / RawStylusInput.cs
using System; using System.Collections; using System.Windows.Media; using System.Security; using System.Security.Permissions; using MS.Internal; using MS.Internal.PresentationCore; // SecurityHelper using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input.StylusPlugIns { ///////////////////////////////////////////////////////////////////////// ////// [TBS] /// public class RawStylusInput { ///////////////////////////////////////////////////////////////////// ////// [TBS] /// /// [TBS] /// [TBS] /// [TBS] internal RawStylusInput( RawStylusInputReport report, GeneralTransform tabletToElementTransform, StylusPlugInCollection targetPlugInCollection) { if (report == null) { throw new ArgumentNullException("report"); } if (tabletToElementTransform.Inverse == null) { throw new ArgumentException(SR.Get(SRID.Stylus_MatrixNotInvertable), "tabletToElementTransform"); } if (targetPlugInCollection == null) { throw new ArgumentNullException("targetPlugInCollection"); } // We should always see this GeneralTransform is frozen since we access this from multiple threads. System.Diagnostics.Debug.Assert(tabletToElementTransform.IsFrozen); _report = report; _tabletToElementTransform = tabletToElementTransform; _targetPlugInCollection = targetPlugInCollection; } ////// /// public int StylusDeviceId { get { return _report.StylusDeviceId; } } ////// /// public int TabletDeviceId { get { return _report.TabletDeviceId; } } ////// /// public int Timestamp { get { return _report.Timestamp; } } ////// Returns a copy of the StylusPoints /// public StylusPointCollection GetStylusPoints() { return GetStylusPoints(Transform.Identity); } ////// Internal method called by StylusDevice to prevent two copies /// ////// Critical: This code accesses InputReport.InputSource /// TreatAsSafe: The method only gets a transform from PresentationSource and returns StylusPointCollection. /// Those operations are considered safe. /// This method is called by: /// RawStylusInput.GetStylusPoints /// StylusDevice.UpdateEventStylusPoints(RawStylusInputReport, Boolean) /// [SecurityCritical, SecurityTreatAsSafe] internal StylusPointCollection GetStylusPoints(GeneralTransform transform) { if (_stylusPoints == null) { // GeneralTransformGroup group = new GeneralTransformGroup(); if ( StylusDeviceId == 0) { // Only do this for the Mouse group.Children.Add(new MatrixTransform(_report.InputSource.CompositionTarget.TransformFromDevice)); } group.Children.Add(_tabletToElementTransform); group.Children.Add(transform); return new StylusPointCollection(_report.StylusPointDescription, _report.GetRawPacketData(), group, Matrix.Identity); } else { return _stylusPoints.Clone(transform, _stylusPoints.Description); } } ////// Replaces the StylusPoints. /// ////// Callers must have Unmanaged code permission to call this API. /// /// stylusPoints ////// Callers must have Unmanaged code permission to call this API. /// public void SetStylusPoints(StylusPointCollection stylusPoints) { // To modify the points we require Unmanaged code permission. SecurityHelper.DemandUnmanagedCode(); if (null == stylusPoints) { throw new ArgumentNullException("stylusPoints"); } if (!StylusPointDescription.AreCompatible( stylusPoints.Description, _report.StylusPointDescription)) { throw new ArgumentException(SR.Get(SRID.IncompatibleStylusPointDescriptions), "stylusPoints"); } if (stylusPoints.Count == 0) { throw new ArgumentException(SR.Get(SRID.Stylus_StylusPointsCantBeEmpty), "stylusPoints"); } _stylusPoints = stylusPoints.Clone(); } ////// Returns the RawStylusInputCustomDataList used to notify plugins before /// PreviewStylus event has been processed by application. /// public void NotifyWhenProcessed(object callbackData) { if (_currentNotifyPlugIn == null) { throw new InvalidOperationException(SR.Get(SRID.Stylus_CanOnlyCallForDownMoveOrUp)); } if (_customData == null) { _customData = new RawStylusInputCustomDataList(); } _customData.Add(new