Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / CompMod / System / ComponentModel / Design / DateTimeEditor.cs / 1 / DateTimeEditor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel.Design { using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using Microsoft.Win32; using System.Drawing; using System.Drawing.Design; using System.Windows.Forms; using System.Windows.Forms.Design; ////// /// /// public class DateTimeEditor : UITypeEditor { ////// This date/time editor is a UITypeEditor suitable for /// visually editing DateTime objects. /// ////// /// Edits the given object value using the editor style provided by /// GetEditorStyle. A service provider is provided so that any /// required editing services can be obtained. /// [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] // everything in this assembly is full trust. public override object EditValue(ITypeDescriptorContext context, IServiceProvider provider, object value) { object returnValue = value; if (provider != null) { IWindowsFormsEditorService edSvc = (IWindowsFormsEditorService)provider.GetService(typeof(IWindowsFormsEditorService)); if (edSvc != null) { using (DateTimeUI dateTimeUI = new DateTimeUI()) { dateTimeUI.Start(edSvc, value); edSvc.DropDownControl(dateTimeUI); value = dateTimeUI.Value; dateTimeUI.End(); } } } return value; } ////// /// Retrieves the editing style of the Edit method. If the method /// is not supported, this will return None. /// [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] // everything in this assembly is full trust. public override UITypeEditorEditStyle GetEditStyle(ITypeDescriptorContext context) { return UITypeEditorEditStyle.DropDown; } ////// /// UI we drop down to pick dates. /// private class DateTimeUI : Control { private MonthCalendar monthCalendar = new DateTimeMonthCalendar(); private object value; private IWindowsFormsEditorService edSvc; ////// /// public DateTimeUI() { InitializeComponent(); Size = monthCalendar.SingleMonthSize; monthCalendar.Resize += new EventHandler(this.MonthCalResize); } ////// /// public object Value { get { return value; } } ////// /// public void End() { edSvc = null; value = null; } private void MonthCalKeyDown(object sender, KeyEventArgs e) { switch (e.KeyCode) { case Keys.Enter: OnDateSelected(sender, null); break; } } ////// /// private void InitializeComponent() { monthCalendar.DateSelected += new DateRangeEventHandler(this.OnDateSelected); monthCalendar.KeyDown += new KeyEventHandler(this.MonthCalKeyDown); this.Controls.Add(monthCalendar); } private void MonthCalResize(object sender, EventArgs e) { this.Size = monthCalendar.Size; } ////// /// private void OnDateSelected(object sender, DateRangeEventArgs e) { value = monthCalendar.SelectionStart; edSvc.CloseDropDown(); } protected override void OnGotFocus(EventArgs e) { base.OnGotFocus(e); monthCalendar.Focus(); } ////// /// public void Start(IWindowsFormsEditorService edSvc, object value) { this.edSvc = edSvc; this.value = value; if (value != null) { DateTime dt = (DateTime) value; monthCalendar.SetDate((dt.Equals(DateTime.MinValue)) ? DateTime.Today : dt); } } class DateTimeMonthCalendar : MonthCalendar { protected override bool IsInputKey(System.Windows.Forms.Keys keyData) { switch (keyData) { case Keys.Enter: return true; } return base.IsInputKey(keyData); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
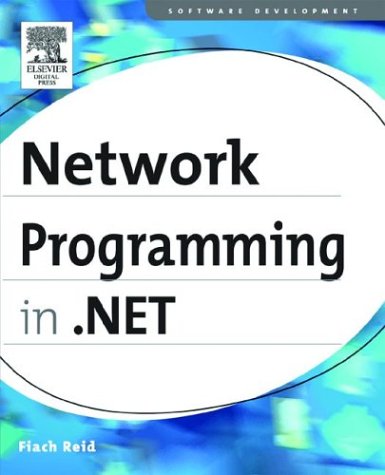
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Command.cs
- ParameterBuilder.cs
- RoutedUICommand.cs
- PersistenceContextEnlistment.cs
- FileDialog_Vista.cs
- SelectorItemAutomationPeer.cs
- TextRangeSerialization.cs
- AuthenticationSchemesHelper.cs
- PolicyStatement.cs
- MethodToken.cs
- SchemaManager.cs
- CallContext.cs
- AsyncResult.cs
- PropertySourceInfo.cs
- WebPartDescription.cs
- AggregationMinMaxHelpers.cs
- AmbientLight.cs
- TextSearch.cs
- CacheAxisQuery.cs
- errorpatternmatcher.cs
- HtmlTableRowCollection.cs
- IISUnsafeMethods.cs
- KeyedHashAlgorithm.cs
- ServicesUtilities.cs
- SizeAnimationClockResource.cs
- ImplicitInputBrush.cs
- WebAdminConfigurationHelper.cs
- Content.cs
- SQLGuid.cs
- LogPolicy.cs
- HtmlForm.cs
- QuadraticBezierSegment.cs
- CodeTypeParameterCollection.cs
- ReflectionTypeLoadException.cs
- LockRenewalTask.cs
- SequenceFullException.cs
- EntityTypeEmitter.cs
- XamlBrushSerializer.cs
- ConnectionsZone.cs
- WebHttpBinding.cs
- BaseCollection.cs
- JoinCqlBlock.cs
- VisualStyleRenderer.cs
- EventProperty.cs
- CatalogZone.cs
- WebPartExportVerb.cs
- IApplicationTrustManager.cs
- SettingsAttributes.cs
- SmuggledIUnknown.cs
- adornercollection.cs
- UTF32Encoding.cs
- IIS7UserPrincipal.cs
- MessageSecurityOverMsmqElement.cs
- ToolboxComponentsCreatingEventArgs.cs
- ScrollBarRenderer.cs
- HTTPNotFoundHandler.cs
- DiscoveryClientDocuments.cs
- SourceSwitch.cs
- XmlQualifiedName.cs
- VirtualPath.cs
- XmlJsonReader.cs
- Int32AnimationBase.cs
- _NestedMultipleAsyncResult.cs
- Dispatcher.cs
- Adorner.cs
- SafeEventLogReadHandle.cs
- DynamicDataResources.Designer.cs
- ManagedIStream.cs
- SystemKeyConverter.cs
- DataGridCaption.cs
- WebSysDefaultValueAttribute.cs
- ResourcesBuildProvider.cs
- TextSchema.cs
- XmlCharType.cs
- LinkConverter.cs
- RegexWriter.cs
- TdsParserSessionPool.cs
- XamlTemplateSerializer.cs
- SparseMemoryStream.cs
- MouseButton.cs
- XMLSchema.cs
- DnsEndpointIdentity.cs
- WebRequest.cs
- FontStyle.cs
- Ref.cs
- RealizationDrawingContextWalker.cs
- WebResourceAttribute.cs
- XmlReaderSettings.cs
- Internal.cs
- Evidence.cs
- DataSourceCacheDurationConverter.cs
- RouteValueDictionary.cs
- MetafileHeader.cs
- DataGridViewRowHeaderCell.cs
- SymbolEqualComparer.cs
- SiteOfOriginContainer.cs
- BinaryObjectWriter.cs
- EntityParameterCollection.cs
- PrinterResolution.cs
- Rotation3DAnimationUsingKeyFrames.cs