Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / DataOracleClient / System / Data / OracleClient / AdapterUtil.cs / 1 / AdapterUtil.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- // SQLBUDT #360734 - [....] tells us that using this attribute makes // ngen images smaller and more efficient, if we always load both of // these assemblies together, which we do. using System.Runtime.CompilerServices; [assembly:DependencyAttribute("System.Data,", LoadHint.Always)] namespace System.Data.Common { using Microsoft.Win32; using System; using System.Collections; using System.ComponentModel; using System.Configuration; using System.Data; using System.Data.OracleClient; using System.Data.ProviderBase; using System.Data.SqlTypes; using System.Diagnostics; using System.Globalization; using System.IO; using System.Reflection; using System.Runtime.InteropServices; using System.Runtime.Serialization; using System.Security; using System.Security.Permissions; using System.Text; using System.Threading; using SysTx = System.Transactions; #if DEBUG using System.Data.OleDb; using System.Data.SqlClient; #endif sealed internal class ADP { // internal const string Parameter = "Parameter"; internal const string ParameterName = "ParameterName"; // static internal int SrcCompare(string strA, string strB) { // this is null safe return ((strA == strB) ? 0 : 1); } static internal int DstCompare(string strA, string strB) { // this is null safe return CultureInfo.CurrentCulture.CompareInfo.Compare(strA, strB, ADP.compareOptions); } static private string ConnectionStateMsg(ConnectionState state) { // MDAC 82165, if the ConnectionState enum to msg the localization looks weird switch(state) { case (ConnectionState.Closed): case (ConnectionState.Connecting|ConnectionState.Broken): // treated the same as closed return Res.GetString(Res.ADP_ConnectionStateMsg_Closed); case (ConnectionState.Connecting): return Res.GetString(Res.ADP_ConnectionStateMsg_Connecting); case (ConnectionState.Open): return Res.GetString(Res.ADP_ConnectionStateMsg_Open); case (ConnectionState.Open|ConnectionState.Executing): return Res.GetString(Res.ADP_ConnectionStateMsg_OpenExecuting); case (ConnectionState.Open|ConnectionState.Fetching): return Res.GetString(Res.ADP_ConnectionStateMsg_OpenFetching); default: return Res.GetString(Res.ADP_ConnectionStateMsg, state.ToString()); } } static internal readonly bool IsWindowsNT = (PlatformID.Win32NT == Environment.OSVersion.Platform); static internal readonly bool IsPlatformNT5 = (ADP.IsWindowsNT && (Environment.OSVersion.Version.Major >= 5)); static internal void CheckArgumentLength(string value, string parameterName) { CheckArgumentNull(value, parameterName); if (0 == value.Length) { throw Argument(Res.GetString(Res.ADP_EmptyString, parameterName)); // MDAC 94859 } } static internal bool CompareInsensitiveInvariant(string strvalue, string strconst) { return (0 == CultureInfo.InvariantCulture.CompareInfo.Compare(strvalue, strconst, CompareOptions.IgnoreCase)); } static internal bool IsEmptyArray(string[] array) { return ((null == array) || (0 == array.Length)); } static internal Exception CollectionNullValue(string parameter, Type collection, Type itemType) { return ArgumentNull(parameter, Res.GetString(Res.ADP_CollectionNullValue, collection.Name, itemType.Name)); } static internal Exception CollectionIndexInt32(int index, Type collection, int count) { return IndexOutOfRange(Res.GetString(Res.ADP_CollectionIndexInt32, index.ToString(CultureInfo.InvariantCulture), collection.Name, count.ToString(CultureInfo.InvariantCulture))); } static internal Exception CollectionIndexString(Type itemType, string propertyName, string propertyValue, Type collection) { return IndexOutOfRange(Res.GetString(Res.ADP_CollectionIndexString, itemType.Name, propertyName, propertyValue, collection.Name)); } static internal Exception CollectionInvalidType(Type collection, Type itemType, object invalidValue) { return InvalidCast(Res.GetString(Res.ADP_CollectionInvalidType, collection.Name, itemType.Name, invalidValue.GetType().Name)); } static internal ArgumentException CollectionRemoveInvalidObject(Type itemType, ICollection collection) { return Argument(Res.GetString(Res.ADP_CollectionRemoveInvalidObject, itemType.Name, collection.GetType().Name)); // MDAC 68201 } static internal Exception ConnectionAlreadyOpen(ConnectionState state) { return InvalidOperation(Res.GetString(Res.ADP_ConnectionAlreadyOpen, ADP.ConnectionStateMsg(state))); } static internal ArgumentException ConnectionStringSyntax(int index) { return Argument(Res.GetString(Res.ADP_ConnectionStringSyntax, index)); } static internal Exception InvalidDataDirectory() { return ADP.InvalidOperation(Res.GetString(Res.ADP_InvalidDataDirectory)); } static internal ArgumentException InvalidKeyname(string parameterName) { return Argument(Res.GetString(Res.ADP_InvalidKey), parameterName); } static internal ArgumentException InvalidValue(string parameterName) { return Argument(Res.GetString(Res.ADP_InvalidValue), parameterName); } static internal Exception DataReaderClosed(string method) { return InvalidOperation(Res.GetString(Res.ADP_DataReaderClosed, method)); } static internal Exception InvalidXMLBadVersion() { return Argument(Res.GetString(Res.ADP_InvalidXMLBadVersion)); } static internal Exception NotAPermissionElement() { return Argument(Res.GetString(Res.ADP_NotAPermissionElement)); } static internal Exception PermissionTypeMismatch() { return Argument(Res.GetString(Res.ADP_PermissionTypeMismatch)); } internal enum ConnectionError { BeginGetConnectionReturnsNull, GetConnectionReturnsNull, ConnectionOptionsMissing, CouldNotSwitchToClosedPreviouslyOpenedState, } static internal Exception InternalConnectionError(ConnectionError internalError) { return InvalidOperation(Res.GetString(Res.ADP_InternalConnectionError, (int)internalError)); } internal enum InternalErrorCode { UnpooledObjectHasOwner = 0, UnpooledObjectHasWrongOwner = 1, PushingObjectSecondTime = 2, PooledObjectHasOwner = 3, PooledObjectInPoolMoreThanOnce = 4, CreateObjectReturnedNull = 5, NewObjectCannotBePooled = 6, NonPooledObjectUsedMoreThanOnce = 7, AttemptingToPoolOnRestrictedToken = 8, // ConnectionOptionsInUse = 9, ConvertSidToStringSidWReturnedNull = 10, // UnexpectedTransactedObject = 11, AttemptingToConstructReferenceCollectionOnStaticObject = 12, AttemptingToEnlistTwice = 13, CreateReferenceCollectionReturnedNull = 14, PooledObjectWithoutPool = 15, UnexpectedWaitAnyResult = 16, NameValuePairNext = 20, InvalidParserState1 = 21, InvalidParserState2 = 22, InvalidBuffer = 30, InvalidLongBuffer = 31, InvalidNumberOfRows = 32, } static internal Exception InternalError(InternalErrorCode internalError) { return InvalidOperation(Res.GetString(Res.ADP_InternalProviderError, (int)internalError)); } static internal Exception InvalidConnectionOptionValue(string key, Exception inner) { return Argument(Res.GetString(Res.ADP_InvalidConnectionOptionValue, key), inner); } static internal Exception InvalidEnumerationValue(Type type, int value) { return ADP.ArgumentOutOfRange(Res.GetString(Res.ADP_InvalidEnumerationValue, type.Name, value.ToString(System.Globalization.CultureInfo.InvariantCulture)), type.Name); } // IDataParameter.SourceVersion static internal Exception InvalidDataRowVersion(DataRowVersion value) { #if DEBUG switch(value) { case DataRowVersion.Default: case DataRowVersion.Current: case DataRowVersion.Original: case DataRowVersion.Proposed: Debug.Assert(false, "valid DataRowVersion " + value.ToString()); break; } #endif return InvalidEnumerationValue(typeof(DataRowVersion), (int) value); } // DBDataPermissionAttribute.KeyRestrictionBehavior static internal Exception InvalidKeyRestrictionBehavior(KeyRestrictionBehavior value) { #if DEBUG switch(value) { case KeyRestrictionBehavior.PreventUsage: case KeyRestrictionBehavior.AllowOnly: Debug.Assert(false, "valid KeyRestrictionBehavior " + value.ToString()); break; } #endif return InvalidEnumerationValue(typeof(KeyRestrictionBehavior), (int) value); } static internal Exception InvalidOffsetValue(int value) { return Argument(Res.GetString(Res.ADP_InvalidOffsetValue, value.ToString(CultureInfo.InvariantCulture))); } static internal Exception InvalidParameterDirection(ParameterDirection value) { #if DEBUG switch(value) { case ParameterDirection.Input: case ParameterDirection.Output: case ParameterDirection.InputOutput: case ParameterDirection.ReturnValue: Debug.Assert(false, "valid ParameterDirection " + value.ToString()); break; } #endif return InvalidEnumerationValue(typeof(ParameterDirection), (int) value); } static internal Exception InvalidParameterType(IDataParameterCollection collection, Type parameterType, object invalidValue) { return CollectionInvalidType(collection.GetType(), parameterType, invalidValue); } static internal Exception InvalidPermissionState(PermissionState value) { #if DEBUG switch(value) { case PermissionState.Unrestricted: case PermissionState.None: Debug.Assert(false, "valid PermissionState " + value.ToString()); break; } #endif return InvalidEnumerationValue(typeof(PermissionState), (int) value); } // IDbCommand.UpdateRowSource static internal Exception