RawStylusInputCustomData(_currentNotifyPlugIn, callbackData)); } ////// True if a StylusPlugIn has modifiedthe StylusPoints. /// internal bool StylusPointsModified { get { return _stylusPoints != null; } } ////// Target StylusPlugInCollection that real time pen input sent to. /// internal StylusPlugInCollection Target { get { return _targetPlugInCollection; } } ////// Real RawStylusInputReport that this report is generated from. /// internal RawStylusInputReport Report { get { return _report; } } ////// Matrix that was used for rawstylusinput packets. /// internal GeneralTransform ElementTransform { get { return _tabletToElementTransform; } } ////// Retrieves the RawStylusInputCustomDataList associated with this input. /// internal RawStylusInputCustomDataList CustomDataList { get { if (_customData == null) { _customData = new RawStylusInputCustomDataList(); } return _customData; } } ////// StylusPlugIn that is adding a notify event. /// internal StylusPlugIn CurrentNotifyPlugIn { get { return _currentNotifyPlugIn; } set { _currentNotifyPlugIn = value; } } ///////////////////////////////////////////////////////////////////// RawStylusInputReport _report; GeneralTransform _tabletToElementTransform; StylusPlugInCollection _targetPlugInCollection; StylusPointCollection _stylusPoints; StylusPlugIn _currentNotifyPlugIn; RawStylusInputCustomDataList _customData; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
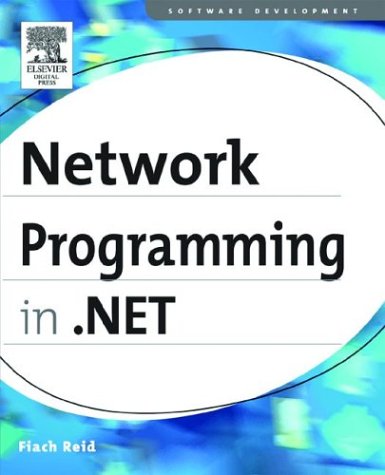
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LocatorManager.cs
- IdentifierCreationService.cs
- ProcessProtocolHandler.cs
- Zone.cs
- UpdateManifestForBrowserApplication.cs
- TableRowCollection.cs
- ConfigurationManager.cs
- OdbcErrorCollection.cs
- CategoryNameCollection.cs
- PlainXmlWriter.cs
- PanningMessageFilter.cs
- GuidelineCollection.cs
- LambdaCompiler.Generated.cs
- DataGridViewRowPrePaintEventArgs.cs
- DecimalAnimationUsingKeyFrames.cs
- BamlTreeMap.cs
- SystemUdpStatistics.cs
- PathFigure.cs
- XmlSchemaParticle.cs
- IndexedString.cs
- ChangeBlockUndoRecord.cs
- WebPartEditorOkVerb.cs
- KeyGesture.cs
- SystemUnicastIPAddressInformation.cs
- CodeSnippetExpression.cs
- RowUpdatedEventArgs.cs
- NamespaceQuery.cs
- TableItemPattern.cs
- NullRuntimeConfig.cs
- Renderer.cs
- BitmapEffectCollection.cs
- EventNotify.cs
- DesignBinding.cs
- HostProtectionException.cs
- LineInfo.cs
- MetadataItemSerializer.cs
- DataFieldConverter.cs
- _emptywebproxy.cs
- Page.cs
- ApplicationFileCodeDomTreeGenerator.cs
- RtfToXamlLexer.cs
- Vector3DAnimationUsingKeyFrames.cs
- RequestCacheValidator.cs
- DependencyObjectPropertyDescriptor.cs
- XmlElementCollection.cs
- DataContext.cs
- MouseDevice.cs
- RenameRuleObjectDialog.Designer.cs
- ExtendedPropertyDescriptor.cs
- UserPersonalizationStateInfo.cs
- SessionEndingCancelEventArgs.cs
- RouteValueExpressionBuilder.cs
- Composition.cs
- CustomErrorCollection.cs
- FragmentQuery.cs
- CompareValidator.cs
- PasswordPropertyTextAttribute.cs
- ColumnProvider.cs
- LifetimeServices.cs
- XamlContextStack.cs
- XmlSerializerOperationBehavior.cs
- DbUpdateCommandTree.cs
- FontWeights.cs
- DynamicRenderer.cs
- Mutex.cs
- SoapElementAttribute.cs
- MessageQueuePermissionAttribute.cs
- Avt.cs
- OptimalTextSource.cs
- ReadOnlyDictionary.cs
- XmlSchemaGroup.cs
- TreeViewDesigner.cs
- ToolStripItemDesigner.cs
- DbConnectionOptions.cs
- SQLBinary.cs
- SmtpDigestAuthenticationModule.cs
- FilterableData.cs
- StreamingContext.cs
- FrugalList.cs
- TraceData.cs
- ClientBuildManagerCallback.cs
- ArcSegment.cs
- CompilationLock.cs
- UndoManager.cs
- MaskedTextBoxTextEditor.cs
- X509CertificateValidationMode.cs
- DerivedKeyCachingSecurityTokenSerializer.cs
- RecordConverter.cs
- StickyNoteAnnotations.cs
- ApplicationServiceManager.cs
- TypeLibConverter.cs
- ZipIOCentralDirectoryBlock.cs
- Array.cs
- DesignerObjectListAdapter.cs
- CommonDialog.cs
- XsltFunctions.cs
- WindowsFormsSynchronizationContext.cs
- HijriCalendar.cs
- PictureBox.cs
- ApplicationProxyInternal.cs