Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / SapiAttributeParser.cs / 1 / SapiAttributeParser.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.Collections.Generic; using System.Globalization; using System.Speech.Internal.SapiInterop; #if !SPEECHSERVER using System.Speech.AudioFormat; #endif namespace System.Speech.Internal { internal static class SapiAttributeParser { //******************************************************************* // // Internal Methods // //******************************************************************* #region Internal Methods static internal CultureInfo GetCultureInfoFromLanguageString (string valueString) { string [] strings = valueString.Split (';'); string langStringTrim = strings [0].Trim (); if (!string.IsNullOrEmpty (langStringTrim)) { try { return new CultureInfo (Int32.Parse (langStringTrim, NumberStyles.HexNumber, CultureInfo.InvariantCulture), false); } catch (ArgumentException) { return null; // If we have an invalid language id ignore it. Otherwise enumerating recognizers or voices would fail. } } return null; } #if !SPEECHSERVER static internal ListGetAudioFormatsFromString(string valueString) { List formatList = new List (); string [] strings = valueString.Split (';'); for (int i = 0; i < strings.Length; i++) { string formatString = strings [i].Trim (); if (!string.IsNullOrEmpty (formatString)) { SpeechAudioFormatInfo formatInfo = AudioFormatConverter.ToSpeechAudioFormatInfo (formatString); if (formatInfo != null) // Skip cases where a Guid is used. { formatList.Add (formatInfo); } } } return formatList; } #endif #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.Collections.Generic; using System.Globalization; using System.Speech.Internal.SapiInterop; #if !SPEECHSERVER using System.Speech.AudioFormat; #endif namespace System.Speech.Internal { internal static class SapiAttributeParser { //******************************************************************* // // Internal Methods // //******************************************************************* #region Internal Methods static internal CultureInfo GetCultureInfoFromLanguageString (string valueString) { string [] strings = valueString.Split (';'); string langStringTrim = strings [0].Trim (); if (!string.IsNullOrEmpty (langStringTrim)) { try { return new CultureInfo (Int32.Parse (langStringTrim, NumberStyles.HexNumber, CultureInfo.InvariantCulture), false); } catch (ArgumentException) { return null; // If we have an invalid language id ignore it. Otherwise enumerating recognizers or voices would fail. } } return null; } #if !SPEECHSERVER static internal ListGetAudioFormatsFromString(string valueString) { List formatList = new List (); string [] strings = valueString.Split (';'); for (int i = 0; i < strings.Length; i++) { string formatString = strings [i].Trim (); if (!string.IsNullOrEmpty (formatString)) { SpeechAudioFormatInfo formatInfo = AudioFormatConverter.ToSpeechAudioFormatInfo (formatString); if (formatInfo != null) // Skip cases where a Guid is used. { formatList.Add (formatInfo); } } } return formatList; } #endif #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
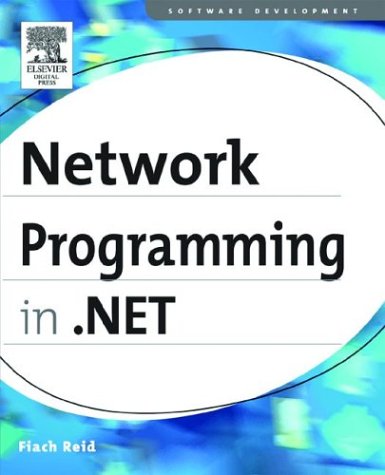
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ListViewItemMouseHoverEvent.cs
- ContextQuery.cs
- MsmqChannelListenerBase.cs
- BaseCAMarshaler.cs
- followingquery.cs
- TextLineBreak.cs
- PermissionSetTriple.cs
- UnsafeNativeMethodsMilCoreApi.cs
- Permission.cs
- StateItem.cs
- metadatamappinghashervisitor.cs
- XmlDataSource.cs
- ProtocolsSection.cs
- DescendantBaseQuery.cs
- DockAndAnchorLayout.cs
- _HTTPDateParse.cs
- SqlParameterCollection.cs
- WorkflowTransactionOptions.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- WebPartConnectionsEventArgs.cs
- RotateTransform.cs
- SvcMapFile.cs
- TriggerActionCollection.cs
- PropertyTabAttribute.cs
- LabelDesigner.cs
- DesignerActionUIStateChangeEventArgs.cs
- CollectionView.cs
- DataGridViewCellContextMenuStripNeededEventArgs.cs
- TraceInternal.cs
- RegisteredArrayDeclaration.cs
- EventDescriptor.cs
- Authorization.cs
- NetworkStream.cs
- FormatStringEditor.cs
- ScrollEventArgs.cs
- EventLogLink.cs
- Point4D.cs
- WindowProviderWrapper.cs
- CommentEmitter.cs
- CodeArrayCreateExpression.cs
- HttpInputStream.cs
- ChangeDirector.cs
- CachedFontFace.cs
- RangeBase.cs
- DocumentAutomationPeer.cs
- Transform.cs
- DecoderBestFitFallback.cs
- StringInfo.cs
- BamlLocalizabilityResolver.cs
- DefaultValueConverter.cs
- VersionPair.cs
- ThreadExceptionEvent.cs
- ServiceNameElement.cs
- SearchExpression.cs
- IndependentlyAnimatedPropertyMetadata.cs
- BufferModesCollection.cs
- ObjectParameter.cs
- ClientBuildManagerCallback.cs
- DataTemplateSelector.cs
- SectionInput.cs
- RangeValidator.cs
- mda.cs
- Mapping.cs
- DropShadowEffect.cs
- EventDescriptor.cs
- ViewManager.cs
- PropertyDescriptor.cs
- MsmqIntegrationValidationBehavior.cs
- ZipIOFileItemStream.cs
- AttributeCollection.cs
- ToolStripItemCollection.cs
- InternalCache.cs
- X509Chain.cs
- CSharpCodeProvider.cs
- Lease.cs
- HtmlFormWrapper.cs
- _OverlappedAsyncResult.cs
- AutomationPropertyInfo.cs
- EditingScopeUndoUnit.cs
- CompiledQueryCacheEntry.cs
- HiddenField.cs
- Operator.cs
- ForceCopyBuildProvider.cs
- FamilyMapCollection.cs
- TextInfo.cs
- TextLineResult.cs
- NativeMethods.cs
- Html32TextWriter.cs
- TypeLoadException.cs
- RMPermissions.cs
- TemplateContent.cs
- ReadOnlyCollection.cs
- AttributeParameterInfo.cs
- EmptyElement.cs
- Clock.cs
- SymbolMethod.cs
- LocatorManager.cs
- PropertyPathWorker.cs
- PerformanceCounterPermission.cs
- DataBoundLiteralControl.cs