Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Recognition / SrgsGrammar / SrgsSubset.cs / 1 / SrgsSubset.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // // History: // 5/1/2004 jeanfp Created from the Kurosawa Code //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Globalization; using System.Speech.Internal; using System.Speech.Internal.SrgsParser; using System.Xml; namespace System.Speech.Recognition.SrgsGrammar { /// TODOC <_include file='doc\Subset.uex' path='docs/doc[@for="Subset"]/*' /> // Note that currently if multiple words are stored in a Subset they are treated internally // and in the result as multiple tokens. [Serializable] [DebuggerDisplay ("{DebuggerDisplayString ()}")] public class SrgsSubset : SrgsElement, ISubset { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors /// TODOC public SrgsSubset (string text) : this (text, SubsetMatchingMode.Subsequence) { } /// TODOC public SrgsSubset (string text, SubsetMatchingMode matchingMode) { Helpers.ThrowIfEmptyOrNull (text, "text"); if (matchingMode != SubsetMatchingMode.OrderedSubset && matchingMode != SubsetMatchingMode.Subsequence && matchingMode != SubsetMatchingMode.OrderedSubsetContentRequired && matchingMode != SubsetMatchingMode.SubsequenceContentRequired) { throw new ArgumentException (SR.Get (SRID.InvalidSubsetAttribute), "matchingMode"); } _matchMode = matchingMode; _text = text.Trim (Helpers._achTrimChars); Helpers.ThrowIfEmptyOrNull (_text, "text"); } #endregion //******************************************************************** // // Public Properties // //******************************************************************* #region public Properties /// TODOC <_include file='doc\Subset.uex' path='docs/doc[@for="Subset.Text"]/*' /> public SubsetMatchingMode MatchingMode { get { return _matchMode; } set { if (value != SubsetMatchingMode.OrderedSubset && value != SubsetMatchingMode.Subsequence && value != SubsetMatchingMode.OrderedSubsetContentRequired && value != SubsetMatchingMode.SubsequenceContentRequired) { throw new ArgumentException (SR.Get (SRID.InvalidSubsetAttribute), "value"); } _matchMode = value; } } /// TODOC <_include file='doc\Subset.uex' path='docs/doc[@for="Subset.Pronunciation"]/*' /> public string Text { get { return _text; } set { Helpers.ThrowIfEmptyOrNull (value, "value"); value = value.Trim (Helpers._achTrimChars); Helpers.ThrowIfEmptyOrNull (value, "value"); _text = value; } } #endregion //******************************************************************** // // Internal methods // //******************************************************************** #region Internal methods internal override void WriteSrgs (XmlWriter writer) { // Write_text writer.WriteStartElement ("sapi", "subset", XmlParser.sapiNamespace); if (_matchMode != SubsetMatchingMode.Subsequence) { string sMatchMode = null; switch (_matchMode) { case SubsetMatchingMode.Subsequence: sMatchMode = "subsequence"; break; case SubsetMatchingMode.OrderedSubset: sMatchMode = "ordered-subset"; break; case SubsetMatchingMode.SubsequenceContentRequired: sMatchMode = "subsequence-content-required"; break; case SubsetMatchingMode.OrderedSubsetContentRequired: sMatchMode = "ordered-subset-content-required"; break; } writer.WriteAttributeString ("sapi", "match", XmlParser.sapiNamespace, sMatchMode); } // If an empty string is provided, skip the WriteString // to have the XmlWrite to putrather than if (_text != null && _text.Length > 0) { writer.WriteString (_text); } writer.WriteEndElement (); } internal override void Validate (SrgsGrammar grammar) { grammar.HasSapiExtension = true; base.Validate (grammar); } internal override string DebuggerDisplayString () { return _text + " [" + _matchMode.ToString () + "]"; } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields private SubsetMatchingMode _matchMode; private string _text; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // // History: // 5/1/2004 jeanfp Created from the Kurosawa Code //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Globalization; using System.Speech.Internal; using System.Speech.Internal.SrgsParser; using System.Xml; namespace System.Speech.Recognition.SrgsGrammar { /// TODOC <_include file='doc\Subset.uex' path='docs/doc[@for="Subset"]/*' /> // Note that currently if multiple words are stored in a Subset they are treated internally // and in the result as multiple tokens. [Serializable] [DebuggerDisplay ("{DebuggerDisplayString ()}")] public class SrgsSubset : SrgsElement, ISubset { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors /// TODOC public SrgsSubset (string text) : this (text, SubsetMatchingMode.Subsequence) { } /// TODOC public SrgsSubset (string text, SubsetMatchingMode matchingMode) { Helpers.ThrowIfEmptyOrNull (text, "text"); if (matchingMode != SubsetMatchingMode.OrderedSubset && matchingMode != SubsetMatchingMode.Subsequence && matchingMode != SubsetMatchingMode.OrderedSubsetContentRequired && matchingMode != SubsetMatchingMode.SubsequenceContentRequired) { throw new