InvalidUpdateRowSource(UpdateRowSource value) { #if DEBUG switch(value) { case UpdateRowSource.None: case UpdateRowSource.OutputParameters: case UpdateRowSource.FirstReturnedRecord: case UpdateRowSource.Both: Debug.Assert(false, "valid UpdateRowSource " + value.ToString()); break; } #endif return InvalidEnumerationValue(typeof(UpdateRowSource), (int) value); } static internal Exception MethodNotImplemented(string methodName) { NotImplementedException e = new NotImplementedException(methodName); TraceExceptionAsReturnValue(e); return e; } static internal Exception NoConnectionString() { return InvalidOperation(Res.GetString(Res.ADP_NoConnectionString)); } static internal Exception ParameterNull(string parameter, IDataParameterCollection collection, Type parameterType) { return CollectionNullValue(parameter, collection.GetType(), parameterType); } static internal Exception ParametersIsNotParent(Type parameterType, IDataParameterCollection collection) { return Argument(Res.GetString(Res.ADP_CollectionIsNotParent, parameterType.Name, collection.GetType().Name)); } static internal ArgumentException ParametersIsParent(Type parameterType, IDataParameterCollection collection) { return Argument(Res.GetString(Res.ADP_CollectionIsNotParent, parameterType.Name, collection.GetType().Name)); } static internal Exception ParametersMappingIndex(int index, IDataParameterCollection collection) { return CollectionIndexInt32(index, collection.GetType(), collection.Count); } static internal Exception ParametersSourceIndex(string parameterName, IDataParameterCollection collection, Type parameterType) { return CollectionIndexString(parameterType, ADP.ParameterName, parameterName, collection.GetType()); } static internal Exception PooledOpenTimeout() { return ADP.InvalidOperation(Res.GetString(Res.ADP_PooledOpenTimeout)); } static internal Exception OpenConnectionPropertySet(string property, ConnectionState state) { return InvalidOperation(Res.GetString(Res.ADP_OpenConnectionPropertySet, property, ADP.ConnectionStateMsg(state))); } static internal Exception AmbigousCollectionName(string collectionName) { return Argument(Res.GetString(Res.MDF_AmbigousCollectionName,collectionName)); } static internal Exception CollectionNameIsNotUnique(string collectionName) { return Argument(Res.GetString(Res.MDF_CollectionNameISNotUnique,collectionName)); } static internal Exception DataTableDoesNotExist(string collectionName) { return Argument(Res.GetString(Res.MDF_DataTableDoesNotExist,collectionName)); } static internal Exception IncorrectNumberOfDataSourceInformationRows() { return Argument(Res.GetString(Res.MDF_IncorrectNumberOfDataSourceInformationRows)); } static internal Exception InvalidXml() { return Argument(Res.GetString(Res.MDF_InvalidXml)); } static internal Exception InvalidXmlMissingColumn(string collectionName, string columnName) { return Argument(Res.GetString(Res.MDF_InvalidXmlMissingColumn, collectionName, columnName)); } static internal Exception InvalidXmlInvalidValue(string collectionName, string columnName) { return Argument(Res.GetString(Res.MDF_InvalidXmlInvalidValue, collectionName, columnName)); } static internal Exception MissingDataSourceInformationColumn() { return Argument(Res.GetString(Res.MDF_MissingDataSourceInformationColumn)); } static internal Exception MissingRestrictionColumn() { return Argument(Res.GetString(Res.MDF_MissingRestrictionColumn)); } static internal Exception MissingRestrictionRow() { return Argument(Res.GetString(Res.MDF_MissingRestrictionRow)); } static internal Exception NoColumns() { return Argument(Res.GetString(Res.MDF_NoColumns)); } static internal Exception QueryFailed(string collectionName, Exception e) { return InvalidOperation(Res.GetString(Res.MDF_QueryFailed,collectionName), e); } static internal Exception TooManyRestrictions(string collectionName) { return Argument(Res.GetString(Res.MDF_TooManyRestrictions,collectionName)); } static internal Exception UndefinedCollection(string collectionName) { return Argument(Res.GetString(Res.MDF_UndefinedCollection,collectionName)); } static internal Exception UndefinedPopulationMechanism(string populationMechanism) { return Argument(Res.GetString(Res.MDF_UndefinedPopulationMechanism,populationMechanism)); } static internal Exception UnsupportedVersion(string collectionName) { return Argument(Res.GetString(Res.MDF_UnsupportedVersion,collectionName)); } ////////////////////////////// //// END OF COMMON CODE STUFF ////////////////////////////// // The class ADP defines the exceptions that are specific to the Adapters. private ADP() { } // only StackOverflowException & ThreadAbortException are sealed classes static private readonly Type StackOverflowType = typeof(StackOverflowException); static private readonly Type OutOfMemoryType = typeof(OutOfMemoryException); static private readonly Type ThreadAbortType = typeof(ThreadAbortException); static private readonly Type NullReferenceType = typeof(NullReferenceException); static private readonly Type AccessViolationType = typeof(AccessViolationException); static private readonly Type SecurityType = typeof(SecurityException); static internal readonly Type ArgumentNullExceptionType = typeof(ArgumentNullException); static internal readonly Type FormatExceptionType = typeof(FormatException); static internal readonly Type OverflowExceptionType = typeof(OverflowException); // The class contains functions that take the proper informational variables and then construct // the appropriate exception with an error string obtained from the resource Framework.txt. // The exception is then returned to the caller, so that the caller may then throw from its // location so that the catcher of the exception will have the appropriate call stack. // This class is used so that there will be compile time checking of error messages. // The resource Framework.txt will ensure proper string text based on the appropriate // locale. //////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////// // // Traced Exception Constructors // //////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////// static private void TraceException(string trace, Exception e) { Debug.Assert(null != e, "TraceException: null Exception"); if (null != e) { Bid.Trace(trace, e.ToString()); // will include callstack if permission is available } } static internal Exception TraceException(Exception e) { TraceExceptionAsReturnValue(e); return e; } static internal void TraceExceptionAsReturnValue(Exception e) { TraceException("'%ls'\n", e); } static internal void TraceExceptionForCapture(Exception e) { Debug.Assert(ADP.IsCatchableExceptionType(e), "Invalid exception type, should have been re-thrown!"); TraceException(" '%ls'\n", e); } static internal void TraceExceptionWithoutRethrow(Exception e) { Debug.Assert(ADP.IsCatchableExceptionType(e), "Invalid exception type, should have been re-thrown!"); TraceException(" '%ls'\n", e); } static internal ArgumentException Argument(string error) { ArgumentException e = new ArgumentException(error); TraceExceptionAsReturnValue(e); return e; } static internal ArgumentException Argument(string error, string parameter) { ArgumentException e = new ArgumentException(error, parameter); TraceExceptionAsReturnValue(e); return e; } static internal ArgumentException Argument(string error, Exception inner) { ArgumentException e = new ArgumentException(error, inner); TraceExceptionAsReturnValue(e); return e; } static internal ArgumentNullException ArgumentNull(string parameter) { ArgumentNullException e = new ArgumentNullException(parameter); TraceExceptionAsReturnValue(e); return e; } static internal ArgumentNullException ArgumentNull(string parameter, string error) { ArgumentNullException e = new ArgumentNullException(parameter, error); TraceExceptionAsReturnValue(e); return e; } static internal ArgumentOutOfRangeException ArgumentOutOfRange(string argName, string message) { ArgumentOutOfRangeException e = new ArgumentOutOfRangeException(argName, message); TraceExceptionAsReturnValue(e); return e; } static internal ConfigurationException Configuration(string message) { ConfigurationException e = new ConfigurationErrorsException(message); TraceExceptionAsReturnValue(e); return e; } static internal Exception ProviderException(string error) { return InvalidOperation(error); } static internal Exception IndexOutOfRange(string error) { return TraceException(new IndexOutOfRangeException(error)); } static internal Exception InvalidCast() { return TraceException(new InvalidCastException()); } static internal Exception InvalidCast(string error) { return TraceException(new InvalidCastException(error)); } static internal Exception InvalidOperation(string error) { return TraceException(new InvalidOperationException(error)); } static internal Exception InvalidOperation(string error, Exception inner) { return TraceException(new InvalidOperationException(error, inner)); } static internal Exception NotSupported() { return TraceException(new NotSupportedException()); } static internal Exception NotSupported(string message) { return TraceException(new NotSupportedException(message)); } static internal Exception ObjectDisposed(string name) { return TraceException(new ObjectDisposedException(name)); } static