Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Documents / StaticTextPointer.cs / 1 / StaticTextPointer.cs
//---------------------------------------------------------------------------- // // File: StaticTextPointer.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using System; using MS.Internal; using System.Threading; using System.Windows; using System.Collections; internal struct StaticTextPointer { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors internal StaticTextPointer(ITextContainer textContainer, object handle0) : this(textContainer, handle0, 0) { } internal StaticTextPointer(ITextContainer textContainer, object handle0, int handle1) { _textContainer = textContainer; _handle0 = handle0; _handle1 = handle1; } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal ITextPointer CreateDynamicTextPointer(LogicalDirection direction) { return _textContainer.CreateDynamicTextPointer(this, direction); } internal TextPointerContext GetPointerContext(LogicalDirection direction) { return _textContainer.GetPointerContext(this, direction); } internal int GetOffsetToPosition(StaticTextPointer position) { return _textContainer.GetOffsetToPosition(this, position); } internal int GetTextInRun(LogicalDirection direction, char[] textBuffer, int startIndex, int count) { return _textContainer.GetTextInRun(this, direction, textBuffer, startIndex, count); } internal object GetAdjacentElement(LogicalDirection direction) { return _textContainer.GetAdjacentElement(this, direction); } internal StaticTextPointer CreatePointer(int offset) { return _textContainer.CreatePointer(this, offset); } internal StaticTextPointer GetNextContextPosition(LogicalDirection direction) { return _textContainer.GetNextContextPosition(this, direction); } internal int CompareTo(StaticTextPointer position) { return _textContainer.CompareTo(this, position); } internal int CompareTo(ITextPointer position) { return _textContainer.CompareTo(this, position); } internal object GetValue(DependencyProperty formattingProperty) { return _textContainer.GetValue(this, formattingProperty); } internal static StaticTextPointer Min(StaticTextPointer position1, StaticTextPointer position2) { return position1.CompareTo(position2) <= 0 ? position1 : position2; } internal static StaticTextPointer Max(StaticTextPointer position1, StaticTextPointer position2) { return position1.CompareTo(position2) >= 0 ? position1 : position2; } #endregion Internal Methods //------------------------------------------------------ // // Internal Properties // //------------------------------------------------------ #region Internal Properties internal ITextContainer TextContainer { get { return _textContainer; } } internal DependencyObject Parent { get { return _textContainer.GetParent(this); } } internal bool IsNull { get { return (_textContainer == null); } } internal object Handle0 { get { return _handle0; } } internal int Handle1 { get { return _handle1; } } #endregion Internal Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal static StaticTextPointer Null = new StaticTextPointer(null, null, 0); #endregion Internal Fields //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private readonly ITextContainer _textContainer; private readonly object _handle0; private readonly int _handle1; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: StaticTextPointer.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using System; using MS.Internal; using System.Threading; using System.Windows; using System.Collections; internal struct StaticTextPointer { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors internal StaticTextPointer(ITextContainer textContainer, object handle0) : this(textContainer, handle0, 0) { } internal StaticTextPointer(ITextContainer textContainer, object handle0, int handle1) { _textContainer = textContainer; _handle0 = handle0; _handle1 = handle1; } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal ITextPointer CreateDynamicTextPointer(LogicalDirection direction) { return _textContainer.CreateDynamicTextPointer(this, direction); } internal TextPointerContext GetPointerContext(LogicalDirection direction) { return _textContainer.GetPointerContext(this, direction); } internal int GetOffsetToPosition(StaticTextPointer position) { return _textContainer.GetOffsetToPosition(this, position); } internal int GetTextInRun(LogicalDirection direction, char[] textBuffer, int startIndex, int count) { return _textContainer.GetTextInRun(this, direction, textBuffer, startIndex, count); } internal object GetAdjacentElement(LogicalDirection direction) { return _textContainer.GetAdjacentElement(this, direction); } internal StaticTextPointer CreatePointer(int offset) { return _textContainer.CreatePointer(this, offset); } internal StaticTextPointer GetNextContextPosition(LogicalDirection direction) { return _textContainer.GetNextContextPosition(this, direction); } internal int CompareTo(StaticTextPointer position) { return _textContainer.CompareTo(this, position); } internal int CompareTo(ITextPointer position) { return _textContainer.CompareTo(this, position); } internal object GetValue(DependencyProperty formattingProperty) { return _textContainer.GetValue(this, formattingProperty); } internal static StaticTextPointer Min(StaticTextPointer position1, StaticTextPointer position2) { return position1.CompareTo(position2) <= 0 ? position1 : position2; } internal static StaticTextPointer Max(StaticTextPointer position1, StaticTextPointer position2) { return position1.CompareTo(position2) >= 0 ? position1 : position2; } #endregion Internal Methods //------------------------------------------------------ // // Internal Properties // //------------------------------------------------------ #region Internal Properties internal ITextContainer TextContainer { get { return _textContainer; } } internal DependencyObject Parent { get { return _textContainer.GetParent(this); } } internal bool IsNull { get { return (_textContainer == null); } } internal object Handle0 { get { return _handle0; } } internal int Handle1 { get { return _handle1; } } #endregion Internal Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal static StaticTextPointer Null = new StaticTextPointer(null, null, 0); #endregion Internal Fields //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private readonly ITextContainer _textContainer; private readonly object _handle0; private readonly int _handle1; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
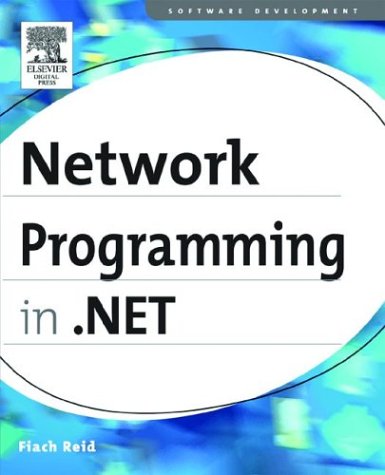
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TemporaryBitmapFile.cs
- SettingsSection.cs
- AssemblyNameProxy.cs
- ReadOnlyCollectionBuilder.cs
- GridView.cs
- TokenBasedSetEnumerator.cs
- PassportAuthenticationEventArgs.cs
- ContractMethodInfo.cs
- SqlDataSourceConfigureSortForm.cs
- SqlUserDefinedTypeAttribute.cs
- CacheHelper.cs
- RuntimeComponentFilter.cs
- DataMisalignedException.cs
- AppDomainUnloadedException.cs
- SafeBitVector32.cs
- CompressedStack.cs
- ScriptingProfileServiceSection.cs
- MediaElement.cs
- FlowDocumentPaginator.cs
- EmptyReadOnlyDictionaryInternal.cs
- KeyTime.cs
- CatalogPartCollection.cs
- _NegoStream.cs
- COM2TypeInfoProcessor.cs
- BindingBase.cs
- TrustManagerPromptUI.cs
- RemoteWebConfigurationHostServer.cs
- AuthenticationSection.cs
- Accessors.cs
- DiagnosticsElement.cs
- ConfigurationSection.cs
- ClientSession.cs
- EventWaitHandle.cs
- Brushes.cs
- SetStateEventArgs.cs
- CqlIdentifiers.cs
- RightsManagementPermission.cs
- Decimal.cs
- AttributeProviderAttribute.cs
- XmlDataSourceNodeDescriptor.cs
- GroupItem.cs
- SecurityUtils.cs
- DynamicDataRoute.cs
- Util.cs
- QueryParameter.cs
- ProfileProvider.cs
- TextContainerHelper.cs
- XsdDateTime.cs
- ClrPerspective.cs
- WebPartMenuStyle.cs
- AuthorizationSection.cs
- DetailsViewUpdatedEventArgs.cs
- XPathDocumentBuilder.cs
- PointKeyFrameCollection.cs
- arabicshape.cs
- AnnotationHelper.cs
- DataGridViewRowHeaderCell.cs
- DesignerSerializationOptionsAttribute.cs
- SmiGettersStream.cs
- CodeDirectionExpression.cs
- HMAC.cs
- FragmentQueryProcessor.cs
- EnumBuilder.cs
- ByteStream.cs
- NodeFunctions.cs
- TextRunProperties.cs
- Line.cs
- GeometryGroup.cs
- EncryptionUtility.cs
- DataControlFieldHeaderCell.cs
- TdsParserSafeHandles.cs
- WebRequestModuleElement.cs
- OleDbCommand.cs
- HttpWriter.cs
- SystemColorTracker.cs
- AddInProcess.cs
- FloaterBaseParaClient.cs
- assemblycache.cs
- ListView.cs
- SafeLibraryHandle.cs
- StorageRoot.cs
- ProviderCommandInfoUtils.cs
- CodeGeneratorAttribute.cs
- MyContact.cs
- HtmlElementErrorEventArgs.cs
- SiteMap.cs
- LocalIdKeyIdentifierClause.cs
- XmlHelper.cs
- SRGSCompiler.cs
- HtmlLink.cs
- DockPattern.cs
- SchemaAttDef.cs
- GlyphInfoList.cs
- SyndicationElementExtensionCollection.cs
- TimeSpanParse.cs
- InternalPolicyElement.cs
- LoginDesignerUtil.cs
- Tablet.cs
- ListBoxAutomationPeer.cs
- QilScopedVisitor.cs