ArgumentException (SR.Get (SRID.InvalidSubsetAttribute), "matchingMode"); } _matchMode = matchingMode; _text = text.Trim (Helpers._achTrimChars); Helpers.ThrowIfEmptyOrNull (_text, "text"); } #endregion //******************************************************************** // // Public Properties // //******************************************************************* #region public Properties /// TODOC <_include file='doc\Subset.uex' path='docs/doc[@for="Subset.Text"]/*' /> public SubsetMatchingMode MatchingMode { get { return _matchMode; } set { if (value != SubsetMatchingMode.OrderedSubset && value != SubsetMatchingMode.Subsequence && value != SubsetMatchingMode.OrderedSubsetContentRequired && value != SubsetMatchingMode.SubsequenceContentRequired) { throw new ArgumentException (SR.Get (SRID.InvalidSubsetAttribute), "value"); } _matchMode = value; } } /// TODOC <_include file='doc\Subset.uex' path='docs/doc[@for="Subset.Pronunciation"]/*' /> public string Text { get { return _text; } set { Helpers.ThrowIfEmptyOrNull (value, "value"); value = value.Trim (Helpers._achTrimChars); Helpers.ThrowIfEmptyOrNull (value, "value"); _text = value; } } #endregion //******************************************************************** // // Internal methods // //******************************************************************** #region Internal methods internal override void WriteSrgs (XmlWriter writer) { // Write_text writer.WriteStartElement ("sapi", "subset", XmlParser.sapiNamespace); if (_matchMode != SubsetMatchingMode.Subsequence) { string sMatchMode = null; switch (_matchMode) { case SubsetMatchingMode.Subsequence: sMatchMode = "subsequence"; break; case SubsetMatchingMode.OrderedSubset: sMatchMode = "ordered-subset"; break; case SubsetMatchingMode.SubsequenceContentRequired: sMatchMode = "subsequence-content-required"; break; case SubsetMatchingMode.OrderedSubsetContentRequired: sMatchMode = "ordered-subset-content-required"; break; } writer.WriteAttributeString ("sapi", "match", XmlParser.sapiNamespace, sMatchMode); } // If an empty string is provided, skip the WriteString // to have the XmlWrite to putrather than if (_text != null && _text.Length > 0) { writer.WriteString (_text); } writer.WriteEndElement (); } internal override void Validate (SrgsGrammar grammar) { grammar.HasSapiExtension = true; base.Validate (grammar); } internal override string DebuggerDisplayString () { return _text + " [" + _matchMode.ToString () + "]"; } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields private SubsetMatchingMode _matchMode; private string _text; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
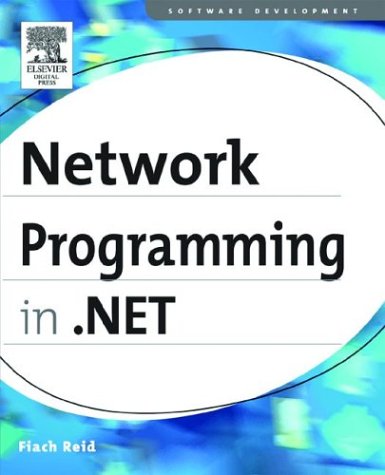
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HeaderedItemsControl.cs
- HasCopySemanticsAttribute.cs
- InfiniteTimeSpanConverter.cs
- Command.cs
- RijndaelManaged.cs
- FirewallWrapper.cs
- PersonalizableAttribute.cs
- BitSet.cs
- RequestResponse.cs
- ClientTargetSection.cs
- HttpServerUtilityBase.cs
- XmlQueryTypeFactory.cs
- CLSCompliantAttribute.cs
- SourceSwitch.cs
- _NestedMultipleAsyncResult.cs
- Delegate.cs
- DataBinding.cs
- XmlBinaryReader.cs
- ApplicationException.cs
- ObjectNotFoundException.cs
- CollectionDataContract.cs
- XmlSchemaAttribute.cs
- DataReaderContainer.cs
- ExclusiveHandle.cs
- Graphics.cs
- ProfileBuildProvider.cs
- HtmlInputReset.cs
- DocumentViewerHelper.cs
- PropertyEmitterBase.cs
- SqlCachedBuffer.cs
- MimeObjectFactory.cs
- ServiceHttpModule.cs
- TextServicesHost.cs
- SecurityState.cs
- AutoGeneratedFieldProperties.cs
- TemplateContainer.cs
- RuntimeConfigLKG.cs
- DebugController.cs
- PreviewPageInfo.cs
- ComUdtElementCollection.cs
- DetailsViewRow.cs
- LineInfo.cs
- CodeAttributeDeclaration.cs
- DocumentXmlWriter.cs
- FillRuleValidation.cs
- ServiceOperation.cs
- RSAPKCS1SignatureFormatter.cs
- DataGridViewSortCompareEventArgs.cs
- Publisher.cs
- UnsignedPublishLicense.cs
- Vector3DAnimation.cs
- WorkItem.cs
- RadioButtonFlatAdapter.cs
- PrintPreviewControl.cs
- MembershipUser.cs
- CellQuery.cs
- BoundField.cs
- handlecollector.cs
- NetworkCredential.cs
- SystemGatewayIPAddressInformation.cs
- SqlFunctionAttribute.cs
- AspProxy.cs
- TaskFileService.cs
- PKCS1MaskGenerationMethod.cs
- SerializationSectionGroup.cs
- EDesignUtil.cs
- ConstructorBuilder.cs
- BinaryFormatterWriter.cs
- ResourceWriter.cs
- CommandHelpers.cs
- DataError.cs
- Tag.cs
- TransactionCache.cs
- TextBoxView.cs
- MethodAccessException.cs
- Vector3DCollectionValueSerializer.cs
- SqlDataSourceAdvancedOptionsForm.cs
- DataRowExtensions.cs
- InkSerializer.cs
- RawStylusInputCustomData.cs
- XamlFigureLengthSerializer.cs
- InputReferenceExpression.cs
- TextParaClient.cs
- TypeSemantics.cs
- AdditionalEntityFunctions.cs
- safesecurityhelperavalon.cs
- ListViewDataItem.cs
- PasswordDeriveBytes.cs
- HttpResponse.cs
- HelpPage.cs
- DBConcurrencyException.cs
- HttpPostedFile.cs
- FixedDocument.cs
- HtmlForm.cs
- XPathBuilder.cs
- SoapWriter.cs
- TabControlDesigner.cs
- TraceUtility.cs
- TablePattern.cs
- StorageMappingItemLoader.cs