internal Exception OracleError(OciErrorHandle errorHandle, int rc) { return TraceException(OracleException.CreateException(errorHandle, rc)); } static internal Exception OracleError(int rc, OracleInternalConnection internalConnection) { return TraceException(OracleException.CreateException(rc, internalConnection)); } static internal Exception Overflow(string error) { return TraceException(new OverflowException(error)); } static internal Exception Simple(string message) { return TraceException(new Exception(message)); } //////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////// // // Provider Specific Exceptions // //////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////// static internal Exception BadBindValueType(Type valueType, OracleType oracleType) { return InvalidCast(Res.GetString(Res.ADP_BadBindValueType, valueType.ToString(), oracleType.ToString())); } static internal Exception UnsupportedOracleDateTimeBinding(OracleType dtType) { return ArgumentOutOfRange("", Res.GetString(Res.ADP_BadBindValueType, typeof(OracleDateTime).ToString(), dtType.ToString())); } static internal Exception BadOracleClientImageFormat(Exception e) { return InvalidOperation(Res.GetString(Res.ADP_BadOracleClientImageFormat), e); } static internal Exception BadOracleClientVersion() { return Simple(Res.GetString(Res.ADP_BadOracleClientVersion)); } static internal Exception BufferExceeded(string argName) { return ArgumentOutOfRange(argName, Res.GetString(Res.ADP_BufferExceeded)); } static internal Exception CannotDeriveOverloaded() { return InvalidOperation(Res.GetString(Res.ADP_CannotDeriveOverloaded)); } static internal Exception CannotOpenLobWithDifferentMode(OracleLobOpenMode newmode, OracleLobOpenMode current) { return InvalidOperation(Res.GetString(Res.ADP_CannotOpenLobWithDifferentMode, newmode.ToString(), current.ToString())); } static internal Exception ChangeDatabaseNotSupported() { return NotSupported(Res.GetString(Res.ADP_ChangeDatabaseNotSupported)); } static internal Exception ClosedConnectionError() { return InvalidOperation(Res.GetString(Res.ADP_ClosedConnectionError)); } static internal Exception ClosedDataReaderError() { return InvalidOperation(Res.GetString(Res.ADP_ClosedDataReaderError)); } static internal Exception CommandTextRequired(string method) { return InvalidOperation(Res.GetString(Res.ADP_CommandTextRequired, method)); } static internal ConfigurationException ConfigUnableToLoadXmlMetaDataFile(string settingName) { return Configuration(Res.GetString(Res.ADP_ConfigUnableToLoadXmlMetaDataFile, settingName));} static internal ConfigurationException ConfigWrongNumberOfValues(string settingName) { return Configuration(Res.GetString(Res.ADP_ConfigWrongNumberOfValues, settingName));} static internal Exception ConnectionRequired(string method) { return InvalidOperation(Res.GetString(Res.ADP_ConnectionRequired, method)); } static internal Exception CouldNotCreateEnvironment(string methodname, int rc) { return Simple(Res.GetString(Res.ADP_CouldNotCreateEnvironment, methodname, rc.ToString(CultureInfo.CurrentCulture))); } static internal ArgumentException ConvertFailed(Type fromType, Type toType, Exception innerException) { return ADP.Argument(Res.GetString(Res.ADP_ConvertFailed, fromType.FullName, toType.FullName), innerException); } static internal Exception DataIsNull() { return InvalidOperation(Res.GetString(Res.ADP_DataIsNull)); } static internal Exception DataReaderNoData() { return InvalidOperation(Res.GetString(Res.ADP_DataReaderNoData)); } static internal Exception DeriveParametersNotSupported(IDbCommand value) { return ProviderException(Res.GetString(Res.ADP_DeriveParametersNotSupported, value.GetType().Name, value.CommandType.ToString())); } static internal Exception DistribTxRequiresOracle9i() { return InvalidOperation(Res.GetString(Res.ADP_DistribTxRequiresOracle9i)); } static internal Exception DistribTxRequiresOracleServicesForMTS(Exception inner) { return InvalidOperation(Res.GetString(Res.ADP_DistribTxRequiresOracleServicesForMTS), inner); } static internal Exception IdentifierIsNotQuoted() { return Argument(Res.GetString(Res.ADP_IdentifierIsNotQuoted)); } static internal Exception InputRefCursorNotSupported(string parameterName) { return InvalidOperation(Res.GetString(Res.ADP_InputRefCursorNotSupported, parameterName)); } static internal Exception InvalidCommandType(CommandType cmdType) { return Argument(Res.GetString(Res.ADP_InvalidCommandType, ((int) cmdType).ToString(CultureInfo.CurrentCulture))); } static internal Exception InvalidConnectionOptionLength(string key, int maxLength) { return Argument(Res.GetString(Res.ADP_InvalidConnectionOptionLength, key, maxLength)); } static internal Exception InvalidConnectionOptionValue(string key) { return Argument(Res.GetString(Res.ADP_InvalidConnectionOptionValue, key)); } static internal Exception InvalidDataLength(long length) { return IndexOutOfRange(Res.GetString(Res.ADP_InvalidDataLength, length.ToString(CultureInfo.CurrentCulture))); } static internal Exception InvalidDataType(TypeCode tc) { return Argument(Res.GetString(Res.ADP_InvalidDataType, tc.ToString())); } static internal Exception InvalidDataTypeForValue(Type dataType, TypeCode tc) { return Argument(Res.GetString(Res.ADP_InvalidDataTypeForValue, dataType.ToString(), tc.ToString())); } static internal Exception InvalidDbType(DbType dbType) { return ArgumentOutOfRange("dbType", Res.GetString(Res.ADP_InvalidDbType, dbType.ToString())); } // INTERNAL EXCEPTION static internal Exception InvalidDestinationBufferIndex(int maxLen, int dstOffset, string parameterName) { return ArgumentOutOfRange(parameterName, Res.GetString(Res.ADP_InvalidDestinationBufferIndex, maxLen.ToString(CultureInfo.CurrentCulture), dstOffset.ToString(CultureInfo.CurrentCulture))); } static internal Exception InvalidLobType(OracleType oracleType) { return InvalidOperation(Res.GetString(Res.ADP_InvalidLobType, oracleType.ToString())); } static internal Exception InvalidMinMaxPoolSizeValues() { return Argument(Res.GetString(Res.ADP_InvalidMinMaxPoolSizeValues)); } static internal Exception InvalidOracleType(OracleType oracleType) { return ArgumentOutOfRange("oracleType", Res.GetString(Res.ADP_InvalidOracleType, oracleType.ToString())); } // INTERNAL EXCEPTION static internal Exception InvalidSeekOrigin(SeekOrigin origin) { return Argument(Res.GetString(Res.ADP_InvalidSeekOrigin, origin.ToString())); } static internal Exception InvalidSizeValue(int value) { return Argument(Res.GetString(Res.ADP_InvalidSizeValue, value.ToString(CultureInfo.InvariantCulture))); } static internal ArgumentException KeywordNotSupported(string keyword) { return Argument(Res.GetString(Res.ADP_KeywordNotSupported, keyword)); } static internal Exception InvalidSourceBufferIndex(int maxLen, long srcOffset, string parameterName) { return ArgumentOutOfRange(parameterName, Res.GetString(Res.ADP_InvalidSourceBufferIndex, maxLen.ToString(CultureInfo.CurrentCulture), srcOffset.ToString(CultureInfo.CurrentCulture))); } static internal Exception InvalidSourceOffset(string argName, long minValue, long maxValue) { return ArgumentOutOfRange(argName, Res.GetString(Res.ADP_InvalidSourceOffset, minValue.ToString(CultureInfo.CurrentCulture), maxValue.ToString(CultureInfo.CurrentCulture))); } static internal Exception LobAmountExceeded(string argName) { return ArgumentOutOfRange(argName, Res.GetString(Res.ADP_LobAmountExceeded)); } static internal Exception LobAmountMustBeEven(string argName) { return ArgumentOutOfRange(argName, Res.GetString(Res.ADP_LobAmountMustBeEven)); } static internal Exception LobPositionMustBeEven() { return InvalidOperation(Res.GetString(Res.ADP_LobPositionMustBeEven)); } static internal Exception LobWriteInvalidOnNull () { return InvalidOperation(Res.GetString(Res.ADP_LobWriteInvalidOnNull)); } static internal Exception LobWriteRequiresTransaction() { return InvalidOperation(Res.GetString(Res.ADP_LobWriteRequiresTransaction)); } static internal Exception MonthOutOfRange() { return InvalidOperation(Res.GetString(Res.ADP_MonthOutOfRange)); } static internal Exception MustBePositive(string argName) { return ArgumentOutOfRange(argName, Res.GetString(Res.ADP_MustBePositive)); } static internal Exception NoCommandText() { return InvalidOperation(Res.GetString(Res.ADP_NoCommandText)); } static internal Exception NoData() { return InvalidOperation(Res.GetString(Res.ADP_NoData)); } static internal Exception NoLocalTransactionInDistributedContext() { return InvalidOperation(Res.GetString(Res.ADP_NoLocalTransactionInDistributedContext)); } static internal Exception NoOptimizedDirectTableAccess() { return Argument(Res.GetString(Res.ADP_NoOptimizedDirectTableAccess)); } static internal Exception NoParallelTransactions() { return InvalidOperation(Res.GetString(Res.ADP_NoParallelTransactions)); } internal const string ConnectionString = "ConnectionString"; static internal Exception OpenConnectionRequired(string method, ConnectionState state) { return InvalidOperation(Res.GetString(Res.ADP_OpenConnectionRequired, method, "ConnectionState", state.ToString())); } static internal Exception OperationFailed(string method, int rc) { return