Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Controls / PageRanges.cs / 1 / PageRanges.cs
/*++ Copyright (C) 2004 - 2005 Microsoft Corporation. All rights reserved. Module Name: PageRanges.cs Abstract: This file contains the implementation of the PageRange class and the PageRangeSelection enum for page range support in the dialog. Author: [....] ([....]) 9-May-2005 --*/ using System; using System.Globalization; using System.Windows; namespace System.Windows.Controls { ////// Enumeration of values for page range options. /// public enum PageRangeSelection { ////// All pages are printed. /// AllPages, ////// A set of user defined pages are printed. /// UserPages } ////// This class defines one single page range from /// a start page to an end page. /// public struct PageRange { #region Constructors ////// Constructs an instance of PageRange with one specified page. /// /// /// Single page of this page range. /// public PageRange( int page ) { _pageFrom = page; _pageTo = page; } ////// Constructs an instance of PageRange with specified values. /// /// /// Starting page of this range. /// /// /// Ending page of this range. /// public PageRange( int pageFrom, int pageTo ) { _pageFrom = pageFrom; _pageTo = pageTo; } #endregion Constructors #region Public properties ////// Gets or sets the start page of the page range. /// public int PageFrom { get { return _pageFrom; } set { _pageFrom = value; } } ////// Gets of sets the end page of the page range. /// public int PageTo { get { return _pageTo; } set { _pageTo = value; } } #endregion Public properties #region Private data private int _pageFrom; private int _pageTo; #endregion Private data #region Override methods ////// Converts this PageRange structure to its string representation. /// ////// A string value containing the range. /// public override string ToString( ) { string rangeText; if (_pageTo != _pageFrom) { rangeText = String.Format(CultureInfo.InvariantCulture, SR.Get(SRID.PrintDialogPageRange), _pageFrom, _pageTo); } else { rangeText = _pageFrom.ToString(CultureInfo.InvariantCulture); } return rangeText; } ////// Tests equality between this instance and the specified object. /// /// /// The object to compare this instance to. /// ////// True if obj is equal to this object, else false. /// Returns false if obj is not of type PageRange. /// public override bool Equals( object obj ) { if (obj == null || obj.GetType() != typeof(PageRange)) { return false; } return Equals((PageRange) obj); } ////// Tests equality between this instance and the specified page range. /// /// /// The page range to compare this instance to. /// ////// True if the page range is equal to this object, else false. /// public bool Equals( PageRange pageRange ) { return (pageRange.PageFrom == this.PageFrom) && (pageRange.PageTo == this.PageTo); } ////// Calculates a hash code for this PageRange. /// ////// Returns an integer hashcode for this instance. /// public override int GetHashCode() { return base.GetHashCode(); } ////// Test for equality. /// public static bool operator ==( PageRange pr1, PageRange pr2 ) { return pr1.Equals(pr2); } ////// Test for inequality. /// public static bool operator !=( PageRange pr1, PageRange pr2 ) { return !(pr1.Equals(pr2)); } #endregion Override methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
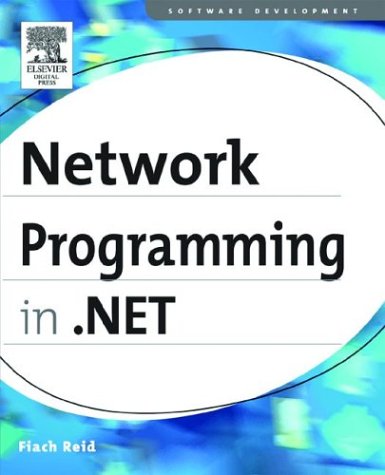
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FixedSOMTable.cs
- GenericNameHandler.cs
- SystemEvents.cs
- LocatorPartList.cs
- DataGridCommandEventArgs.cs
- _NegoState.cs
- PenContext.cs
- ComponentChangingEvent.cs
- HMACRIPEMD160.cs
- ActiveDocumentEvent.cs
- DbCommandTree.cs
- PostBackOptions.cs
- HtmlTableRow.cs
- PointIndependentAnimationStorage.cs
- PathSegmentCollection.cs
- GridViewDeletedEventArgs.cs
- Encoder.cs
- DataRow.cs
- SpeechAudioFormatInfo.cs
- CompositeFontFamily.cs
- HandledMouseEvent.cs
- BuildResult.cs
- LiteralControl.cs
- SmiEventSink_Default.cs
- FamilyMapCollection.cs
- Model3DGroup.cs
- EnvironmentPermission.cs
- XmlEncodedRawTextWriter.cs
- XmlWriterSettings.cs
- TraceContext.cs
- DataGridTextBoxColumn.cs
- TextMetrics.cs
- HttpModuleActionCollection.cs
- HtmlObjectListAdapter.cs
- XmlDesignerDataSourceView.cs
- IPHostEntry.cs
- CodeMemberField.cs
- AuthorizationRuleCollection.cs
- BreakSafeBase.cs
- HandlerElementCollection.cs
- InternalCache.cs
- TemplateFactory.cs
- CustomAttributeSerializer.cs
- TimeSpanValidator.cs
- RegistrySecurity.cs
- EntityDataSourceDesigner.cs
- Pen.cs
- XmlCodeExporter.cs
- FunctionMappingTranslator.cs
- DrawingContextDrawingContextWalker.cs
- DbConnectionPoolGroup.cs
- DesignRelation.cs
- StylusDevice.cs
- SHA384.cs
- ManagementNamedValueCollection.cs
- DragEvent.cs
- DiagnosticsConfigurationHandler.cs
- ScaleTransform3D.cs
- SchemaImporterExtension.cs
- DataViewSetting.cs
- ComponentCommands.cs
- TrackingExtract.cs
- ProfileSettings.cs
- ConstructorArgumentAttribute.cs
- CodeAttributeDeclaration.cs
- DetailsViewDeleteEventArgs.cs
- ThaiBuddhistCalendar.cs
- TdsEnums.cs
- BufferedResponseStream.cs
- PageVisual.cs
- ExpandCollapsePattern.cs
- OleDbInfoMessageEvent.cs
- Int32CollectionValueSerializer.cs
- DesignerActionTextItem.cs
- CancelRequestedQuery.cs
- WindowProviderWrapper.cs
- SettingsProperty.cs
- XamlTypeWithExplicitNamespace.cs
- UniqueConstraint.cs
- FactoryGenerator.cs
- Transaction.cs
- DefaultPropertyAttribute.cs
- NullEntityWrapper.cs
- FormClosedEvent.cs
- ArrayElementGridEntry.cs
- MarkupProperty.cs
- DataTableExtensions.cs
- GridViewCommandEventArgs.cs
- FileSystemEventArgs.cs
- GridView.cs
- XmlSecureResolver.cs
- DBConnectionString.cs
- MessageQueueCriteria.cs
- IgnoreSectionHandler.cs
- SuppressMessageAttribute.cs
- DmlSqlGenerator.cs
- CommandHelper.cs
- Rights.cs
- ClientTargetSection.cs
- PolyLineSegment.cs