Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Recognition / SrgsGrammar / SrgsGrammarCompiler.cs / 1 / SrgsGrammarCompiler.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // // History: // 6/1/2004 jeanfp //--------------------------------------------------------------------------- using System; using System.IO; using System.Xml; using System.Speech.Internal; using System.Speech.Internal.SrgsCompiler; namespace System.Speech.Recognition.SrgsGrammar { ////// Compiles Xml Srgs data into a CFG /// public static class SrgsGrammarCompiler { //******************************************************************* // // Public Methods // //******************************************************************* #region Public Methods ////// Compiles a grammar to a file /// /// /// static public void Compile (string inputPath, Stream outputStream) { Helpers.ThrowIfEmptyOrNull (inputPath, "inputPath"); Helpers.ThrowIfNull (outputStream, "outputStream"); using (XmlTextReader reader = new XmlTextReader (new Uri (inputPath, UriKind.RelativeOrAbsolute).ToString ())) { SrgsCompiler.CompileStream (new XmlReader [] { reader }, null, outputStream, true, null, null, null); } } ////// Compiles an Srgs documentto a file /// /// /// static public void Compile (SrgsDocument srgsGrammar, Stream outputStream) { Helpers.ThrowIfNull (srgsGrammar, "srgsGrammar"); Helpers.ThrowIfNull (outputStream, "outputStream"); SrgsCompiler.CompileStream (srgsGrammar, null, outputStream, true, null, null); } ////// Compiles a grammar to a file /// /// /// static public void Compile (XmlReader reader, Stream outputStream) { Helpers.ThrowIfNull (reader, "reader"); Helpers.ThrowIfNull (outputStream, "outputStream"); SrgsCompiler.CompileStream (new XmlReader [] { reader }, null, outputStream, true, null, null, null); } #if !NO_STG ////// Compiles a grammar to a file /// /// /// /// /// static public void CompileClassLibrary (string [] inputPaths, string outputPath, string [] referencedAssemblies, string keyFile) { Helpers.ThrowIfNull (inputPaths, "inputPaths"); Helpers.ThrowIfEmptyOrNull (outputPath, "outputPath"); XmlTextReader [] readers = new XmlTextReader [inputPaths.Length]; try { for (int iFile = 0; iFile < inputPaths.Length; iFile++) { if (inputPaths [iFile] == null) { throw new ArgumentException (SR.Get (SRID.ArrayOfNullIllegal), "inputPaths"); } readers [iFile] = new XmlTextReader (new Uri (inputPaths [iFile], UriKind.RelativeOrAbsolute).ToString ()); } SrgsCompiler.CompileStream (readers, outputPath, null, false, null, referencedAssemblies, keyFile); } finally { for (int iReader = 0; iReader < readers.Length; iReader++) { XmlTextReader srgsGrammar = readers [iReader]; if (srgsGrammar != null) { ((IDisposable) srgsGrammar).Dispose (); } } } } ////// Compiles an Srgs documentto a file /// /// /// /// /// static public void CompileClassLibrary (SrgsDocument srgsGrammar, string outputPath, string [] referencedAssemblies, string keyFile) { Helpers.ThrowIfNull (srgsGrammar, "srgsGrammar"); Helpers.ThrowIfEmptyOrNull (outputPath, "outputPath"); SrgsCompiler.CompileStream (srgsGrammar, outputPath, null, false, referencedAssemblies, keyFile); } ////// Compiles a grammar to a file /// /// /// /// /// static public void CompileClassLibrary (XmlReader reader, string outputPath, string [] referencedAssemblies, string keyFile) { Helpers.ThrowIfNull (reader, "reader"); Helpers.ThrowIfEmptyOrNull (outputPath, "outputPath"); SrgsCompiler.CompileStream (new XmlReader [] { reader }, outputPath, null, false, null, referencedAssemblies, keyFile); } #endif #endregion //******************************************************************** // // Internal Methods // //******************************************************************* #region Internal Methods // Decide if the input stream is a cfg. // If not assume it's an xml grammar. // The stream parameter points to the start of the data on entry and is reset to that point on exit. static private bool CheckIfCfg (Stream stream, out int cfgLength) { long initialPosition = stream.Position; bool isCfg = CfgGrammar.CfgSerializedHeader.IsCfg (stream, out cfgLength); // Reset stream