Simple(Res.GetString(Res.ADP_OperationFailed, method, rc)); } static internal Exception OperationResultedInOverflow() { return Overflow(Res.GetString(Res.ADP_OperationResultedInOverflow)); } static internal Exception ParameterConversionFailed(object value, Type destType, Exception inner) { Debug.Assert(null != value, "null value on conversion failure"); Debug.Assert(null != inner, "null inner on conversion failure"); Exception e; string message = Res.GetString(Res.ADP_ParameterConversionFailed, value.GetType().Name, destType.Name); if (inner is ArgumentException) { e = new ArgumentException(message, inner); } else if (inner is FormatException) { e = new FormatException(message, inner); } else if (inner is InvalidCastException) { e = new InvalidCastException(message, inner); } else if (inner is OverflowException) { e = new OverflowException(message, inner); } else { e = inner; } TraceExceptionAsReturnValue(e); return e; } static internal Exception ParameterSizeIsTooLarge(string parameterName) { return Simple(Res.GetString(Res.ADP_ParameterSizeIsTooLarge, parameterName)); } static internal Exception ParameterSizeIsMissing(string parameterName, Type dataType) { return Simple(Res.GetString(Res.ADP_ParameterSizeIsMissing, parameterName, dataType.Name)); } static internal Exception ReadOnlyLob() { return NotSupported(Res.GetString(Res.ADP_ReadOnlyLob)); } static internal Exception SeekBeyondEnd(string parameter) { return ArgumentOutOfRange(parameter, Res.GetString(Res.ADP_SeekBeyondEnd)); } static internal Exception SyntaxErrorExpectedCommaAfterColumn() { return TraceException(InvalidOperation(Res.GetString(Res.ADP_SyntaxErrorExpectedCommaAfterColumn))); } static internal Exception SyntaxErrorExpectedCommaAfterTable() { return TraceException(InvalidOperation(Res.GetString(Res.ADP_SyntaxErrorExpectedCommaAfterTable))); } static internal Exception SyntaxErrorExpectedIdentifier() { return TraceException(InvalidOperation(Res.GetString(Res.ADP_SyntaxErrorExpectedIdentifier))); } static internal Exception SyntaxErrorExpectedNextPart() { return TraceException(InvalidOperation(Res.GetString(Res.ADP_SyntaxErrorExpectedNextPart))); } static internal Exception SyntaxErrorMissingParenthesis() { return TraceException(InvalidOperation(Res.GetString(Res.ADP_SyntaxErrorMissingParenthesis))); } static internal Exception SyntaxErrorTooManyNameParts() { return TraceException(InvalidOperation(Res.GetString(Res.ADP_SyntaxErrorTooManyNameParts))); } static internal Exception TransactionCompleted() { return InvalidOperation(Res.GetString(Res.ADP_TransactionCompleted)); } static internal Exception TransactionConnectionMismatch() { return InvalidOperation(Res.GetString(Res.ADP_TransactionConnectionMismatch)); } static internal Exception TransactionPresent() { return InvalidOperation(Res.GetString(Res.ADP_TransactionPresent)); } static internal Exception TransactionRequired() { return InvalidOperation(Res.GetString(Res.ADP_TransactionRequired_Execute)); } static internal Exception TypeNotSupported(OCI.DATATYPE ociType) { return NotSupported(Res.GetString(Res.ADP_TypeNotSupported, ociType.ToString())); } static internal Exception UnknownDataTypeCode(Type dataType, TypeCode tc) { return Simple(Res.GetString(Res.ADP_UnknownDataTypeCode, dataType.ToString(), tc.ToString())); } static internal Exception UnsupportedIsolationLevel() { return Argument(Res.GetString(Res.ADP_UnsupportedIsolationLevel)); } static internal Exception WriteByteForBinaryLobsOnly() { return NotSupported(Res.GetString(Res.ADP_WriteByteForBinaryLobsOnly)); } static internal Exception WrongType(Type got, Type expected) { return Argument(Res.GetString(Res.ADP_WrongType, got.ToString(), expected.ToString())); } //////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////// // // Helper Functions // //////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////// static public void CheckArgumentNull(object value, string parameterName) { if (null == value) { throw ArgumentNull(parameterName); } } static internal bool IsCatchableExceptionType (Exception e) { // a 'catchable' exception is defined by what it is not. Debug.Assert(e != null, "Unexpected null exception!"); Type type = e.GetType(); return ( (type != StackOverflowType) && (type != OutOfMemoryType) && (type != ThreadAbortType) && (type != NullReferenceType) && (type != AccessViolationType) && !SecurityType.IsAssignableFrom(type)); } static internal Delegate FindBuilder(MulticastDelegate mcd) { // V1.2.3300 if (null != mcd) { Delegate[] d = mcd.GetInvocationList(); for (int i = 0; i < d.Length; i++) { if (d[i].Target is DbCommandBuilder) return d[i]; } } return null; } static internal IntPtr IntPtrOffset(IntPtr pbase, Int32 offset) { if (4 == ADP.PtrSize) { return (IntPtr) (pbase.ToInt32() + offset); } Debug.Assert(8 == ADP.PtrSize, "8 != IntPtr.Size"); return (IntPtr) (pbase.ToInt64() + offset); } static internal bool IsDirection(IDataParameter value, ParameterDirection condition) { return (condition == (condition & value.Direction)); } static internal bool IsDirection(ParameterDirection value, ParameterDirection condition) { return (condition == (condition & value)); } static internal bool IsEmpty(string str) { return ((null == str) || (0 == str.Length)); } static internal bool IsNull(object value) { if ((null == value) || (DBNull.Value == value)) { return true; } INullable nullable = (value as INullable); return ((null != nullable) && nullable.IsNull); } internal static readonly String NullString = Res.GetString(Res.SqlMisc_NullString); static internal SysTx.Transaction GetCurrentTransaction() { SysTx.Transaction transaction = SysTx.Transaction.Current; return transaction; } static internal SysTx.IDtcTransaction GetOletxTransaction(SysTx.Transaction transaction){ SysTx.IDtcTransaction oleTxTransaction = null; if (null != transaction) { oleTxTransaction = SysTx.TransactionInterop.GetDtcTransaction(transaction); } return oleTxTransaction; } [FileIOPermission(SecurityAction.Assert, AllFiles=FileIOPermissionAccess.PathDiscovery)] static internal string GetFullPath(string filename) { // MDAC 77686 return Path.GetFullPath(filename); } static internal readonly int CharSize = System.Text.UnicodeEncoding.CharSize; static internal readonly byte[] EmptyByteArray = new Byte[0]; internal const CompareOptions compareOptions = CompareOptions.IgnoreKanaType | CompareOptions.IgnoreWidth | CompareOptions.IgnoreCase; static internal readonly int PtrSize = IntPtr.Size; static internal readonly String StrEmpty = ""; // String.Empty static internal readonly HandleRef NullHandleRef = new HandleRef(null, IntPtr.Zero); static internal Stream GetFileStream(string filename) { (new FileIOPermission(FileIOPermissionAccess.Read, filename)).Assert(); try { return new FileStream(filename,FileMode.Open,FileAccess.Read,FileShare.Read); } finally { FileIOPermission.RevertAssert(); } } static internal Stream GetXmlStreamFromValues(String[] values,String errorString) { if (values.Length != 1){ throw ADP.ConfigWrongNumberOfValues(errorString); } return ADP.GetXmlStream(values[0],errorString); } static internal Stream GetXmlStream(String value,String errorString) { Stream XmlStream; const string config = "config\\"; // get location of config directory string rootPath = System.Runtime.InteropServices.RuntimeEnvironment.GetRuntimeDirectory(); if (rootPath == null) { throw ADP.ConfigUnableToLoadXmlMetaDataFile(errorString); } StringBuilder tempstring = new StringBuilder(rootPath.Length+config.Length+value.Length); tempstring.Append(rootPath); tempstring.Append(config); tempstring.Append(value); String fullPath = tempstring.ToString(); // don't allow relative paths if (ADP.GetFullPath(fullPath) != fullPath) { throw ADP.ConfigUnableToLoadXmlMetaDataFile(errorString); } try { XmlStream = ADP.GetFileStream(fullPath); } // if GetFileStrem throws it will reveal the path which could be a securiy issue catch(Exception e){ // if (!ADP.IsCatchableExceptionType(e)) { throw; } throw ADP.ConfigUnableToLoadXmlMetaDataFile(errorString); } return XmlStream; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- // SQLBUDT #360734 - [....] tells us that using this attribute makes // ngen images smaller and more efficient, if we always load both of // these assemblies together, which we do. using System.Runtime.CompilerServices; [assembly:DependencyAttribute("System.Data,", LoadHint.Always)] namespace System.Data.Common { using Microsoft.Win32; using System; using System.Collections; using System.ComponentModel; using System.Configuration; using System.Data; using System.Data.OracleClient; using System.Data.ProviderBase; using System.Data.SqlTypes; using System.Diagnostics; using System.Globalization; using System.IO; using System.Reflection; using System.Runtime.InteropServices; using System.Runtime.Serialization; using System.Security; using System.Security.Permissions; using System.Text; using System.Threading; using SysTx = System.Transactions; #if DEBUG using System.Data.OleDb; using System.Data.SqlClient; #endif sealed internal class ADP { // internal const string Parameter = "Parameter"; internal const string ParameterName = "ParameterName"; // static internal int SrcCompare(string strA, string strB) { // this is null safe return ((strA == strB) ? 