Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / CompMod / System / ComponentModel / Design / ObjectSelectorEditor.cs / 1 / ObjectSelectorEditor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel.Design { using System.Design; using System.Runtime.Serialization.Formatters; using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Windows.Forms; using System.Drawing; using System.Windows.Forms.PropertyGridInternal; using System.Windows.Forms.Design; using System.Windows.Forms.ComponentModel; using Microsoft.Win32; using System.Drawing.Design; ////// /// /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1012:AbstractTypesShouldNotHaveConstructors")] public abstract class ObjectSelectorEditor : UITypeEditor { ////// /// public bool SubObjectSelector = false; ///[To be supplied.] ////// /// protected object prevValue = null; ///[To be supplied.] ////// /// protected object currValue = null; private Selector selector = null; // ///[To be supplied.] ////// /// public ObjectSelectorEditor() { } // ///[To be supplied.] ////// /// public ObjectSelectorEditor(bool subObjectSelector) { this.SubObjectSelector = subObjectSelector; } ///[To be supplied.] ////// /// [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] // everything in this assembly is full trust. public override object EditValue(ITypeDescriptorContext context, IServiceProvider provider, object value) { if (null != provider) { IWindowsFormsEditorService edSvc = (IWindowsFormsEditorService)provider.GetService(typeof(IWindowsFormsEditorService)); if (edSvc != null) { if (null == selector) { selector = new Selector(this); } prevValue = value; currValue = value; FillTreeWithData(selector, context, provider); selector.Start(edSvc, value); edSvc.DropDownControl(selector); selector.Stop(); if (prevValue != currValue) { value = currValue; } } } return value; } ////// /// [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] // everything in this assembly is full trust. public override UITypeEditorEditStyle GetEditStyle(ITypeDescriptorContext context) { return UITypeEditorEditStyle.DropDown; } // ////// /// public bool EqualsToValue(object value) { if (value == currValue) return true; else return false; } // ///[To be supplied.] ////// /// protected virtual void FillTreeWithData(Selector selector, ITypeDescriptorContext context, IServiceProvider provider) { selector.Clear(); } // // override this method to add validation code for new value // ///[To be supplied.] ////// /// public virtual void SetValue(object value) { this.currValue = value; } // // // ///[To be supplied.] ////// /// /// public class Selector : System.Windows.Forms.TreeView { // private ObjectSelectorEditor editor = null; private IWindowsFormsEditorService edSvc = null; ////// /// public bool clickSeen = false; // ///[To be supplied.] ////// /// public Selector(ObjectSelectorEditor editor) { CreateHandle(); this.editor = editor; this.BorderStyle = BorderStyle.None; this.FullRowSelect = !editor.SubObjectSelector; this.Scrollable = true; this.CheckBoxes = false; this.ShowPlusMinus = editor.SubObjectSelector; this.ShowLines = editor.SubObjectSelector; this.ShowRootLines = editor.SubObjectSelector; AfterSelect += new TreeViewEventHandler(this.OnAfterSelect); } // ///[To be supplied.] ////// /// public SelectorNode AddNode(string label, object value, SelectorNode parent) { SelectorNode newNode = new SelectorNode(label, value); if (parent != null) { parent.Nodes.Add(newNode); } else { Nodes.Add(newNode); } return newNode; } private bool ChooseSelectedNodeIfEqual() { if (editor != null && edSvc != null) { editor.SetValue(((SelectorNode)SelectedNode).value); if (editor.EqualsToValue(((SelectorNode)SelectedNode).value)) { edSvc.CloseDropDown(); return true; } } return false; } // ///[To be supplied.] ////// /// public void Clear() { clickSeen = false; Nodes.Clear(); } // ///[To be supplied.] ////// /// protected void OnAfterSelect(object sender, TreeViewEventArgs e) { if (clickSeen) { ChooseSelectedNodeIfEqual(); clickSeen = false; } } // ///[To be supplied.] ////// /// protected override void OnKeyDown(KeyEventArgs e) { Keys key = e.KeyCode; switch (key) { case Keys.Return: if (ChooseSelectedNodeIfEqual()) { e.Handled = true; } break; case Keys.Escape: editor.SetValue(editor.prevValue); e.Handled = true; edSvc.CloseDropDown(); break; } base.OnKeyDown(e); } ///[To be supplied.] ////// /// protected override void OnKeyPress(KeyPressEventArgs e) { switch (e.KeyChar) { case '\r': // Enter key e.Handled = true; break; } base.OnKeyPress(e); } ///[To be supplied.] ////// /// /// protected override void OnNodeMouseClick(TreeNodeMouseClickEventArgs e) { // we won't get an OnAfterSelect