position: stream.Position = initialPosition; return isCfg; } static internal void CompileXmlOrCopyCfg ( Stream inputStream, Stream outputStream, Uri orginalUri) { // Wrap stream in case Seek is not supported: SeekableReadStream seekableInputStream = new SeekableReadStream (inputStream); // See if CFG or XML document: int cfgLength; bool isCFG = CheckIfCfg (seekableInputStream, out cfgLength); seekableInputStream.CacheDataForSeeking = false; // Stop buffering data if (isCFG) { // Just copy the input to the output: // {We later check the header on the output stream - we could do it on the input stream but it may not be seekable}. Helpers.CopyStream (seekableInputStream, outputStream, cfgLength); } else { // Else compile the Xml: SrgsCompiler.CompileStream (new XmlReader [] { new XmlTextReader (seekableInputStream) }, null, outputStream, true, orginalUri, null, null); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // // History: // 6/1/2004 jeanfp //--------------------------------------------------------------------------- using System; using System.IO; using System.Xml; using System.Speech.Internal; using System.Speech.Internal.SrgsCompiler; namespace System.Speech.Recognition.SrgsGrammar { ////// Compiles Xml Srgs data into a CFG /// public static class SrgsGrammarCompiler { //******************************************************************* // // Public Methods // //******************************************************************* #region Public Methods ////// Compiles a grammar to a file /// /// /// static public void Compile (string inputPath, Stream outputStream) { Helpers.ThrowIfEmptyOrNull (inputPath, "inputPath"); Helpers.ThrowIfNull (outputStream, "outputStream"); using (XmlTextReader reader = new XmlTextReader (new Uri (inputPath, UriKind.RelativeOrAbsolute).ToString ())) { SrgsCompiler.CompileStream (new XmlReader [] { reader }, null, outputStream, true, null, null, null); } } ////// Compiles an Srgs documentto a file /// /// /// static public void Compile (SrgsDocument srgsGrammar, Stream outputStream) { Helpers.ThrowIfNull (srgsGrammar, "srgsGrammar"); Helpers.ThrowIfNull (outputStream, "outputStream"); SrgsCompiler.CompileStream (srgsGrammar, null, outputStream, true, null, null); } ////// Compiles a grammar to a file /// /// /// static public void Compile (XmlReader reader, Stream outputStream) { Helpers.ThrowIfNull (reader, "reader"); Helpers.ThrowIfNull (outputStream, "outputStream"); SrgsCompiler.CompileStream (new XmlReader [] { reader }, null, outputStream, true, null, null, null); } #if !NO_STG ////// Compiles a grammar to a file /// /// /// /// /// static public void CompileClassLibrary (string [] inputPaths, string outputPath, string [] referencedAssemblies, string keyFile) { Helpers.ThrowIfNull (inputPaths, "inputPaths"); Helpers.ThrowIfEmptyOrNull (outputPath, "outputPath"); XmlTextReader [] readers = new XmlTextReader [inputPaths.Length]; try { for (int iFile = 0; iFile < inputPaths.Length; iFile++) { if (inputPaths [iFile] == null) { throw new ArgumentException (SR.Get (SRID.ArrayOfNullIllegal), "inputPaths"); } readers [iFile] = new XmlTextReader (new Uri (inputPaths [iFile], UriKind.RelativeOrAbsolute).ToString ()); } SrgsCompiler.CompileStream (readers, outputPath, null, false, null, referencedAssemblies, keyFile); } finally { for (int iReader = 0; iReader < readers.Length; iReader++) { XmlTextReader srgsGrammar = readers [iReader]; if (srgsGrammar != null) { ((IDisposable) srgsGrammar).Dispose (); } } } } ////// Compiles an Srgs documentto a file /// /// /// /// /// static public void CompileClassLibrary (SrgsDocument srgsGrammar, string outputPath, string [] referencedAssemblies, string keyFile) { Helpers.ThrowIfNull (srgsGrammar, "srgsGrammar"); Helpers.ThrowIfEmptyOrNull (outputPath, "outputPath"); SrgsCompiler.CompileStream (srgsGrammar, outputPath, null, false, referencedAssemblies, keyFile); } ////// Compiles a grammar to a file /// /// /// /// /// static public void CompileClassLibrary (XmlReader reader, string outputPath, string [] referencedAssemblies, string keyFile) { Helpers.ThrowIfNull (reader, "reader"); Helpers.ThrowIfEmptyOrNull (outputPath, "outputPath"); SrgsCompiler.CompileStream (new XmlReader [] { reader }, outputPath, null, false, null, referencedAssemblies, keyFile); } #endif #endregion //******************************************************************** // // Internal Methods // //******************************************************************* #region Internal Methods // Decide if the input stream is a cfg. // If not assume it's an xml grammar. // The stream parameter points to the start of the data on entry and is reset to that point on exit. static private bool CheckIfCfg (Stream stream, out int cfgLength) { long initialPosition = stream.Position; bool isCfg = CfgGrammar.CfgSerializedHeader.IsCfg (stream, out cfgLength); // Reset stream position: stream.Position = initialPosition; return isCfg; } static internal void CompileXmlOrCopyCfg ( Stream inputStream, Stream outputStream, Uri orginalUri) { // Wrap stream in case Seek is not supported: SeekableReadStream seekableInputStream = new SeekableReadStream (inputStream); // See if CFG or XML document: int cfgLength; bool isCFG = CheckIfCfg (seekableInputStream, out cfgLength); seekableInputStream.CacheDataForSeeking = false; // Stop buffering data if (isCFG) { // Just copy the input to the output: // {We later check the header on the output stream - we could do it on the input stream but it may not be seekable}. Helpers.CopyStream (seekableInputStream, outputStream, cfgLength); } else { // Else compile the Xml: SrgsCompiler.CompileStream (new XmlReader [] { new XmlTextReader (seekableInputStream) }, null, outputStream, true, orginalUri, null, null); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
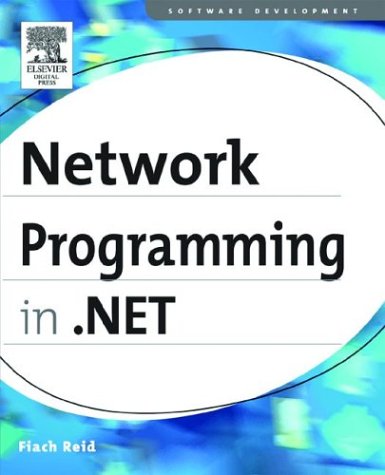
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PackagePartCollection.cs
- XmlResolver.cs
- SpanIndex.cs
- ApplicationCommands.cs
- ResizeGrip.cs
- xsdvalidator.cs
- ListBox.cs
- StreamHelper.cs
- ACE.cs
- HighlightComponent.cs
- CryptoConfig.cs
- Configuration.cs
- MultitargetingHelpers.cs
- CompositeClientFormatter.cs
- SkipStoryboardToFill.cs
- DesignerAttribute.cs
- UserNameSecurityTokenProvider.cs
- BamlResourceDeserializer.cs
- SqlConnectionPoolProviderInfo.cs
- NetStream.cs
- ContentOperations.cs
- ToolStripItemRenderEventArgs.cs
- RowUpdatingEventArgs.cs
- CompiledELinqQueryState.cs
- OleDbConnectionInternal.cs
- EncodingTable.cs
- PointLight.cs
- DataPager.cs
- SqlAliasesReferenced.cs
- LinearGradientBrush.cs
- MsmqAuthenticationMode.cs
- MetadataPropertyAttribute.cs
- VirtualDirectoryMapping.cs
- SqlVisitor.cs
- JavaScriptObjectDeserializer.cs
- DataPagerFieldItem.cs
- MethodSignatureGenerator.cs
- SimpleHandlerBuildProvider.cs
- DoubleConverter.cs
- OleDbConnectionInternal.cs
- TTSVoice.cs
- UInt64.cs
- Point3D.cs
- StagingAreaInputItem.cs
- shaperfactory.cs
- ControlCollection.cs
- COM2EnumConverter.cs
- UntypedNullExpression.cs
- ThicknessKeyFrameCollection.cs
- VoiceChangeEventArgs.cs
- BitmapFrame.cs
- BaseResourcesBuildProvider.cs
- CurrencyManager.cs
- PathBox.cs
- SemaphoreFullException.cs
- Pen.cs
- Model3DCollection.cs
- TraceLevelStore.cs
- InputMethodStateChangeEventArgs.cs
- LinkUtilities.cs
- HtmlShim.cs
- LabelDesigner.cs
- TextEndOfSegment.cs
- AtomEntry.cs
- WebPartCloseVerb.cs
- DecoratedNameAttribute.cs
- DbgCompiler.cs
- IntegerCollectionEditor.cs
- WindowsFormsHost.cs
- FrameworkPropertyMetadata.cs
- Membership.cs
- WindowsSolidBrush.cs
- HelpKeywordAttribute.cs
- AddInSegmentDirectoryNotFoundException.cs
- SessionStateItemCollection.cs
- IisTraceListener.cs
- QueryExpression.cs
- TextProperties.cs
- GetImportedCardRequest.cs
- InstanceDataCollection.cs
- SQLDecimal.cs
- SHA256Managed.cs
- Encoder.cs
- XmlSchemaSimpleTypeRestriction.cs
- WebMessageEncodingElement.cs
- DataSysAttribute.cs
- HoistedLocals.cs
- Tracer.cs
- AnnouncementDispatcherAsyncResult.cs
- EntityDataSourceSelectedEventArgs.cs
- PersonalizationStateInfo.cs
- TypeDescriptionProviderAttribute.cs
- _BufferOffsetSize.cs
- DataGridViewRowCancelEventArgs.cs
- DbConnectionPool.cs
- DataRelationPropertyDescriptor.cs
- BamlWriter.cs
- GenericEnumerator.cs
- MsmqTransportBindingElement.cs
- __ComObject.cs