0 : 1); } static internal int DstCompare(string strA, string strB) { // this is null safe return CultureInfo.CurrentCulture.CompareInfo.Compare(strA, strB, ADP.compareOptions); } static private string ConnectionStateMsg(ConnectionState state) { // MDAC 82165, if the ConnectionState enum to msg the localization looks weird switch(state) { case (ConnectionState.Closed): case (ConnectionState.Connecting|ConnectionState.Broken): // treated the same as closed return Res.GetString(Res.ADP_ConnectionStateMsg_Closed); case (ConnectionState.Connecting): return Res.GetString(Res.ADP_ConnectionStateMsg_Connecting); case (ConnectionState.Open): return Res.GetString(Res.ADP_ConnectionStateMsg_Open); case (ConnectionState.Open|ConnectionState.Executing): return Res.GetString(Res.ADP_ConnectionStateMsg_OpenExecuting); case (ConnectionState.Open|ConnectionState.Fetching): return Res.GetString(Res.ADP_ConnectionStateMsg_OpenFetching); default: return Res.GetString(Res.ADP_ConnectionStateMsg, state.ToString()); } } static internal readonly bool IsWindowsNT = (PlatformID.Win32NT == Environment.OSVersion.Platform); static internal readonly bool IsPlatformNT5 = (ADP.IsWindowsNT && (Environment.OSVersion.Version.Major >= 5)); static internal void CheckArgumentLength(string value, string parameterName) { CheckArgumentNull(value, parameterName); if (0 == value.Length) { throw Argument(Res.GetString(Res.ADP_EmptyString, parameterName)); // MDAC 94859 } } static internal bool CompareInsensitiveInvariant(string strvalue, string strconst) { return (0 == CultureInfo.InvariantCulture.CompareInfo.Compare(strvalue, strconst, CompareOptions.IgnoreCase)); } static internal bool IsEmptyArray(string[] array) { return ((null == array) || (0 == array.Length)); } static internal Exception CollectionNullValue(string parameter, Type collection, Type itemType) { return ArgumentNull(parameter, Res.GetString(Res.ADP_CollectionNullValue, collection.Name, itemType.Name)); } static internal Exception CollectionIndexInt32(int index, Type collection, int count) { return IndexOutOfRange(Res.GetString(Res.ADP_CollectionIndexInt32, index.ToString(CultureInfo.InvariantCulture), collection.Name, count.ToString(CultureInfo.InvariantCulture))); } static internal Exception CollectionIndexString(Type itemType, string propertyName, string propertyValue, Type collection) { return IndexOutOfRange(Res.GetString(Res.ADP_CollectionIndexString, itemType.Name, propertyName, propertyValue, collection.Name)); } static internal Exception CollectionInvalidType(Type collection, Type itemType, object invalidValue) { return InvalidCast(Res.GetString(Res.ADP_CollectionInvalidType, collection.Name, itemType.Name, invalidValue.GetType().Name)); } static internal ArgumentException CollectionRemoveInvalidObject(Type itemType, ICollection collection) { return Argument(Res.GetString(Res.ADP_CollectionRemoveInvalidObject, itemType.Name, collection.GetType().Name)); // MDAC 68201 } static internal Exception ConnectionAlreadyOpen(ConnectionState state) { return InvalidOperation(Res.GetString(Res.ADP_ConnectionAlreadyOpen, ADP.ConnectionStateMsg(state))); } static internal ArgumentException ConnectionStringSyntax(int index) { return Argument(Res.GetString(Res.ADP_ConnectionStringSyntax, index)); } static internal Exception InvalidDataDirectory() { return ADP.InvalidOperation(Res.GetString(Res.ADP_InvalidDataDirectory)); } static internal ArgumentException InvalidKeyname(string parameterName) { return Argument(Res.GetString(Res.ADP_InvalidKey), parameterName); } static internal ArgumentException InvalidValue(string parameterName) { return Argument(Res.GetString(Res.ADP_InvalidValue), parameterName); } static internal Exception DataReaderClosed(string method) { return InvalidOperation(Res.GetString(Res.ADP_DataReaderClosed, method)); } static internal Exception InvalidXMLBadVersion() { return Argument(Res.GetString(Res.ADP_InvalidXMLBadVersion)); } static internal Exception NotAPermissionElement() { return Argument(Res.GetString(Res.ADP_NotAPermissionElement)); } static internal Exception PermissionTypeMismatch() { return Argument(Res.GetString(Res.ADP_PermissionTypeMismatch)); } internal enum ConnectionError { BeginGetConnectionReturnsNull, GetConnectionReturnsNull, ConnectionOptionsMissing, CouldNotSwitchToClosedPreviouslyOpenedState, } static internal Exception InternalConnectionError(ConnectionError internalError) { return InvalidOperation(Res.GetString(Res.ADP_InternalConnectionError, (int)internalError)); } internal enum InternalErrorCode { UnpooledObjectHasOwner = 0, UnpooledObjectHasWrongOwner = 1, PushingObjectSecondTime = 2, PooledObjectHasOwner = 3, PooledObjectInPoolMoreThanOnce = 4, CreateObjectReturnedNull = 5, NewObjectCannotBePooled = 6, NonPooledObjectUsedMoreThanOnce = 7, AttemptingToPoolOnRestrictedToken = 8, // ConnectionOptionsInUse = 9, ConvertSidToStringSidWReturnedNull = 10, // UnexpectedTransactedObject = 11, AttemptingToConstructReferenceCollectionOnStaticObject = 12, AttemptingToEnlistTwice = 13, CreateReferenceCollectionReturnedNull = 14, PooledObjectWithoutPool = 15, UnexpectedWaitAnyResult = 16, NameValuePairNext = 20, InvalidParserState1 = 21, InvalidParserState2 = 22, InvalidBuffer = 30, InvalidLongBuffer = 31, InvalidNumberOfRows = 32, } static internal Exception InternalError(InternalErrorCode internalError) { return InvalidOperation(Res.GetString(Res.ADP_InternalProviderError, (int)internalError)); } static internal Exception InvalidConnectionOptionValue(string key, Exception inner) { return Argument(Res.GetString(Res.ADP_InvalidConnectionOptionValue, key), inner); } static internal Exception InvalidEnumerationValue(Type type, int value) { return ADP.ArgumentOutOfRange(Res.GetString(Res.ADP_InvalidEnumerationValue, type.Name, value.ToString(System.Globalization.CultureInfo.InvariantCulture)), type.Name); } // IDataParameter.SourceVersion static internal Exception InvalidDataRowVersion(DataRowVersion value) { #if DEBUG switch(value) { case DataRowVersion.Default: case DataRowVersion.Current: case DataRowVersion.Original: case DataRowVersion.Proposed: Debug.Assert(false, "valid DataRowVersion " + value.ToString()); break; } #endif return InvalidEnumerationValue(typeof(DataRowVersion), (int) value); } // DBDataPermissionAttribute.KeyRestrictionBehavior static internal Exception InvalidKeyRestrictionBehavior(KeyRestrictionBehavior value) { #if DEBUG switch(value) { case KeyRestrictionBehavior.PreventUsage: case KeyRestrictionBehavior.AllowOnly: Debug.Assert(false, "valid KeyRestrictionBehavior " + value.ToString()); break; } #endif return InvalidEnumerationValue(typeof(KeyRestrictionBehavior), (int) value); } static internal Exception InvalidOffsetValue(int value) { return Argument(Res.GetString(Res.ADP_InvalidOffsetValue, value.ToString(CultureInfo.InvariantCulture))); } static internal Exception InvalidParameterDirection(ParameterDirection value) { #if DEBUG switch(value) { case ParameterDirection.Input: case ParameterDirection.Output: case ParameterDirection.InputOutput: case ParameterDirection.ReturnValue: Debug.Assert(false, "valid ParameterDirection " + value.ToString()); break; } #endif return InvalidEnumerationValue(typeof(ParameterDirection), (int) value); } static internal Exception InvalidParameterType(IDataParameterCollection collection, Type parameterType, object invalidValue) { return CollectionInvalidType(collection.GetType(), parameterType, invalidValue); } static internal Exception InvalidPermissionState(PermissionState value) { #if DEBUG switch(value) { case PermissionState.Unrestricted: case PermissionState.None: Debug.Assert(false, "valid PermissionState " + value.ToString()); break; } #endif return InvalidEnumerationValue(typeof(PermissionState), (int) value); } // IDbCommand.UpdateRowSource static internal Exception InvalidUpdateRowSource(UpdateRowSource value) { #if DEBUG switch(value) { case UpdateRowSource.None: case UpdateRowSource.OutputParameters: case UpdateRowSource.FirstReturnedRecord: case UpdateRowSource.Both: Debug.Assert(false, "valid UpdateRowSource " + value.ToString()); break; } #endif return InvalidEnumerationValue(typeof(UpdateRowSource), (int) value); } static internal Exception MethodNotImplemented(string methodName) { NotImplementedException e = new NotImplementedException(methodName); TraceExceptionAsReturnValue(e); return e; } static internal Exception NoConnectionString() { return InvalidOperation(Res.GetString(Res.ADP_NoConnectionString)); } static internal Exception ParameterNull(string parameter, IDataParameterCollection collection, Type parameterType) { return CollectionNullValue(parameter, collection.GetType(), parameterType); } static internal Exception ParametersIsNotParent(Type parameterType, IDataParameterCollection collection) { return Argument(Res.GetString(Res.ADP_CollectionIsNotParent, parameterType.Name, collection.GetType().Name)); } static internal ArgumentException ParametersIsParent(Type parameterType, IDataParameterCollection collection) { return Argument(Res.GetString(Res.ADP_CollectionIsNotParent, parameterType.Name, collection.GetType().Name)); } static internal Exception