if it's already selected, so use this instead if (e.Node == SelectedNode) { ChooseSelectedNodeIfEqual(); } base.OnNodeMouseClick(e); } ///[To be supplied.] ////// /// public bool SetSelection(object value, System.Windows.Forms.TreeNodeCollection nodes) { TreeNode[] treeNodes; if (nodes == null) { treeNodes = new TreeNode[this.Nodes.Count]; this.Nodes.CopyTo(treeNodes, 0); } else { treeNodes = new TreeNode[nodes.Count]; nodes.CopyTo(treeNodes, 0); } int len = treeNodes.Length; if (len == 0) return false; for (int i=0; i[To be supplied.] ////// /// public void Start(IWindowsFormsEditorService edSvc, object value) { this.edSvc = edSvc; this.clickSeen = false; SetSelection(value, Nodes); } // ///[To be supplied.] ////// /// public void Stop() { this.edSvc = null; } // ///[To be supplied.] ////// /// [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] // everything in this assembly is full trust. protected override void WndProc(ref Message m) { switch (m.Msg) { case NativeMethods.WM_GETDLGCODE: m.Result = (IntPtr)((long)m.Result | NativeMethods.DLGC_WANTALLKEYS); return; case NativeMethods.WM_MOUSEMOVE: if (clickSeen) { clickSeen = false; } break; case NativeMethods.WM_REFLECT + NativeMethods.WM_NOTIFY: NativeMethods.NMTREEVIEW nmtv = (NativeMethods.NMTREEVIEW)Marshal.PtrToStructure(m.LParam, typeof(NativeMethods.NMTREEVIEW)); if (nmtv.nmhdr.code == NativeMethods.NM_CLICK) { clickSeen = true; } break; } base.WndProc(ref m); } } // ///[To be supplied.] ////// /// /// /// Suppressed because although the type implements ISerializable --its on the base class and this class /// is not modifying the stream to include its local information. Therefore, we should not publicly advertise this as /// Serializable unless explicitly required. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA2237:MarkISerializableTypesWithSerializable")] public class SelectorNode : System.Windows.Forms.TreeNode { ////// /// public object value = null; ///[To be supplied.] ////// /// public SelectorNode(string label, object value) : base (label) { this.value = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.[To be supplied.] ///
Link Menu
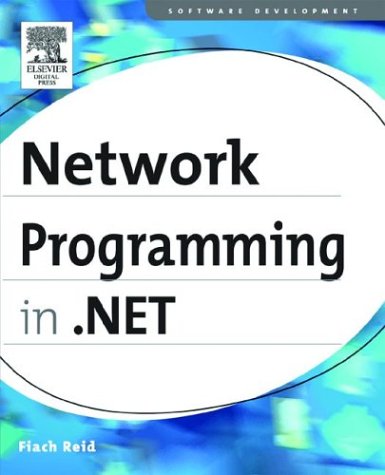
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- VariableQuery.cs
- SinglePageViewer.cs
- SchemaInfo.cs
- SemaphoreSecurity.cs
- DbConnectionHelper.cs
- ExpressionValueEditor.cs
- TextTreeTextElementNode.cs
- DataServiceQueryException.cs
- PageHandlerFactory.cs
- Matrix.cs
- TransportationConfigurationTypeInstallComponent.cs
- ICspAsymmetricAlgorithm.cs
- TextViewSelectionProcessor.cs
- NamedObject.cs
- EndpointAddress.cs
- CapabilitiesPattern.cs
- XmlSerializationWriter.cs
- ObjectViewEntityCollectionData.cs
- OleDbPermission.cs
- FillErrorEventArgs.cs
- OptimalBreakSession.cs
- Directory.cs
- JsonWriter.cs
- ChtmlImageAdapter.cs
- UnsafeNativeMethods.cs
- TextTreeExtractElementUndoUnit.cs
- SelectingProviderEventArgs.cs
- ValidationError.cs
- DataList.cs
- WebPartCatalogAddVerb.cs
- TableItemStyle.cs
- RegexCaptureCollection.cs
- PropertyGridCommands.cs
- LogWriteRestartAreaAsyncResult.cs
- PrtCap_Base.cs
- StringUtil.cs
- XamlPathDataSerializer.cs
- AtomMaterializerLog.cs
- VirtualizedContainerService.cs
- ZipArchive.cs
- DataGridViewAccessibleObject.cs
- CssTextWriter.cs
- PropertyEntry.cs
- TextWriterTraceListener.cs
- BindingNavigatorDesigner.cs
- EntitySqlException.cs
- DEREncoding.cs
- DES.cs
- PublisherMembershipCondition.cs
- ThaiBuddhistCalendar.cs
- QueryCacheKey.cs
- DescendantBaseQuery.cs
- BamlRecordReader.cs
- SrgsOneOf.cs
- ButtonStandardAdapter.cs
- ColorDialog.cs
- OperationPickerDialog.cs
- FileLevelControlBuilderAttribute.cs
- Base64Stream.cs
- SecurityPermission.cs
- DataObjectCopyingEventArgs.cs
- PeerNameRecordCollection.cs
- PageWrapper.cs
- SymbolEqualComparer.cs
- ResolvePPIDRequest.cs
- CacheChildrenQuery.cs
- ConvertBinder.cs
- HandoffBehavior.cs
- RequestContext.cs
- DSACryptoServiceProvider.cs
- ServiceOperation.cs
- RelationshipConstraintValidator.cs
- SocketElement.cs
- CommentEmitter.cs
- RuleSettings.cs
- Unit.cs
- HyperLinkField.cs
- MappingModelBuildProvider.cs
- XslAst.cs
- DetailsViewCommandEventArgs.cs
- DataControlButton.cs
- ImageSource.cs
- RequestResizeEvent.cs
- Propagator.JoinPropagator.cs
- FrameworkName.cs
- UnicastIPAddressInformationCollection.cs
- Debug.cs
- ThicknessConverter.cs
- EntityDataSourceDataSelection.cs
- TextElementEditingBehaviorAttribute.cs
- LicenseException.cs
- ConfigXmlText.cs
- ClientUrlResolverWrapper.cs
- unitconverter.cs
- jithelpers.cs
- SerializationInfo.cs
- OpacityConverter.cs
- TemplateInstanceAttribute.cs
- UnhandledExceptionEventArgs.cs
- Visual3DCollection.cs