ParametersMappingIndex(int index, IDataParameterCollection collection) { return CollectionIndexInt32(index, collection.GetType(), collection.Count); } static internal Exception ParametersSourceIndex(string parameterName, IDataParameterCollection collection, Type parameterType) { return CollectionIndexString(parameterType, ADP.ParameterName, parameterName, collection.GetType()); } static internal Exception PooledOpenTimeout() { return ADP.InvalidOperation(Res.GetString(Res.ADP_PooledOpenTimeout)); } static internal Exception OpenConnectionPropertySet(string property, ConnectionState state) { return InvalidOperation(Res.GetString(Res.ADP_OpenConnectionPropertySet, property, ADP.ConnectionStateMsg(state))); } static internal Exception AmbigousCollectionName(string collectionName) { return Argument(Res.GetString(Res.MDF_AmbigousCollectionName,collectionName)); } static internal Exception CollectionNameIsNotUnique(string collectionName) { return Argument(Res.GetString(Res.MDF_CollectionNameISNotUnique,collectionName)); } static internal Exception DataTableDoesNotExist(string collectionName) { return Argument(Res.GetString(Res.MDF_DataTableDoesNotExist,collectionName)); } static internal Exception IncorrectNumberOfDataSourceInformationRows() { return Argument(Res.GetString(Res.MDF_IncorrectNumberOfDataSourceInformationRows)); } static internal Exception InvalidXml() { return Argument(Res.GetString(Res.MDF_InvalidXml)); } static internal Exception InvalidXmlMissingColumn(string collectionName, string columnName) { return Argument(Res.GetString(Res.MDF_InvalidXmlMissingColumn, collectionName, columnName)); } static internal Exception InvalidXmlInvalidValue(string collectionName, string columnName) { return Argument(Res.GetString(Res.MDF_InvalidXmlInvalidValue, collectionName, columnName)); } static internal Exception MissingDataSourceInformationColumn() { return Argument(Res.GetString(Res.MDF_MissingDataSourceInformationColumn)); } static internal Exception MissingRestrictionColumn() { return Argument(Res.GetString(Res.MDF_MissingRestrictionColumn)); } static internal Exception MissingRestrictionRow() { return Argument(Res.GetString(Res.MDF_MissingRestrictionRow)); } static internal Exception NoColumns() { return Argument(Res.GetString(Res.MDF_NoColumns)); } static internal Exception QueryFailed(string collectionName, Exception e) { return InvalidOperation(Res.GetString(Res.MDF_QueryFailed,collectionName), e); } static internal Exception TooManyRestrictions(string collectionName) { return Argument(Res.GetString(Res.MDF_TooManyRestrictions,collectionName)); } static internal Exception UndefinedCollection(string collectionName) { return Argument(Res.GetString(Res.MDF_UndefinedCollection,collectionName)); } static internal Exception UndefinedPopulationMechanism(string populationMechanism) { return Argument(Res.GetString(Res.MDF_UndefinedPopulationMechanism,populationMechanism)); } static internal Exception UnsupportedVersion(string collectionName) { return Argument(Res.GetString(Res.MDF_UnsupportedVersion,collectionName)); } ////////////////////////////// //// END OF COMMON CODE STUFF ////////////////////////////// // The class ADP defines the exceptions that are specific to the Adapters. private ADP() { } // only StackOverflowException & ThreadAbortException are sealed classes static private readonly Type StackOverflowType = typeof(StackOverflowException); static private readonly Type OutOfMemoryType = typeof(OutOfMemoryException); static private readonly Type ThreadAbortType = typeof(ThreadAbortException); static private readonly Type NullReferenceType = typeof(NullReferenceException); static private readonly Type AccessViolationType = typeof(AccessViolationException); static private readonly Type SecurityType = typeof(SecurityException); static internal readonly Type ArgumentNullExceptionType = typeof(ArgumentNullException); static internal readonly Type FormatExceptionType = typeof(FormatException); static internal readonly Type OverflowExceptionType = typeof(OverflowException); // The class contains functions that take the proper informational variables and then construct // the appropriate exception with an error string obtained from the resource Framework.txt. // The exception is then returned to the caller, so that the caller may then throw from its // location so that the catcher of the exception will have the appropriate call stack. // This class is used so that there will be compile time checking of error messages. // The resource Framework.txt will ensure proper string text based on the appropriate // locale. //////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////// // // Traced Exception Constructors // //////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////// static private void TraceException(string trace, Exception e) { Debug.Assert(null != e, "TraceException: null Exception"); if (null != e) { Bid.Trace(trace, e.ToString()); // will include callstack if permission is available } } static internal Exception TraceException(Exception e) { TraceExceptionAsReturnValue(e); return e; } static internal void TraceExceptionAsReturnValue(Exception e) { TraceException("'%ls'\n", e); } static internal void TraceExceptionForCapture(Exception e) { Debug.Assert(ADP.IsCatchableExceptionType(e), "Invalid exception type, should have been re-thrown!"); TraceException(" '%ls'\n", e); } static internal void TraceExceptionWithoutRethrow(Exception e) { Debug.Assert(ADP.IsCatchableExceptionType(e), "Invalid exception type, should have been re-thrown!"); TraceException(" '%ls'\n", e); } static internal ArgumentException Argument(string error) { ArgumentException e = new ArgumentException(error); TraceExceptionAsReturnValue(e); return e; } static internal ArgumentException Argument(string error, string parameter) { ArgumentException e = new ArgumentException(error, parameter); TraceExceptionAsReturnValue(e); return e; } static internal ArgumentException Argument(string error, Exception inner) { ArgumentException e = new ArgumentException(error, inner); TraceExceptionAsReturnValue(e); return e; } static internal ArgumentNullException ArgumentNull(string parameter) { ArgumentNullException e = new ArgumentNullException(parameter); TraceExceptionAsReturnValue(e); return e; } static internal ArgumentNullException ArgumentNull(string parameter, string error) { ArgumentNullException e = new ArgumentNullException(parameter, error); TraceExceptionAsReturnValue(e); return e; } static internal ArgumentOutOfRangeException ArgumentOutOfRange(string argName, string message) { ArgumentOutOfRangeException e = new ArgumentOutOfRangeException(argName, message); TraceExceptionAsReturnValue(e); return e; } static internal ConfigurationException Configuration(string message) { ConfigurationException e = new ConfigurationErrorsException(message); TraceExceptionAsReturnValue(e); return e; } static internal Exception ProviderException(string error) { return InvalidOperation(error); } static internal Exception IndexOutOfRange(string error) { return TraceException(new IndexOutOfRangeException(error)); } static internal Exception InvalidCast() { return TraceException(new InvalidCastException()); } static internal Exception InvalidCast(string error) { return TraceException(new InvalidCastException(error)); } static internal Exception InvalidOperation(string error) { return TraceException(new InvalidOperationException(error)); } static internal Exception InvalidOperation(string error, Exception inner) { return TraceException(new InvalidOperationException(error, inner)); } static internal Exception NotSupported() { return TraceException(new NotSupportedException()); } static internal Exception NotSupported(string message) { return TraceException(new NotSupportedException(message)); } static internal Exception ObjectDisposed(string name) { return TraceException(new ObjectDisposedException(name)); } static internal Exception OracleError(OciErrorHandle errorHandle, int rc) { return TraceException(OracleException.CreateException(errorHandle, rc)); } static internal Exception OracleError(int rc, OracleInternalConnection internalConnection) { return TraceException(OracleException.CreateException(rc, internalConnection)); } static internal Exception Overflow(string error) { return TraceException(new OverflowException(error)); } static internal Exception Simple(string message) { return TraceException(new Exception(message)); } //////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////// // // Provider Specific Exceptions // //////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////// static internal Exception BadBindValueType(Type valueType, OracleType oracleType) { return InvalidCast(Res.GetString(Res.ADP_BadBindValueType, valueType.ToString(), oracleType.ToString())); } static internal Exception UnsupportedOracleDateTimeBinding(OracleType dtType) { return ArgumentOutOfRange("", Res.GetString(Res.ADP_BadBindValueType, typeof(OracleDateTime).ToString(), dtType.ToString())); } static internal Exception BadOracleClientImageFormat(Exception e) { return InvalidOperation(Res.GetString(Res.ADP_BadOracleClientImageFormat), e); } static internal Exception BadOracleClientVersion() { return Simple(Res.GetString(Res.ADP_BadOracleClientVersion)); } static internal Exception BufferExceeded(string argName) { return ArgumentOutOfRange(argName, Res.GetString(Res.ADP_BufferExceeded)); } static internal Exception CannotDeriveOverloaded() { return InvalidOperation(Res.GetString(Res.ADP_CannotDeriveOverloaded)); } static internal Exception CannotOpenLobWithDifferentMode(OracleLobOpenMode newmode, OracleLobOpenMode current) { return InvalidOperation(Res.GetString(Res.ADP_CannotOpenLobWithDifferentMode, newmode.ToString(), current.ToString())); } static internal Exception ChangeDatabaseNotSupported() { return NotSupported(Res.GetString(Res.ADP_ChangeDatabaseNotSupported)); } static internal Exception ClosedConnectionError() { return InvalidOperation(Res.GetString(Res.ADP_ClosedConnectionError)); } static internal Exception ClosedDataReaderError() { return InvalidOperation(Res.GetString(Res.ADP_ClosedDataReaderError)); } static internal Exception CommandTextRequired(string method) { return InvalidOperation(Res.GetString(Res.ADP_CommandTextRequired, method)); } static internal ConfigurationException ConfigUnableToLoadXmlMetaDataFile(string settingName) { return Configuration(Res.GetString(Res.ADP_ConfigUnableToLoadXmlMetaDataFile, settingName));} static internal ConfigurationException ConfigWrongNumberOfValues(string settingName) { return Configuration(Res.GetString(Res.ADP_ConfigWrongNumberOfValues, settingName));} static internal Exception ConnectionRequired(string method) { return InvalidOperation(Res.GetString(Res.ADP_ConnectionRequired, method)); } static internal Exception CouldNotCreateEnvironment(string methodname, int rc) { return Simple(Res.GetString(Res.ADP_CouldNotCreateEnvironment, methodname, rc.ToString(CultureInfo.CurrentCulture))); } static internal ArgumentException ConvertFailed(Type fromType, Type toType, Exception innerException) { return ADP.Argument(Res.GetString(Res.ADP_ConvertFailed, fromType.FullName, toType.FullName), innerException); } static internal Exception DataIsNull() { return InvalidOperation(Res.GetString(Res.ADP_DataIsNull)); } static internal Exception DataReaderNoData() { return InvalidOperation(Res.GetString(Res.ADP_DataReaderNoData)); } static internal Exception DeriveParametersNotSupported(IDbCommand value) { return ProviderException(Res.GetString(Res.ADP_DeriveParametersNotSupported, value.GetType().Name, value.CommandType.ToString())); } static internal Exception DistribTxRequiresOracle9i() { return InvalidOperation(Res.GetString(Res.ADP_DistribTxRequiresOracle9i)); } static internal Exception DistribTxRequiresOracleServicesForMTS(Exception inner) { return InvalidOperation(Res.GetString(Res.ADP_DistribTxRequiresOracleServicesForMTS), inner); } static internal Exception IdentifierIsNotQuoted() { return Argument(Res.GetString(Res.ADP_IdentifierIsNotQuoted)); } static internal Exception InputRefCursorNotSupported(string parameterName) { return InvalidOperation(Res.GetString(Res.ADP_InputRefCursorNotSupported, parameterName)); } static internal Exception InvalidCommandType(CommandType cmdType) { return Argument(Res.GetString(Res.ADP_InvalidCommandType, ((int) cmdType).ToString(CultureInfo.CurrentCulture))); } static internal Exception InvalidConnectionOptionLength(string key, int maxLength) { return Argument(Res.GetString(Res.ADP_InvalidConnectionOptionLength, key, maxLength)); } static internal Exception InvalidConnectionOptionValue(string key) { return Argument(Res.GetString(Res.ADP_InvalidConnectionOptionValue, key)); } static internal Exception InvalidDataLength(long length) { return IndexOutOfRange(Res.GetString(Res.ADP_InvalidDataLength, length.ToString(CultureInfo.CurrentCulture))); } static internal Exception InvalidDataType(TypeCode tc) { return Argument(Res.GetString(Res.ADP_InvalidDataType, tc.ToString())); } static internal Exception InvalidDataTypeForValue(Type dataType, TypeCode tc) { return Argument(Res.GetString(Res.ADP_InvalidDataTypeForValue, dataType.ToString(), tc.ToString())); } static internal Exception InvalidDbType(DbType dbType) { return ArgumentOutOfRange("dbType", Res.GetString(Res.ADP_InvalidDbType, dbType.ToString())); } // INTERNAL EXCEPTION static internal Exception InvalidDestinationBufferIndex(int maxLen, int dstOffset, string parameterName) { return ArgumentOutOfRange(parameterName, Res.GetString(Res.ADP_InvalidDestinationBufferIndex, maxLen.ToString(CultureInfo.CurrentCulture), dstOffset.ToString(CultureInfo.CurrentCulture))); } static internal Exception InvalidLobType(OracleType oracleType) { return InvalidOperation(Res.GetString(Res.ADP_InvalidLobType, oracleType.ToString())); } static internal Exception InvalidMinMaxPoolSizeValues() { return Argument(Res.GetString(Res.ADP_InvalidMinMaxPoolSizeValues)); } static internal Exception InvalidOracleType(OracleType oracleType) { return ArgumentOutOfRange("oracleType", Res.GetString(Res.ADP_InvalidOracleType, oracleType.ToString())); } // INTERNAL EXCEPTION static internal Exception InvalidSeekOrigin(SeekOrigin origin) { return Argument(Res.GetString(Res.ADP_InvalidSeekOrigin, origin.ToString())); } static internal Exception InvalidSizeValue(int value) { return Argument(Res.GetString(Res.ADP_InvalidSizeValue, value.ToString(CultureInfo.InvariantCulture))); } static internal ArgumentException KeywordNotSupported(string keyword) { return Argument(Res.GetString(Res.ADP_KeywordNotSupported, keyword)); } static internal Exception InvalidSourceBufferIndex(int maxLen, long srcOffset, string parameterName) { return ArgumentOutOfRange(parameterName, Res.GetString(Res.ADP_InvalidSourceBufferIndex, maxLen.ToString(CultureInfo.CurrentCulture), srcOffset.ToString(CultureInfo.CurrentCulture))); } static internal Exception InvalidSourceOffset(string argName, long minValue, long maxValue) { return ArgumentOutOfRange(argName, Res.GetString(Res.ADP_InvalidSourceOffset, minValue.ToString(CultureInfo.CurrentCulture), maxValue.ToString(CultureInfo.CurrentCulture))); } static internal Exception LobAmountExceeded(string argName) { return ArgumentOutOfRange(argName, Res.GetString(Res.ADP_LobAmountExceeded)); } static internal Exception LobAmountMustBeEven(string argName) { return ArgumentOutOfRange(argName, Res.GetString(Res.ADP_LobAmountMustBeEven)); } static internal Exception LobPositionMustBeEven() { return InvalidOperation(Res.GetString(Res.ADP_LobPositionMustBeEven)); } static internal Exception LobWriteInvalidOnNull () { return InvalidOperation(Res.GetString(Res.ADP_LobWriteInvalidOnNull)); } static internal Exception LobWriteRequiresTransaction() { return InvalidOperation(Res.GetString(Res.ADP_LobWriteRequiresTransaction)); } static internal Exception MonthOutOfRange() { return InvalidOperation(Res.GetString(Res.ADP_MonthOutOfRange)); } static internal Exception MustBePositive(string argName) { return ArgumentOutOfRange(argName, Res.GetString(Res.ADP_MustBePositive)); } static internal Exception NoCommandText() { return InvalidOperation(Res.GetString(Res.ADP_NoCommandText)); } static internal Exception NoData() { return InvalidOperation(Res.GetString(Res.ADP_NoData)); } static internal Exception NoLocalTransactionInDistributedContext() { return InvalidOperation(Res.GetString(Res.ADP_NoLocalTransactionInDistributedContext)); } static internal Exception NoOptimizedDirectTableAccess() { return Argument(Res.GetString(Res.ADP_NoOptimizedDirectTableAccess)); } static internal Exception NoParallelTransactions() { return InvalidOperation(Res.GetString(Res.ADP_NoParallelTransactions)); } internal const string ConnectionString = "ConnectionString"; static internal Exception OpenConnectionRequired(string method, ConnectionState state) { return InvalidOperation(Res.GetString(Res.ADP_OpenConnectionRequired, method, "ConnectionState", state.ToString())); } static internal Exception OperationFailed(string method, int rc) { return Simple(Res.GetString(Res.ADP_OperationFailed, method, rc)); } static internal Exception OperationResultedInOverflow() { return Overflow(Res.GetString(Res.ADP_OperationResultedInOverflow)); } static internal Exception ParameterConversionFailed(object value, Type destType, Exception inner) { Debug.Assert(null != value, "null value on conversion failure"); Debug.Assert(null != inner, "null inner on conversion failure"); Exception e; string message = Res.GetString(Res.ADP_ParameterConversionFailed, value.GetType().Name, destType.Name); if (inner is ArgumentException) { e = new ArgumentException(message, inner); } else if (inner is FormatException) { e = new FormatException(message, inner); } else if (inner is InvalidCastException) { e = new InvalidCastException(message, inner); } else if (inner is OverflowException) { e = new OverflowException(message, inner); } else { e = inner; } TraceExceptionAsReturnValue(e); return e; } static internal Exception ParameterSizeIsTooLarge(string parameterName) { return Simple(Res.GetString(Res.ADP_ParameterSizeIsTooLarge, parameterName)); } static internal Exception ParameterSizeIsMissing(string parameterName, Type dataType) { return Simple(Res.GetString(Res.ADP_ParameterSizeIsMissing, parameterName, dataType.Name)); } static internal Exception ReadOnlyLob() { return NotSupported(Res.GetString(Res.ADP_ReadOnlyLob)); } static internal Exception SeekBeyondEnd(string parameter) { return ArgumentOutOfRange(parameter, Res.GetString(Res.ADP_SeekBeyondEnd)); } static internal Exception SyntaxErrorExpectedCommaAfterColumn() { return TraceException(InvalidOperation(Res.GetString(Res.ADP_SyntaxErrorExpectedCommaAfterColumn))); } static internal Exception SyntaxErrorExpectedCommaAfterTable() { return TraceException(InvalidOperation(Res.GetString(Res.ADP_SyntaxErrorExpectedCommaAfterTable))); } static internal Exception SyntaxErrorExpectedIdentifier() { return TraceException(InvalidOperation(Res.GetString(Res.ADP_SyntaxErrorExpectedIdentifier))); } static internal Exception SyntaxErrorExpectedNextPart() { return TraceException(InvalidOperation(Res.GetString(Res.ADP_SyntaxErrorExpectedNextPart))); } static internal Exception SyntaxErrorMissingParenthesis() { return TraceException(InvalidOperation(Res.GetString(Res.ADP_SyntaxErrorMissingParenthesis))); } static internal Exception SyntaxErrorTooManyNameParts() { return TraceException(InvalidOperation(Res.GetString(Res.ADP_SyntaxErrorTooManyNameParts))); } static internal Exception TransactionCompleted() { return InvalidOperation(Res.GetString(Res.ADP_TransactionCompleted)); } static internal Exception TransactionConnectionMismatch() { return InvalidOperation(Res.GetString(Res.ADP_TransactionConnectionMismatch)); } static internal Exception TransactionPresent() { return InvalidOperation(Res.GetString(Res.ADP_TransactionPresent)); } static internal Exception TransactionRequired() { return InvalidOperation(Res.GetString(Res.ADP_TransactionRequired_Execute)); } static internal Exception TypeNotSupported(OCI.DATATYPE ociType) { return NotSupported(Res.GetString(Res.ADP_TypeNotSupported, ociType.ToString())); } static internal Exception UnknownDataTypeCode(Type dataType, TypeCode tc) { return Simple(Res.GetString(Res.ADP_UnknownDataTypeCode, dataType.ToString(), tc.ToString())); } static internal Exception UnsupportedIsolationLevel() { return Argument(Res.GetString(Res.ADP_UnsupportedIsolationLevel)); } static internal Exception WriteByteForBinaryLobsOnly() { return NotSupported(Res.GetString(Res.ADP_WriteByteForBinaryLobsOnly)); } static internal Exception WrongType(Type got, Type expected) { return Argument(Res.GetString(Res.ADP_WrongType, got.ToString(), expected.ToString())); } //////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////// // // Helper Functions // //////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////// static public void CheckArgumentNull(object value, string parameterName) { if (null == value) { throw ArgumentNull(parameterName); } } static internal bool IsCatchableExceptionType (Exception e) { // a 'catchable' exception is defined by what it is not. Debug.Assert(e != null, "Unexpected null exception!"); Type type = e.GetType(); return ( (type != StackOverflowType) && (type != OutOfMemoryType) && (type != ThreadAbortType) && (type != NullReferenceType) && (type != AccessViolationType) && !SecurityType.IsAssignableFrom(type)); } static internal Delegate FindBuilder(MulticastDelegate mcd) { // V1.2.3300 if (null != mcd) { Delegate[] d = mcd.GetInvocationList(); for (int i = 0; i < d.Length; i++) { if (d[i].Target is DbCommandBuilder) return d[i]; } } return null; } static internal IntPtr IntPtrOffset(IntPtr pbase, Int32 offset) { if (4 == ADP.PtrSize) { return (IntPtr) (pbase.ToInt32() + offset); } Debug.Assert(8 == ADP.PtrSize, "8 != IntPtr.Size"); return (IntPtr) (pbase.ToInt64() + offset); } static internal bool IsDirection(IDataParameter value, ParameterDirection condition) { return (condition == (condition & value.Direction)); } static internal bool IsDirection(ParameterDirection value, ParameterDirection condition) { return (condition == (condition & value)); } static internal bool IsEmpty(string str) { return ((null == str) || (0 == str.Length)); } static internal bool IsNull(object value) { if ((null == value) || (DBNull.Value == value)) { return true; } INullable nullable = (value as INullable); return ((null != nullable) && nullable.IsNull); } internal static readonly String NullString = Res.GetString(Res.SqlMisc_NullString); static internal SysTx.Transaction GetCurrentTransaction() { SysTx.Transaction transaction = SysTx.Transaction.Current; return transaction; } static internal SysTx.IDtcTransaction GetOletxTransaction(SysTx.Transaction transaction){ SysTx.IDtcTransaction oleTxTransaction = null; if (null != transaction) { oleTxTransaction = SysTx.TransactionInterop.GetDtcTransaction(transaction); } return oleTxTransaction; } [FileIOPermission(SecurityAction.Assert, AllFiles=FileIOPermissionAccess.PathDiscovery)] static internal string GetFullPath(string filename) { // MDAC 77686 return Path.GetFullPath(filename); } static internal readonly int CharSize = System.Text.UnicodeEncoding.CharSize; static internal readonly byte[] EmptyByteArray = new Byte[0]; internal const CompareOptions compareOptions = CompareOptions.IgnoreKanaType | CompareOptions.IgnoreWidth | CompareOptions.IgnoreCase; static internal readonly int PtrSize = IntPtr.Size; static internal readonly String StrEmpty = ""; // String.Empty static internal readonly HandleRef NullHandleRef = new HandleRef(null, IntPtr.Zero); static internal Stream GetFileStream(string filename) { (new FileIOPermission(FileIOPermissionAccess.Read, filename)).Assert(); try { return new FileStream(filename,FileMode.Open,FileAccess.Read,FileShare.Read); } finally { FileIOPermission.RevertAssert(); } } static internal Stream GetXmlStreamFromValues(String[] values,String errorString) { if (values.Length != 1){ throw ADP.ConfigWrongNumberOfValues(errorString); } return ADP.GetXmlStream(values[0],errorString); } static internal Stream GetXmlStream(String value,String errorString) { Stream XmlStream; const string config = "config\\"; // get location of config directory string rootPath = System.Runtime.InteropServices.RuntimeEnvironment.GetRuntimeDirectory(); if (rootPath == null) { throw ADP.ConfigUnableToLoadXmlMetaDataFile(errorString); } StringBuilder tempstring = new StringBuilder(rootPath.Length+config.Length+value.Length); tempstring.Append(rootPath); tempstring.Append(config); tempstring.Append(value); String fullPath = tempstring.ToString(); // don't allow relative paths if (ADP.GetFullPath(fullPath) != fullPath) { throw ADP.ConfigUnableToLoadXmlMetaDataFile(errorString); } try { XmlStream = ADP.GetFileStream(fullPath); } // if GetFileStrem throws it will reveal the path which could be a securiy issue catch(Exception e){ // if (!ADP.IsCatchableExceptionType(e)) { throw; } throw ADP.ConfigUnableToLoadXmlMetaDataFile(errorString); } return XmlStream; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
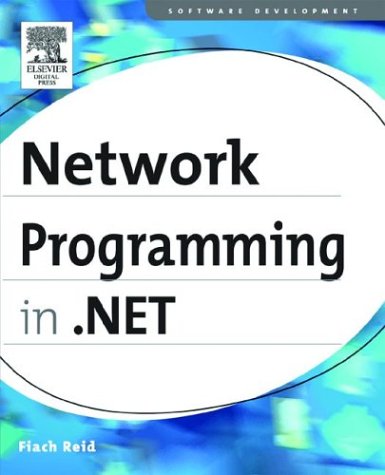
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PropertyNames.cs
- Color.cs
- mda.cs
- StylusCaptureWithinProperty.cs
- ColumnCollection.cs
- DataRowIndexBuffer.cs
- Rotation3DAnimation.cs
- SingleAnimationUsingKeyFrames.cs
- StreamResourceInfo.cs
- XmlDocumentType.cs
- NetTcpBindingCollectionElement.cs
- _TimerThread.cs
- DecimalKeyFrameCollection.cs
- StrongName.cs
- BinarySerializer.cs
- DeviceContext.cs
- ConfigXmlWhitespace.cs
- pingexception.cs
- Rule.cs
- ScriptComponentDescriptor.cs
- HTTPRemotingHandler.cs
- Emitter.cs
- BreakSafeBase.cs
- CharUnicodeInfo.cs
- TextTreeRootNode.cs
- ConnectionPoolManager.cs
- ApplicationContext.cs
- TextParaClient.cs
- ToolStripOverflowButton.cs
- ManagementPath.cs
- OdbcCommandBuilder.cs
- RuntimeArgumentHandle.cs
- HwndMouseInputProvider.cs
- ButtonFieldBase.cs
- HtmlInputFile.cs
- CounterSampleCalculator.cs
- SoapMessage.cs
- StatusBarPanel.cs
- Thumb.cs
- Aggregates.cs
- LabelLiteral.cs
- HyperLinkStyle.cs
- DomainConstraint.cs
- XmlReader.cs
- CheckBoxField.cs
- StatusBar.cs
- ThemeDirectoryCompiler.cs
- PropertyValueUIItem.cs
- DataObjectAttribute.cs
- RootContext.cs
- ChannelHandler.cs
- NotConverter.cs
- CategoryNameCollection.cs
- ComponentResourceKey.cs
- DependencyObject.cs
- CaseInsensitiveHashCodeProvider.cs
- SafeRightsManagementPubHandle.cs
- ObjectTypeMapping.cs
- IIS7UserPrincipal.cs
- altserialization.cs
- LoggedException.cs
- MobileFormsAuthentication.cs
- ToolStripSettings.cs
- dsa.cs
- SnapshotChangeTrackingStrategy.cs
- EmbeddedMailObjectsCollection.cs
- XPathNavigatorKeyComparer.cs
- SecurityHelper.cs
- TraceFilter.cs
- ValidationResult.cs
- DocumentReferenceCollection.cs
- SystemWebExtensionsSectionGroup.cs
- XmlSchemaComplexContentRestriction.cs
- ComponentCodeDomSerializer.cs
- CuspData.cs
- XmlImplementation.cs
- HostedTransportConfigurationBase.cs
- HtmlImage.cs
- MruCache.cs
- CardSpacePolicyElement.cs
- ProfilePropertySettings.cs
- SqlStatistics.cs
- TextControlDesigner.cs
- SyncMethodInvoker.cs
- CommandDesigner.cs
- StyleModeStack.cs
- DataControlField.cs
- XmlEntityReference.cs
- ExtractorMetadata.cs
- ProxySimple.cs
- WebPartUtil.cs
- SystemIPv4InterfaceProperties.cs
- SimpleTableProvider.cs
- WebConvert.cs
- WorkflowMarkupSerializationException.cs
- TagNameToTypeMapper.cs
- ApplyTemplatesAction.cs
- TransportSecurityProtocolFactory.cs
- ExtractedStateEntry.cs
- WindowsListViewItem.cs